demo的结构:
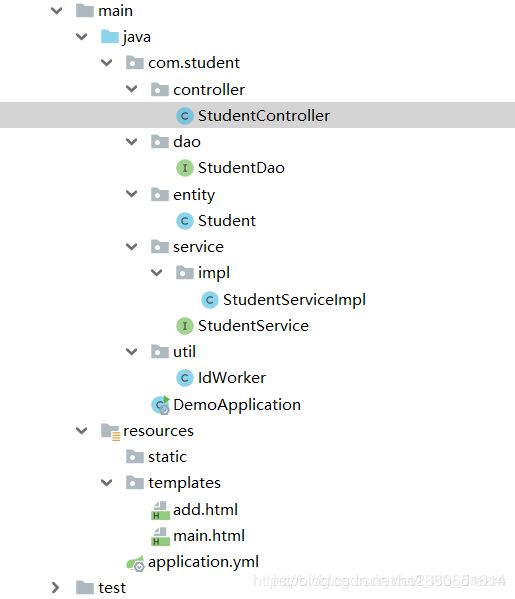
配置文件:
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/student?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
username: root
password: root
jpa:
show-sql: true
hibernate:
ddl-auto: update
naming:
implicit-strategy: org.hibernate.boot.model.naming.ImplicitNamingStrategyComponentPathImpl
physical-strategy: org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl
database: mysql
pom文件:
<dependencies>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-data-jpaartifactId>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-thymeleafartifactId>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-devtoolsartifactId>
<scope>runtimescope>
<optional>trueoptional>
dependency>
<dependency>
<groupId>mysqlgroupId>
<artifactId>mysql-connector-javaartifactId>
<scope>runtimescope>
dependency>
<dependency>
<groupId>org.projectlombokgroupId>
<artifactId>lombokartifactId>
<optional>trueoptional>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-testartifactId>
<scope>testscope>
dependency>
<dependency>
<groupId>org.junit.jupitergroupId>
<artifactId>junit-jupiter-apiartifactId>
<version>RELEASEversion>
<scope>compilescope>
dependency>
dependencies>
实体类代码:
@Data
@Entity
@Table(name = "students")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String name;
private String sex;
}
dao层代码:
@Repository
public interface StudentDao extends JpaRepository<Student,Integer> {
}
实现层代码:
public interface StudentService {
Student save(Student student);
Student update(Student student);
void delete(Integer id);
Page<Student> findByPage(Integer page, Integer size,Student student);
Student findById(Integer id);
}
接口
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentDao studentDao;
@Override
public Student save(Student student) {
return studentDao.save(student);
}
@Override
public Student update(Student student) {
return studentDao.save(student);
}
@Override
public void delete(Integer id) {
studentDao.deleteById(id);
}
@Override
public Page<Student> findByPage(Integer page, Integer size,Student student) {
ExampleMatcher matcher = ExampleMatcher.matching().withMatcher("name",ExampleMatcher.GenericPropertyMatchers.startsWith());
Example<Student> studentExample = Example.of(student,matcher);
PageRequest request = PageRequest.of(page, size);
Page<Student> studentPage =studentDao.findAll(studentExample,request);
return studentPage;
}
@Override
public Student findById(Integer id) {
return studentDao.findById(id).get();
}
}
控制层:
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
@RequestMapping("/add")
public String add(Student student ,Model model){
model.addAttribute("student",student);
return "add";
}
@RequestMapping("/save")
public String save(Student student) {
studentService.update(student);
return "redirect:findByPage";
}
@RequestMapping("/delete")
public String delete(@RequestParam(value = "id",required = false) Integer id,
Model model) {
studentService.delete(id);
return "redirect:findByPage";
}
@RequestMapping("/findByPage")
public String findByPage(@RequestParam(value = "page",required = false) Integer page,
@RequestParam(value = "size",required = false) Integer size,
Model model,Student student) {
if (page == null) {
page = 0;
}
if (size == null) {
size = 2;
}
Page<Student> byPage = studentService.findByPage(page, size,student);
model.addAttribute("student", byPage);
model.addAttribute("name",student.getName());
return "main";
}
@RequestMapping("/findById")
public String findById(@RequestParam(value = "id",required = false) Integer id,
Model model) {
Student byId = studentService.findById(id);
model.addAttribute("student",byId);
return "add";
}
}
html首页:
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Titletitle>
head>
<body>
<form action="/findByPage" method="post">
姓名:<input type="text" name="name"/><br />
<input type="submit" value="查询"/>
form>
<table border="1" cellspacing="0" cellpadding="5">
<tr>
<th>编号th>
<th>姓名th>
<th>性别th>
<th colspan="2">操作th>
tr>
<tr th:each="s:${student}">
<td th:text="${s.id}">td>
<td th:text="${s.name}">td>
<td th:text="${s.sex}">td>
<td><a th:href="@{/findById(id=${s.id})}">修改a>td>
<td><a th:href="@{/delete(id=${s.id})}">删除a>td>
tr>
table>
第<span th:text="${student.getNumber()+1}">span>页/共<span th:text="${student.getTotalPages()}">span>页<br/>
<a th:href="@{/findByPage(page=0,name=${name})}">首页a>
<span th:if="${student.getNumber()} > 0">
<a th:href="@{/findByPage(page=${student.getNumber()- 1},name=${name})}">上一页a>
span>
<span th:if="${student.getNumber()} < ${student.getTotalPages()-1}">
<a th:href="@{/findByPage(page=${student.getNumber()+ 1},name=${name})}">下一页a>
span>
<a th:href="@{/findByPage(page=${student.getTotalPages()-1},name=${name})}">尾页a>
<a href="/add">新增a>
body>
html>
增加和修改页面
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Titletitle>
head>
<body>
<form action="/save" method="post">
<input type="hidden" name="id" th:value="${student.id}" />
姓名:<input type="text" name="name" th:value="${student.name}"/>
性别:<input type="text" name="sex" th:value="${student.sex}"/>
<input type="submit" value="保存"/>
form>
body>
html>