今后会多收集项目中常用的小功能,提高以后的开发效率,好记星不如烂笔头,好好写博客,好好学习。
1.验证EditText
/** * <判断EditText是否为空> * @param edText * @return * @see [类、类#方法、类#成员] */
public static boolean isEmptyEditText(EditText edText)
{
if (edText.getText().toString().trim().length() > 0)
return false;
else
return true;
}
/** * <验证邮箱是否合法> * @param email * @return * @see [类、类#方法、类#成员] */
public static boolean isEmailIdValid(String email)
{
String expression = "^[\\w\\.-]+@([\\w\\-]+\\.)+[A-Z]{2,4}$";
CharSequence inputStr = email;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputStr);
if (matcher.matches())
return true;
else
return false;
}
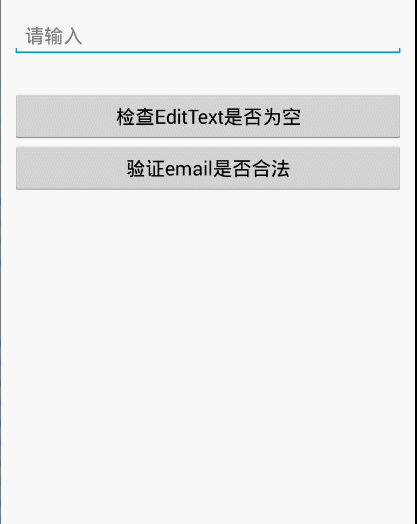
2.检查网络连接状态
/** * 检查网络连接状态 * * @param context * @return true or false */
public static boolean isNetworkAvailable(Context context)
{
ConnectivityManager cm = (ConnectivityManager)context.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo netInfo = cm.getActiveNetworkInfo();
if (netInfo != null && netInfo.isConnectedOrConnecting())
{
return true;
}
return false;
}
3.获取应用图标
/** * <获取当前应用的图标> * @param mContext * @return * @see [类、类#方法、类#成员] */
public static Drawable getAppIcon(Context mContext)
{
Drawable icon = null;
final PackageManager pm = mContext.getPackageManager();
String packageName = mContext.getPackageName();
try
{
icon = pm.getApplicationIcon(packageName);
return icon;
}
catch (NameNotFoundException e1)
{
e1.printStackTrace();
}
return null;
}
4.发送本地通知
@SuppressLint("NewApi")
@SuppressWarnings({"static-access"})
public static void sendLocatNotification(Context mContext, String title, String message, Intent mIntent)
{
int appIconResId = 0
PendingIntent pIntent = null
if (mIntent != null)
pIntent = PendingIntent.getActivity(mContext, 0, mIntent, PendingIntent.FLAG_UPDATE_CURRENT)
final PackageManager pm = mContext.getPackageManager()
String packageName = mContext.getPackageName()
ApplicationInfo applicationInfo
try
{
applicationInfo = pm.getApplicationInfo(packageName, PackageManager.GET_META_DATA)
appIconResId = applicationInfo.icon
}
catch (NameNotFoundException e1)
{
e1.printStackTrace()
}
// Notification notification = new Notification.Builder(mContext)
// .setSmallIcon(appIconResId).setWhen(System.currentTimeMillis())
// .setContentTitle(title).setContentText(message)
// .setContentIntent(pIntent).getNotification()
Notification notification
if (mIntent == null)
{
notification =
new Notification.Builder(mContext).setSmallIcon(appIconResId)
.setWhen(System.currentTimeMillis())
.setContentTitle(message)
.setStyle(new Notification.BigTextStyle().bigText(message))
.setAutoCancel(true)
.setContentText(message)
.setContentIntent(PendingIntent.getActivity(mContext, 0, new Intent(), 0))
.getNotification()
}
else
{
notification =
new Notification.Builder(mContext).setSmallIcon(appIconResId)
.setWhen(System.currentTimeMillis())
.setContentTitle(message)
.setContentText(message)
.setAutoCancel(true)
.setStyle(new Notification.BigTextStyle().bigText(message))
.setContentIntent(pIntent)
.getNotification()
}
// 点击之后清除通知
notification.flags |= Notification.FLAG_AUTO_CANCEL
// 开启通知提示音
notification.defaults |= Notification.DEFAULT_SOUND
// 开启震动
notification.defaults |= Notification.DEFAULT_VIBRATE
NotificationManager manager = (NotificationManager)mContext.getSystemService(mContext.NOTIFICATION_SERVICE)
// manager.notify(0, notification)
manager.notify(R.string.app_name, notification)
}
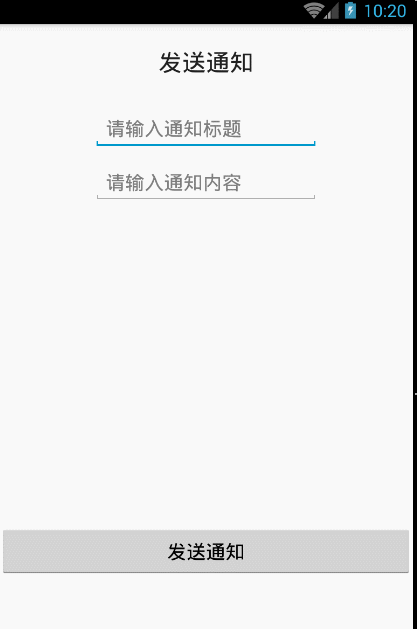
5.随机获取
/** * <随机获取字母a-z> * @return random num * @see [类、类#方法、类#成员] */
public static char getRandomCharacter()
{
Random r = new Random();
char c = (char)(r.nextInt(26) + 'a');
return c;
}
/** * <获取0到number之间的随机数> * @param number * @return * @see [类、类#方法、类#成员] */
public static int getRandom(int number)
{
Random rand = new Random();
return rand.nextInt(number);
}
6.打开日期和时间选择器
private static Calendar dateTime = Calendar.getInstance();
/** * 打开日期选择器 * * @param mContext * @param format * @param mTextView 要显示的TextView */
public static void showDatePickerDialog(final Context mContext, final String format, final TextView mTextView)
{
new DatePickerDialog(mContext, new OnDateSetListener()
{
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth)
{
SimpleDateFormat dateFormatter = new SimpleDateFormat(format);
dateTime.set(year, monthOfYear, dayOfMonth);
mTextView.setText(dateFormatter.format(dateTime.getTime()).toString());
}
}, dateTime.get(Calendar.YEAR), dateTime.get(Calendar.MONTH), dateTime.get(Calendar.DAY_OF_MONTH)).show();
}
/** * 时间选择器 * * @param mContext * @param mTextView 要显示的TextView * @return show timepicker */
public static void showTimePickerDialog(final Context mContext, final TextView mTextView)
{
new TimePickerDialog(mContext, new OnTimeSetListener()
{
@Override
public void onTimeSet(TimePicker view, int hourOfDay, int minute)
{
SimpleDateFormat timeFormatter = new SimpleDateFormat("hh:mm a");
dateTime.set(Calendar.HOUR_OF_DAY, hourOfDay);
dateTime.set(Calendar.MINUTE, minute);
mTextView.setText(timeFormatter.format(dateTime.getTime()).toString());
}
}, dateTime.get(Calendar.HOUR_OF_DAY), dateTime.get(Calendar.MINUTE), false).show();
}
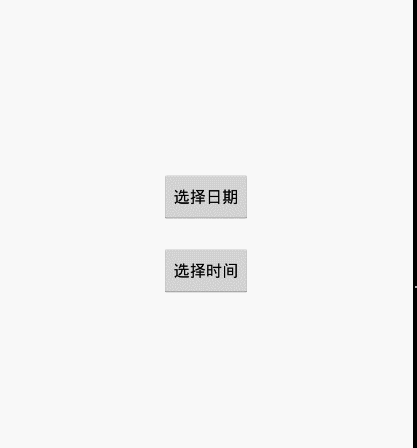
7.获取设备宽高
/** * 获取设备高度 * * @param mContext * @return 设备高度 */
public static int getDeviceHeight(Context mContext)
{
DisplayMetrics displaymetrics = new DisplayMetrics();
((Activity)mContext).getWindowManager().getDefaultDisplay().getMetrics(displaymetrics);
return displaymetrics.heightPixels;
}
/** * 获取设备宽度 * * @param mContext * @return 设备宽度 */
public static int getDeviceWidth(Context mContext)
{
DisplayMetrics displaymetrics = new DisplayMetrics();
((Activity)mContext).getWindowManager().getDefaultDisplay().getMetrics(displaymetrics);
return displaymetrics.widthPixels;
}
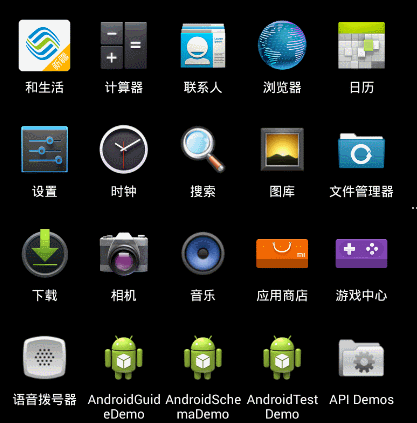
8.String和List互转
/** * <String转化为List> * @param string * @return * @see [类、类#方法、类#成员] */
public static List<String> stringToArrayList(String string)
{
List<String> strValueList = new ArrayList<String>();
strValueList = Arrays.asList(string.split(","));
return strValueList;
}
/** * <List转化为String> * @param string * @return * @see [类、类#方法、类#成员] */
public static String arrayListToString(List<String> list)
{
String strValue = null;
StringBuilder sb = new StringBuilder();
for (String s : list)
{
sb.append(s + ",");
strValue = sb.toString();
}
if (strValue.length() > 0 && strValue.charAt(strValue.length() - 1) == ',')
{
strValue = strValue.substring(0, strValue.length() - 1);
}
return strValue;
}
9.Bitmap和Drawable互转
/** * * <drawable转bitmap> * @param mContext * @param drawable * @return * @see [类、类#方法、类#成员] */
public static Bitmap drawableTobitmap(Context mContext, int drawable)
{
Drawable myDrawable = mContext.getResources().getDrawable(drawable);
return ((BitmapDrawable)myDrawable).getBitmap();
}
/** * * <bitmap转drawable> * @param mContext * @param drawable * @return * @see [类、类#方法、类#成员] */
public static Drawable bitmapToDrawable(Context mContext, Bitmap bitmap)
{
return new BitmapDrawable(bitmap);
}
10.获取应用版本号
/** * <获取应用版本号> * @param mContext * @return * @see [类、类#方法、类#成员] */
public static int getAppVersionCode(Context mContext)
{
PackageInfo pInfo = null;
try
{
pInfo = mContext.getPackageManager().getPackageInfo(mContext.getPackageName(), 0);
}
catch (NameNotFoundException e)
{
e.printStackTrace();
}
return pInfo.versionCode;
}