- golang 加密
大鲤余
Golanggolang开发语言后端
代码示例packageutilsimport("crypto/md5""encoding/hex""golang.org/x/crypto/bcrypt")//BcryptHash使用bcrypt对数据进行加密funcBcryptHash(passwordstring)string{bytes,_:=bcrypt.GenerateFromPassword([]byte(password),bcry
- TLS/SSL工作原理
penrryw
信息安全
HTTPS协议的主要功能基本都依赖于TLS/SSL协议,本节分析TLS/SSL协议工作原理。TLS/SSL的功能实现主要依赖于三类基本算法:散列函数Hash、对称加密和非对称加密,其利用非对称加密实现身份认证和密钥协商,对称加密算法采用协商的密钥对数据加密,基于散列函数验证信息的完整性。散列函数Hash常见的有MD5、SHA1、SHA256,该类函数特点是函数单向不可逆、对输入非常敏感、输出长度固
- 报文加密的对称方式和非对称分享
zw3e
我们和多部门之间联调经常需要涉及报文加解密的场景,梳理出一部分常用的加解密实用文档供参考加密算法(DES,AES,RSA,MD5,SHA1,Base64)比较和项目应用加密技术通常分为两大类:"对称式"和"非对称式"。对称性加密算法:对称式加密就是加密和解密使用同一个密钥。信息接收双方都需事先知道密匙和加解密算法且其密匙是相同的,之后便是对数据进行加解密了。对称加密算法用来对敏感数据等信息进行加密
- 查看android keystore的md5以及其他信息的命令
前方路远
服务器运维
keytool-list-v-keystorexxx.keystorekeytool-exportcert-keystorexxx.keystore|openssldgst-md5
- 在Linux/Ubuntu/Debian中计算MD5,SHA256的方法
理工男老K
计算机相关linuxubuntudebianmd5sha256
MD5(消息摘要算法5)和SHA-256(安全哈希算法256位)等流行的哈希算法广泛用于从任意数据生成固定大小的哈希值或校验和。以下是这些算法及其计算方式的简要概述:MD5(消息摘要算法5):算法:MD5是一种广泛使用的加密哈希函数,可生成128位(16字节)哈希值,通常表示为32个字符的十六进制数。Linux/Unix中的计算:md5sumyour_file此命令计算指定文件的MD5哈希值。SH
- 从0开始python学习-54.python中flask创建MD5和base64加密校验的接口
不会代码的小测试
pythonpython学习flask测试工具开发语言后端
MD5加密接口importhashlibfromflaskimportFlask,request,jsonify#初始化一个flask的对象app=Flask(__name__)#MD5加密校验数据请求#定义用户数据user_data=[{"username":"admin1","password":"E10ADC3949BA59ABBE56E057F20F883E"},#123456{"user
- 【国产化】禁止使用不安全的密码算法:DES、RC2,RSA(1024位及以下),MD5,SHA1
python
一、引言随着互联网的普及和技术的发展,网络安全问题日益严重。密码算法作为网络安全的基石,其安全性直接关系到用户数据的安全。一些不安全的密码算法不断被曝光,给用户带来了极大的安全隐患。二、不安全的密码算法1.DESDES(DataEncryptionStandard)是一种对称加密算法,自1977年发布以来,一直是全球最广泛使用的加密算法之一。然而,随着计算机处理能力的提高,DES的密钥长度(56位
- BUUCTF misc 专题(30)webshell后门
tt_npc
python网络安全安全
看题目,又是一道后门查杀的题目,下载了资源以后我们发现是一个rar的文件解压里面的文件后打开D盾进行后门查杀在这里找到了,并根据路径打开所在的文件夹,找到文件根据题目提示,我们在这里找到了md5再根据md5中的pass我们可以找到pass包上flag就是答案了flag{ba8e6c6f35a53933b871480bb9a9545c}
- 查看文件的md5值
chen_znn
Linux系统linuxmacoswindowsmd5
MD5的定义MD5(MessageDigestAlgorithm5)是一种常用的哈希函数算法,用于生成数据的唯一标识。它接受任意长度的输入数据,并将其转换为固定长度的哈希值(通常为128位),该哈希值被称为MD5值。MD5算法经常用于验证数据的完整性和一致性。当两个文件的MD5值相同时,可以非常高的概率认为这两个文件是相同的。即使文件只有微小的改动,其生成的MD5值也会有较大的差异。查看文件的MD
- 检查两个文件是否一样,可以通过比较两个文件的md5值
早起早起早起up
1.计算文件的md5,SHA1查看传输过程中有没有修改检查两个文件是否一样,可以通过比较两个文件的md5值(后续可以用这个方法来检验kevin.sql文件是否被修改)。(1).windows查看方式certutil-hashfileD:\BaiduNetdiskDownload\coding-265-master.zipMD5压缩文件查看(2)linux方式catkevin.sql.md5
- mysql xtrabackup
SkTj
1、安装rpm-ivhlibev-4.15-1.el6.rf.x86_64.rpmyuminstallperl-DBIyum-yinstallperlperl-devellibaiolibaio-develperl-Time-HiResperl-DBD-MySQLyum-yinstallperl-Digest-MD5wgethttps://www.percona.com/downloads/Xtr
- 某婚恋App _t 签名分析
fenfei331
一、目标最近也不让加班了,李老板每天早早的就回家,小视频也刷的没意思了。还是好好找个mm正经聊聊吧。今天我们的目标是某婚恋App的v11.3.2。二、步骤抓个包main.png_t参数,看上去像是时间戳加上一个md5(掰指头数了数,一共32位)。jadx搜一搜_t,我去,10几万条结果。一时激动,都忘了我的独门秘籍了。这种签名一般会以字符串的方式存入一个map。所以我们应该搜索"_t"find.p
- python计算md5值
夏2018
#计算字符串md5值defget_str_md5(str):myMd5=hashlib.md5()myMd5.update(str)myMd5_Digest=myMd5.hexdigest()returnmyMd5_Digest#计算文件md5值defget_md5(file_name):withopen(file_name)asfile_to_check:data=file_to_check.r
- nuscenes数据集下载脚本
端木的AI探索屋
nuscenes数据集下载bevmd5自动驾驶
目录一、介绍二、Nuscenes数据集准备工作2.1登录账号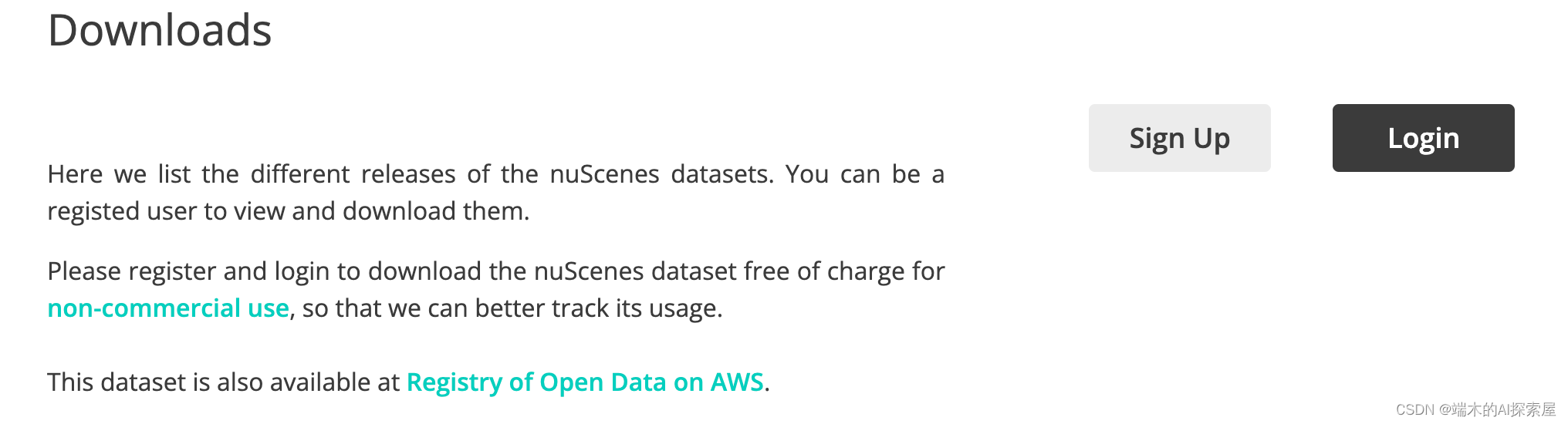2.2复制下载链接的方法三、脚本3.1下载脚本3.2验证md53.3解压所有文件一、介绍Nuscenes数据集过于庞大,且下载链接不断变化。网络上有很多资源已经把Nusc
- Hbase - 自定义Rowkey规则
kikiki5
>在Flink中我们有时候需要分析数据1点到2点的范围,可是经过Region又比较慢,这时候我们就可以定制`TableInputFormat`来实现我们的需求了,我们还可以采用Flink的`DataSet`的方式读取,另外下面还有`Spark`读取的例子。##使用教程Md5Util.java```importorg.apache.commons.codec.binary.Hex;importjav
- python3爬虫--入门篇3--url去重策略
布口袋_天晴了
1.访问过的url保存到数据库中[频繁存取,时间消耗高]2.将访问过的url保存到set中,只需要o(1)的代价[内存的占用量会较高]3.url经过md5等哈希后保存到set中。[Scrapy默认采用md5方法压缩url的,内存占用会大大减小]4.用bitmap方法,将访问过的url通过hash函数映射到某一位[压缩更多,极大节省内存,但哈希冲突的可能性还是比较大]5.bloomfilter方法对
- [BJDCTF2020]EasySearch1 不会编程的崽
不会编程的崽
安全网络安全web安全
先来看看界面源代码,抓包似乎都没什么线索,sql注入没什么反应。用dirsearch扫描3遍也没什么结果。。。最后使用dirmap才发现了网站源码文件。真够隐蔽的。index.php.swp源码也很简单,大概分为两层意思1.username存在即可,password经过md5加密后的前6位必须是6d0bc1。2.会利用get_shtml函数生成一个public文件夹下的shtml文件,并将内容写入
- 【Java成王之路】EE初阶第十二篇:(网络原理) 2
K稳重
网络开发语言服务器运维java
上节回顾网络原理,应用层传输层:端口号UDP校验和接上篇校验和继续实际使用的校验和算法有很多,其中比较常见的,crc,md5.crc:循环冗余校验md5,也是一种算法.md5应用场景非常多,用来作为校验,只是其中一个场景而已.本质上是一个"非对称的哈希算法"要了解校验和,是如何校验的TCP协议TCP协议段格式关于TCP的特性1.可靠性2.超时重传3.连接管理图中A表示的是客户端,B表示的服务器.服
- 【北京航空航天大学】【信息网络安全实验】【实验一、密码学:DES+RSA+MD5编程实验】
不是AI
网络攻防密码学pythonweb安全密码学网络
信息网络安全实验实验一、DESRSAMD5一、实验目的1.通过对DES算法的代码编写,了解分组密码算法的设计思想和分组密码算法工作模式;2.掌握RSA算法的基本原理以及素数判定中的Rabin-Miller测试原理、Montgomery快速模乘(模幂)算法,了解公钥加密体制的优缺点及其常见应用方式;3.掌握MD5算法的基本原理,了解其主要应用方法。二、实验内容1.DES编程实验2.RSA编程实验3.
- Shiro学习(三)之MD5+随机盐salt+Hash散列认证
Solitude_dong
Shiroshiro
MD5:加密算法不可逆(只能根据明文生成密文;如果内容相同,不论执行多少次md5生成结果始终一致),通常和随机盐配合使用一般用来加密或签名签名:也称为校验和,就是用来判断两个内容是否一致eg:tomcat.ziptomcat.md5.fdfd…看是否下载完整,就可以点击tomcat.md5.看生成的签名和tomcat生成的是否一致两个文件是否一致?a.txt和bb.txt分别md5看签名是否一致破
- MATLAB R2019b 完整激活教程
华庭仔仔
MATLAB作为一款高级商业数学软件,广泛被各行各业使用,其软件的激活过程也比较繁琐,所以今天奶酪为大家写一篇详细的MATLABR2019b激活教程,希望可以帮助到大家。#准备工作:1.下载MATLABR2019b安装包2.安装大概需要26G空间3.全程断网!注:文件必须完整的下载,下载好文件后请对比MD5校验码!校验码不一致肯定会提示损坏#一、安装:1.双击「matlab_R2019b_mac6
- 基于Java+SpringBoot+Vue+ElementUI的超市管理系统
不懂代码的胖子@
Java实战案例vue.jsjavaspringboot
目录系统背景系统总体设计运行环境技术选型系统架构系统用例系统详细设计系统功能截图首页统计RBAC权限管理商品管理订单管理销量统计售后订单收银系统商品采购供应商管理采购统计系统核心功能设计RBAC权限设计逻辑删除EXCEL数据导出功能EXCEL数据导入功能SQL监控MD5加密RESTful架构风格数据存储设计模型图表结构获取源码文章末尾免费获取源码、软件和教程~系统背景最初的超市资料管理,都是靠人力
- Android Studio 的 Gradle Task 没有显示
飞滕人生TYF
androidstudioandroidide
问题AndroidStudio的GradleTask没有显示详细问题笔者需要获取android应用MD5,一种方法是但是笔者的AndroidStudio的GradleTask没有显示解决方案依次点击:File->Settings->Experimental->取消勾选“DonotbuildGradletasklistduringGradlesync”,如下图所示点击SyncProjectwithG
- 数字签名算法MD5withRSA
Just_Paranoid
技术流Clipmd5rsasignatrue
数字签名MD5withRSA,:将正文通过MD5数字摘要后,将密文再次通过生成的RSA密钥加密,生成数字签名,将明文与密文以及公钥发送给对方,对方拿到私钥/公钥对数字签名进行解密,然后解密后的,与明文经过MD5加密进行比较,如果一致则通过使用Signature的API来实现MD5withRSARSA原理:RSA算法基于一个十分简单的数论事实,将两个大素数相乘十分容易,但反过来想要对其乘积进行因式分
- 报文鉴别、实体鉴别
山间未相见
计算机网络哈希算法网络
目录鉴别1报文鉴别1.1用数字签名进行鉴别(原理)可保证机密性的数字签名1.2密码散列函数MD5算法MD5算法计算步骤安全散列算法SHA-11.3用报文鉴别码实现报文鉴别用报文鉴别码MAC鉴别报文使用已签名的报文鉴别码MAC对报文鉴别2实体鉴别最简单的实体鉴别过程使用不重数进行鉴别使用公钥体制进行不重数鉴别假冒攻击中间人攻击鉴别·鉴别(authentication)是网络安全中一个很重要的问题。鉴
- [NSSRound#17 Basic]WEB
zmjjtt
CTFctfweb
1.真·签到看robots.txt密码先base32再base64得到md5加密的密文,在线解得到密码为Nsshint用16进制转字符串,提示新生赛遇到过是一个敲击码加密账号是ctfer,登录之后源码提示在F111n4l.php要求nss参数若比较等于732339662,但是不能是数字nss=732339662,1绕过2.真的是文件上传吗?根据报错信息,是一个py后端用py命令执行环境变量读取到f
- Hbase - 自定义Rowkey规则
kikiki2
在Flink中我们有时候需要分析数据1点到2点的范围,可是经过Region又比较慢,这时候我们就可以定制TableInputFormat来实现我们的需求了,我们还可以采用Flink的DataSet的方式读取,另外下面还有Spark读取的例子。使用教程Md5Util.javaimportorg.apache.commons.codec.binary.Hex;importjava.security.M
- 2018-03-28 强网杯和安恒杯题目总结
ckj123
经过了两天和小伙伴们的熬夜奋战和艰苦奋斗我们终于强网杯web签到md5第一关这道题主要是md5的各种绕过首先就是第一题空数组就可以绕过了param1[]=sdfasdf¶m2[]=asdfasdf所得到的值都是0的md5值所以相等md5第二关s878926199a,s155964671a的md5值都是0e开头然后param1[]=s878926199a&m2[]=s155964671a就好
- 关于mysql兼容emojo表情
java_飞
一般这个问题会在需要通过第三方登录的产品中会遇到,因为直接从第三方那边获取过来的昵称很有可能是带各种emojo表情的,然后假如你的mysql数据库不兼容的话,那么会给你报错,使原本可以正常登录的系统报错;当然在选择想要兼容emojo表情前必须确认一下自己的mysql版本,要>=MySQL5.5.3版本、从库也必须是5.5的了、低版本不支持这个字符集、复制报错;否则我就建议因md5或者什么其他方法入
- UDP报文结构和注意事项
不是懒大王
udp网络协议网络
udp由报头和载荷组成,报头由四部分组成源端口目的端口udp长度检验和,每部分由两个字节组成。源端口和目的端口分别表示发送方和接收方的位置udp长度表示了该数据报的大小,一个udp数据报为64kb检验和用来检验所得到的数据是否正确。CRC算法这个算法将每个字节的数据加起来进行比较,来判断数据是否正确。在发生bit反转后加和数据就会发生改变,从而判断数据是否正确。md5算法定长不论数据多长,md5过
- Hadoop(一)
朱辉辉33
hadooplinux
今天在诺基亚第一天开始培训大数据,因为之前没接触过Linux,所以这次一起学了,任务量还是蛮大的。
首先下载安装了Xshell软件,然后公司给了账号密码连接上了河南郑州那边的服务器,接下来开始按照给的资料学习,全英文的,头也不讲解,说锻炼我们的学习能力,然后就开始跌跌撞撞的自学。这里写部分已经运行成功的代码吧.
在hdfs下,运行hadoop fs -mkdir /u
- maven An error occurred while filtering resources
blackproof
maven报错
转:http://stackoverflow.com/questions/18145774/eclipse-an-error-occurred-while-filtering-resources
maven报错:
maven An error occurred while filtering resources
Maven -> Update Proje
- jdk常用故障排查命令
daysinsun
jvm
linux下常见定位命令:
1、jps 输出Java进程
-q 只输出进程ID的名称,省略主类的名称;
-m 输出进程启动时传递给main函数的参数;
&nb
- java 位移运算与乘法运算
周凡杨
java位移运算乘法
对于 JAVA 编程中,适当的采用位移运算,会减少代码的运行时间,提高项目的运行效率。这个可以从一道面试题说起:
问题:
用最有效率的方法算出2 乘以8 等於几?”
答案:2 << 3
由此就引发了我的思考,为什么位移运算会比乘法运算更快呢?其实简单的想想,计算机的内存是用由 0 和 1 组成的二
- java中的枚举(enmu)
g21121
java
从jdk1.5开始,java增加了enum(枚举)这个类型,但是大家在平时运用中还是比较少用到枚举的,而且很多人和我一样对枚举一知半解,下面就跟大家一起学习下enmu枚举。先看一个最简单的枚举类型,一个返回类型的枚举:
public enum ResultType {
/**
* 成功
*/
SUCCESS,
/**
* 失败
*/
FAIL,
- MQ初级学习
510888780
activemq
1.下载ActiveMQ
去官方网站下载:http://activemq.apache.org/
2.运行ActiveMQ
解压缩apache-activemq-5.9.0-bin.zip到C盘,然后双击apache-activemq-5.9.0-\bin\activemq-admin.bat运行ActiveMQ程序。
启动ActiveMQ以后,登陆:http://localhos
- Spring_Transactional_Propagation
布衣凌宇
springtransactional
//事务传播属性
@Transactional(propagation=Propagation.REQUIRED)//如果有事务,那么加入事务,没有的话新创建一个
@Transactional(propagation=Propagation.NOT_SUPPORTED)//这个方法不开启事务
@Transactional(propagation=Propagation.REQUIREDS_N
- 我的spring学习笔记12-idref与ref的区别
aijuans
spring
idref用来将容器内其他bean的id传给<constructor-arg>/<property>元素,同时提供错误验证功能。例如:
<bean id ="theTargetBean" class="..." />
<bean id ="theClientBean" class=&quo
- Jqplot之折线图
antlove
jsjqueryWebtimeseriesjqplot
timeseriesChart.html
<script type="text/javascript" src="jslib/jquery.min.js"></script>
<script type="text/javascript" src="jslib/excanvas.min.js&
- JDBC中事务处理应用
百合不是茶
javaJDBC编程事务控制语句
解释事务的概念; 事务控制是sql语句中的核心之一;事务控制的作用就是保证数据的正常执行与异常之后可以恢复
事务常用命令:
Commit提交
- [转]ConcurrentHashMap Collections.synchronizedMap和Hashtable讨论
bijian1013
java多线程线程安全HashMap
在Java类库中出现的第一个关联的集合类是Hashtable,它是JDK1.0的一部分。 Hashtable提供了一种易于使用的、线程安全的、关联的map功能,这当然也是方便的。然而,线程安全性是凭代价换来的――Hashtable的所有方法都是同步的。此时,无竞争的同步会导致可观的性能代价。Hashtable的后继者HashMap是作为JDK1.2中的集合框架的一部分出现的,它通过提供一个不同步的
- ng-if与ng-show、ng-hide指令的区别和注意事项
bijian1013
JavaScriptAngularJS
angularJS中的ng-show、ng-hide、ng-if指令都可以用来控制dom元素的显示或隐藏。ng-show和ng-hide根据所给表达式的值来显示或隐藏HTML元素。当赋值给ng-show指令的值为false时元素会被隐藏,值为true时元素会显示。ng-hide功能类似,使用方式相反。元素的显示或
- 【持久化框架MyBatis3七】MyBatis3定义typeHandler
bit1129
TypeHandler
什么是typeHandler?
typeHandler用于将某个类型的数据映射到表的某一列上,以完成MyBatis列跟某个属性的映射
内置typeHandler
MyBatis内置了很多typeHandler,这写typeHandler通过org.apache.ibatis.type.TypeHandlerRegistry进行注册,比如对于日期型数据的typeHandler,
- 上传下载文件rz,sz命令
bitcarter
linux命令rz
刚开始使用rz上传和sz下载命令:
因为我们是通过secureCRT终端工具进行使用的所以会有上传下载这样的需求:
我遇到的问题:
sz下载A文件10M左右,没有问题
但是将这个文件A再传到另一天服务器上时就出现传不上去,甚至出现乱码,死掉现象,具体问题
解决方法:
上传命令改为;rz -ybe
下载命令改为:sz -be filename
如果还是有问题:
那就是文
- 通过ngx-lua来统计nginx上的虚拟主机性能数据
ronin47
ngx-lua 统计 解禁ip
介绍
以前我们为nginx做统计,都是通过对日志的分析来完成.比较麻烦,现在基于ngx_lua插件,开发了实时统计站点状态的脚本,解放生产力.项目主页: https://github.com/skyeydemon/ngx-lua-stats 功能
支持分不同虚拟主机统计, 同一个虚拟主机下可以分不同的location统计.
可以统计与query-times request-time
- java-68-把数组排成最小的数。一个正整数数组,将它们连接起来排成一个数,输出能排出的所有数字中最小的。例如输入数组{32, 321},则输出32132
bylijinnan
java
import java.util.Arrays;
import java.util.Comparator;
public class MinNumFromIntArray {
/**
* Q68输入一个正整数数组,将它们连接起来排成一个数,输出能排出的所有数字中最小的一个。
* 例如输入数组{32, 321},则输出这两个能排成的最小数字32132。请给出解决问题
- Oracle基本操作
ccii
Oracle SQL总结Oracle SQL语法Oracle基本操作Oracle SQL
一、表操作
1. 常用数据类型
NUMBER(p,s):可变长度的数字。p表示整数加小数的最大位数,s为最大小数位数。支持最大精度为38位
NVARCHAR2(size):变长字符串,最大长度为4000字节(以字符数为单位)
VARCHAR2(size):变长字符串,最大长度为4000字节(以字节数为单位)
CHAR(size):定长字符串,最大长度为2000字节,最小为1字节,默认
- [强人工智能]实现强人工智能的路线图
comsci
人工智能
1:创建一个用于记录拓扑网络连接的矩阵数据表
2:自动构造或者人工复制一个包含10万个连接(1000*1000)的流程图
3:将这个流程图导入到矩阵数据表中
4:在矩阵的每个有意义的节点中嵌入一段简单的
- 给Tomcat,Apache配置gzip压缩(HTTP压缩)功能
cwqcwqmax9
apache
背景:
HTTP 压缩可以大大提高浏览网站的速度,它的原理是,在客户端请求网页后,从服务器端将网页文件压缩,再下载到客户端,由客户端的浏览器负责解压缩并浏览。相对于普通的浏览过程HTML ,CSS,Javascript , Text ,它可以节省40%左右的流量。更为重要的是,它可以对动态生成的,包括CGI、PHP , JSP , ASP , Servlet,SHTML等输出的网页也能进行压缩,
- SpringMVC and Struts2
dashuaifu
struts2springMVC
SpringMVC VS Struts2
1:
spring3开发效率高于struts
2:
spring3 mvc可以认为已经100%零配置
3:
struts2是类级别的拦截, 一个类对应一个request上下文,
springmvc是方法级别的拦截,一个方法对应一个request上下文,而方法同时又跟一个url对应
所以说从架构本身上 spring3 mvc就容易实现r
- windows常用命令行命令
dcj3sjt126com
windowscmdcommand
在windows系统中,点击开始-运行,可以直接输入命令行,快速打开一些原本需要多次点击图标才能打开的界面,如常用的输入cmd打开dos命令行,输入taskmgr打开任务管理器。此处列出了网上搜集到的一些常用命令。winver 检查windows版本 wmimgmt.msc 打开windows管理体系结构(wmi) wupdmgr windows更新程序 wscrip
- 再看知名应用背后的第三方开源项目
dcj3sjt126com
ios
知名应用程序的设计和技术一直都是开发者需要学习的,同样这些应用所使用的开源框架也是不可忽视的一部分。此前《
iOS第三方开源库的吐槽和备忘》中作者ibireme列举了国内多款知名应用所使用的开源框架,并对其中一些框架进行了分析,同样国外开发者
@iOSCowboy也在博客中给我们列出了国外多款知名应用使用的开源框架。另外txx's blog中详细介绍了
Facebook Paper使用的第三
- Objective-c单例模式的正确写法
jsntghf
单例iosiPhone
一般情况下,可能我们写的单例模式是这样的:
#import <Foundation/Foundation.h>
@interface Downloader : NSObject
+ (instancetype)sharedDownloader;
@end
#import "Downloader.h"
@implementation
- jquery easyui datagrid 加载成功,选中某一行
hae
jqueryeasyuidatagrid数据加载
1.首先你需要设置datagrid的onLoadSuccess
$(
'#dg'
).datagrid({onLoadSuccess :
function
(data){
$(
'#dg'
).datagrid(
'selectRow'
,3);
}});
2.onL
- jQuery用户数字打分评价效果
ini
JavaScripthtmljqueryWebcss
效果体验:http://hovertree.com/texiao/jquery/5.htmHTML文件代码:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>jQuery用户数字打分评分代码 - HoverTree</
- mybatis的paramType
kerryg
DAOsql
MyBatis传多个参数:
1、采用#{0},#{1}获得参数:
Dao层函数方法:
public User selectUser(String name,String area);
对应的Mapper.xml
<select id="selectUser" result
- centos 7安装mysql5.5
MrLee23
centos
首先centos7 已经不支持mysql,因为收费了你懂得,所以内部集成了mariadb,而安装mysql的话会和mariadb的文件冲突,所以需要先卸载掉mariadb,以下为卸载mariadb,安装mysql的步骤。
#列出所有被安装的rpm package rpm -qa | grep mariadb
#卸载
rpm -e mariadb-libs-5.
- 利用thrift来实现消息群发
qifeifei
thrift
Thrift项目一般用来做内部项目接偶用的,还有能跨不同语言的功能,非常方便,一般前端系统和后台server线上都是3个节点,然后前端通过获取client来访问后台server,那么如果是多太server,就是有一个负载均衡的方法,然后最后访问其中一个节点。那么换个思路,能不能发送给所有节点的server呢,如果能就
- 实现一个sizeof获取Java对象大小
teasp
javaHotSpot内存对象大小sizeof
由于Java的设计者不想让程序员管理和了解内存的使用,我们想要知道一个对象在内存中的大小变得比较困难了。本文提供了可以获取对象的大小的方法,但是由于各个虚拟机在内存使用上可能存在不同,因此该方法不能在各虚拟机上都适用,而是仅在hotspot 32位虚拟机上,或者其它内存管理方式与hotspot 32位虚拟机相同的虚拟机上 适用。
- SVN错误及处理
xiangqian0505
SVN提交文件时服务器强行关闭
在SVN服务控制台打开资源库“SVN无法读取current” ---摘自网络 写道 SVN无法读取current修复方法 Can't read file : End of file found
文件:repository/db/txn_current、repository/db/current
其中current记录当前最新版本号,txn_current记录版本库中版本