GUI 这个东西重要性还是很重要的,所谓GUI就是图形用户界面。与用户直接进行交互的部分,自然重要,当然游戏整个运行中都是在与用户进行交互。Unity自带了一套GUI工具,虽然可能比较简陋,但是对于我这种初学者来说还是有很多帮助的。
OnGUI: Called multiple times per frame in response to GUI events. The Layout and Repaint events are processed first, followed by a Layout and keyboard/mouse event for each input event.
这个函数其实就是用来渲染GUI以及相应处理GUI事件的函数。每一帧可能被调起很多次来处理这些事件。
Canvas是一种带有canvas组件的GameObject。用来包含UI控件元素,所有UI元素都必须是这种Canvas的子对象。整个canvas画布在场景视图中是一个矩形。
包含在画布里面的UI元素会按照从上到下这样的先后顺序进行渲染,所以后渲染的元素是有可能覆盖掉前面先渲染的元素的,这就像刷油漆,你在一面墙上刷了第一个方块,再刷第二个的时候不小心刷到第一个,当然第一个就被你的第二个挡住了。
其实就是创建一些button,对应计算器的按钮,然后附上相应的行为,计算器的实现主要是双栈求值。
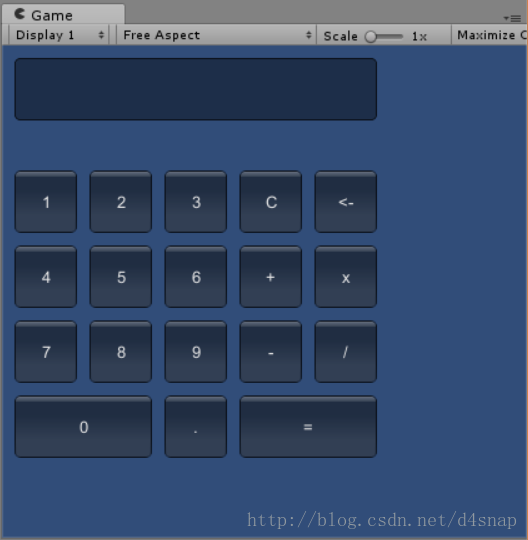
下面是具体代码。
using UnityEngine;
using System.Collections;
public class GUIcreater : MonoBehaviour {
public GUIStyle buttonstyle;
string text="";
public class mycocluator{
float[] operation_number = new float[100];
int on_top = -1;
char[] operation = new char[100];
int o_top = -1;
public string computer(string s) {
int i = 0;
while (i < s.Length) {
switch (s [i]) {
case '+':
operation [o_top + 1] = '+';
o_top++;
break;
case '-':
operation [o_top + 1] = '-';
o_top++;
break;
case '*':
operation [o_top + 1] = '*';
o_top++;
break;
case '/':
operation [o_top + 1] = '/';
o_top++;
break;
}
int j = 0;
string temps = "";
while (((i+j) < s.Length) && (s [i + j] == '.' || (s [i + j] <= '9' && s [i + j] >= '0'))) {
temps += s [i + j];
j++;
}
if (j != 0) {
float temp = float.Parse (temps);
on_top++;
operation_number [on_top] = temp;
i += j;
} else {
i++;
}
}
int k = 0;
while (o_top > -1) {
Debug.Log (k);
k++;
float temp1, temp2;
char tempc;
Debug.Log ("o_top:" + o_top);
if (o_top == -1)
break;
tempc = operation [o_top];
o_top--;
Debug.Log ("o_top:" + o_top);
temp1 = operation_number [on_top];
on_top--;
temp2 = operation_number [on_top];
on_top--;
switch (tempc) {
case '+':
operation_number [on_top + 1] = temp1 + temp2;
on_top++;
break;
case '-':
operation_number [on_top + 1] = temp2 - temp1;
on_top++;
break;
case '*':
operation_number [on_top + 1] = temp1 * temp2;
on_top++;
break;
case '/':
operation_number [on_top + 1] = temp2 / temp1;
on_top++;
break;
}
}
Debug.Log (operation_number [on_top].ToString ());
return operation_number [on_top].ToString();
}
}
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
}
void OnGUI(){
GUI.Box(new Rect (10, 10, 290, 50), text);
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (GUI.Button (new Rect (10 + i * 60, 100 + j * 60, 50, 50), 1 + j * 3 + i + "", "button")) {
text += 1 + j * 3 + i;
}
}
}
if (GUI.Button (new Rect (190, 100 , 50, 50), "C", "button")) {
text ="";
}
if (GUI.Button (new Rect (250, 100 , 50, 50), "<-", "button")) {
if (text.Length > 0)
text = text.Remove(text.Length-1,1) ;
}
if (GUI.Button (new Rect (10, 280 , 110, 50), "0", "button")) {
text +="0";
}
if (GUI.Button (new Rect (130, 280 , 50, 50), ".", "button")) {
text +=".";
}
if (GUI.Button (new Rect (190, 160 , 50, 50), "+", "button")) {
text +="+";
}
if (GUI.Button (new Rect (190, 220 , 50, 50), "-", "button")) {
text +="-";
}
if (GUI.Button (new Rect (250, 160 , 50, 50), "x", "button")) {
text +="*";
}
if (GUI.Button (new Rect (250, 220 , 50, 50), "/", "button")) {
text +="/";
}
if (GUI.Button (new Rect (190, 280 , 110, 50), "=", "button")) {
mycocluator c = new mycocluator ();
text = c.computer(text);
}
}
}