welcome to my blog
LeetCode Top Interview Questions 36. Valid Sudoku (Java版; Medium)
题目描述
Determine if a 9x9 Sudoku board is valid. Only the filled cells need to be validated according to the following rules:
Each row must contain the digits 1-9 without repetition.
Each column must contain the digits 1-9 without repetition.
Each of the 9 3x3 sub-boxes of the grid must contain the digits 1-9 without repetition.
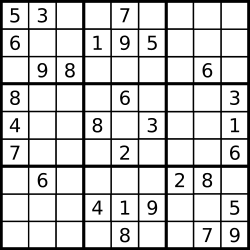
A partially filled sudoku which is valid.
The Sudoku board could be partially filled, where empty cells are filled with the character '.'.
Example 1:
Input:
[
["5","3",".",".","7",".",".",".","."],
["6",".",".","1","9","5",".",".","."],
[".","9","8",".",".",".",".","6","."],
["8",".",".",".","6",".",".",".","3"],
["4",".",".","8",".","3",".",".","1"],
["7",".",".",".","2",".",".",".","6"],
[".","6",".",".",".",".","2","8","."],
[".",".",".","4","1","9",".",".","5"],
[".",".",".",".","8",".",".","7","9"]
]
Output: true
Example 2:
Input:
[
["8","3",".",".","7",".",".",".","."],
["6",".",".","1","9","5",".",".","."],
[".","9","8",".",".",".",".","6","."],
["8",".",".",".","6",".",".",".","3"],
["4",".",".","8",".","3",".",".","1"],
["7",".",".",".","2",".",".",".","6"],
[".","6",".",".",".",".","2","8","."],
[".",".",".","4","1","9",".",".","5"],
[".",".",".",".","8",".",".","7","9"]
]
Output: false
Explanation: Same as Example 1, except with the 5 in the top left corner being
modified to 8. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
Note:
A Sudoku board (partially filled) could be valid but is not necessarily solvable.
Only the filled cells need to be validated according to the mentioned rules.
The given board contain only digits 1-9 and the character '.'.
The given board size is always 9x9.
第一次做;采用位运算,优化了下面的做法; 位运算别弄混…
class Solution {
public boolean isValidSudoku(char[][] board) {
int[] row = new int[9];
int[] col = new int[9];
int[] sub = new int[9];
for(int i=0; i<9; i++){
for(int j=0; j<9; j++){
if(board[i][j]=='.')
continue;
if(!valid(row, i, board[i][j]-48))
return false;
if(!valid(col, j, board[i][j]-48))
return false;
int index = i/3*3 + j/3;
if(!valid(sub, index, board[i][j]-48))
return false;
}
}
return true;
}
public boolean valid(int[] arr, int i, int cur){
if(((arr[i]>>cur)&1)==1)
return false;
arr[i] = arr[i] | (1<<cur);
return true;
}
}
第一次做; 核心:如何遍历子数独, i/3*3 + j/3, 非常巧妙! 注意如声明哈希表数组, 声明数组大小时不能加泛型, 以及如何初始化, 初始化时可以加泛型; 其实可以不用哈希表, 直接用位运算就行, 因为数字范围仅仅是[0,9]; 看注释
import java.util.HashSet;
class Solution {
public boolean isValidSudoku(char[][] board) {
if(board==null||board.length!=9||board[0]==null ||board[0].length!=9)
return false;
HashSet<Character>[] row = new HashSet[9];
HashSet<Character>[] col = new HashSet[9];
HashSet<Character>[] sub = new HashSet[9];
for(int i=0; i<9; i++){
row[i] = new HashSet();
col[i] = new HashSet();
sub[i] = new HashSet();
}
for(int i=0; i<9; i++){
for(int j=0; j<9; j++){
char ch = board[i][j];
if(ch=='.')
continue;
if(row[i].contains(ch)){
return false;
}
else{
row[i].add(ch);
}
if(col[j].contains(ch)){
return false;
}
else{
col[j].add(ch);
}
int index = i/3*3 + j/3;
if(sub[index].contains(ch)){
return false;
}
else{
sub[index].add(ch);
}
}
}
return true;
}
}
坐标推导, 来自力扣题解
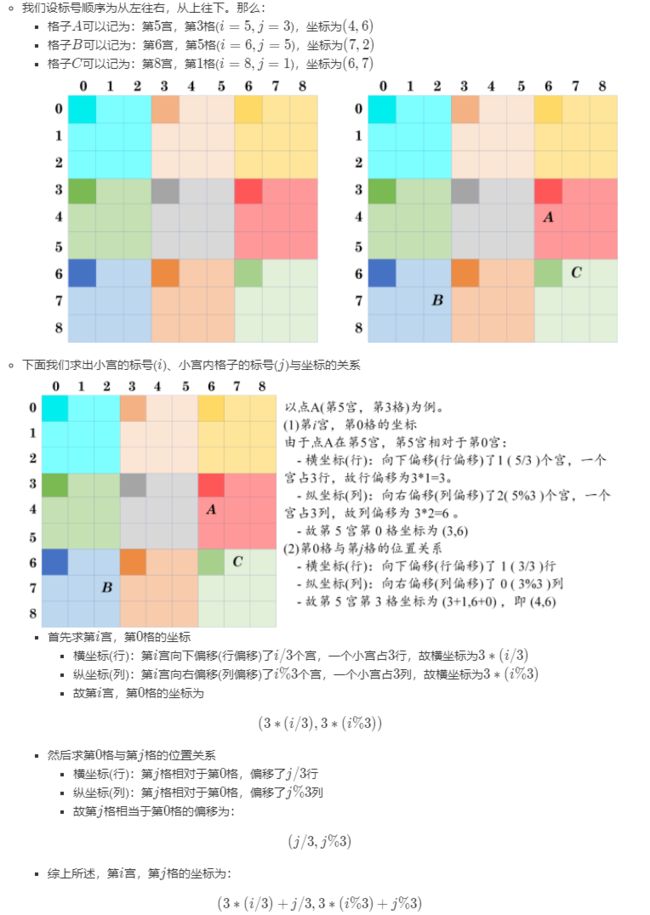
力扣官方题解; 如何枚举子数独, 非常巧妙!
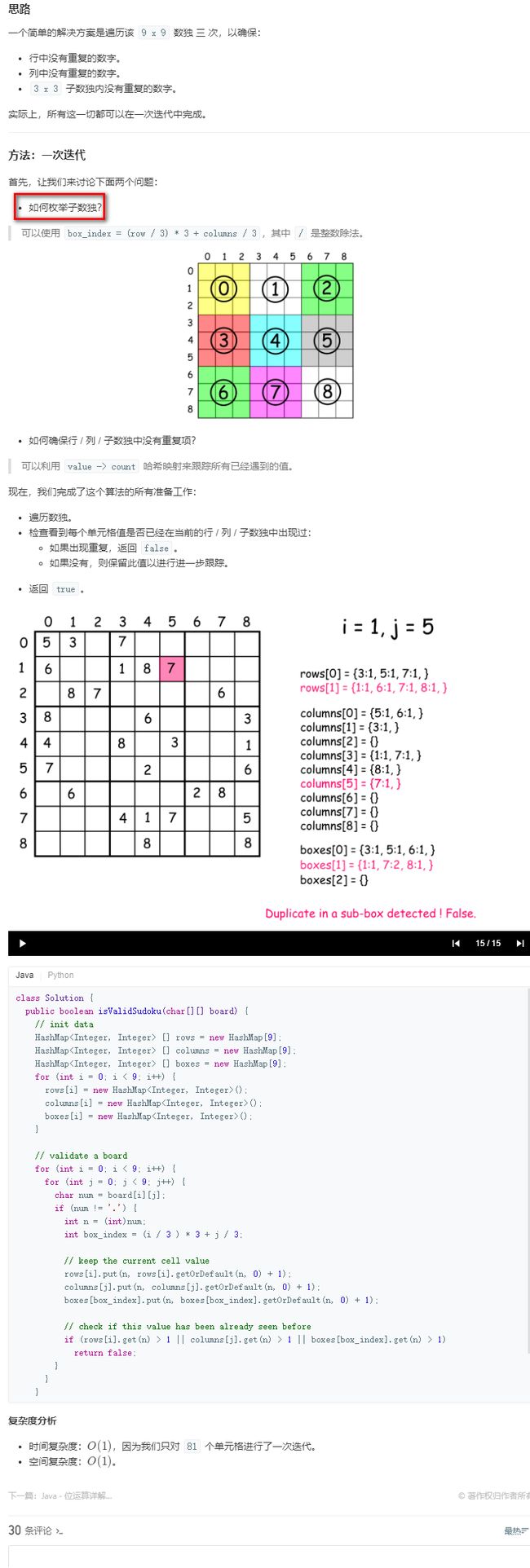
力扣题解; 不需要创建数组, 解决第i行的同时也会解决第i列, 会有重复计算, 相当于不创建数组的代价(时间换空间); 本题创建数组也无妨, 因为大小是固定的
public boolean isValidSudoku(char[][] board) {
for(int i = 0; i < 9; i ++){
int hori = 0, veti = 0, sqre = 0;
for(int j = 0; j < 9; j ++){
int h = board[i][j] - 48;
int v = board[j][i] - 48;
int s = board[3 * (i / 3) + j / 3][3 * (i % 3) + j % 3] - 48;
if(h > 0){
hori = sodokuer(h, hori);
}
if(v > 0){
veti = sodokuer(v, veti);
}
if(s > 0){
sqre = sodokuer(s, sqre);
}
if(hori == -1 || veti == -1 || sqre == -1){
return false;
}
}
}
return true;
}
private int sodokuer(int n, int val){
return ((val >> n) & 1) == 1 ? -1 : val ^ (1 << n);
}