github地址: 刷新控件地址
implementation 'com.scwang.smartrefresh:SmartRefreshLayout:1.1.0' //1.0.5及以前版本的老用户升级需谨慎,API改动过大
implementation 'com.scwang.smartrefresh:SmartRefreshHeader:1.1.0' //没有使用特殊Header,可以不加这行
注意区别于AndroidX:
如果使用 AndroidX 在 gradle.properties 中添加
android.useAndroidX=true
android.enableJetifier=true
<com.scwang.smartrefresh.layout.SmartRefreshLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/refreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:overScrollMode="never"
android:background="#fff" />
</com.scwang.smartrefresh.layout.SmartRefreshLayout>
初始化控件:
RefreshLayout refreshLayout = (RefreshLayout)findViewById(R.id.refreshLayout);
refreshLayout.setOnRefreshListener(new OnRefreshListener() {
@Override
public void onRefresh(RefreshLayout refreshlayout) {
// refreshLayout.finishRefresh(); 简单的设置刷新完成
refreshlayout.finishRefresh(2000/*,false*/);//传入false表示刷新失败
//重新刷新的逻辑代码
}
});
refreshLayout.setOnLoadMoreListener(new OnLoadMoreListener() {
@Override
public void onLoadMore(RefreshLayout refreshlayout) {
// refreshLayout.finishLoadMore(); // 简单的设置加载更多完成
refreshlayout.finishLoadMore(2000/*,false*/);//传入false表示加载失败
// 加载更多的逻辑
}
});
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-AYrLGohu-1570788044728)(https://ws1.sinaimg.cn/large/e25f3775ly1g7u8cq6ehij20u01hcadj.jpg)]
简单的设置(好几种设置方法,我们使用xml设置的)
在原先的xml布局中,添加head和foot就OK了.
<com.scwang.smartrefresh.layout.SmartRefreshLayout
android:id="@+id/refreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/text_time">
<com.scwang.smartrefresh.layout.header.ClassicsHeader
android:layout_width="match_parent"
android:layout_height="wrap_content">
</com.scwang.smartrefresh.layout.header.ClassicsHeader>
<android.support.v7.widget.RecyclerView
android:id="@+id/bdtv_fragment_rv"
android:layout_width="match_parent"
android:layout_height="match_parent">
</android.support.v7.widget.RecyclerView>
<com.scwang.smartrefresh.layout.footer.ClassicsFooter
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
</com.scwang.smartrefresh.layout.footer.ClassicsFooter>
</com.scwang.smartrefresh.layout.SmartRefreshLayout>
实现 Header 和 Footer 时,继承 InternalAbstract 的话可以少写很多接口方法
堃堃5love:
刷新head R.layout.layout_ptr_header
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<ProgressBar
android:id="@+id/progress_bar"
style="@style/Widget.AppCompat.ProgressBar"
android:layout_width="12dp"
android:layout_height="12dp"
android:visibility="gone"
android:layout_gravity="center_horizontal" />
<ImageView
android:id="@+id/refresh_head"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/refresh_anim"
android:contentDescription="@null"/>
<TextView
android:id="@+id/tv_ptr_header"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:gravity="center_vertical"
android:textColor="#333"
android:textSize="12dp" />
</LinearLayout>
堃堃5love:
加载更多: R.layout.layout_load_more
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:gravity="center"
android:paddingTop="10dp"
android:paddingBottom="10dp"
android:layout_height="wrap_content">
<ProgressBar
android:id="@+id/progress"
style="@style/MyProgressBar"
android:layout_width="16dp"
android:layout_height="16dp"/>
<TextView
android:id="@+id/tv_loadmore"
android:text="加载更多"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:textColor="#333"
android:textSize="13dp" />
</LinearLayout>
创建自定义Head,后续会将这个类上传,现在有一个问题是下拉的距离太短
设置下拉刷新的高度:
// 设置下拉刷新的Head高度
refreshLayout.setHeaderHeight(200);
自己的下拉刷新:
/**
* PACKAGE_NAME: cn.com.enorth.easymakeapp.view.customsmartview
* PROJECT_NAME:learnbaodi
* 创建日期 :2019/10/11
* 创建时间: 15/49
* author kunkun5love
* 说明: smartRefrush中自定义的head 需要实现RefreshHeader
***/
public class CustomSmartRefrushHead extends LinearLayout implements RefreshHeader {
private View mRefrushSmartHeadView;
private ProgressBar mRefrush_smart_head_pg;
private ImageView mRefrush_smart_head_iv;
private TextView mRefrush_smart_head_tv;
private AnimationDrawable mAnimationDrawable;
/**
* 我们这边写好了 两个布局堃堃5love:
* 刷新head R.layout.layout_ptr_header
*
* 堃堃5love:
* 加载更多: R.layout.layout_load_more
*
* @param context
*/
public CustomSmartRefrushHead(Context context) {
super(context);
initRefrushView(context);
}
public CustomSmartRefrushHead(Context context, AttributeSet attrs) {
super(context, attrs);
initRefrushView(context);
}
public CustomSmartRefrushHead(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initRefrushView(context);
}
/**
* 打气一个view,并进行初始化
*
* @param context
*/
private void initRefrushView(Context context) {
// 设置居中
setGravity(Gravity.CENTER);
mRefrushSmartHeadView = LayoutInflater.from(context).inflate(R.layout.layout_ptr_header, null);
addView(mRefrushSmartHeadView);
mRefrush_smart_head_pg = mRefrushSmartHeadView.findViewById(R.id.progress_bar);
mRefrush_smart_head_iv = mRefrushSmartHeadView.findViewById(R.id.refresh_head);
mRefrush_smart_head_tv = mRefrushSmartHeadView.findViewById(R.id.tv_ptr_header);
//先设置图片的开始动画 设置的时候src直接设置的 animation
mRefrush_smart_head_iv.getDrawable();
mAnimationDrawable = (AnimationDrawable) mRefrush_smart_head_iv.getDrawable();
}
// 下面的都是RefreshHeader继承的方法 start
/**
* 获取真实视图(必须返回,不能为null) 当前的head就是一个view
*
* @return
*/
@NonNull
@Override
public View getView() {
return this;
}
/**
* 获取变换方式(必须指定一个:平移、拉伸、固定、全屏) 我们类似于经典刷新 选择平移
*
* @return
*/
@NonNull
@Override
public SpinnerStyle getSpinnerStyle() {
return SpinnerStyle.Translate;//指定为平移,不能null;
}
/**
* 设置主题颜色 (如果自定义的Header没有注意颜色,本方法可以什么都不处理)
*
* @param colors 对应Xml中配置的 srlPrimaryColor srlAccentColor
*/
@Override
public void setPrimaryColors(int... colors) {
}
/**
* 尺寸定义初始化完成 (如果高度不改变(代码修改:setHeader),只调用一次, 在RefreshLayout#onMeasure中调用)
*
* @param kernel RefreshKernel 核心接口(用于完成高级Header功能)
* @param height HeaderHeight or FooterHeight
* @param maxDragHeight 最大拖动高度
*/
@Override
public void onInitialized(@NonNull RefreshKernel kernel, int height, int maxDragHeight) {
}
/**
* 手指拖动下拉(会连续多次调用,用于实时控制动画关键帧)
*
* @param percent 下拉的百分比 值 = offset/headerHeight (0 - percent - (headerHeight+maxDragHeight) / headerHeight )
* @param offset 下拉的像素偏移量 0 - offset - (headerHeight+maxDragHeight)
* @param height Header的高度
* @param maxDragHeight 最大拖动高度
*/
@Override
public void onMoving(boolean isDragging, float percent, int offset, int height, int maxDragHeight) {
}
@Override
public void onReleased(@NonNull RefreshLayout refreshLayout, int height, int maxDragHeight) {
}
/**
* 开始动画(开始刷新或者开始加载动画)
*
* @param refreshLayout RefreshLayout
* @param height HeaderHeight or FooterHeight
* @param maxDragHeight 最大拖动高度
*/
@Override
public void onStartAnimator(@NonNull RefreshLayout refreshLayout, int height, int maxDragHeight) {
mAnimationDrawable.start();
// 设置下拉刷新
mRefrush_smart_head_tv.setText("下拉刷新");
}
/**
* 动画结束
*
* @param refreshLayout RefreshLayout
* @param success 数据是否成功刷新或加载
* @return 完成动画所需时间 如果返回 Integer.MAX_VALUE 将取消本次完成事件,继续保持原有状态
*/
@Override
public int onFinish(@NonNull RefreshLayout refreshLayout, boolean success) {
// 图片动画定义
mAnimationDrawable.stop();
if (success) {
mRefrush_smart_head_tv.setText("刷新成功");
} else {
mRefrush_smart_head_tv.setText("刷新失败");
}
return 500;
}
@Override
public void onHorizontalDrag(float percentX, int offsetX, int offsetMax) {
}
@Override
public boolean isSupportHorizontalDrag() {
return false;
}
/**
* 不同的状态控制内部控件的显示和旋转
*
* @param refreshLayout
* @param oldState
* @param newState
*/
@Override
public void onStateChanged(@NonNull RefreshLayout refreshLayout, @NonNull RefreshState oldState,
@NonNull RefreshState newState) {
switch (newState){
case None:
case ReleaseToRefresh: //下拉将要释放开始刷新
mRefrush_smart_head_tv.setText("释放刷新");
break;
case PullDownToRefresh: // 开始下拉
mRefrush_smart_head_tv.setText("下拉开始刷新");
break;
case Refreshing: // 正在刷新
mRefrush_smart_head_tv.setText("正在刷新");
break;
}
}
// 下面的都是RefreshHeader继承的方法 end
}
展示效果图:
art_head_tv.setText(“释放刷新”);
break;
case PullDownToRefresh: // 开始下拉
mRefrush_smart_head_tv.setText(“下拉开始刷新”);
break;
case Refreshing: // 正在刷新
mRefrush_smart_head_tv.setText(“正在刷新”);
break;
}
}
// 下面的都是RefreshHeader继承的方法 end
}
展示效果图:
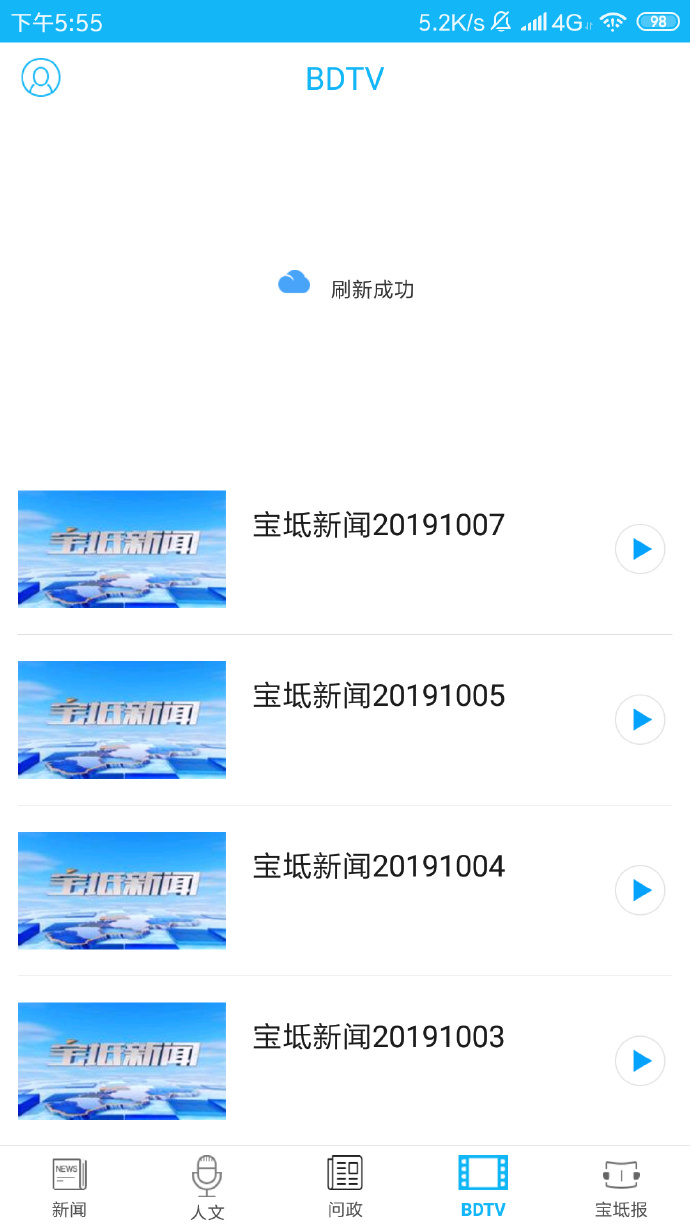