pku2894-2903 Tehran 2005 比赛总结
Solved | ID | Title | Ratio(AC/att) | |
---|---|---|---|---|
Yes | Problem A | Ancient Keyboard | 100.0%(1/1) | Submit |
Yes | Problem B | Best SMS to Type | 50.0%(1/2) | Submit |
Yes | Problem C | Changing Phone Numbers | 100.0%(1/1) | Submit |
Yes | Problem D | Dramatic Multiplications | 33.33%(1/3) | Submit |
Yes | Problem E | Entertainment | 100.0%(1/1) | Submit |
Yes | Problem F | Fortune at El Dorado | 100.0%(1/1) | Submit |
Yes | Problem G | Griddy Hobby | 100.0%(1/1) | Submit |
Problem H | Hotel | 0.0%(0/0) | Submit | |
Problem I | Intercepting Missiles | 0.0%(0/0) | Submit | |
Yes | Problem J | Joy of Mobile Routing | 100.0%(1/1) | Submit |
这场比赛题目难度适中,有2题没有做出来。。
pku2894 Ancient Keyboard
直接开个数组来模拟就可以了,数据量再大的话就线段树吧- -
代码:
1
# include
<
iostream
>
2
# include
<
cstring
>
3
using
namespace
std;
4
int
main()
5
{
6
int test;
7
cin>>test;
8
while(test--)
9
{
10
int n;
11
cin>>n;
12
char l;
13
int s,e,c[1001],max=-1,min=0xfffffff;
14
memset(c,0,sizeof(c));
15
while(n--)
16
{
17
cin>>l>>s>>e;
18
for(int i=s;i<e;i++)
19
c[i]++;
20
if(e>max) max=e;
21
if(s<min) min=s;
22
}
23
for(int i=min;i<=max;i++)
24
if(c[i])
25
cout<<(char)(c[i]+64);
26
cout<<endl;
27
}
28
return 0;
29
}

2

3

4

5



6

7

8

9



10

11

12

13

14

15

16



17

18

19

20

21

22

23

24

25

26

27

28

29

pku2896 Best SMS to Type
同样是个没有难度的模拟,细心点就可以了~
1
# include
<
iostream
>
2
using
namespace
std;
3
inline
bool
equal(
int
a,
int
b)
4
{
5
if(a<15&&b<15&&a/3==b/3) return true;
6
else if(a<19&&b<19&&a>=15&&b>=15) return true;
7
else if(a<22&&b<22&&a>=19&&b>=19) return true;
8
else if(a>=22&&b>=22) return true;
9
else return false;
10
}
11
int
main()
12
{
13
int test,map[255];
14
map['A']=map['D']=map['G']=map['J']=map['M']=map['P']=map['T']=map['W']=1;
15
map['B']=map['E']=map['H']=map['K']=map['N']=map['Q']=map['U']=map['X']=2;
16
map['C']=map['F']=map['I']=map['L']=map['O']=map['R']=map['V']=map['Y']=3;
17
map['S']=map['Z']=4;
18
map[' ']=1;
19
cin>>test;
20
while(test--)
21
{
22
int t1,t2,total=0;
23
char str[1024];
24
cin>>t1>>t2;
25
cin.get();
26
cin.getline(str,1024);
27
total+=t1*map[str[0]];
28
for(int i=1;str[i]!='\0';i++)
29
if(str[i]!=' '&&str[i-1]!=' '&&equal(str[i]-65,str[i-1]-65))
30
total+=map[str[i]]*t1+t2;
31
else total+=map[str[i]]*t1;
32
cout<<total<<endl;
33
}
34
return 0;
35
}

2

3

4



5

6

7

8

9

10

11

12



13

14

15

16

17

18

19

20

21



22

23

24

25

26

27

28

29

30

31

32

33

34

35

pku2896 Changing Phone Numbers
各种数据结构的应用,先按时间排序,然后借助于堆、字典树之类的东西设计一个离线算法应该不难。记得看清题目: [s+1,e]区间内的规则适用于一个询问。
代码:
1
import
java.util.
*
;
2
import
java.io.
*
;
3
class
dic
4
{
5
class node
6
{
7
node nxt[]=new node[10];
8
String str;
9
boolean end=false;
10
node()
11
{
12
Arrays.fill(nxt,null);
13
}
14
}
15
node head=new node();
16
void insert(String num,String str)
17
{
18
node p=head;
19
for(int i=0;i<num.length();i++)
20
{
21
if(p.nxt[num.charAt(i)-'0']==null)
22
p.nxt[num.charAt(i)-'0']=new node();
23
p=p.nxt[num.charAt(i)-'0'];
24
}
25
p.end=true;
26
p.str=str;
27
}
28
void erase(String num)
29
{
30
node p=head;
31
for(int i=0;i<num.length();i++)
32
p=p.nxt[num.charAt(i)-'0'];
33
p.end=false;
34
}
35
String get(String num)
36
{
37
node p=head;
38
for(int i=0;i<num.length();i++)
39
{
40
if(p.end) return p.str;
41
p=p.nxt[num.charAt(i)-'0'];
42
}
43
return p.str;
44
}
45
}
46
public
class
Main
{
47
48
/** *//**
49
* @param argsi
50
*/
51
52
static class ins implements Comparable<ins>
53
{
54
int year,type;
55
String name,op;
56
public int compareTo(ins pos)
57
{
58
return year-pos.year;
59
}
60
ins(int year,int type,String name,String op)
61
{
62
this.year=year;
63
this.type=type;
64
this.name=name;
65
this.op=op;
66
}
67
}
68
static class node
69
{
70
int s,e,id;
71
String code;
72
node(int id,int s,int e,String code)
73
{
74
this.s=s;
75
this.e=e;
76
this.code=code;
77
this.id=id;
78
}
79
}
80
static class local
81
{
82
String num;
83
TreeSet<node> list=new TreeSet<node>(new cmp_e());
84
local(String str)
85
{
86
num=str;
87
}
88
void ChangeCode(String code)
89
{
90
String name=antirefer.get(num);
91
antirefer.erase(num);
92
num=code;
93
antirefer.insert(num, name);
94
}
95
void add(node pos)
96
{
97
pos.code=pos.code.substring(num.length());
98
list.add(pos);
99
}
100
void repeat(int pos)
101
{
102
for(node p:list)
103
p.code=p.code.substring(0,pos)+p.code.substring(pos-1);
104
}
105
void swap(int pos)
106
{
107
for(node p:list)
108
p.code=p.code.substring(0,pos-1)+p.code.charAt(pos)+p.code.charAt(pos-1)+p.code.substring(pos+1);
109
}
110
void move(int year)
111
{
112
while(!list.isEmpty()&&list.first().e<year)
113
{
114
list.first().code=num+list.first().code;
115
ans.add(list.pollFirst());
116
}
117
118
119
}
120
}
121
static class cmp_s implements Comparator<node>
122
{
123
public int compare(node a,node b)
124
{
125
return a.s-b.s;
126
}
127
}
128
static class cmp_e implements Comparator<node>
129
{
130
public int compare(node a,node b)
131
{
132
if(a.e!=b.e) return a.e-b.e;
133
else return a.id-b.id;
134
}
135
}
136
static class cmp_id implements Comparator<node>
137
{
138
public int compare(node a,node b)
139
{
140
return a.id-b.id;
141
}
142
}
143
static dic antirefer=new dic();
144
static ArrayList<node> ans=new ArrayList<node>();
145
public static void main(String[] args) throws IOException
{
146
Scanner in=new Scanner(new BufferedReader(new InputStreamReader(System.in)));
147
HashMap<String,local> refer=new HashMap<String,local>();
148
ArrayList<ins> list=new ArrayList<ins>();
149
ArrayList<node> ques=new ArrayList<node>();
150
int n=in.nextInt();
151
while((n--)!=0)
152
{
153
String code=in.next(),name=in.next();
154
refer.put(name, new local(code));
155
antirefer.insert(code, name);
156
}
157
n=in.nextInt();
158
while((n--)!=0)
159
list.add(new ins(in.nextInt(),in.nextInt(),in.next(),in.next()));
160
int tc=0;
161
while(true)
162
{
163
int s=in.nextInt(),e=in.nextInt();
164
String code=in.next();
165
if(s==0&&e==0&&code.equals("0")) break;
166
ques.add(new node(tc++,s,e,code));
167
}
168
Collections.sort(list);
169
Collections.sort(ques,new cmp_s());
170
int p=0;
171
for(ins i:list)
172
{
173
while(p<ques.size()&&ques.get(p).s<i.year)
174
{
175
if(antirefer.get(ques.get(p).code)==null)
176
{
177
System.out.println("wa");
178
}
179
refer.get(antirefer.get(ques.get(p).code)).add(ques.get(p));
180
p++;
181
}
182
refer.get(i.name).move(i.year);
183
switch(i.type)
184
{
185
case 1:
186
refer.get(i.name).repeat(Integer.parseInt(i.op));
187
break;
188
case 2:
189
refer.get(i.name).swap(Integer.parseInt(i.op));
190
break;
191
case 3:
192
refer.get(i.name).ChangeCode(i.op);
193
break;
194
};
195
196
}
197
for(;p<ques.size();p++)
198
ans.add(ques.get(p));
199
for(local i:refer.values())
200
i.move(Integer.MAX_VALUE);
201
Collections.sort(ans,new cmp_id());
202
for(node i:ans)
203
System.out.println(i.code);
204
205
}
206
207
}

2

3

4



5

6



7

8

9

10

11



12

13

14

15

16

17



18

19

20



21

22

23

24

25

26

27

28

29



30

31

32

33

34

35

36



37

38

39



40

41

42

43

44

45

46



47

48


49

50

51

52

53



54

55

56

57



58

59

60

61



62

63

64

65

66

67

68

69



70

71

72

73



74

75

76

77

78

79

80

81



82

83

84

85



86

87

88

89



90

91

92

93

94

95

96



97

98

99

100

101



102

103

104

105

106



107

108

109

110

111



112

113



114

115

116

117

118

119

120

121

122



123

124



125

126

127

128

129



130

131



132

133

134

135

136

137



138

139



140

141

142

143

144

145



146

147

148

149

150

151

152



153

154

155

156

157

158

159

160

161

162



163

164

165

166

167

168

169

170

171

172



173

174



175

176



177

178

179

180

181

182

183

184



185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

pku2897 Dramatic Multiplications
一道数学题,MS现在权位展开的题目不少- -。这题可以从低位向高位逐位确定。
AP
* K
-------
PA
什么情况无解?出现了循环~。注意!第一位不能为0
代码:
1
# include
<
iostream
>
2
# include
<
stack
>
3
# include
<
cstring
>
4
using
namespace
std;
5
bool
used[
10
][
10
];
6
7
int
main()
8
{
9
int test;
10
cin>>test;
11
while(test--)
12
{
13
int n,k,last,add;
14
bool flag=false;
15
cin>>n>>k;
16
stack<int> ans;
17
memset(used,false,sizeof(used));
18
last=k,add=0;
19
while(!used[last][add])
20
{
21
used[last][add]=true;
22
int nxt=last*n+add;
23
last=nxt%10;
24
add=nxt/10;
25
if(!add&&last==k&&(ans.empty()||ans.top()))
26
{
27
for(;!ans.empty();ans.pop())
28
cout<<ans.top();
29
cout<<k<<endl;
30
flag=true;
31
break;
32
}
33
else ans.push(last);
34
}
35
if(!flag) cout<<0<<endl;
36
}
37
return 0;
38
}

2

3

4

5

6

7

8



9

10

11

12



13

14

15

16

17

18

19

20



21

22

23

24

25

26



27

28

29

30

31

32

33

34

35

36

37

38

pku2898 Entertainment
又是一个模拟的题目,记得POJ上有题叫什么game的和这个一样。用数组模拟就可以了。好久不写BFS,忘了一点,BFS判重的时候是在进队之前~,还有就是,字符输入输出尽量用gets,puts,getchar,putchar
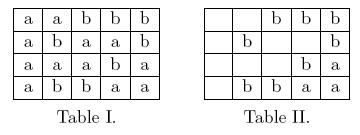
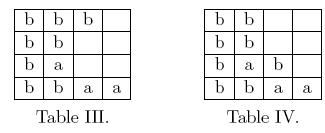
代码:


1

2

3

4

5

6

7

8

9

10

11

12

13



14

15

16

17

18

19

20



21

22

23

24

25

26



27

28

29

30

31



32

33

34

35

36



37

38

39

40

41



42

43

44

45

46

47

48

49



50

51

52



53

54

55

56



57

58

59

60

61

62

63

64

65

66



67

68

69



70

71

72

73



74

75

76

77

78

79

80

81

82

83



84

85



86

87

88

89

90

91

92

93



94

95

96

97

98



99

100

101



102

103

104



105

106

107

108

109

110

111

112



113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

pku2899 Fortune at El Dorado
经典的二维动态统计:扫描线+树状数组。
这题有点新意的地方就是要枚举扫描线宽度,然后以前动态维护一个树状数组的算法有点不适用了,所以就开了n个树状数组,然后相减即可~复杂度仍然为o(nlogn),BS这题给出的数据范围,点总数只有100个却写1000个。。1000个点估计没有什么好算法了吧。。
代码:


1

2

3

4

5

6

7

8

9

10



11

12

13

14



15

16

17

18

19

20

21

22

23



24

25

26

27



28

29

30

31

32

33

34



35

36

37



38

39

40

41

42

43

44

45



46

47

48

49

50

51



52

53

54

55

56

57

58

59

60

61

62

63

64

65



66

67

68

69

70

71

72

73



74

75

76

77



78

79

80

81

82

83

84

85

86

pku2900 Griddy Hobby
有趣的题目,一条光线从一点45度角射出,不断反弹,直到循环或者射出为止。求包含的最小长方形数目。我用的方法很笨。。将所有的线段模拟出来后然后整个坐标系旋转45度,用上题的动态统计的方法做的。。MS可以直接根据交点数来算。。不过我的那种方法更通用
模拟的时候考虑周全一点,四种向量,8种情况(每一个向量落在不同的边上时旋转地方向不同)
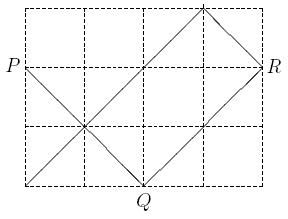
代码:


1

2

3

4

5

6

7

8

9

10

11

12

13



14

15

16

17



18

19

20

21

22



23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40



41

42

43

44



45

46



47

48

49

50

51

52

53

54

55

56

57



58

59

60

61

62

63

64



65

66

67

68

69



70

71

72

73



74

75

76

77

78

79

80

81

82

83

84

85

86



87

88

89

90



91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120



121

122

123

124

125

126

127

128

129

130

131

132

133



134

135



136

137

138

139

140



141

142

143

144

145

146

147

148

149

pku2903 Joy of Mobile Routing
一道三维向量的题目。首先枚举岔口和发射站的工作省不了的,这里n2m2的复杂度,然后就是判断发射站能不能发射到岔口。
这里MS要详细讨论4种情况:岔口在发射站的左上、右上、左下、右下四种情况,然后上取整和下取整注意下,行列分别验证~总复杂度n3m2,还有向量的方向不能反,必须是从发射站到岔口,应为反过来会有一种很难处理的东西,就是射线根本过不了第一个障碍物,但是到达障碍物对面的时候是比它高的,这样就造成了误判。无权图最短路径不用说了吧,BFS
1
# include
<
cstdio
>
2
using
namespace
std;
3
# include
<
algorithm
>
4
# include
<
cmath
>
5
# include
<
queue
>
6
# include
<
cstring
>
7
# include
<
utility
>
8
# include
<
functional
>
9
# define N
55
10
# define eps 1e
-
8
11
# define equ(a,b) (fabs((a)
-
(b))
<
eps)
12
# define gt(a,b) (
!
equ(a,b)
&&
(a)
>
(b))
13
int
h[N][N],len[N][N];
14
bool
used[N][N];
15
int
n,m,num,sr,sc,er,ec;
16
struct
node
17
{
18
int r,c,h;
19
bool operator<(const node &pos) const
20
{
21
return h>pos.h;
22
}
23
}
sta[
105
];
24
int
bfs()
25
{
26
used[er][ec]=true;
27
if(!used[sr][sc]||!used[er][ec]) return -1;
28
queue<pair<int,int> > q;
29
memset(len,-1,sizeof(len));
30
len[sr][sc]=0;
31
q.push(make_pair<int,int>(sr,sc));
32
while(!q.empty())
33
{
34
pair<int,int> top=q.front();
35
q.pop();
36
if(top.first-1>=0&&used[top.first-1][top.second]&&len[top.first-1][top.second]==-1)
37
{
38
len[top.first-1][top.second]=len[top.first][top.second]+1;
39
q.push(make_pair<int,int>(top.first-1,top.second));
40
}
41
if(top.first+1<=n&&used[top.first+1][top.second]&&len[top.first+1][top.second]==-1)
42
{
43
len[top.first+1][top.second]=len[top.first][top.second]+1;
44
q.push(make_pair<int,int>(top.first+1,top.second));
45
}
46
if(top.second-1>=0&&used[top.first][top.second-1]&&len[top.first][top.second-1]==-1)
47
{
48
len[top.first][top.second-1]=len[top.first][top.second]+1;
49
q.push(make_pair<int,int>(top.first,top.second-1));
50
}
51
if(top.second+1<=m&&used[top.first][top.second+1]&&len[top.first][top.second+1]==-1)
52
{
53
len[top.first][top.second+1]=len[top.first][top.second]+1;
54
q.push(make_pair<int,int>(top.first,top.second+1));
55
}
56
}
57
return len[er][ec]==-1?-1:len[er][ec]*10;
58
}
59
bool
detect(
int
r,
int
c,
int
pos)
60
{
61
if(sta[pos].r==r||sta[pos].c==c) return true;
62
double dr=10*(r-sta[pos].r),dc=10*(c-sta[pos].c),dh=-sta[pos].h;
63
bool f1=r>sta[pos].r,f2=c>sta[pos].c;
64
//test row
65
for(int i=f1?sta[pos].r+1:sta[pos].r-1;f1?i<=r:i>=r;f1?i++:i--)
66
{
67
double k=10*(i-sta[pos].r)/dr,nc=sta[pos].c*10+k*dc,nh=sta[pos].h+k*dh;
68
int t1=f1?i-1:i,t2=f2?ceil(nc/10-eps)-1+eps:nc/10+eps;
69
if(gt(h[t1][t2],nh)) return false;
70
}
71
//test col
72
for(int i=f2?sta[pos].c+1:sta[pos].c-1;f2?i<=c:i>=c;f2?i++:i--)
73
{
74
double k=10*(i-sta[pos].c)/dc,nr=sta[pos].r*10+k*dr,nh=sta[pos].h+k*dh;
75
int t1=f1?ceil(nr/10-eps)-1+eps:nr/10+eps,t2=f2?i-1:i;
76
if(gt(h[t1][t2],nh)) return false;
77
}
78
return true;
79
}
80
int
main()
81
{
82
int test;
83
scanf("%d",&test);
84
while(test--)
85
{
86
scanf("%d%d",&n,&m);
87
for(int i=0;i<n;i++)
88
for(int j=0;j<m;j++)
89
scanf("%d",&h[i][j]);
90
scanf("%d%d%d%d%d",&sr,&sc,&er,&ec,&num);
91
for(int i=0;i<num;i++)
92
scanf("%d%d%d",&sta[i].r,&sta[i].c,&sta[i].h);
93
//sort(sta,sta+num);
94
for(int i=0;i<=n;i++)
95
for(int j=0;j<=m;j++)
96
{
97
used[i][j]=false;
98
for(int k=0;k<num&&!used[i][j];k++)
99
if(detect(i,j,k)) used[i][j]=true;
100
}
101
/**//*printf("\n");
102
for(int i=0;i<=n;i++)
103
{
104
for(int j=0;j<=m;j++)
105
printf("%d ",used[i][j]);
106
printf("\n");
107
}*/
108
printf("%d\n",bfs());
109
}
110
return 0;
111
}

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17



18

19

20



21

22

23

24

25



26

27

28

29

30

31

32

33



34

35

36

37



38

39

40

41

42



43

44

45

46

47



48

49

50

51

52



53

54

55

56

57

58

59

60



61

62

63

64

65

66



67

68

69

70

71

72

73



74

75

76

77

78

79

80

81



82

83

84

85



86

87

88

89

90

91

92

93

94

95

96



97

98

99

100

101


102

103

104

105

106

107

108

109

110

111

pku2902 Intercepting Missiles
这题我不知道说什么好,就是一个二分匹配模型,但是精度问题搞死我了,一直没能A,有A的请将代码贴在下面,万分感谢