类拟框架:Apache OJB,JDO,Toplink,EJB(CMP)JPA,IBatis
适合查询及单个对象的编辑,适合于对象之间有清晰的关系,不适用于批量修改,关系复杂的对象及特定的sql功能
第一个项目
1 新建java项目
2 创建User Library,加入如下jar
* hibernate_home/hibernate3.jar
* hibernate_home/lib/*.jar
* MySql jdbc验动
3 创建hibernate配置文件hibernate.cfg.xml,为了便于调试最好加入log4j配置文件
<
hibernate-configuration
>
<
session-factory
>
<!--
连接串
-->
<
property
name
="hibernate.connection.url"
>
jdbc:mysql://localhost/hibernate1
</
property
>
<!--
驱动类
-->
<
property
name
="hibernate.connection.driver_class"
>
com.mysql.jdbc.Driver
</
property
>
<!--
用户名
-->
<
property
name
="hibernate.connection.username"
>
root
</
property
>
<!--
密码
-->
<
property
name
="hibernate.connection.password"
>
root
</
property
>
<!--
适配器(反译)
-->
<
property
name
="hibernate.dialect"
>
org.hibernate.dialect.MySQLDialect
</
property
>
<
property
name
="hibernate.dialect"
>
org.hibernate.dialect.SQLServerDialect
</
property
>
<!--
显示sql语句
-->
<
property
name
="hibernate.show_sql"
>
true
</
property
>
<!--
可以防表被重新建立
-->
<
property
name
="hibernate.hbm2ddl.auto"
>
update
</
property
>

<
mapping
resource
="com/myobj/hibername/User.hbm.xml"
/>
</
session-factory
>
</
hibernate-configuration
>
4 定义实体类,继承java.io.ser
5 定义User类的映射文件User.hbm.xml
<
hibernate-mapping
>
<
class
name
="com.myobj.hibername.User"
table
=""
>
<
id
name
="id"
>
<
generator
class
="uuid"
/>
<
generator
class
="native"
/>
<
generator
class
="assigned"
/>
</
id
>
<
property
name
="name"
/>
<
property
name
="password"
/>
<
property
name
="createTime"
/>
<
property
name
="expireTime"
/>
</
class
>
</
hibernate-mapping
>
6 将User.hbm.xml文件加入到hibernate.cfg.xml文件中
<
session-factory
>
<
property
name
="hibernate.connection.url"
>
jdbc:mysql://localhost/hibernate1
</
property
>
<
property
name
="hibernate.connection.driver_class"
>
com.mysql.jdbc.Driver
</
property
>
<
property
name
="hibernate.connection.username"
>
root
</
property
>
<
property
name
="hibernate.connection.password"
>
root
</
property
>
<
property
name
="hibernate.dialect"
>
org.hibernate.dialect.MySQLDialect
</
property
>
<
mapping
resource
="com/myobj/hibername/User.hbm.xml"
/>
</
session-factory
>
7 编写hbm2ddl工具类,将实体类生成数据库表,手工建数据库
public
class
ExportDB
{

public static void main(String[] args)
{
//读取hibernate.cfg.xml文件,默认为.properties文件,
//读取xml文件用new Configuration().configure()
Configuration cfg=new Configuration().configure();
//生成工具类
SchemaExport export=new SchemaExport(cfg);
//生成数据库表
export.create(true, true);
}
}
8 开发客户端Client类添加数据
public
class
Client
{


public static void main(String[] args)
{
//读取hibernate.cfg.xml文件
Configuration cfg=new Configuration().configure();
//创建SessionFactory,与数据库绑定,一个数据库对应一个SessionFactory,与二级缓存相关,为重量级对象,是线程安全的
SessionFactory factory=cfg.buildSessionFactory();
//
Session session=null;//不同于connction,是对其的封装,用时到连接池拿来conn

try
{
session = factory.openSession();
//开启事务
session.beginTransaction();
User user = new User();
user.setName("张三");
user.setPassword("123");
user.setCreateTime(new Date());
user.setExpireTime(new Date());
//保存数据
session.save(user);
//提交事务
session.getTransaction().commit();

} catch (Exception e)
{
e.printStackTrace();
//回滚事务
session.getTransaction().rollback();

}finally
{

if(session!=null)
{

if(session.isOpen())
{
//关闭session,是非线程安程的
session.close();
}
}
}
}
}
******session.update()相关类都改,session.merge()只改当前类,session.delete(),都要开启事务******
9 为了方便跟踪SQL执行,在hibernate.cfg.xml中加入
<property name="hibernate.show_sql">true</property>
持久化对象的状态
1 瞬时对象:使用new操作符初始化的对象不是立刻就持久的。
2 持久化对象:持久实例是任何具有数据库标识的实便函。
3 离线对象:Session关闭后持久化对象就变为离线对名象。
持久化对象的生命周期:
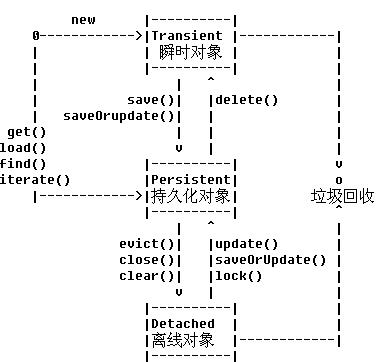
Transient对象new但在数据库中没有记录且没有被Session管理,
Persistent对象数据库中有记录,并Session在管理。在清理缓存(或脏数据检查)时与数据库同步。Session与一级缓存绑定.
Detached对象数据库中有记录,Session没有管理它。
创建HibernateUtils类,对重量级容量进行优化:
public
class
HibernateUtils
{
private static SessionFactory factory;
//static块只执行一次

static
{

try
{
Configuration cfg = new Configuration().configure();

} catch (Exception e)
{
e.printStackTrace();
}
}


public static SessionFactory getSessionFactory()
{
return factory;
}

public static Session getSession()
{
return factory.openSession();
}

public static void closeSession(Session session)
{

if(session!=null)
{

if(session.isOpen())
{
session.close();
}
}
}
}
Session接口的CRUD操作
瞬时/持久化/离线对象示例:
public
class
SessionTest
extends
TestCase
{

public void testSave1()
{
Session session=null;
Transaction tx=null;
User user=null;

try
{
session=HibernateUtils.getSession();
tx=session.beginTransaction();
//Transient状态,未被Session管理
user=new User();
user.setName("ssssss");
user.setPassword("123");
user.setCreateTime(new Date());
user.setExpireTime(new Date());
//persistent状态,被Session管理,当属性发生改变的时候,hibernate会自动和数据库同步。
session.save(user);
user.setName("王五");
tx.commit();

}catch(Exception e)
{
e.printStackTrace();
tx.rollback();

}finally
{
HibernateUtils.closeSession(session);
}
//detached状态,被Session踢出缓存,未被管理
user.setName("张三");

try
{
session = HibernateUtils.getSession();
session.beginTransaction();
session.update(user);
session.getTransaction().commit();

} catch (Exception e)
{
e.printStackTrace();
session.getTransaction().rollback();

}finally
{
HibernateUtils.closeSession(session);
}
}
}
Session接口
加载数据get与load的区别:
lazy代理:get没有, load支持lazy;
查不存在数据:get返回null,load抛出异常ObjectNotFoundException
//
get查询数据

public
void
testReadByGetMethod1()
{
Session session=null;

try
{
session=HibernateUtils.getSession();
session.beginTransaction();
//采用get方式,马上发出查询sql,加载波User对象
User user=(User)session.get(User.class, "5c68c3ed206a327d01206a3281fa0001");
http://www.blogjava.net/Images/OutliningIn
分享到:
评论