股票买卖

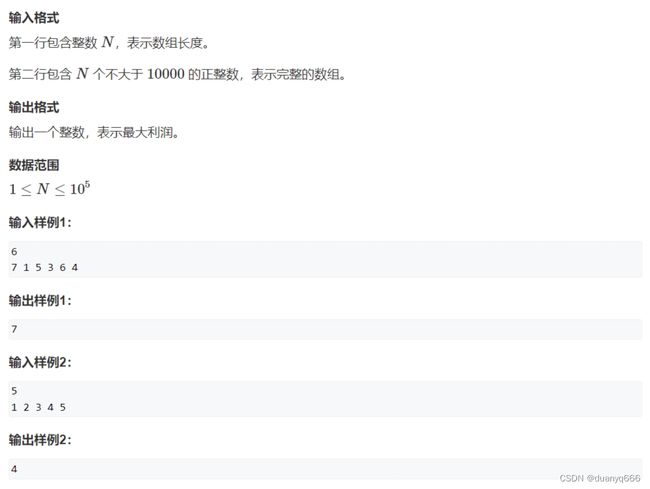
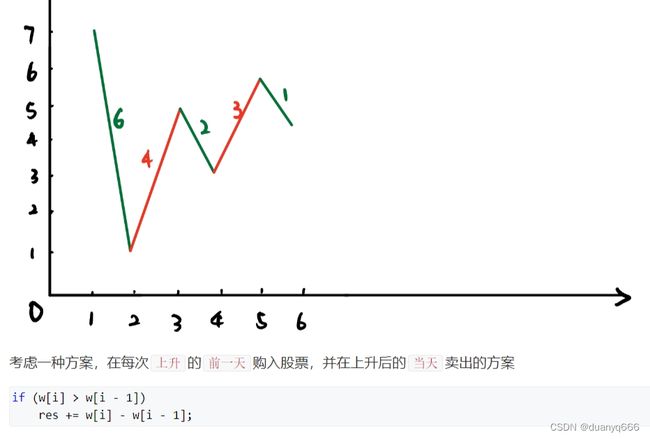
低买高卖
#include
using namespace std;
const int N = 1e5 + 10;
int a[N];
int n, res;
int main(){
cin>>n;
for(int i = 0; i < n; i++){
cin>>a[i];
}
for(int i = 1; i < n; i++){
if(a[i] > a[i - 1]){
res += a[i] - a[i - 1];
}
}
cout<<res;
return 0;
}
防晒
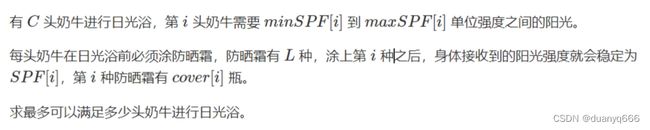
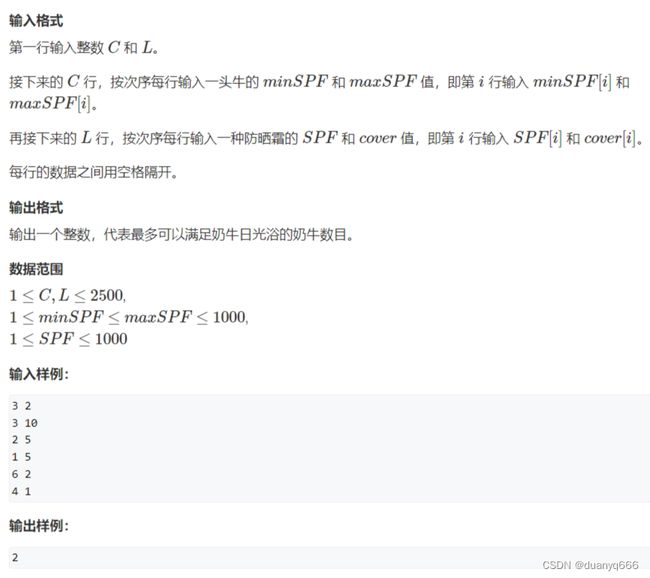
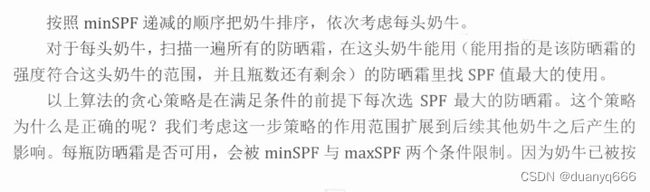
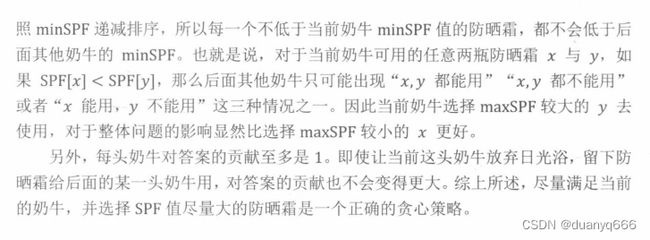
#include
#include
using namespace std;
const int N = 2510;
pair<int, int> a[N], b[N];
int c, l;
int ans;
bool cmp(const pair<int, int> p, const pair<int, int> q){
return p.first > q.first;
}
int main(){
cin>>c>>l;
int x, y;
for(int i = 0; i < c; i++){
cin>>x>>y;
a[i] = make_pair(x, y);
}
sort(a, a + c, cmp);
for(int i = 0; i < l; i++){
cin>>x>>y;
b[i] = make_pair(x, y);
}
sort(b, b + l, cmp);
for(int i = 0; i < c; i++){
for(int j = 0; j < l; j++){
if(a[i].first <= b[j].first && a[i].second >= b[j].first && b[j].second){
ans++;
b[j].second--;
break;
}
}
}
cout<<ans;
return 0;
}
畜栏预定
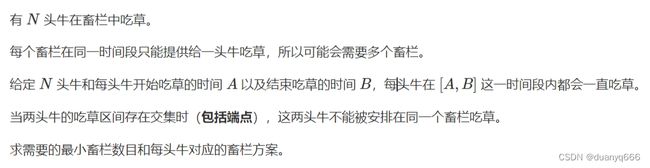
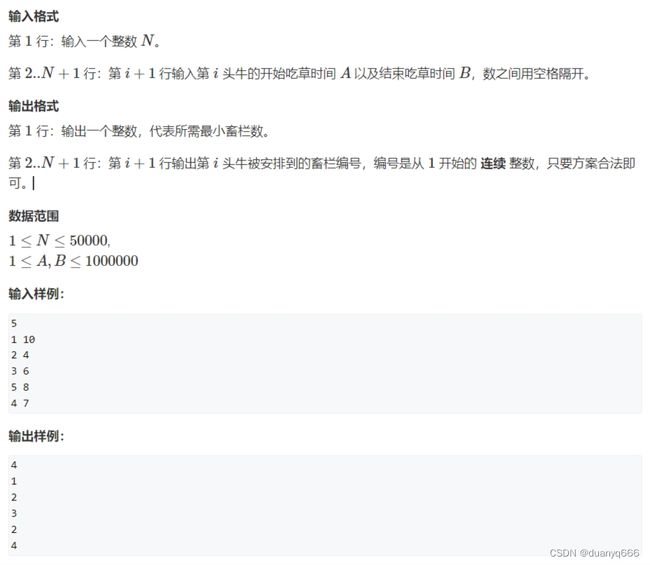
#include
#include
#include
using namespace std;
const int N = 5e4 + 10;
pair<pair<int, int>, int> a[N];
int id[N];
int n;
bool cmp(const pair<pair<int, int>, int> p, const pair<pair<int, int>, int> q){
return p.first.first < q.first.first;
}
int main(){
cin>>n;
int x, y;
for(int i = 1; i <= n; i++){
cin>>x>>y;
a[i] = make_pair(make_pair(x, y), i);
}
sort(a + 1, a + n + 1, cmp);
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> h;
for(int i = 1; i <= n; i++){
if(h.empty() || h.top().first >= a[i].first.first){
pair<int, int> t = make_pair(a[i].first.second, h.size() + 1);
id[a[i].second] = t.second;
h.push(t);
}else{
pair<int, int> t = h.top();
h.pop();
t.first = a[i].first.second;
id[a[i].second] = t.second;
h.push(t);
}
}
cout<<h.size()<<endl;
for(int i = 1; i <= n; i++){
cout<<id[i]<<endl;
}
return 0;
}
雷达设备
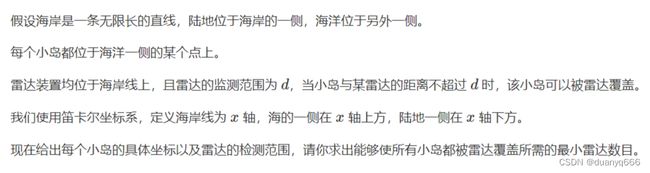
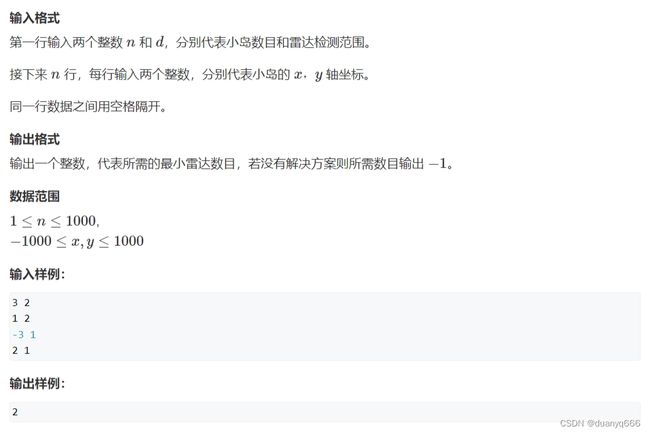
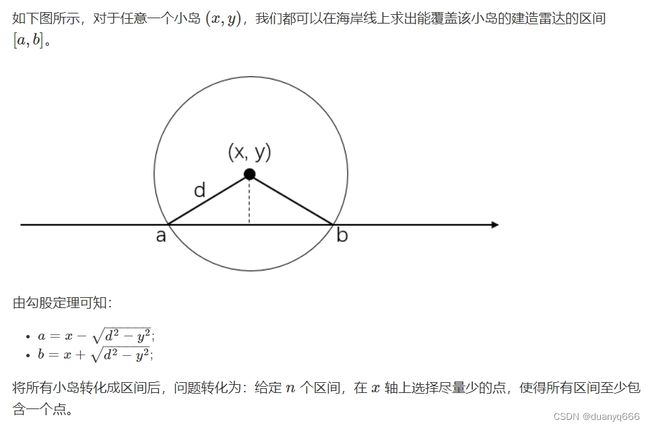
#include
#include
#include
using namespace std;
const int N = 1e3 + 10;
pair<double, double> a[N], b[N];
int n, d;
int res;
int main(){
cin>>n>>d;
double x, y;
for(int i = 0; i < n; i++){
cin>>x>>y;
a[i] = make_pair(x, y);
}
for(int i = 0; i < n; i++){
if(a[i].second > d){
cout<<-1;
return 0;
}else{
x = a[i].first, y = a[i].second;
double z = sqrt(d * d - y * y);
b[i] = make_pair(x - z, x + z);
}
}
sort(b, b + n);
double ed = -1e9;
for(int i = 0; i < n; i++){
if(b[i].first > ed){
res++;
ed = b[i].second;
}else{
ed = min(ed, b[i].second);
}
}
cout<<res;
return 0;
}
#include
#include
#include
using namespace std;
const int N = 1e3 + 10;
pair<double, double> a[N], b[N];
int n, d;
int res;
int main(){
cin>>n>>d;
double x, y;
for(int i = 0; i < n; i++){
cin>>x>>y;
a[i] = make_pair(x, y);
}
for(int i = 0; i < n; i++){
if(a[i].second > d){
cout<<-1;
return 0;
}else{
x = a[i].first, y = a[i].second;
double z = sqrt(d * d - y * y);
b[i] = make_pair(x + z, x - z);
}
}
sort(b, b + n);
double ed = -1e9;
for(int i = 0; i < n; i++){
if(b[i].second > ed){
res++;
ed = b[i].first;
}
}
cout<<res;
return 0;
}
国王游戏
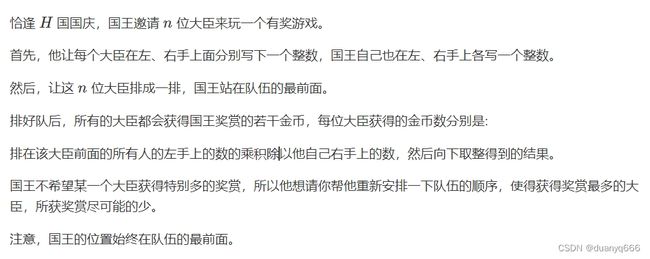
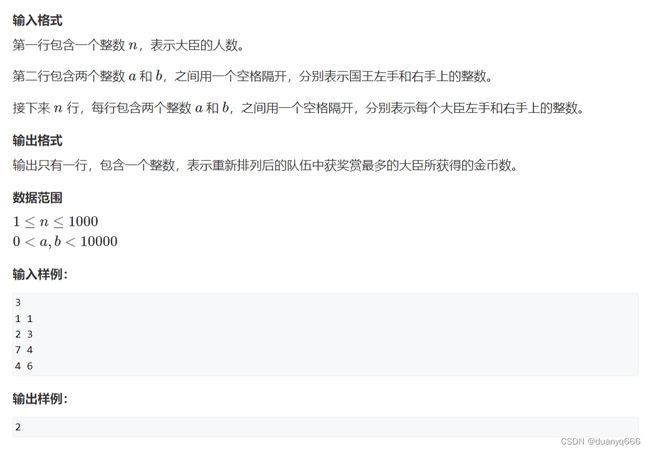
#include
#include
using namespace std;
const int N = 1e4 + 10;
pair<int, int> res[N];
int n, ans;
bool cmp(const pair<int, int> p, const pair<int, int> q){
return p.first * p.second < q.first * q.second;
}
int main(){
cin>>n;
int x, y;
for(int i = 0; i <= n; i++){
cin>>x>>y;
res[i] = make_pair(x, y);
}
sort(res + 1, res + n + 1, cmp);
for(int i = 1; i <= n; i++){
int ans1 = 1, ans2;
for(int j = 0; j < i; j++){
ans1 *= res[j].first;
}
ans2 = ans1 / res[i].second;
ans = max(ans, ans2);
}
cout<<ans;
return 0;
}
#include
#include
#include
using namespace std;
int v, Max;
int n;
int A, B;
int ans[40000001], len;
void mul(int x)
{
for (int i = 1; i <= len; i++)
ans[i] *= x;
for (int i = 1; i <= len + 15; i++)
{
ans[i + 1] += ans[i] / 10;
ans[i] %= 10;
}
for (int i = len + 15; i >= len; i--)
if (ans[i] != 0)
len = i;
return;
}
void div(int x)
{
int cnt = 0;
for (int i = len; i >= 1; i--)
{
cnt = cnt * 10 + ans[i];
if (cnt < x)
{
ans[i] = 0;
continue;
}
ans[i] = cnt / x;
cnt %= x;
}
for (int i = len; i >= 1; i--)
if (ans[i] != 0)
{
len = i;
break;
}
return;
}
int main()
{
cin >> n;
ans[1] = 1; len = 1;
for (int i = 1; i <= n + 1; i++)
{
int a, b;
cin >> a >> b;
mul(a);
v = a * b;
if (v > Max && i != 1)
Max = v, B = b, A = a;
}
div(A * B);
if (ans[len] == 0)
ans[len] = 1;
for (int i = len; i >= 1; i--)
cout << ans[i];
cout << endl;
return 0;
}
给树染色
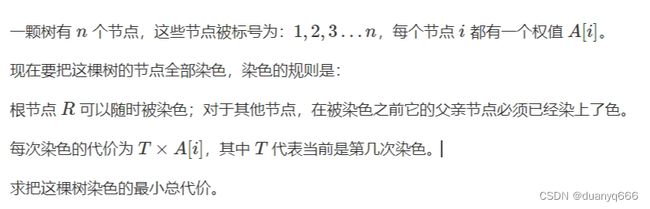
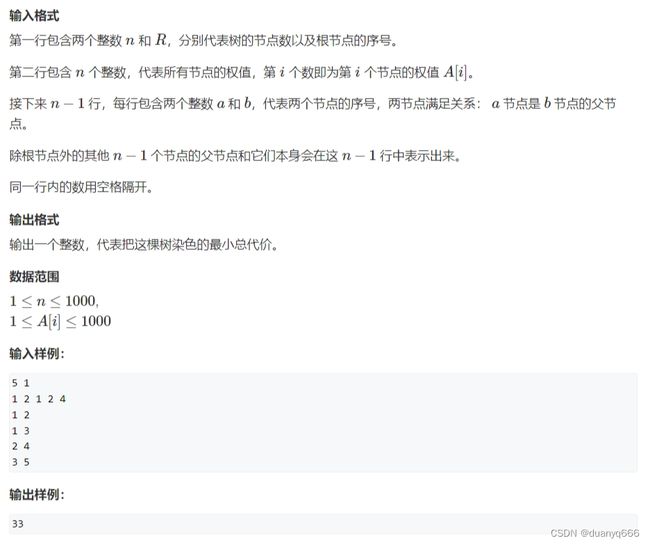
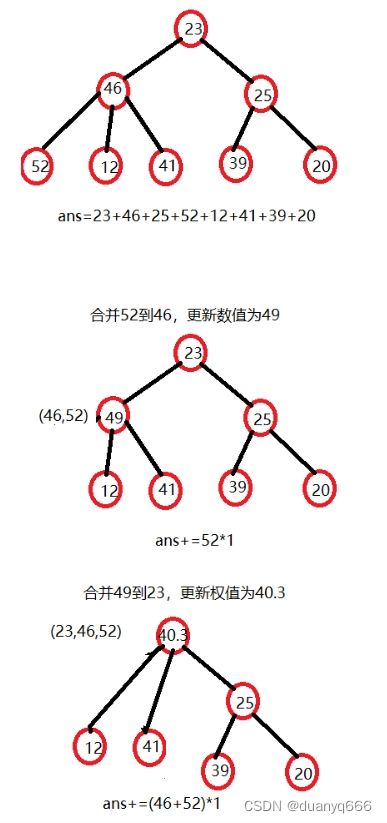
#include
using namespace std;
const int N = 1e3 + 10;
struct Node{
int fa, cnt, sum;
double avg;
}nodes[N];
int n, root;
int find(){
int res = -1;
double avg = 0;
for(int i = 1; i <= n; i++){
if(i != root && nodes[i].avg > avg){
avg = nodes[i].avg;
res = i;
}
}
return res;
}
int main(){
cin>>n>>root;
int res = 0;
for(int i = 1; i <= n; i++){
auto &t = nodes[i];
cin>>t.sum;
t.cnt = 1;
t.avg = t.sum;
res += t.sum;
}
int x, y;
for(int i = 0; i < n - 1; i++){
cin>>x>>y;
nodes[y].fa = x;
}
for(int i = 0; i < n - 1; i++){
int u = find();
int f = nodes[u].fa;
res += nodes[u].sum * nodes[f].cnt;
nodes[u].avg = -1;
for(int j = 1; j <= n; j++){
if(nodes[j].fa == u){
nodes[j].fa = f;
}
}
nodes[f].sum += nodes[u].sum;
nodes[f].cnt += nodes[u].cnt;
nodes[f].avg = (double)nodes[f].sum / nodes[f].cnt;
}
cout<<res;
return 0;
}