4.
#include
#include
int main()
{
int a,b,c,temp;
scanf("%d %d %d",&a,&b,&c);
if(a < b){
temp = a;
a = b;
b = temp;
}
if(a < c){
temp = a;
a = c;
c = temp;
}
printf("最大值为: %d",a);
return 0;
}
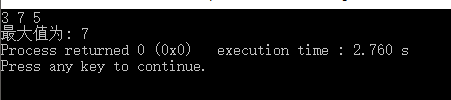
5.
#include
#include
#include
int main()
{
int num,sqrtNum;
scanf("%d",&num);
if(num >= 1000){
printf("请重新输入小于1000的数\n");
scanf("%d",&num);
}
sqrtNum = sqrt(num);
printf("%d平方根整数部分为:%d",num,sqrtNum);
return 0;
}
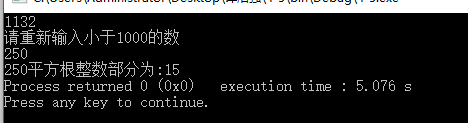
6.
#include
#include
int main()
{
int x, y;
printf("请输入x的值:\n");
scanf("%d", &x);
if(x < 1){
y = x;
}
else if(x < 10){
y = 2 * x - 1;
}
else{
y = 3 * x - 11;
}
printf("y的值为: %d", y);
return 0;
}
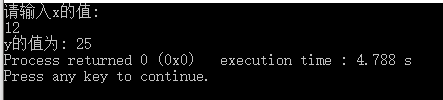
7.
#include
#include
int main()
{
float grade;
printf("请输入成绩\n");
scanf("%f",&grade);
switch((int)grade/10)
{
case 0:
case 1:
case 2:
case 3:
case 4:
case 5: printf("成绩等级为E\n");break;
case 6: printf("成绩等级为D\n");break;
case 7: printf("成绩等级为C\n");break;
case 8: printf("成绩等级为B\n");break;
case 9:
case 10:printf("成绩等级为A\n");break;
}
return 0;
}
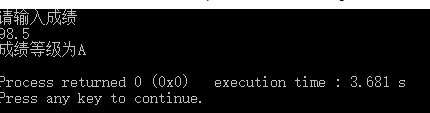
9.
#include
#include
int main()
{
int num,digit,firstDigit,secondDigit,thirdDigit,fourthDigit,fifthDigit;
printf("请输入不多于五位的正整数\n");
scanf("%d",&num);
if(num < 1||num >99999){
printf("输入出错!");
}
else
{
if(num > 9999){
digit = 5;
}
else if(num >999){
digit = 4;
}
else if(num > 99){
digit = 3;
}
else if(num > 9){
digit = 2;
}
else digit = 1;
printf("该数字是%d位数",digit);
firstDigit = num/10000;
secondDigit = (num%10000)/1000;
thirdDigit = (num%1000)/100;
fourthDigit = (num%100)/10;
fifthDigit = num%10;
switch(digit)
{
case 5:printf("每一位数字为:%d,%d,%d,%d,%d\n",firstDigit,secondDigit,thirdDigit,fourthDigit,fifthDigit);
printf("倒序输出结果为: %d%d%d%d%d",fifthDigit,fourthDigit,thirdDigit,secondDigit,firstDigit);
break;
case 4:printf("每一位数字为:%d,%d,%d,%d\n",secondDigit,thirdDigit,fourthDigit,fifthDigit);
printf("倒序输出结果为: %d%d%d%d",fifthDigit,fourthDigit,thirdDigit,secondDigit);
break;
case 3:printf("每一位数字为:%d,%d,%d\n",thirdDigit,fourthDigit,fifthDigit);
printf("倒序输出结果为: %d%d%d",fifthDigit,fourthDigit,thirdDigit);
break;
case 2:printf("每一位数字为:%d,%d\n",fourthDigit,fifthDigit);
printf("倒序输出结果为: %d%d",fifthDigit,fourthDigit);
break;
case 1:printf("每一位数字为:%d\n",fifthDigit);
printf("倒序输出结果为: %d",fifthDigit);
break;
}
}
return 0;
}
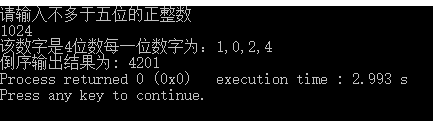
10.
(1)
#include
#include
int main()
{
int i;
double addition;
printf("请输入当月利润!\n");
scanf("%d", &i);
if(i <= 100000){
addition = i * 0.1;
}
else if(i <= 200000){
addition = 100000 * 0.1 + (i-100000)*0.075;
}
else if(i <= 400000){
addition = 100000*0.1 + 100000 * 0.075 + (i-200000)*0.05;
}
else if(i <= 600000){
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + (i-400000)*0.03;
}
else if(i <= 1000000){
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + 200000*0.03 + (i-600000)* 0.015;
}
else if(i > 1000000){
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + 200000*0.03 + 400000*0.015 + (i-1000000)*0.01;
}
printf("奖金为:%.2f\n", addition);
return 0;
}
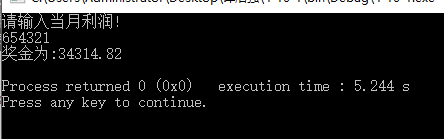
(2)
#include
#include
int main()
{
int i,total;
double addition;
printf("请输入当月利润!\n");
scanf("%d", &i);
if(i > 1000000)
total = 10;
else
total = i/100000;
switch(total)
{
case 0:
addition = i * 0.1;
break;
case 1:
addition = 100000 * 0.1 + (i-100000)*0.075;
break;
case 2:
case 3:
addition = 100000*0.1 + 100000 * 0.075 + (i-200000)*0.05;
break;
case 4:
case 5:
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + (i-400000)*0.03;
break;
case 6:
case 7:
case 8:
case 9:
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + 200000*0.03 + (i-600000)* 0.015;
break;
case 10:
addition = 100000 * 0.1 + 100000 * 0.075 + 200000*0.05 + 200000*0.03 + 400000*0.015 + (i-1000000)*0.01;
break;
}
printf("奖金为:%.2f\n", addition);
return 0;
}
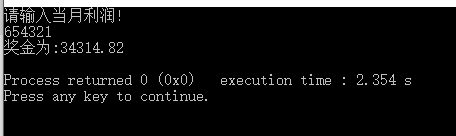
11.
#include
#include
int main()
{
int a, b, c, d,temp;
printf("请输入四个整数:\n");
scanf("%d %d %d %d",&a,&b,&c,&d);
if(a > b){
temp = a;
a = b;
b = temp;
}
if(a > c){
temp = a;
a = c;
c = temp;
}
if(a > d){
temp = a;
a = d;
d = temp;
}
if(b > c){
temp = b;
b= c;
c = temp;
}
if(b > d){
temp = b;
b= d;
d = temp;
}
if(c > d){
temp = c;
c = d;
d = temp;
}
printf("按从小到大排序结果为:\n%d,%d,%d,%d,",a,b,c,d);
return 0;
}
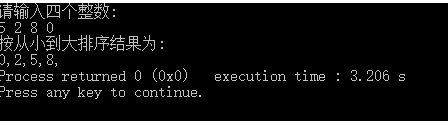
12.
#include
#include
int main()
{
double x1 = 2, y1 = 2, x2 = -2, y2 = 2, x3 = -2, y3 = -2, x4 = 2, y4 = -2,x,y;
printf("请输入x和y的坐标:\n");
scanf("%lf %lf",&x,&y);
if(((x-x1)*(x-x1) + (y-y1)*(y-y1)) < 1){
printf("该点的高度为10m");
}
else if(((x-x2)*(x-x2) + (y-y2)*(y-y2)) < 1){
printf("该点的高度为10m");
}
else if(((x-x3)*(x-x3) + (y-y3)*(y-y3)) < 1){
printf("该点的高度为10m");
}
else if(((x-x4)*(x-x4) + (y-y4)*(y-y4)) < 1){
printf("该点的高度为10m");
}
else
printf("该点的高度为0m");
return 0;
}
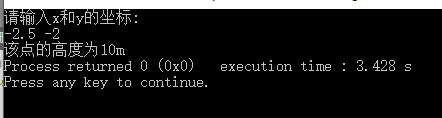