package assignment2;
import java.awt.*;
import javax.swing.*;
public class DrawingGraphics extends JFrame{
public DrawingGraphics(){
super("Drawing curve of the function");
setSize(1000,1000);
setVisible(true);
}
public void paint(Graphics g)
{
super.paint(g);
int mid = 500;
g.setColor(Color.black);
g.drawLine(150,mid,850,mid);
g.drawLine(mid,80,mid,850);
int interval = 70;
for(int i = 1; i < 10; i++)
{
g.drawLine(150+i*interval,mid-10,150+i*interval,mid);
g.drawString(((-2.5 + 0.5*i) + ""),145+i*interval,mid+15);
}
for(int i = 1; i <= 10; i++)
{
g.drawLine(mid+10,80+i*interval,mid,80+i*interval);
g.drawString((12-2*i) + "", mid - 15, 85+i*interval);
}
int xValues0[] = {mid-10,mid+10,mid};
int yValues0[] = {80,80,60};
int xValues1[] = {850,850,870};
int yValues1[] = {mid+10,mid-10,mid};
Polygon polygon0 = new Polygon(xValues0,yValues0,3);
Polygon polygon1 = new Polygon(xValues1,yValues1,3);
g.fillPolygon(polygon0);
g.fillPolygon(polygon1);
g.setColor(Color.red);
int xValues2[] = new int[100];
int yValues2[] = new int[100];
int j = 0;
for(double i = -2; i <= 2; i += 0.1)
{
xValues2[j] = (int)(i*140+mid);
yValues2[j] = (int)(mid - (Math.pow(Math.E, i))*35);
j++;
}
for(int i = 0; i+1 < j; i += 2)
g.drawLine(xValues2[i],yValues2[i], xValues2[i+1], yValues2[i+1]);
g.setColor(Color.blue);
j = 0;
for(double i = -2; i <= 2; i+= 0.1)
{
xValues2[j] = (int)(i*140+mid);
yValues2[j] = (int)(mid - (i*i+1)*35);
j++;
}
for(int i = 0; i+1 < j; i += 2)
g.drawLine(xValues2[i],yValues2[i], xValues2[i+1], yValues2[i+1]);
g.setColor(Color.gray);
j = 0;
for(double i = -2; i <= 2; i+= 0.1)
{
xValues2[j] = (int)(i*140+mid);
yValues2[j] = (int)(mid - (i*i*i+1)*35);
j++;
}
for(int i = 0; i+1 < j; i += 2)
g.drawLine(xValues2[i],yValues2[i], xValues2[i+1], yValues2[i+1]);
g.setColor(Color.black);
g.drawRect(650, 650, 120, 150);
g.drawString("e", 720, 680);
g.drawString("x", 725, 670);
g.drawString("x + 1", 720, 730);
g.drawString("2", 725, 720);
g.drawString("x + 1", 720, 780);
g.drawString("3", 725, 770);
g.setColor(Color.red);
g.drawLine(660, 675, 710, 675);
g.setColor(Color.blue);
g.drawLine(660, 725, 710, 725);
g.setColor(Color.gray);
g.drawLine(660, 775, 710, 775);
}
public static void main(String args[])
{
DrawingGraphics application = new DrawingGraphics();
application.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
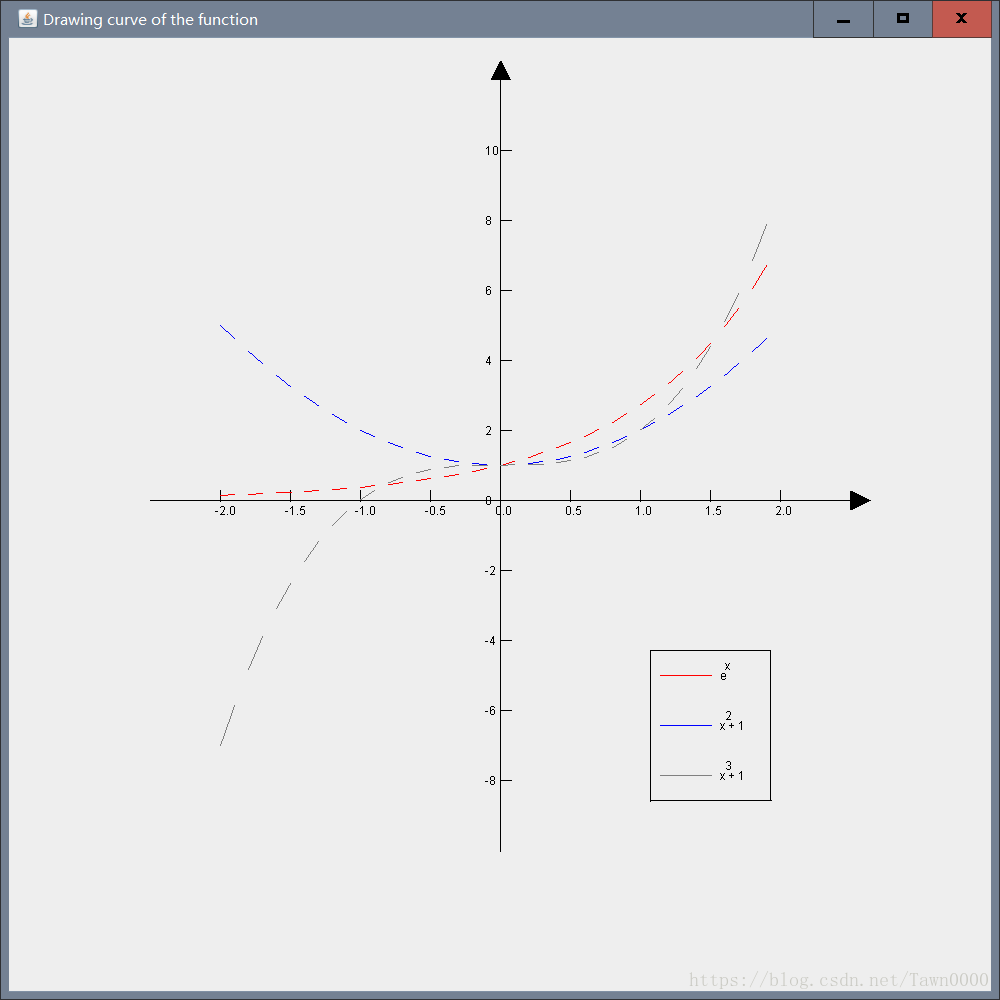
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
public class Main
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
JFrame frame = new DrawFrame();
frame.setTitle("Draw2D");
frame.setSize(600,600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
class DrawFrame extends JFrame
{
public DrawFrame()
{
add(new DrawComponent());
pack();
}
}
class DrawComponent extends JComponent
{
private static final int DEAFAULT_WIDTH = 500;
private static final int DEAFAULT_HEIGHT = 500;
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D)g;
double X = 100;
double Y = 100;
double w = 300;
double h = 200;
Rectangle2D re = new Rectangle2D.Double(X,Y,w,h);
g2.draw(re);
Ellipse2D e = new Ellipse2D.Double(X,Y,w,h);
g2.draw(e);
e.setFrame(re);
g2.draw(e);
double rx = re.getCenterX();
double ry = re.getCenterY();
double radius = 200;
Ellipse2D c = new Ellipse2D.Double();
c.setFrameFromCenter(rx,ry,rx + radius,ry + radius);
g2.draw(c);
}
public Dimension getPreferredSize(){return new Dimension(DEAFAULT_WIDTH,DEAFAULT_HEIGHT);}
}
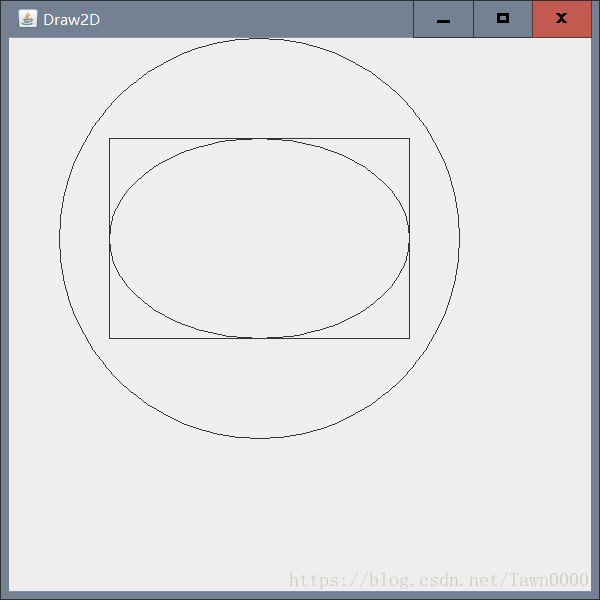
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
public class Main
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
JFrame frame = new DrawFrame();
frame.setTitle("Draw2D");
frame.setSize(600,600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
class DrawFrame extends JFrame
{
public DrawFrame()
{
add(new DrawComponent());
pack();
}
}
class DrawComponent extends JComponent
{
private static final int DEAFAULT_WIDTH = 500;
private static final int DEAFAULT_HEIGHT = 500;
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D)g;
double X = 100;
double Y = 100;
double w = 300;
double h = 200;
g2.setPaint(Color.RED);
Rectangle2D re = new Rectangle2D.Double(X,Y,w,h);
g2.fill(re);
g2.setPaint(Color.YELLOW);
Ellipse2D e = new Ellipse2D.Double(X,Y,w,h);
g2.fill(e);
e.setFrame(re);
g2.draw(e);
double rx = re.getCenterX();
double ry = re.getCenterY();
double radius = 100;
g2.setPaint(Color.GREEN);
Ellipse2D c = new Ellipse2D.Double();
c.setFrameFromCenter(rx,ry,rx + radius,ry + radius);
g2.fill(c);
}
public Dimension getPreferredSize(){return new Dimension(DEAFAULT_WIDTH,DEAFAULT_HEIGHT);}
}
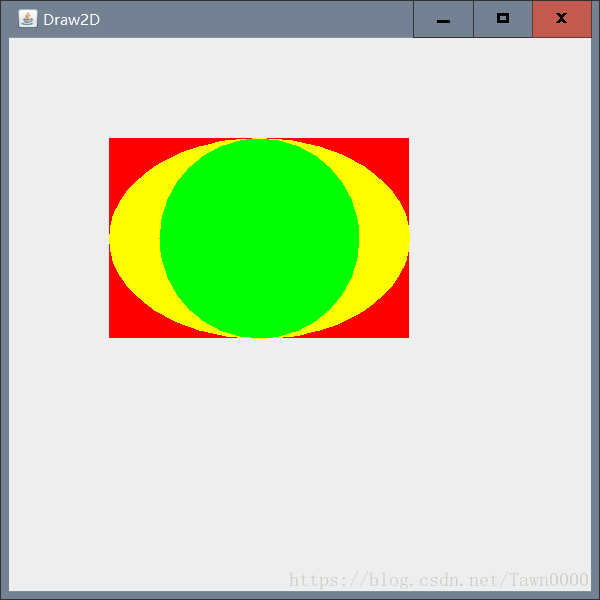