1.
#include
#include
int main()
{
int a,b,c,*p1,*p2,*p3,*p;
printf("请输入三个整数\n");
scanf("%d %d %d",&a,&b,&c);
p1=&a;
p2=&b;
p3=&c;
if(a>b)
{
p=p1;
p1=p2;
p2=p;
}
if(a>c)
{
p=p1;
p1=p3;
p3=p;
}
if(b>c)
{
p=p2;
p2=p3;
p3=p;
}
printf("从小到大输出为:\n");
printf("%d %d %d",*p1,*p2,*p3);
return 0;
}
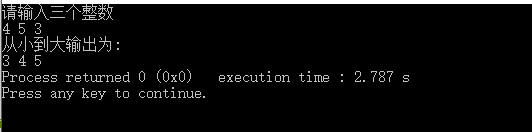
2.
#include
#include
#include
int main()
{
void swap(char *,char *);
char str1[30],str2[30],str3[30];
printf("请输入三个字符串\n");
gets(str1);
gets(str2);
gets(str3);
if(strcmp(str1,str2)>0) swap(str1,str2);
if(strcmp(str1,str3)>0) swap(str1,str3);
if(strcmp(str2,str3)>0) swap(str2,str3);
printf("排序后的三个字符串\n");
puts(str1);
puts(str2);
puts(str3);
return 0;
}
void swap(char *p1,char *p2)
{
char p[30];
strcpy(p,p1);
strcpy(p1,p2);
strcpy(p2,p);
}
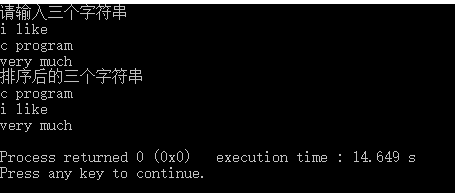
3.
#include
#include
int main()
{
void enter_digit(int *);
void swap_digit(int *);
void printf_digit(int *);
int a[20];
enter_digit(a);
swap_digit(a);
printf_digit(a);
return 0;
}
void enter_digit(int *a)
{
printf("请输入十个数\n");
int i,*p;
for(i=0,p=a;i<10;i++,p++)
scanf("%d",p);
}
void swap_digit(int *a)
{
int *min,*max,mini,maxi,i,temp,*p;
p=a;
min=max=a;
for(i=0;i<10;i++,p++)
{
if(*p<*min)
min=p;
}
temp=a[0];
a[0]=*min;
*min=temp;
p=a;
for(i=0;i<10;i++,p++)
{
if(*p>*max)
max=p;
}
temp=a[9];
a[9]=*max;
*max=temp;
}
void printf_digit(int *a)
{
int i, *p;
printf("调整后的结果为:\n");
for(i=0,p=a;i<10;i++,p++)
printf("%d ",*p);
}
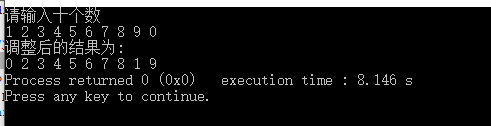
4.
#include
#include
int main()
{
void adjust_list(int *,int);
int i,n,digit[20];
printf("请输入整数的个数:\n");
scanf("%d",&n);
printf("请输入每个数字\n");
for(i=0;i<n;i++)
{
scanf("%d",&digit[i]);
}
adjust_list(digit,n);
printf("移动后的结果为:\n");
for(i=0;i<n;i++)
{
printf("%d ",digit[i]);
}
return 0;
}
void adjust_list(int *a,int n)
{
int i,j,m,last;
printf("请输入向后移动的个数:\n");
scanf("%d",&m);
for(i=0;i<m;i++)
{
last=a[n-1];
for(j=n-1;j>0;j--)
{
a[j]=a[j-1];
}
a[0]=last;
}
}
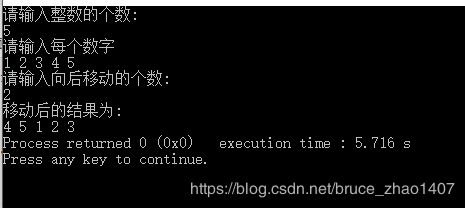
5.
#include
#include
int main()
{
int i,k,n,num[50],*p,last;
printf("请输入人数:\n");
scanf("%d",&n);
p=num;
for(i=0;i<n;i++)
{
*(p+i)=i+1;
}
last=n;
for(i=0,k=0;last>1;)
{
if(*(p+i)!=0)
k++;
if(k==3)
{
k=0;
*(p+i)=0;
last--;
}
i++;
if(i==n)
{
i=0;
}
}
p=num;
for(i=0;i<n;i++)
{
if(*(p+i)!=0)
printf("最后留下的是第%d个",i+1);
}
return 0;
}
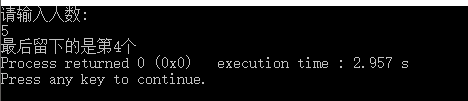
6.
#include
#include
int main()
{
int get_length(char *);
int len;
char ch[50];
printf("请输入一串字符\n");
gets(ch);
len=get_length(ch);
printf("这串字符串有%d个字符",len);
return 0;
}
int get_length(char *p)
{
int n=0;
while(*p!='\0')
{
n++;
p++;
}
return n;
}
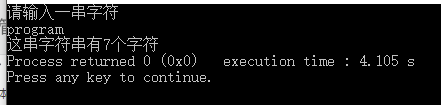
7.
#include
#include
int main()
{
void trans_another(char *, char *);
char a[50],b[50];
printf("请输入一串字符\n");
gets(a);
trans_another(a,b);
printf("复制后的字符串为");
int i=0;
while(b[i]!='\0')
{
printf("%c",b[i]);
i++;
}
return 0;
}
void trans_another(char *p, char *q)
{
int m,i,j;
printf("请输入复制起始位置\n");
scanf("%d",&m);
i=m-1;
j=0;
while(*(p+i)!='\0')
{
*(q+j)=*(p+i);
i++;
j++;
}
*(q+j)='\0';
}
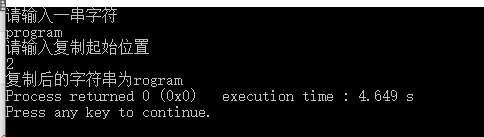
8.
#include
#include
int main()
{
void adjust_array(int *);
int a[5][5],i,j;
printf("请输入一个5*5的矩阵\n");
for(i=0;i<5;i++)
{
for(j=0;j<5;j++)
scanf("%d",&a[i][j]);
}
adjust_array(*a);
return 0;
}
void adjust_array(int *a)
{
int i,j,*max,*min[4],*p,temp;
p=a;
max=p;
for(i=0;i<4;i++)
{
min[i]=p;
}
for(i=0;i<5;i++)
{
for(j=0;j<5;j++)
{
if(*(p+5*i+j)>*max)
{
max=p+5*i+j;
}
if(*(p+5*i+j)<*min[0])
{
min[0]=p+5*i+j;
}
}
}
int k;
for(k=1;k<4;k++)
{
for(i=0;i<5;i++)
{
for(j=0;j<5;j++)
{
if(((p+5*i+j)==min[k-1])||(*(p+5*i+j)<*min[k-1]))
{
continue;
}
if(*(p+5*i+j)<*min[k])
{
min[k]=p+5*i+j;
}
}
}
}
temp=*max;
*max=*(p+12);
*(p+12)=temp;
temp=*min[0];
*min[0]=*p;
*p=temp;
temp=*min[1];
*min[1]=*(p+4);
*(p+4)=temp;
temp=*min[2];
*min[2]=*(p+20);
*(p+20)=temp;
temp=*min[3];
*min[3]=*(p+24);
*(p+24)=temp;
printf("调整后的数组为:\n");
for(i=0;i<5;i++)
{
for(j=0;j<5;j++)
{
printf("%d ",*(p+5*i+j));
}
printf("\n");
}
}

12.
#include
#include
#include
int main()
{
void sort_string(char *[]);
char ch[10][50],*p[10];
int i;
for(i=0;i<10;i++)
{
p[i]=ch[i];
}
printf("请输入十个的字符串\n");
for(i=0;i<10;i++)
{
gets(ch[i]);
}
sort_string(p);
printf("排序后的结果为:\n");
for(i=0;i<10;i++)
{
printf("%s\n",p[i]);
}
return 0;
}
void sort_string(char *a[])
{
char *temp;
int i,j;
for(i=0;i<9;i++)
{
for(j=0;j<9-i;j++)
{
if(strcmp(*(a+j),*(a+j+1))>0)
{
temp=*(a+j);
*(a+j)=*(a+j+1);
*(a+j+1)=temp;
}
}
}
}
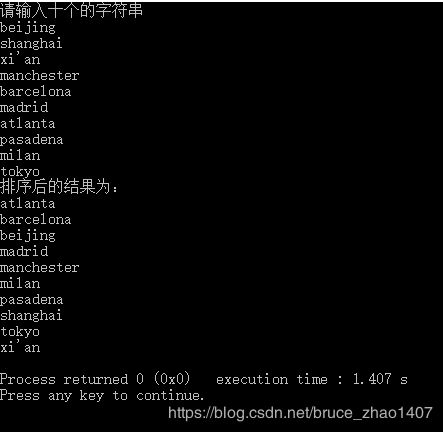
13.
#include
#include
#include
#define N 50;
int main()
{
double get_integral(double(*)(double),double,double);
double get_sin(double);
double get_cos(double);
double get_exp(double);
int a,b;
a=0;b=1;
double sinResult,cosResult,expResult,(*p)(double);
p=get_sin;
sinResult=get_integral(p,a,b);
p=get_cos;
cosResult=get_integral(p,a,b);
p=get_exp;
expResult=get_integral(p,a,b);
printf("结果分别为:\n sin: %f\n cos: %f\n exp: %f",sinResult,cosResult,expResult);
return 0;
}
double get_integral(double(*p)(double),double a,double b)
{
int i;
double x,h,s;
h=(b-a)/N;
x=a;
s=0;
for(i=1;i<=50;i++)
{
x+=h;
s+=(*p)(x)*h;
}
return s;
}
double get_sin(double x)
{
return sin(x);
}
double get_cos(double x)
{
return cos(x);
}
double get_exp(double x)
{
return exp(x);
}
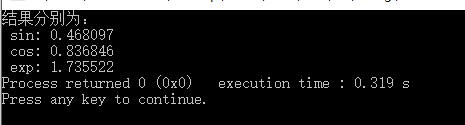
20.
#include
#include
#include
int main()
{
void sort_str(char **q);
int i;
char ch[5][50],*p[5],**q;
for(i=0;i<5;i++)
p[i]=ch[i];
printf("请输入五个字符串:\n");
for(i=0;i<5;i++)
scanf("%s",p[i]);
q=p;
sort_str(q);
printf("排序后的结果为:\n");
for(i=0;i<5;i++)
printf("%s\n",p[i]);
return 0;
}
void sort_str(char **q)
{
int i,j;
char *temp;
for(i=0;i<5;i++)
{
for(j=i+1;j<5;j++)
{
if(strcmp(*(q+i),*(q+j))>0)
{
temp=*(q+i);
*(q+i)=*(q+j);
*(q+j)=temp;
}
}
}
}
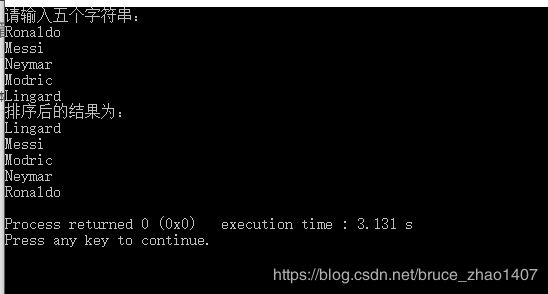