转自:https://blog.csdn.net/tj807126663/article/details/33738563
简介
Qt图形应用中经常会遇到从一个界面跳转到另一个界面应用,这里简单介绍一种使用信号-槽机制实现的界面跳转方案。创建one,two, three三个图像界面类,每一个界面类中实现一个信号函数,在按钮的槽函数中发送该信号, 使用QStackedLayout存放所有的界面,在主窗口使用connect将信号和QStackedLayout::setCurrentIndex关联起来。
界面one
界面one图形如下:
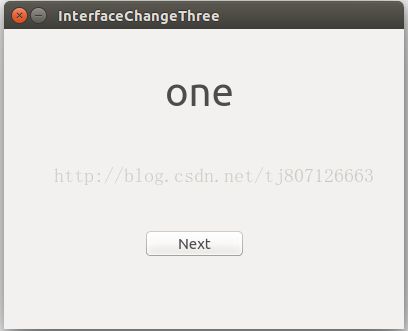
one.h
- #ifndef ONE_H
- #define ONE_H
-
- #include
-
- class Two;
-
- namespace Ui {
- class One;
- }
-
- class One : public QWidget
- {
- Q_OBJECT
-
- public:
- explicit One(QWidget *parent = 0);
- ~One();
-
- signals:
- void display(int number);
-
- private slots:
- void on_nextPushButton_clicked();
-
- private:
- Ui::One *ui;
- Two *two;
- };
-
- #endif // ONE_H
one.cpp
- #include "one.h"
- #include "ui_one.h"
- #include "two.h"
- #include "widget.h"
-
- One::One(QWidget *parent) :
- QWidget(parent),
- ui(new Ui::One)
- {
- ui->setupUi(this);
-
- }
-
- One::~One()
- {
- delete ui;
- }
-
- void One::on_nextPushButton_clicked()
- {
- emit display(1);
- }
界面two
界面two图形如下:
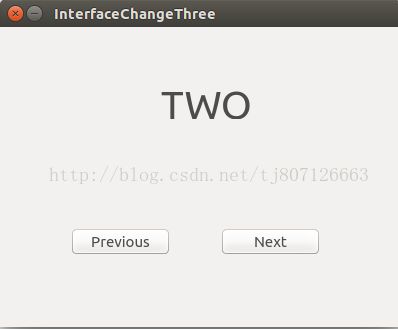
two.h
- #ifndef TWO_H
- #define TWO_H
-
- #include
-
- namespace Ui {
- class Two;
- }
-
- class Two : public QWidget
- {
- Q_OBJECT
-
- public:
- explicit Two(QWidget *parent = 0);
- ~Two();
-
- signals:
- void display(int number);
-
-
- private slots:
- void on_previousPushButton_clicked();
-
- void on_nextPushButton_clicked();
-
- private:
- Ui::Two *ui;
- };
-
- #endif // TWO_H
two.cpp
- #include "two.h"
- #include "ui_two.h"
-
- Two::Two(QWidget *parent) :
- QWidget(parent),
- ui(new Ui::Two)
- {
- ui->setupUi(this);
- }
-
- Two::~Two()
- {
- delete ui;
- }
-
- void Two::on_previousPushButton_clicked()
- {
- emit display(0);
- }
-
- void Two::on_nextPushButton_clicked()
- {
- emit display(2);
- }
界面three
界面three图形如下:
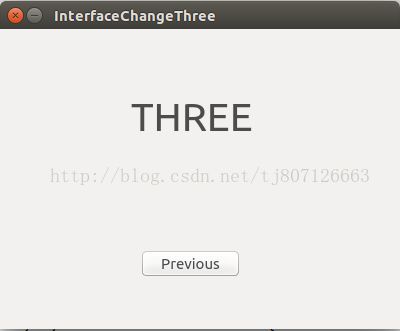
three.h
- #ifndef THREE_H
- #define THREE_H
-
- #include
-
- namespace Ui {
- class Three;
- }
-
- class Three : public QWidget
- {
- Q_OBJECT
-
- public:
- explicit Three(QWidget *parent = 0);
- ~Three();
-
- signals:
- void display(int number);
-
- private slots:
- void on_previousPushButton_clicked();
-
- private:
- Ui::Three *ui;
- };
-
- #endif // THREE_H
three.cpp
- #include "three.h"
- #include "ui_three.h"
-
- Three::Three(QWidget *parent) :
- QWidget(parent),
- ui(new Ui::Three)
- {
- ui->setupUi(this);
- }
-
- Three::~Three()
- {
- delete ui;
- }
-
- void Three::on_previousPushButton_clicked()
- {
- emit display(1);
- }
主界面
widget.h
- #ifndef WIDGET_H
- #define WIDGET_H
-
- #include
-
- class One;
- class Two;
- class Three;
- class QStackedLayout;
- class QVBoxLayout;
-
- class Widget : public QWidget
- {
- Q_OBJECT
-
- public:
- explicit Widget(QWidget *parent = 0);
- ~Widget();
-
- private:
- One *one;
- Two *two;
- Three *three;
- QStackedLayout *stackLayout;
- QVBoxLayout *mainLayout;
- };
-
- #endif // WIDGET_H
widget.cpp
- #include "widget.h"
- #include "ui_widget.h"
- #include "one.h"
- #include "two.h"
- #include "three.h"
- #include
- #include
- #include
-
- Widget::Widget(QWidget *parent) :
- QWidget(parent)
- {
- setFixedSize(400, 300);
- one = new One;
- two = new Two;
- three = new Three;
- stackLayout = new QStackedLayout;
- stackLayout->addWidget(one);
- stackLayout->addWidget(two);
- stackLayout->addWidget(three);
- connect(one, &One::display, stackLayout, &QStackedLayout::setCurrentIndex);
- connect(two, &Two::display, stackLayout, &QStackedLayout::setCurrentIndex);
- connect(three, &Three::display, stackLayout, &QStackedLayout::setCurrentIndex);
-
- mainLayout = new QVBoxLayout;
- mainLayout->addLayout(stackLayout);
- setLayout(mainLayout);
-
- }
-
- Widget::~Widget()
- {
- }
main.cpp
- #include "widget.h"
- #include
-
- int main(int argc, char *argv[])
- {
- QApplication a(argc, argv);
- Widget w;
- w.show();
-
- return a.exec();
- }
- 注:源代码下载地址http://download.csdn.net/detail/tj807126663/7540203