- JavaScript基础-创建对象的三种方式
難釋懷
javascript开发语言
在JavaScript编程中,对象(Object)是构建复杂数据结构和实现面向对象编程的基础。了解如何有效地创建对象对于编写高效、可维护的代码至关重要。本文将介绍三种主要的创建对象的方式:对象字面量、构造函数模式以及Object.create()方法,并讨论它们的特点及适用场景。一、使用对象字面量1.简介对象字面量是最简单直接的创建对象的方法。它允许你以一种非常直观的方式定义属性和方法。示例:le
- 一份C#的笔试题及答案
网际游侠
c#面试笔试
C#笔试题一、基础知识OOP的基本概念面向对象编程的核心思想包括四个主要特性:继承、多态、封装和信息隐藏。请简述这四个特性的具体内容。值类型与引用类型的区别值类型(如int,string)在内存中直接存储数据,而引用类型(如object,ref)通过引用间接存储,指出值的位置。请举例说明两者的不同。静态与非静态关键字的作用静态和非静态关键字用于区分依赖于实例还是不依赖于实例的成员。请分别解释它们的
- python基于django/flask网上书城系统Django-SpringBoot-php-Node.js-flask
QQ_1963288475
pythondjangoflaskspringbootphplaravelnode.js
目录技术栈介绍具体实现截图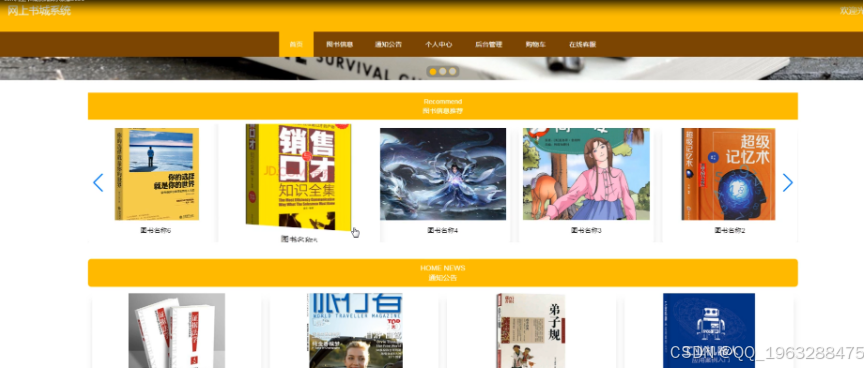系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研
- 在 CentOS 7 上安装 PHP 7.3
wjf63000
centosphplinux
在CentOS7上安装PHP7.3可以按照以下步骤进行操作:1.安装必要的依赖和EPEL仓库EPEL(ExtraPackagesforEnterpriseLinux)是为企业级Linux提供额外软件包的仓库,yum-utils用于管理yum仓库。sudoyuminstall-yepel-releaseyum-utils2.添加Remi仓库Remi仓库包含了丰富的PHP版本,你可以从中选择PHP7.
- 面试中必会的Java基础(一)
每次的天空
面试java学习
Java是面向对象编程所以第一就是面向对象编程的特点是什么?面向对象编程类与对象:掌握类的定义、成员变量和成员方法的声明与使用,以及如何通过类创建对象。理解对象的生命周期,包括创建、使用和销毁。封装:明白封装的概念,即把数据和操作数据的方法封装在一个类中,通过访问修饰符(public、private、protected等)来控制对类成员的访问。继承:理解继承的概念和作用,掌握通过extends关键
- 软件开发面试题C#,.NET知识点(续)
.房东的猫
c#.net
1.C#中的封装是什么,以及它的重要性。封装(Encapsulation)是面向对象编程(OOP)的一个基本概念。它指的是将对象的状态(属性)和行为(方法)绑定在一起,并且将这些细节隐藏起来,只暴露必要的接口给外部使用。这样做的好处包括:提高代码的安全性:通过将数据隐藏在类内部,并通过公共方法(getters和setters)来访问和修改这些数据,可以防止外部代码直接修改类的内部状态,从而保护数据
- GIT日常记录
码农汉子
elasticsearch大数据搜索引擎
sudogitclonehttps://gitee.com/yiketalks/yike_admin.git命令行下载项目命令(进入本地项目地址下)//查看远程仓库地址gitremote-v//切换远程仓库gitremoteset-urloriginhttps://gitee.com/yike_php/yike_work.git分支操作gitbranch//显示分支一览表,同时确认当前所在的分支1
- 【2025年春季】全国CTF夺旗赛-从零基础入门到竞赛,看这一篇就稳了!
白帽子凯哥
web安全学习安全CTF夺旗赛网络安全
基于入门网络安全/黑客打造的:黑客&网络安全入门&进阶学习资源包目录一、CTF简介二、CTF竞赛模式三、CTF各大题型简介四、CTF学习路线4.1、初期1、html+css+js(2-3天)2、apache+php(4-5天)3、mysql(2-3天)4、python(2-3天)5、burpsuite(1-2天)4.2、中期1、SQL注入(7-8天)2、文件上传(7-8天)3、其他漏洞(14-15
- 批量检查微信小程序是否被封的Go代码
微信微信小程序
概述:这段Go代码通过请求接口https://api.52an.fun/xcx/checkxcx.php?appid={appid},批量检查多个微信小程序是否被封禁。接口返回的JSON数据中包含code字段,code为1表示小程序正常,code为0表示小程序被封禁,并且会返回封禁原因。程序会根据返回结果输出每个小程序的状态。Go代码示例:packagemainimport("encoding/j
- 批量检查QQ域名是否被封的C++代码示例
qq
概述:此C++代码示例展示了如何批量请求指定的API接口,检查QQ域名的状态。根据API返回的status值,status为1表示域名正常,status为0表示域名被封禁。我们将使用libcurl来进行HTTP请求,使用jsoncpp解析返回的JSON数据。目标:通过C++编写代码,批量请求https://api.52an.fun/qq/api.php?url=接口,检查多个QQ域名的封禁状态,并
- C++和C语言的区别有哪些
残余的记忆
c++c语言数据结构开发语言
C++和C语言是两种不同的编程语言,虽然它们有许多相似之处,但是它们之间也存在着很多区别。本文将介绍C++和C语言之间的一些主要区别。1.面向对象编程C++是一种面向对象编程语言,相较于C语言,其具有更多的特性。面向对象编程(OOP)作为一种编程方法论,通过对数据进行封装、继承、多态等操作,来实现程序的灵活性和可维护性。C++提供了很多面向对象编程的特性,例如类、继承、多态等。这些特性能够让程序员
- Lua语言的计算机体系结构
凌嘉遥
包罗万象golang开发语言后端
Lua语言的计算机体系结构引言Lua是一种轻量级、高效且可扩展的脚本语言,最早由巴西的一个小组开发。它的设计目标是为嵌入式系统提供一个简洁的语言,从而使开发者能够在不同的应用程序中方便地进行扩展和集成。尽管Lua本身是一种面向过程的语言,但它也支持面向对象编程、函数式编程等多种编程范式。因此,研究Lua的计算机体系结构,不仅能帮助我们理解Lua如何实现其功能,还能为其他编程语言的设计与实现提供参考
- PHP与数据库连接常见问题及解决办法
奥顺互联_老张
php教程php数据库
PHP与数据库连接常见问题及解决办法在现代Web开发中,PHP与数据库的连接是不可或缺的一部分。无论是构建动态网站、内容管理系统(CMS)还是电子商务平台,PHP与数据库的交互都是核心功能之一。然而,在实际开发过程中,开发者常常会遇到各种与数据库连接相关的问题。本文将探讨PHP与数据库连接中的常见问题,并提供相应的解决办法。1.数据库连接失败问题描述在PHP中,连接数据库时最常见的错误是无法连接到
- AJAX PHP:深入理解与实际应用
wjs2024
开发语言
AJAXPHP:深入理解与实际应用引言随着互联网技术的不断发展,前端与后端交互变得更加频繁。AJAX(AsynchronousJavaScriptandXML)和PHP(HypertextPreprocessor)作为两种流行的技术,在实现动态网页和应用程序方面扮演着重要角色。本文将深入探讨AJAXPHP的工作原理、应用场景以及实际开发中的注意事项。AJAXPHP概述AJAXAJAX是一种基于Ja
- mongodb基本使用(四)
dibisha7239
数据库javascript数据结构与算法ViewUI
MongoDB条件操作符描述条件操作符用于比较两个表达式并从mongoDB集合中获取数据。MongoDB中条件操作符有:(>)大于-$gt(=)大于等于-$gte(db.col.insert({title:'PHP教程',description:'PHP是一种创建动态交互性站点的强有力的服务器端脚本语言。',by:'菜鸟教程',url:'http://www.runoob.com',tags:['
- Flutter Dart 面向对象编程全面解析
顾林海
Flutter系列教程flutter前端android
引言在Flutter开发中,Dart作为其编程语言,采用了面向对象的编程范式。面向对象编程(OOP)将数据和操作数据的方法封装在一起,形成对象,以提高代码的可维护性、可扩展性和可重用性。本文将详细介绍Dart面向对象编程的核心概念,包括类、对象、继承、多态、抽象类和接口等,并结合代码示例进行说明。1.类和对象类是对象的蓝图,它定义了对象的属性和方法。对象是类的实例,通过类可以创建多个不同的对象。代
- Flutter开发之Dart语言
caiyajie666
Flutterflutter开发语言
Dart是Flutter框架的官方开发语言,Flutter应用几乎完全使用Dart编写。Flutter的跨平台特性使得Dart在移动应用开发中非常受欢迎。Dart是面向对象的、类定义的、单继承的语言,支持面向对象编程,包括封装、继承和多态等特性;Dart支持接口(interfaces)、混入(mixins)、抽象类(abstractclasses)、具体化泛型、可选类型。Dart中很多概念跟其他语
- 树莓派搭php,Raspberry Pi 树莓派搭LAMP服务器
平平无奇的美女
树莓派搭php
目录:为什么要用树莓派?DebianLinux安全性操作系统性能优化配置网络开启sshMakingtheserveravailableontheInternetDNS安装apache安全MySQL安装PHP配置完成本文将会介绍如何把树莓派配置为一台LAMP服务器.这和把XUbuntu配成LAMP服务器有些相似,但是针对树莓派有些需要特殊处理的地方.下面是LAMP服务器的最通用配置:Linux–操作
- Android进行Post提交JSON数据注意事项
kerry1789
Android感慨androidjava单元测试
刚好业务需要写一个测试程序给客户使用,直接入主题吧!请求地址:http://12.32.12.32:91889/testx.php请求参数:{"test1":"顾家家居口味咯咯咯","test2":"把1册","test3":"13512341234","test4":"5rW35Y2X55uR54ux566h55CG5bGA6LCD5bqm5oyH5oyl5bmz5Y","test5":"123
- 树莓派raspberry搭建web服务(基于LAMP)
最古琴
撸了今年阿里、头条和美团的面试,我有一个重要发现.......>>>本文永久地址:https://my.oschina.net/bysu/blog/15502121.安装apachesudoapt-getinstallapache2php-gdphp安装完之后,怎么确认是否安装成功了呢?可以通过以下几种方式确认。a.可以查看是否已有相应的服务ps-ef|grepapache会看到4条服务,其中主进
- Python面向对象编程原则
运维开发小白
python服务器运维
Python面向对象编程原则1.基本概面向对象编程(Object-OrientedProgramming,OOP)是一种编程范式,它使用"对象"来表示现实世界中的事物和它们之间的关系。在Python中,面向对象编程遵循以下原则:封装(Encapsulation):封装是将数据(属性)和操作数据的方法(函数)包装在一个类(Class)中的过程。这隐藏了对象内部的实现细节,只暴露出有限的接口供外部访问
- lighttpd安装和配置https
hailangnet
Debian笔记httpshttplighttpd
aptinstalllighttpdapt-getinstallphp-cgilighttpd-enable-modfastcgifastcgi-phpservicelighttpdforce-reloadlighttpd配置httpssudonano/etc/lighttpd/lighttpd.conf加入:server.modules+=("mod_openssl")$SERVER["sock
- 【C语言开源库】lw_oopc:轻量级的C语言面向对象编程框架
机载软件与适航
C语言C/C++开源库c语言
文章目录轻量级的面向对象C语言编程框架LW_OOPC介绍摘要s为什么要用面向对象?为什么不直接使用C++?LW_OOPC是什么?LW_OOPC宏介绍问题描述解决方案方案的可扩展性如何?LW_OOPC最佳实践LW_OOPC的优点LW_OOPC的缺点总结幕后花絮参考资料轻量级的面向对象C语言编程框架LW_OOPC介绍轻量级的面向对象C语言编程框架LW_OOPC介绍摘要s本文介绍一种轻量级的面向对象的C
- 基于责任链与策略模式的轻量级PHP日志库设计
苏琢玉
策略模式php责任链模式
你有没有遇到过这样的情况:代码被各种人拷来拷去,散落在不同的服务器上,它们运行着同样的代码,却各有各的脾气。A服务器风平浪静,B服务器炸成烟花,C服务器似乎活着但又不太对劲……而你,每天都在面对来自四面八方的“XX功能炸了”“接口500了”“部署完直接寄了”的灵魂拷问。最离谱的是,它们都会从你这同步最新的代码,但到底是代码问题还是服务器环境问题,你根本没办法第一时间知道。于是,问题就变成了:如何把
- Web 开发都需要学什么?
Duiz33237
前端html5css3web
Web开发是指开发和构建用于互联网的网站和应用程序的过程。它涉及使用各种编程语言、框架和技术来创建功能丰富、用户友好的网站和应用程序。常见的web开发技术包括HTML、CSS和JavaScript。HTML用于创建网页的结构,CSS用于样式和布局,而JavaScript用于实现交互和动态效果。此外,还有许多其他的编程语言和框架,如Python、PHP、Ruby、React、Angular等,用于开
- 2025最新版易支付正版源码开源免授权搭建下载
阿辉博客
开源
搭建教程服务器环境推荐使用宝塔、AMH、XP等面板一键部署服务器环境。PHP版本:>=7.1,推荐7.4或8.0MySQL版本:5.6或5.7伪静态配置直接上传后访问即可完成安装!创建好网站之后,需要配置伪静态才能正常发起支付。以下分别是Nginx、Apache、IIS服务器的伪静态配置方法:Nginx如果是Nginx,伪静态规则在源码包根目录的nginx.txt文件里面。将nginx.txt里面
- PHP语言的区块链扩展性
叶雅茗
包罗万象golang开发语言后端
PHP语言的区块链扩展性引言区块链技术因其去中心化、透明性和不可篡改的特性而备受关注,已被广泛应用于金融、物流、供应链管理、数字身份等多个领域。而在构建区块链应用时,开发语言的选择至关重要。PHP作为一种流行的服务器端脚本语言,凭借其简单易学的特性和丰富的生态系统,逐渐开始在区块链应用开发中崭露头角。本文将探讨PHP语言在区块链应用中的扩展性,包括其优点、局限性,以及如何利用现有的框架和库来构建高
- php中文乱码问号,如何解决PHP中文乱码问题?
Helios-Yang
php中文乱码问号
作为该国家/区域内信息处理的基础,字符编码集起着统一编码的重要作用。字符编码集按长度分为SBCS(单字节字符集),DBCS(双字节字符集)两大类。早期的软件(尤其是操作系统),为了解决本地字符信息的计算机处理,出现了各种本地化版本(L10N),为了区分,引进了LANG,Codepage等概念。但是由于各个本地字符集代码范围重叠,相互间信息交换困难;软件各个本地化版本独立维护成本较高。因此有必要将本
- php中文乱码无法解决_PHP基础|如何解决中文乱码问题?
梦里一只喵
php中文乱码无法解决
为什么会出现中文乱码?很多新手朋友学习PHP的时候,发现程序中的中文在输出的时候会出现乱码的问题,那么为什么会出现这种乱码的情况呢?一般来说,乱码的出现有2种原因,一种是由于编码(charset)设置错误,导致浏览器以错误的编码来解析,从而出现了满屏乱七八糟的“天书”,第二种就是文件被以错误的编码打开,然后保存,比如一个文本文件原先是GB2312编码的,却以UTF-8编码打开再保存,就会出现乱码的
- PHP 处理csv 文件 解决中文乱码
MountainYanYL
PHPcsvphp
/***读取csv格式的数据*@param$file*@returnarray*/publicstaticfunctionread_csv($file){setlocale(LC_ALL,'zh_CN');//linux系统下生效$data=[];//返回的文件数据行if(!is_file($file)&&!file_exists($file)){return$data;}$cvs_file=fo
- 遍历dom 并且存储(将每一层的DOM元素存在数组中)
换个号韩国红果果
JavaScripthtml
数组从0开始!!
var a=[],i=0;
for(var j=0;j<30;j++){
a[j]=[];//数组里套数组,且第i层存储在第a[i]中
}
function walkDOM(n){
do{
if(n.nodeType!==3)//筛选去除#text类型
a[i].push(n);
//con
- Android+Jquery Mobile学习系列(9)-总结和代码分享
白糖_
JQuery Mobile
目录导航
经过一个多月的边学习边练手,学会了Android基于Web开发的毛皮,其实开发过程中用Android原生API不是很多,更多的是HTML/Javascript/Css。
个人觉得基于WebView的Jquery Mobile开发有以下优点:
1、对于刚从Java Web转型过来的同学非常适合,只要懂得HTML开发就可以上手做事。
2、jquerym
- impala参考资料
dayutianfei
impala
记录一些有用的Impala资料
1. 入门资料
>>官网翻译:
http://my.oschina.net/weiqingbin/blog?catalog=423691
2. 实用进阶
>>代码&架构分析:
Impala/Hive现状分析与前景展望:http
- JAVA 静态变量与非静态变量初始化顺序之新解
周凡杨
java静态非静态顺序
今天和同事争论一问题,关于静态变量与非静态变量的初始化顺序,谁先谁后,最终想整理出来!测试代码:
import java.util.Map;
public class T {
public static T t = new T();
private Map map = new HashMap();
public T(){
System.out.println(&quo
- 跳出iframe返回外层页面
g21121
iframe
在web开发过程中难免要用到iframe,但当连接超时或跳转到公共页面时就会出现超时页面显示在iframe中,这时我们就需要跳出这个iframe到达一个公共页面去。
首先跳转到一个中间页,这个页面用于判断是否在iframe中,在页面加载的过程中调用如下代码:
<script type="text/javascript">
//<!--
function
- JAVA多线程监听JMS、MQ队列
510888780
java多线程
背景:消息队列中有非常多的消息需要处理,并且监听器onMessage()方法中的业务逻辑也相对比较复杂,为了加快队列消息的读取、处理速度。可以通过加快读取速度和加快处理速度来考虑。因此从这两个方面都使用多线程来处理。对于消息处理的业务处理逻辑用线程池来做。对于加快消息监听读取速度可以使用1.使用多个监听器监听一个队列;2.使用一个监听器开启多线程监听。
对于上面提到的方法2使用一个监听器开启多线
- 第一个SpringMvc例子
布衣凌宇
spring mvc
第一步:导入需要的包;
第二步:配置web.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi=
- 我的spring学习笔记15-容器扩展点之PropertyOverrideConfigurer
aijuans
Spring3
PropertyOverrideConfigurer类似于PropertyPlaceholderConfigurer,但是与后者相比,前者对于bean属性可以有缺省值或者根本没有值。也就是说如果properties文件中没有某个bean属性的内容,那么将使用上下文(配置的xml文件)中相应定义的值。如果properties文件中有bean属性的内容,那么就用properties文件中的值来代替上下
- 通过XSD验证XML
antlove
xmlschemaxsdvalidationSchemaFactory
1. XmlValidation.java
package xml.validation;
import java.io.InputStream;
import javax.xml.XMLConstants;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schem
- 文本流与字符集
百合不是茶
PrintWrite()的使用字符集名字 别名获取
文本数据的输入输出;
输入;数据流,缓冲流
输出;介绍向文本打印格式化的输出PrintWrite();
package 文本流;
import java.io.FileNotFound
- ibatis模糊查询sqlmap-mapping-**.xml配置
bijian1013
ibatis
正常我们写ibatis的sqlmap-mapping-*.xml文件时,传入的参数都用##标识,如下所示:
<resultMap id="personInfo" class="com.bijian.study.dto.PersonDTO">
<res
- java jvm常用命令工具——jdb命令(The Java Debugger)
bijian1013
javajvmjdb
用来对core文件和正在运行的Java进程进行实时地调试,里面包含了丰富的命令帮助您进行调试,它的功能和Sun studio里面所带的dbx非常相似,但 jdb是专门用来针对Java应用程序的。
现在应该说日常的开发中很少用到JDB了,因为现在的IDE已经帮我们封装好了,如使用ECLI
- 【Spring框架二】Spring常用注解之Component、Repository、Service和Controller注解
bit1129
controller
在Spring常用注解第一步部分【Spring框架一】Spring常用注解之Autowired和Resource注解(http://bit1129.iteye.com/blog/2114084)中介绍了Autowired和Resource两个注解的功能,它们用于将依赖根据名称或者类型进行自动的注入,这简化了在XML中,依赖注入部分的XML的编写,但是UserDao和UserService两个bea
- cxf wsdl2java生成代码super出错,构造函数不匹配
bitray
super
由于过去对于soap协议的cxf接触的不是很多,所以遇到了也是迷糊了一会.后来经过查找资料才得以解决. 初始原因一般是由于jaxws2.2规范和jdk6及以上不兼容导致的.所以要强制降为jaxws2.1进行编译生成.我们需要少量的修改:
我们原来的代码
wsdl2java com.test.xxx -client http://.....
修改后的代
- 动态页面正文部分中文乱码排障一例
ronin47
公司网站一部分动态页面,早先使用apache+resin的架构运行,考虑到高并发访问下的响应性能问题,在前不久逐步开始用nginx替换掉了apache。 不过随后发现了一个问题,随意进入某一有分页的网页,第一页是正常的(因为静态化过了);点“下一页”,出来的页面两边正常,中间部分的标题、关键字等也正常,唯独每个标题下的正文无法正常显示。 因为有做过系统调整,所以第一反应就是新上
- java-54- 调整数组顺序使奇数位于偶数前面
bylijinnan
java
import java.util.Arrays;
import java.util.Random;
import ljn.help.Helper;
public class OddBeforeEven {
/**
* Q 54 调整数组顺序使奇数位于偶数前面
* 输入一个整数数组,调整数组中数字的顺序,使得所有奇数位于数组的前半部分,所有偶数位于数组的后半
- 从100PV到1亿级PV网站架构演变
cfyme
网站架构
一个网站就像一个人,存在一个从小到大的过程。养一个网站和养一个人一样,不同时期需要不同的方法,不同的方法下有共同的原则。本文结合我自已14年网站人的经历记录一些架构演变中的体会。 1:积累是必不可少的
架构师不是一天练成的。
1999年,我作了一个个人主页,在学校内的虚拟空间,参加了一次主页大赛,几个DREAMWEAVER的页面,几个TABLE作布局,一个DB连接,几行PHP的代码嵌入在HTM
- [宇宙时代]宇宙时代的GIS是什么?
comsci
Gis
我们都知道一个事实,在行星内部的时候,因为地理信息的坐标都是相对固定的,所以我们获取一组GIS数据之后,就可以存储到硬盘中,长久使用。。。但是,请注意,这种经验在宇宙时代是不能够被继续使用的
宇宙是一个高维时空
- 详解create database命令
czmmiao
database
完整命令
CREATE DATABASE mynewdb USER SYS IDENTIFIED BY sys_password USER SYSTEM IDENTIFIED BY system_password LOGFILE GROUP 1 ('/u01/logs/my/redo01a.log','/u02/logs/m
- 几句不中听却不得不认可的话
datageek
1、人丑就该多读书。
2、你不快乐是因为:你可以像猪一样懒,却无法像只猪一样懒得心安理得。
3、如果你太在意别人的看法,那么你的生活将变成一件裤衩,别人放什么屁,你都得接着。
4、你的问题主要在于:读书不多而买书太多,读书太少又特爱思考,还他妈话痨。
5、与禽兽搏斗的三种结局:(1)、赢了,比禽兽还禽兽。(2)、输了,禽兽不如。(3)、平了,跟禽兽没两样。结论:选择正确的对手很重要。
6
- 1 14:00 PHP中的“syntax error, unexpected T_PAAMAYIM_NEKUDOTAYIM”错误
dcj3sjt126com
PHP
原文地址:http://www.kafka0102.com/2010/08/281.html
因为需要,今天晚些在本机使用PHP做些测试,PHP脚本依赖了一堆我也不清楚做什么用的库。结果一跑起来,就报出类似下面的错误:“Parse error: syntax error, unexpected T_PAAMAYIM_NEKUDOTAYIM in /home/kafka/test/
- xcode6 Auto layout and size classes
dcj3sjt126com
ios
官方GUI
https://developer.apple.com/library/ios/documentation/UserExperience/Conceptual/AutolayoutPG/Introduction/Introduction.html
iOS中使用自动布局(一)
http://www.cocoachina.com/ind
- 通过PreparedStatement批量执行sql语句【sql语句相同,值不同】
梦见x光
sql事务批量执行
比如说:我有一个List需要添加到数据库中,那么我该如何通过PreparedStatement来操作呢?
public void addCustomerByCommit(Connection conn , List<Customer> customerList)
{
String sql = "inseret into customer(id
- 程序员必知必会----linux常用命令之十【系统相关】
hanqunfeng
Linux常用命令
一.linux快捷键
Ctrl+C : 终止当前命令
Ctrl+S : 暂停屏幕输出
Ctrl+Q : 恢复屏幕输出
Ctrl+U : 删除当前行光标前的所有字符
Ctrl+Z : 挂起当前正在执行的进程
Ctrl+L : 清除终端屏幕,相当于clear
二.终端命令
clear : 清除终端屏幕
reset : 重置视窗,当屏幕编码混乱时使用
time com
- NGINX
IXHONG
nginx
pcre 编译安装 nginx
conf/vhost/test.conf
upstream admin {
server 127.0.0.1:8080;
}
server {
listen 80;
&
- 设计模式--工厂模式
kerryg
设计模式
工厂方式模式分为三种:
1、普通工厂模式:建立一个工厂类,对实现了同一个接口的一些类进行实例的创建。
2、多个工厂方法的模式:就是对普通工厂方法模式的改进,在普通工厂方法模式中,如果传递的字符串出错,则不能正确创建对象,而多个工厂方法模式就是提供多个工厂方法,分别创建对象。
3、静态工厂方法模式:就是将上面的多个工厂方法模式里的方法置为静态,
- Spring InitializingBean/init-method和DisposableBean/destroy-method
mx_xiehd
javaspringbeanxml
1.initializingBean/init-method
实现org.springframework.beans.factory.InitializingBean接口允许一个bean在它的所有必须属性被BeanFactory设置后,来执行初始化的工作,InitialzingBean仅仅指定了一个方法。
通常InitializingBean接口的使用是能够被避免的,(不鼓励使用,因为没有必要
- 解决Centos下vim粘贴内容格式混乱问题
qindongliang1922
centosvim
有时候,我们在向vim打开的一个xml,或者任意文件中,拷贝粘贴的代码时,格式莫名其毛的就混乱了,然后自己一个个再重新,把格式排列好,非常耗时,而且很不爽,那么有没有办法避免呢? 答案是肯定的,设置下缩进格式就可以了,非常简单: 在用户的根目录下 直接vi ~/.vimrc文件 然后将set pastetoggle=<F9> 写入这个文件中,保存退出,重新登录,
- netty大并发请求问题
tianzhihehe
netty
多线程并发使用同一个channel
java.nio.BufferOverflowException: null
at java.nio.HeapByteBuffer.put(HeapByteBuffer.java:183) ~[na:1.7.0_60-ea]
at java.nio.ByteBuffer.put(ByteBuffer.java:832) ~[na:1.7.0_60-ea]
- Hadoop NameNode单点问题解决方案之一 AvatarNode
wyz2009107220
NameNode
我们遇到的情况
Hadoop NameNode存在单点问题。这个问题会影响分布式平台24*7运行。先说说我们的情况吧。
我们的团队负责管理一个1200节点的集群(总大小12PB),目前是运行版本为Hadoop 0.20,transaction logs写入一个共享的NFS filer(注:NetApp NFS Filer)。
经常遇到需要中断服务的问题是给hadoop打补丁。 DataNod