转载地址:http://blog.csdn.net/xiaobijia/article/details/40738879
在登陆应用时,信息有更新,为了引起用户注意,会有提示标签:
百度:
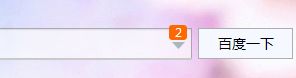
网易:
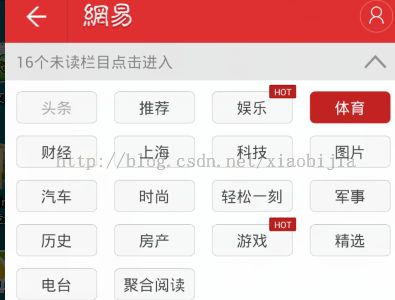
如果给标签加入动画效果,会更加醒目,如淘宝:
本例效果:
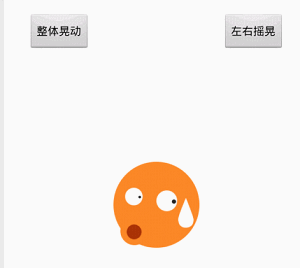
使用属性动画实现,稍微修改就可以实现淘宝的效果
实现动画类:
- package com.example.swingaimation;
-
- import android.animation.Keyframe;
- import android.animation.ObjectAnimator;
- import android.animation.PropertyValuesHolder;
- import android.annotation.SuppressLint;
- import android.view.View;
-
-
-
-
- public class AnimationUtils {
-
- public static ObjectAnimator tada(View view) {
- return tada(view, 1f);
- }
-
- @SuppressLint("NewApi")
- public static ObjectAnimator tada(View view, float shakeFactor) {
-
- PropertyValuesHolder pvhScaleX = PropertyValuesHolder.ofKeyframe(View.SCALE_X,
- Keyframe.ofFloat(0f, 1f),
- Keyframe.ofFloat(.1f, .9f),
- Keyframe.ofFloat(.2f, .9f),
- Keyframe.ofFloat(.3f, 1.1f),
- Keyframe.ofFloat(.4f, 1.1f),
- Keyframe.ofFloat(.5f, 1.1f),
- Keyframe.ofFloat(.6f, 1.1f),
- Keyframe.ofFloat(.7f, 1.1f),
- Keyframe.ofFloat(.8f, 1.1f),
- Keyframe.ofFloat(.9f, 1.1f),
- Keyframe.ofFloat(1f, 1f)
- );
-
- PropertyValuesHolder pvhScaleY = PropertyValuesHolder.ofKeyframe(View.SCALE_Y,
- Keyframe.ofFloat(0f, 1f),
- Keyframe.ofFloat(.1f, .9f),
- Keyframe.ofFloat(.2f, .9f),
- Keyframe.ofFloat(.3f, 1.1f),
- Keyframe.ofFloat(.4f, 1.1f),
- Keyframe.ofFloat(.5f, 1.1f),
- Keyframe.ofFloat(.6f, 1.1f),
- Keyframe.ofFloat(.7f, 1.1f),
- Keyframe.ofFloat(.8f, 1.1f),
- Keyframe.ofFloat(.9f, 1.1f),
- Keyframe.ofFloat(1f, 1f)
- );
-
- PropertyValuesHolder pvhRotate = PropertyValuesHolder.ofKeyframe(View.ROTATION,
- Keyframe.ofFloat(0f, 0f),
- Keyframe.ofFloat(.1f, -3f * shakeFactor),
- Keyframe.ofFloat(.2f, -3f * shakeFactor),
- Keyframe.ofFloat(.3f, 3f * shakeFactor),
- Keyframe.ofFloat(.4f, -3f * shakeFactor),
- Keyframe.ofFloat(.5f, 3f * shakeFactor),
- Keyframe.ofFloat(.6f, -3f * shakeFactor),
- Keyframe.ofFloat(.7f, 3f * shakeFactor),
- Keyframe.ofFloat(.8f, -3f * shakeFactor),
- Keyframe.ofFloat(.9f, 3f * shakeFactor),
- Keyframe.ofFloat(1f, 0)
- );
-
- return ObjectAnimator.ofPropertyValuesHolder(view, pvhScaleX, pvhScaleY, pvhRotate).
- setDuration(1000);
- }
-
- @SuppressLint("NewApi")
- public static ObjectAnimator nope(View view) {
- int delta = view.getResources().getDimensionPixelOffset(R.dimen.activity_horizontal_margin);
-
- PropertyValuesHolder pvhTranslateX = PropertyValuesHolder.ofKeyframe(View.TRANSLATION_X,
- Keyframe.ofFloat(0f, 0),
- Keyframe.ofFloat(.10f, -delta),
- Keyframe.ofFloat(.26f, delta),
- Keyframe.ofFloat(.42f, -delta),
- Keyframe.ofFloat(.58f, delta),
- Keyframe.ofFloat(.74f, -delta),
- Keyframe.ofFloat(.90f, delta),
- Keyframe.ofFloat(1f, 0f)
- );
-
- return ObjectAnimator.ofPropertyValuesHolder(view, pvhTranslateX).
- setDuration(500);
- }
-
- }
主类即调用类:
- package com.example.swingaimation;
-
- import android.os.Bundle;
- import android.annotation.SuppressLint;
- import android.app.Activity;
- import android.view.View;
- import android.widget.ImageView;
-
- public class MainActivity extends Activity {
-
- private ImageView imageview;
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- imageview = (ImageView) findViewById(R.id.imageview);
-
- }
-
- @SuppressLint("NewApi")
- public void wholeShake(View view) {
- AnimationUtils.tada(imageview).start();
- }
-
- @SuppressLint("NewApi")
- public void lefToRightShake(View view) {
- AnimationUtils.nope(imageview).start();
- }
- }
参考:
消息更新提示动画 - 下载频道 - CSDN.NET
http://download.csdn.net/detail/xiaobijia/8115009