[PAT A1052] Linked list Sorting
注意事项:
1. 输出格式的控制,比如换行
2. n是一个陷阱,最后链表可能会提前结束,所以需要遍历静态链表确定链表的实际长度。
3. 感慨一句,PAT不亏是ZJU的看门武器,对测试数据的封锁,对思维严密性的要求,都在leetcode之上。
#include
#include
using namespace std;
const int maxn = 100010;
struct Node
{
int addr;
int data;
int next;
bool flag;
Node()
{
flag = false;
}
}node[maxn];
bool cmp(const Node &a, const Node &b)
{
if(a.flag == false || b.flag == false)
return a.flag > b.flag;
else
return a.data < b.data;
}
int main()
{
int n, begin, addr;
scanf("%d%d", &n, &begin);
for(int i = 0; i < n; ++i)
{
scanf("%d",&addr);
scanf("%d%d", &node[addr].data, &node[addr].next);
node[addr].addr = addr;
}
int count = 0;
for(int p = begin; p != -1; p = node[p].next)
{
count += 1;
node[p].flag = true;
}
if(count == 0)
printf("0 -1");
else
{
sort(node, node + maxn, cmp);
printf("%d %05d\n", count, node[0].addr);
for(int i = 0; i < count; ++i)
{
if(i != count - 1)
printf("%05d %d %05d\n", node[i].addr, node[i].data, node[i+1].addr);
else
printf("%05d %d -1", node[i].addr, node[i].data);
}
}
return 0;
}

[PAT A1032 ] Sharing
注意事项:
1. = 和== 一定要注意区别
2. 多次使用scanf可能会超时,尽可能的减少使用scanf的次数
3. 注意特殊情况,这就是相当于数学题目中简单情况但是又会忽略的地方,不考虑失分很严重。PAT考察的是细致和耐心。
const int maxn = 100010;
struct Node
{
char data;
int next;
bool flag;
Node()
{
flag = false;
}
}node[maxn];
int main()
{
int addr1, addr2, n;
scanf("%d%d%d", &addr1, &addr2, &n);
int addr, next;
char data;
for(int i = 0; i < n; ++i)
{
scanf("%d %c %d", &addr, &data, &next);
node[addr].data = data;
node[addr].next = next;
}
int p1 = addr1, p2 = addr2;
while(p1 != -1)
{
node[p1].flag = true;
p1 = node[p1].next;
}
while(p2 != -1)
{
if(node[p2].flag == true)
break;
p2 = node[p2].next;
}
if(p2 == -1)
printf("-1");
else
printf("%05d", p2);
return 0;
}

注意事项:
1. 利用字符数组存储每一位数,便于处理’,’
2. 数组最高位可能有多余的’,’,索引回退,去掉多余的’,’
3. PAT通式: 现处理特殊情况
#include
const int maxn = 10;
char buf[maxn];
int main()
{
int a, b, sum;
bool sign = false;
int bufIndex = 0;
int numIndex = 0;
scanf("%d%d", &a, &b);
sum = a + b;
if(sum < 1000 && sum > -1000)
printf("%d", sum);
else
{
if(sum < 0)
{
sign = true;
sum = 0 - sum;
}
while(sum != 0)
{
buf[bufIndex++] = sum % 10 + '0';
sum /= 10;
numIndex += 1;
if(numIndex % 3 == 0)
buf[bufIndex++] = ',';
}
if(buf[--bufIndex] == ',')
bufIndex -= 1;
if(sign)
printf("-");
for(int i = bufIndex; i >= 0; --i)
printf("%c", buf[i]);
}
return 0;
}

[PAT A1025 ] PAT Ranking
注意事项:
思路简单,但是处理起来还是比较麻烦的,PAT题目有时候在输入输出的格式上花的时间比算法本身还要多……
#include
#include
#include
using namespace std;
const int maxn = 100010;
struct Student
{
char registration_number[20];
int score;
int location_number;
int local_rank;
}student[maxn];
bool cmp(const Student &a, const Student &b)
{
return (a.score != b.score) ? a.score > b.score : \
strcmp(a.registration_number, b.registration_number) < 0;
}
int main()
{
int n;
scanf("%d", &n);
int i = 0;
int total = 0;
int studentIndex = 0;
while(i < n)
{
int k;
scanf("%d", &k);
total += k;
for(int j = 0; j < k; ++j)
{
scanf("%s %d", student[studentIndex].registration_number,\
&student[studentIndex].score);
student[studentIndex].location_number = i + 1;
studentIndex += 1;
}
sort(student + studentIndex - k, student + studentIndex, cmp);
student[studentIndex - k].local_rank = 1;
for(int j = studentIndex - k + 1; j < studentIndex; ++j)
{
if(student[j].score == student[j - 1].score)
student[j].local_rank = student[j - 1].local_rank;
else
student[j].local_rank = j + 1 - (studentIndex - k);
}
i += 1;
}
sort(student, student + studentIndex, cmp);
printf("%d\n", total);
int final_rank = 1;
printf("%s %d %d %d\n", student[0].registration_number,final_rank,\
student[0].location_number, student[0].local_rank);
for(int i = 1; i < total; ++i)
{
if(student[i].score == student[i - 1].score)
printf("%s %d %d %d\n", student[i].registration_number,final_rank,\
student[i].location_number, student[i].local_rank);
else
{
final_rank = i + 1;
printf("%s %d %d %d\n", student[i].registration_number,final_rank,\
student[i].location_number, student[i].local_rank);
}
}
return 0;
}

[PAT A1028 ] List Sorting
注意事项:
Presentation Error,这是格式错误,出现这个错误最可能的原因是,在输出结果的后面,多了或少了没什么意义的空格,tab,换行符等等。所以,请先认真检查程序的输出结果是否与标准完!全!一!致!OJ平台对格式的检查可以说是非!常!严!格!
#include
#include
#include
using namespace std;
const int maxn = 100010;
struct Student
{
int ID;
char name[10];
int score;
}student[maxn];
bool cmp1(const Student &a, const Student &b)
{
return a.ID < b.ID;
}
bool cmp2(const Student &a, const Student &b)
{
if(strcmp(a.name, b.name) != 0)
return strcmp(a.name, b.name) < 0;
else
return a.ID < b.ID;
}
bool cmp3(const Student &a, const Student &b)
{
if(a.score != b.score)
return a.score < b.score;
else
return a.ID < b.ID;
}
int main()
{
int n, c;
scanf("%d%d", &n, &c);
for(int i = 0; i < n; ++i)
{
scanf("%d %s %d", &student[i].ID, student[i].name, &student[i].score);
}
if(c == 1)
sort(student, student + n, cmp1);
else if(c == 2)
sort(student, student + n, cmp2);
else
sort(student, student + n, cmp3);
for(int i = 0; i < n; ++i)
{
printf("%06d %s %d\n", student[i].ID, student[i].name, student[i].score);
}
return 0;
}
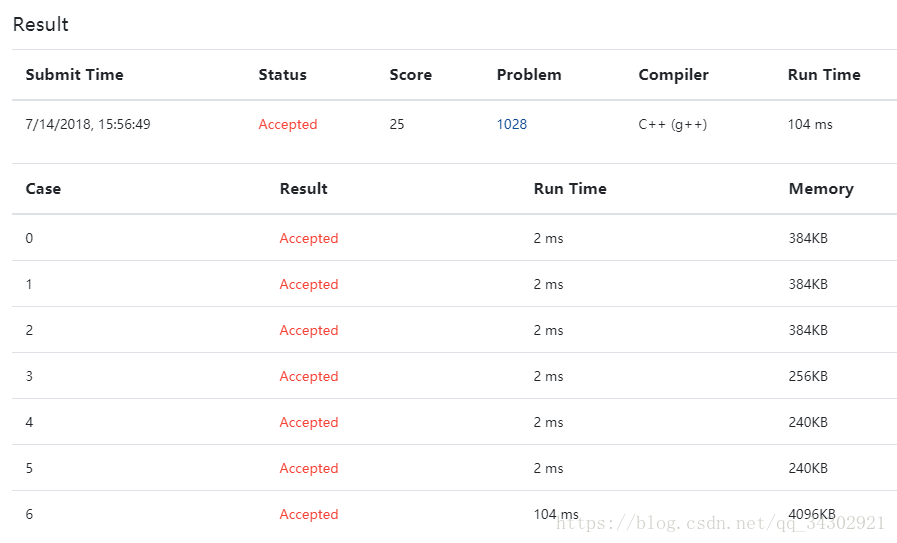
[PAT A1012] Reversible Primes
注意事项:
1. 这道题本身不难,但是花了很长时间,主要是对素数的判断考虑情况不全:判断一个数是否是素数,注意两点:(1)对1进行特判,1不是素数。(2)循环终止条件是i * i <= num, = 不能少。
#include
#include
bool isPrime(int num)
{
if(1 == num)
return false;
for(int i = 2; i * i <= num; ++i)
{
if(num % i == 0)
return false;
}
return true;
}
int reverseWithRadix(int num, int d)
{
int i = 0;
int ans = 0;
while(num != 0)
{
ans = ans * d + num % d;
num /= d;
}
return ans;
}
int main()
{
int n, d;
int i = 0;
while(scanf("%d%d", &n, &d), n > 0)
{
if(isPrime(n) && isPrime(reverseWithRadix(n, d)))
printf("Yes\n");
else
printf("No\n");
}
return 0;
}
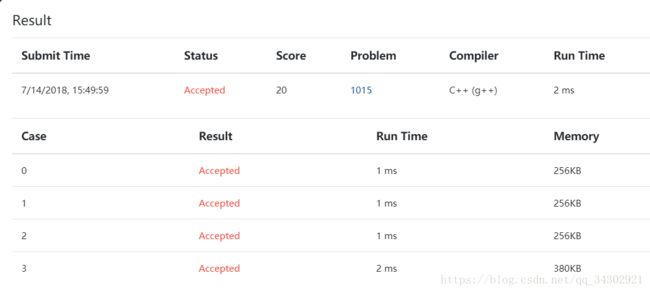
[PAT A1023 ]Have Fun with Numbers
注意事项:
1. 注意读入数据时,数据高位在数组的低位索引
2. 字符数组转换成int只要-‘0’
#include
#include
const int maxn = 11;
int check[maxn];
int *multi(char *num, int len)
{
int *result, carry = 0;
int resLen = (num[0] >= '5') ? (len + 1) : len;
result = new int[resLen];
for(int i = len - 1; i >= 0; --i)
{
int tmp = 2 * (num[i] - '0') + carry;
result[i] = tmp % 10;
carry = tmp / 10;
}
if(carry != 0)
result[len] = carry;
return result;
}
int main()
{
char num[20];
scanf("%s", num);
int len = strlen(num);
for(int i = 0; i < len; ++i)
check[num[i] - '0'] += 1;
int *result= multi(num, len);
int resLen = (num[0] >= '5') ? (len + 1) : len;
if(num[0] >= '5')
printf("No\n");
else
{
for(int i = 0; i < len; ++i)
if(--check[result[i]] == -1)
{
printf("No\n");
goto logo;
}
printf("Yes\n");
}
logo:
if(resLen > len)
printf("%d", result[len]);
for(int i = 0; i < len; ++i)
printf("%d", result[i]);
return 0;
}

[PAT A1015 ]The Best Rank
注意事项:
1. 这个题目直接爆破,竟然没有超时!!!
2. 不要轻易粘贴代码,节省的时间远远比不上Debug的时间。
3. emmm, 写代码要认真……
#include
#include
#include
#include
#include
using namespace std;
const int maxn = 2010;
typedef struct Student
{
char ID[8];
int C, math, English;
int average;
int rank1, rank2, rank3, rank4;
}Student;
bool cmp1(const Student &a, const Student &b)
{
return a.C > b.C;
}
bool cmp2(const Student &a, const Student &b)
{
return a.math > b.math;
}
bool cmp3(const Student &a, const Student &b)
{
return a.English > b.English;
}
bool cmp4(const Student &a, const Student &b)
{
return a.average > b.average;
}
void print(const Student &a)
{
int m = maxn;
char ch;
if(a.rank3 < m)
{
m = a.rank3;
ch = 'E';
}
if(a.rank2 <= m)
{
m = a.rank2;
ch = 'M';
}
if(a.rank1 <= m)
{
m = a.rank1;
ch = 'C';
}
if(a.rank4 <= m)
{
m = a.rank4;
ch = 'A';
}
printf("%d %c\n", m, ch);
}
int main()
{
Student student[maxn];
int n, m;
scanf("%d %d", &n ,&m);
for(int i = 0; i < n; ++i)
{
scanf("%s %d %d %d", student[i].ID, &student[i].C, &student[i].math,\
&student[i].English);
student[i].average = (int)round((student[i].C + student[i].math + student[i].English)\
/ 3.0);
}
sort(student, student + n, cmp1);
student[0].rank1 = 1;
for(int i = 1; i < n; ++i)
{
if(student[i].C == student[i-1].C)
student[i].rank1 = student[i-1].rank1;
else
student[i].rank1 = i + 1;
}
sort(student, student+n, cmp2);
student[0].rank2 = 1;
for(int i = 1; i < n; ++i)
{
if(student[i].math == student[i-1].math)
student[i].rank2 = student[i-1].rank2;
else
student[i].rank2 = i + 1;
}
sort(student, student+n, cmp3);
student[0].rank3 = 1;
for(int i = 1; i < n; ++i)
{
if(student[i].English == student[i-1].English)
student[i].rank3 = student[i-1].rank3;
else
student[i].rank3 = i + 1;
}
sort(student, student+n, cmp4);
student[0].rank4 = 1;
for(int i = 1; i < n; ++i)
{
if(student[i].average == student[i-1].average)
student[i].rank4 = student[i-1].rank4;
else
student[i].rank4 = i + 1;
}
map<string, Student> mp;
for(int i = 0; i < n; ++i)
{
string ID = student[i].ID;
mp[ID] = student[i];
}
char str[8];
Student tmp;
for(int i = 0; i < m; ++i)
{
scanf("%s", str);
if(mp.count(str) > 0)
{
tmp = mp[str];
print(tmp);
}
else
printf("N/A\n");
}
return 0;
}

…….未完待续