①. 案例演示:
<dependencies>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
<dependency>
<groupId>com.itextpdfgroupId>
<artifactId>itext-asianartifactId>
<version>5.1.1version>
dependency>
<dependency>
<groupId>com.itextpdfgroupId>
<artifactId>itextpdfartifactId>
<version>5.1.3version>
dependency>
<dependency>
<groupId>com.itextpdf.toolgroupId>
<artifactId>xmlworkerartifactId>
<version>5.5.13version>
dependency>
dependencies>
package com.xiaozhi.controller.water;
import java.awt.FontMetrics;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URL;
import java.util.UUID;
import javax.swing.JLabel;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.BaseFont;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfGState;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
public class TestWaterPrint {
public static void main(String[] args) throws DocumentException, IOException {
File desc = new File("src/main/resources/test88.pdf");
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(desc));
File directory = new File("src/main/resources");
String courseFile = directory.getCanonicalPath()+"\\"+"fbsl.pdf";
System.out.println(courseFile);
setWatermark(bos, courseFile, "null-admin");
}
public static void setWatermark(BufferedOutputStream bos, String input, String waterMarkName)
throws DocumentException, IOException {
PdfReader reader = new PdfReader(input);
PdfStamper stamper = new PdfStamper(reader, bos);
int total = reader.getNumberOfPages() + 1;
BaseFont base = null;
try {
base =BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.EMBEDDED);
} catch (Exception e) {
e.printStackTrace();
}
int interval = 15;
int textH = 0, textW = 0;
JLabel label = new JLabel();
label.setText(waterMarkName);
FontMetrics metrics = label.getFontMetrics(label.getFont());
textH = metrics.getHeight();
textW = metrics.stringWidth(label.getText());
System.out.println("textH: " + textH);
System.out.println("textW: " + textW);
PdfGState gs = new PdfGState();
gs.setFillOpacity(0.8f);
gs.setStrokeOpacity(0.8f);
Rectangle pageSizeWithRotation = null;
PdfContentByte content = null;
for (int i = 1; i < total; i++) {
content = stamper.getOverContent(i);
content.saveState();
content.setGState(gs);
content.beginText();
content.setFontAndSize(base, 20);
pageSizeWithRotation = reader.getPageSizeWithRotation(i);
float pageHeight = pageSizeWithRotation.getHeight();
float pageWidth = pageSizeWithRotation.getWidth();
for (int height = interval + textH; height < pageHeight-30; height = height + textH * 4) {
if(height>0){
height=height+80;
}
for (int width = interval + textW; width < pageWidth + textW; width = width + textW * 3) {
content.showTextAligned(Element.ALIGN_LEFT, waterMarkName, width - textW, height - textH, 15);
}
}
content.endText();
}
stamper.close();
reader.close();
}
}
- ③. 生成文件
(这里实现的效果是先通过resource下加载到fbsl.pdf文件,通过代码打上水印,生成一个test99.pdf的文件)
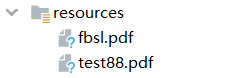
②. 项目实战
compile 'com.itextpdf:itextpdf:5.5.13'
- ②. 这里实现的效果是,拿取到resourc/pdf下的pdf,加上水印,最后直接通过io进行页面的输出
package com.devplatform.biz.web.controller.biz.operations;
import com.devplatform.biz.service.log.LogService;
import com.devplatform.common.controller.BaseController;
import com.devplatform.org.vo.IPStaffVO;
import com.itextpdf.text.BaseColor;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Element;
import com.itextpdf.text.Rectangle;
import com.itextpdf.text.pdf.*;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.swing.*;
import java.awt.*;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
@Api(tags = "运营标准相关", value = "运营标准相关")
@Slf4j
@RestController
@RequestMapping(value = "/watermarkPdf")
public class TBizOperationPdfController extends BaseController {
@Autowired
LogService logService;
@ApiOperation(value = "运营标准加水印打开pdf", notes = "pdfParams是pdf的名称")
@GetMapping("/openPdf.do")
public void openPdf(HttpServletResponse response, HttpServletRequest request) {
IPStaffVO ipStaffVO = this.getCurrentStaff(request);
String waterMarkName = "";
String code = ipStaffVO.getOwnerunitid();
String staffName = ipStaffVO.getStaffname();
String unitUnionName = ipStaffVO.getUnitunionname();
if ("1".equals(ipStaffVO.getStatus())) {
if("admin".equals(ipStaffVO.getStaffname())){
waterMarkName = "null-" + staffName;
}else{
waterMarkName = code + "-" + staffName;
}
} else {
waterMarkName = unitUnionName + "-" + staffName;
}
String pdfName = "/pdf/" + request.getParameter("pdfParams");
InputStream in = null;
OutputStream out = null;
try {
response.setContentType("application/pdf");
in = this.getClass().getResourceAsStream(pdfName);
out = response.getOutputStream();
setWatermark(out, in, waterMarkName,request,ipStaffVO);
byte[] b = new byte[512];
while ((in.read(b)) != -1) {
out.write(b);
}
} catch (IOException e) {
log.error("", e);
} finally {
try {
out.flush();
} catch (IOException e) {
log.error("", e);
}
try {
in.close();
} catch (IOException e) {
log.error("", e);
}
try {
out.close();
} catch (IOException e) {
log.error("", e);
}
}
}
private void setWatermark(OutputStream bos, InputStream input, String waterMarkName,HttpServletRequest request,IPStaffVO ipStaffVO) {
PdfReader reader=null;
PdfStamper stamper=null;
try{
reader = new PdfReader(input);
stamper = new PdfStamper(reader, bos);
int total = reader.getNumberOfPages() + 1;
BaseFont base = null;
String name = Thread.currentThread().getContextClassLoader().getResource("fonts/SIMSUN.TTC").getPath() + ",0";
base = BaseFont.createFont(name, BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
int interval = 15;
int textH = 0, textW = 0;
JLabel label = new JLabel();
label.setText(waterMarkName);
FontMetrics metrics = label.getFontMetrics(label.getFont());
textH = metrics.getHeight();
textW = metrics.stringWidth(label.getText());
log.info("textH: " + textH);
log.info("textW: " + textW);
PdfGState gs = new PdfGState();
gs.setFillOpacity(0.8f);
gs.setStrokeOpacity(0.8f);
Rectangle pageSizeWithRotation = null;
PdfContentByte content = null;
for (int i = 1; i < total; i++) {
content = stamper.getOverContent(i);
content.saveState();
content.setGState(gs);
content.beginText();
BaseColor baseColor=new BaseColor(136,136,136);
content.setColorFill(baseColor);
content.setFontAndSize(base, 10);
pageSizeWithRotation = reader.getPageSizeWithRotation(i);
float pageHeight = pageSizeWithRotation.getHeight();
float pageWidth = pageSizeWithRotation.getWidth();
for (int height = interval + textH; height < pageHeight - 30; height = height + textH * 4) {
if (height > 0) {
height = height + 80;
}
for (int width = interval + textW; width < pageWidth + textW; width = width + textW * 1) {
if(width>0){
width=width+80;
}
content.showTextAligned(Element.ALIGN_LEFT, waterMarkName, width - textW, height - textH, 15);
}
}
content.endText();
}
logService.log("打开了有水印的pdf", request.getHeader(HTTP_X_FORWARDED_FOR), ipStaffVO.getStaffid(),
ipStaffVO.getStaffname(), "标准运营", "02", "02");
} catch (DocumentException e) {
log.error("",e);
} catch (IOException e) {
log.error("",e);
} catch (Exception e) {
log.error("",e);
} finally {
try {
stamper.close();
} catch (DocumentException e) {
log.error("",e);
} catch (IOException e) {
log.error("",e);
}
reader.close();
}
}
}
<template>
<el-container>
<el-header class="query_params query_params_header">
el-header>
<el-main>
<table class="hovertable">
<tr>
<td @click="showlnp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="lnpImg" alt="理念篇" />div>
<h3>1-理念篇h3>
div>
td>
<td @click="showzzp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="zzpImg" alt="组织篇" />div>
<h3>2-组织篇h3>
div>
td>
<td @click="showhjp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="hjpImg" alt="环境篇" />div>
<h3>3-环境篇h3>
div>
td>
tr>
<tr>
<td @click="showsjzcp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="sjzcpImg" alt="数据支持篇" />div>
<h3>4-数据支持篇h3>
div>
td>
<td @click="showgljdp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="gljdpImg" alt="管理监督篇" />div>
<h3>5-管理监督篇h3>
div>
td>
<td @click="showkhwxp()">
<div class="bk" onmouseover="this.style.backgroundColor='#eef3f7';" onmouseout="this.style.backgroundColor='#fff';">
<div><img :src="khwxpImg" alt="客户维系篇" />div>
<h3>6-客户维系篇h3>
div>
td>
tr>
table>
el-main>
<el-footer>
el-footer>
el-container>
template>
<script>
import lnp from "@/views/business/operations/operationquery/img/lnp.jpg"
import zzp from "@/views/business/operations/operationquery/img/zzp.jpg"
import hjp from "@/views/business/operations/operationquery/img/hjp.jpg"
import sjzcp from "@/views/business/operations/operationquery/img/sjzcp.jpg"
import gljdp from "@/views/business/operations/operationquery/img/gljdp.jpg"
import khwxp from "@/views/business/operations/operationquery/img/khwxp.jpg"
import { getToken } from '@/utils/auth'
export default {
name: 'standardsManualQuery',
components: {},
data(){
return{
lnpImg:lnp,
zzpImg:zzp,
hjpImg:hjp,
sjzcpImg:sjzcp,
gljdpImg:gljdp,
khwxpImg:khwxp,
url:''
}
},
methods:{
showlnp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=lnp.pdf");
},
showzzp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=zzp.pdf");
},
showhjp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=hjp.pdf");
},
showsjzcp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=sjzcp.pdf");
},
showgljdp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=gljdp.pdf");
},
showkhwxp(){
window.open("/shoa/api/biz/standardsManual/openPdf.do?usertoken="+getToken()+"&&pdfParams=khwxp.pdf");
}
}
}
script>
<style scoped>
.query_params_header{
height:auto !important;
}
table.hovertable {
font-family: verdana,arial,sans-serif;
font-size:15px;
color:#333333;
border-width: 1px;
border-color: #999999;
border-collapse: collapse;
}
img{
width: 150px;
height: 190px;
cursor:pointer;
}
h3{
color: #3A0088;
}
.bk {
margin: 30px;
width: 154px;
height: 268px;
text-align:center;
border:3px solid #cceff5;
}
style>