(本文讲解了在Android中实现列表下拉刷新的动态效果的过程,文末附有源码。)
看完本文,您可以学到:
1.下拉刷新的实现原理
2.自定义Android控件,重写其ListView
3.ScrollListener滚动监听
4.Adapter适配器的使用
话不多说,先来看看效果图:
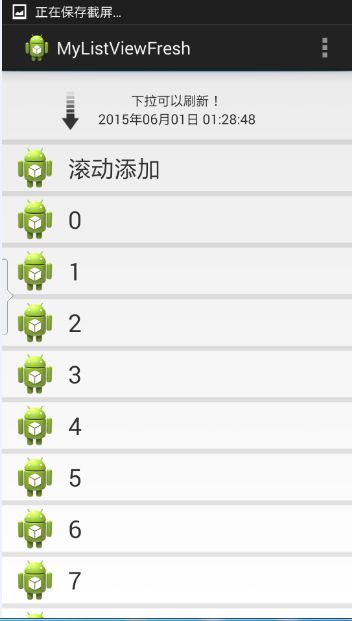

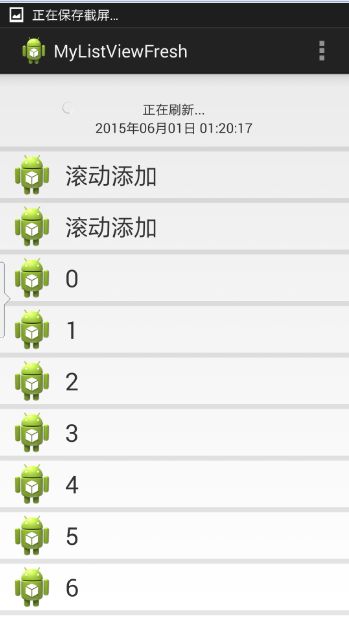
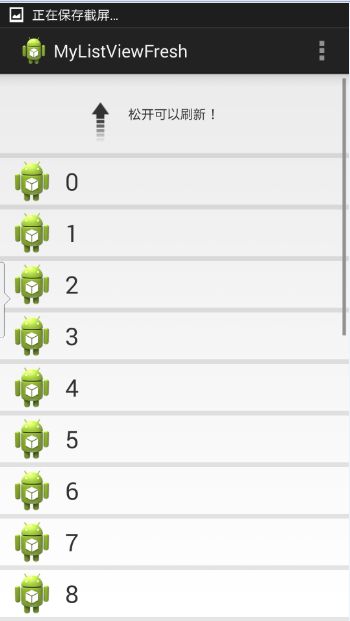
接下来我们一步一步地实现以上的效果。
一、图文并茂的ListViewItem
看一下这一步的效果图:
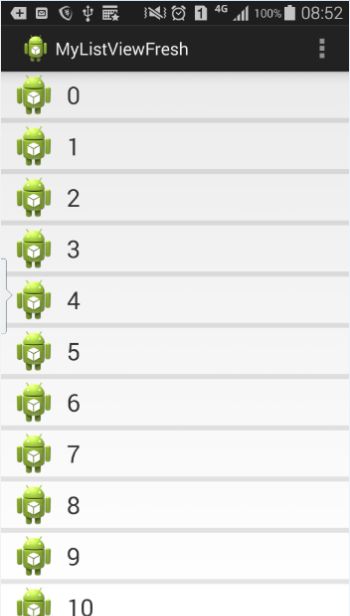
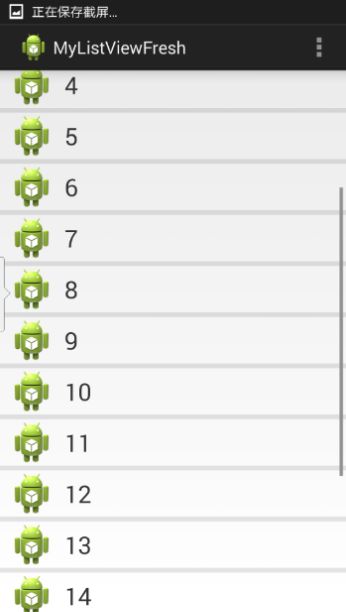
首先,我们要实现的是带下拉刷新效果的ListView。所以我们选择自己重写原生控件ListView。只需要写一个类继承它就可以了,先不添加任何的具体实现。
RefreshListView.java:
- public class RefreshListView extends ListView {
- public RefreshListView(Context context) {
- super(context);
-
- }
-
- public RefreshListView(Context context, AttributeSet attrs) {
- super(context, attrs);
-
- }
-
- public RefreshListView(Context context, AttributeSet attrs, int defStyle) {
- super(context, attrs, defStyle);
-
- }
- }
要实现图文并茂的ListViewItem,接下去就要自己定义它Item的布局,这个可以无限发挥,我这里就只取图和文做一个简单的实现:
listview_item.xml:
- xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:gravity="center_vertical"
- android:orientation="horizontal" >
-
- <ImageView
- android:id="@+id/image"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_marginLeft="10dp" />
-
- <TextView
- android:id="@+id/text"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_marginLeft="10dp"
- android:textSize="25sp" />
-
- LinearLayout>
在这个布局中,我就只放了一个Image和一个Text。您可以自己定义地更复杂。
然后需要我们注意的是,既然我们自己定义了ListView,那我们主界面的布局也要响应地修改了:
activity_main.xml:
- <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- tools:context=".MainActivity" >
-
- <com.example.mylistviewrefresh.RefreshListView
- android:id="@+id/listview"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:cacheColorHint="#00000000"
- android:dividerHeight="5dip" />
-
- RelativeLayout>
可以一眼看出我们修改了它的控件标签,改为我们自己定义的类的完全路径。
最后是主角MainActivity.java,里面的一些代码我详细地给了注释。这里要注意的是适配器的使用。
- public class MainActivity extends Activity {
- private RefreshListView listView;
- private SimpleAdapter simple_adapter;
- private List
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- listView = (RefreshListView) findViewById(R.id.listview);
- iniData();
-
-
-
-
-
-
-
-
-
-
- simple_adapter = new SimpleAdapter(MainActivity.this, list,
- R.layout.listview_item, new String[] { "image", "text" },
- new int[] { R.id.image, R.id.text });
-
- listView.setAdapter(simple_adapter);
-
- }
-
-
- private List
- list = new ArrayList
- for (int i = 0; i < 20; i++) {
- Map map = new HashMap();
-
- map.put("text", i);
- map.put("image", R.drawable.ic_launcher);
- list.add(map);
- }
-
- return list;
- }
-
- @Override
- public boolean onCreateOptionsMenu(Menu menu) {
-
- getMenuInflater().inflate(R.menu.main, menu);
- return true;
- }
-
- }
到了这一步,应该能够实现图片+文字的listview了吧,喝口茶,我们继续看下去。
二、加入隐藏的Header
这里我们要说一下下拉刷新的实现思路了:
首先,我们平常用到的下拉刷新,都是在下拉后屏幕上方显示出一些之前被隐藏的控件,类似下拉的箭头、progress bar等等。
那我们可以直接把它们设置为不可见吗?显然是不可以的。因为这些空间的显示与否,有一个渐变的过程,不是刷一下就出来的。
所以我们应该这样做:
加入一个隐藏的布局,放在屏幕上方。根据下拉的范围来显示响应的控件。
这一步,我们要实现的是加入隐藏的布局,具体怎样根据下拉的状态来实时调整Header的显示状态,我们在下文细说。
我们为了需要隐藏的header再自定义个新的布局,header.xml:
-
- android:layout_width="match_parent"
- android:layout_height="wrap_content"
- android:orientation="vertical" >
-
-
- android:layout_width="match_parent"
- android:layout_height="wrap_content"
- android:paddingBottom="10dip"
- android:paddingTop="10dip" >
-
-
- android:id="@+id/layout"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_centerInParent="true"
- android:gravity="center"
- android:orientation="vertical" >
-
-
- android:id="@+id/tip"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="下拉可以刷新!" />
-
-
- android:id="@+id/lastupdate_time"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content" />
-
-
-
- android:id="@+id/arrow"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_toLeftOf="@id/layout"
- android:layout_marginRight="20dip"
- android:src="@drawable/pull_to_refresh_arrow" />
-
-
- android:id="@+id/progress"
- style="?android:attr/progressBarStyleSmall"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_toLeftOf="@id/layout"
- android:layout_marginRight="20dip"
- android:visibility="gone" />
-
-
-
这个布局中包含了提示语“下拉可以刷新”、最新更新时间、下拉箭头的图片(已经预先放在drawable文件夹中了,读者可以自己找个图片放进去,命名为pull_to_refresh_arrow.phg)、一个更新时才显示的progressbar(现在是隐藏的)。
为了把这个布局加到我们定义的List,我们需要改写之前自定义的RefreshLIstview控件:
- public class RefreshListView extends ListView {
- View header;
- int headerHeight;
- public RefreshListView(Context context) {
- super(context);
-
- initView(context);
- }
-
- public RefreshListView(Context context, AttributeSet attrs) {
- super(context, attrs);
-
- initView(context);
- }
-
- public RefreshListView(Context context, AttributeSet attrs, int defStyle) {
- super(context, attrs, defStyle);
-
- initView(context);
- }
-
-
-
-
- private void initView(Context context) {
- LayoutInflater inflater = LayoutInflater.from(context);
- header = inflater.inflate(R.layout.header, null);
- measureView(header);
- headerHeight = header.getMeasuredHeight();
- Log.i("tag", "headerHeight = " + headerHeight);
-
- this.addHeaderView(header);
- }
-
-
-
-
- private void measureView(View view) {
- ViewGroup.LayoutParams p = view.getLayoutParams();
- if (p == null) {
- p = new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT,
- ViewGroup.LayoutParams.WRAP_CONTENT);
- }
- int width = ViewGroup.getChildMeasureSpec(0, 0, p.width);
- int height;
- int tempHeight = p.height;
- if (tempHeight > 0) {
- height = MeasureSpec.makeMeasureSpec(tempHeight,
- MeasureSpec.EXACTLY);
- } else {
- height = MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED);
- }
- view.measure(width, height);
- }
-
-
-
-
- private void topPadding(int topPadding) {
- header.setPadding(header.getPaddingLeft(), topPadding,
- header.getPaddingRight(), header.getPaddingBottom());
- header.invalidate();
- }
- }
主要实现的是把新的header加入进去,同时把它隐藏。现在我把隐藏header的那行代码注释了,我们看看现在的效果:
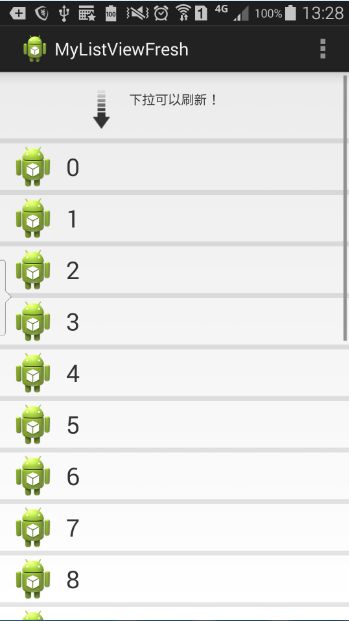
如果去除注释,header就被隐藏。
三、加入滚动监听实时调整Header显示状态以及刷新后添加数据
思路:
添加屏幕触摸监听和屏幕滚动监听。
触摸监听尤其重要:
在触摸时记录下触摸坐标的Y值即startY,然后在移动过程中监听当前的Y值,根据两者的插值判断当前的移动距离,与一些临界值做比较。
比较之后得出当前的状态:提示下拉状态、提示释放状态、刷新状态。根据当前的状态来刷新header布局的显示情况。
滚动监听的作用是判断当前是否是列表的顶端(通过判断当前可见的第一个item的position是否为0),以及在之后判断屏幕的滚动状态。
另外在自定义的Listview类中定义了一个接口,在mainactivity中实现这个接口,用来对数据进行刷新。我们在刷新的时候用了Handler延迟了两秒,以清晰地看到刷新的效果。
修改后的MainActivity以及ListView:
ListView: (里面很多注释,自己看着应该很好理解)
- public class RefreshListView extends ListView implements OnScrollListener {
- View header;
- int headerHeight;
- int firstVisibleItem;
- int scrollState;
- boolean isRemark;
- int startY;
-
- int state;
- final int NONE = 0;
- final int PULL = 1;
- final int RELEASE = 2;
- final int REFRESHING = 3;
- IRefreshListener iRefreshListener;
- public RefreshListView(Context context) {
- super(context);
-
- initView(context);
- }
-
- public RefreshListView(Context context, AttributeSet attrs) {
- super(context, attrs);
-
- initView(context);
- }
-
- public RefreshListView(Context context, AttributeSet attrs, int defStyle) {
- super(context, attrs, defStyle);
-
- initView(context);
- }
-
-
-
-
-
-
- private void initView(Context context) {
- LayoutInflater inflater = LayoutInflater.from(context);
- header = inflater.inflate(R.layout.header, null);
- measureView(header);
- headerHeight = header.getMeasuredHeight();
- Log.i("tag", "headerHeight = " + headerHeight);
- topPadding(-headerHeight);
- this.addHeaderView(header);
- this.setOnScrollListener(this);
- }
-
-
-
-
-
-
- private void measureView(View view) {
- ViewGroup.LayoutParams p = view.getLayoutParams();
- if (p == null) {
- p = new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT,
- ViewGroup.LayoutParams.WRAP_CONTENT);
- }
- int width = ViewGroup.getChildMeasureSpec(0, 0, p.width);
- int height;
- int tempHeight = p.height;
- if (tempHeight > 0) {
- height = MeasureSpec.makeMeasureSpec(tempHeight,
- MeasureSpec.EXACTLY);
- } else {
- height = MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED);
- }
- view.measure(width, height);
- }
-
-
-
-
-
-
- private void topPadding(int topPadding) {
- header.setPadding(header.getPaddingLeft(), topPadding,
- header.getPaddingRight(), header.getPaddingBottom());
- header.invalidate();
- }
-
- @Override
- public void onScroll(AbsListView view, int firstVisibleItem,
- int visibleItemCount, int totalItemCount) {
-
- this.firstVisibleItem = firstVisibleItem;
- }
-
- @Override
- public void onScrollStateChanged(AbsListView view, int scrollState) {
-
- this.scrollState = scrollState;
- }
-
-
-
-
-
-
-
-
- @Override
- public boolean onTouchEvent(MotionEvent ev) {
-
- switch (ev.getAction()) {
- case MotionEvent.ACTION_DOWN:
- if (firstVisibleItem == 0) {
- isRemark = true;
- startY = (int) ev.getY();
- }
- break;
-
- case MotionEvent.ACTION_MOVE:
- onMove(ev);
- break;
- case MotionEvent.ACTION_UP:
- if (state == RELEASE) {
-
- state = REFRESHING;
-
- refreshViewByState();
- iRefreshListener.onRefresh();
- } else if (state == PULL) {
-
- state = NONE;
- isRemark = false;
- refreshViewByState();
- }
- break;
- }
- return super.onTouchEvent(ev);
- }
-
-
-
-
-
-
-
-
-
- private void onMove(MotionEvent ev) {
- if (!isRemark) {
- return;
- }
- int tempY = (int) ev.getY();
- int space = tempY - startY;
- int topPadding = space - headerHeight;
- switch (state) {
- case NONE:
- if (space > 0) {
- state = PULL;
- refreshViewByState();
- }
- break;
- case PULL:
- topPadding(topPadding);
-
- if (space > headerHeight + 30
- && scrollState == SCROLL_STATE_TOUCH_SCROLL) {
- state = RELEASE;
- refreshViewByState();
- }
- break;
- case RELEASE:
- topPadding(topPadding);
-
- if (space < headerHeight + 30) {
- state = PULL;
- refreshViewByState();
- }
- break;
- }
- }
-
-
-
-
- private void refreshViewByState() {
-
- TextView tip = (TextView) header.findViewById(R.id.tip);
- ImageView arrow = (ImageView) header.findViewById(R.id.arrow);
- ProgressBar progress = (ProgressBar) header.findViewById(R.id.progress);
- RotateAnimation anim = new RotateAnimation(0, 180,
- RotateAnimation.RELATIVE_TO_SELF, 0.5f,
- RotateAnimation.RELATIVE_TO_SELF, 0.5f);
- anim.setDuration(500);
- anim.setFillAfter(true);
- RotateAnimation anim1 = new RotateAnimation(180, 0,
- RotateAnimation.RELATIVE_TO_SELF, 0.5f,
- RotateAnimation.RELATIVE_TO_SELF, 0.5f);
- anim1.setDuration(500);
- anim1.setFillAfter(true);
- switch (state) {
- case NONE:
- arrow.clearAnimation();
- topPadding(-headerHeight);
- break;
-
- case PULL:
- arrow.setVisibility(View.VISIBLE);
- progress.setVisibility(View.GONE);
- tip.setText("下拉可以刷新!");
- arrow.clearAnimation();
- arrow.setAnimation(anim1);
- break;
- case RELEASE:
- arrow.setVisibility(View.VISIBLE);
- progress.setVisibility(View.GONE);
- tip.setText("松开可以刷新!");
- arrow.clearAnimation();
- arrow.setAnimation(anim);
- break;
- case REFRESHING:
- topPadding(50);
- arrow.setVisibility(View.GONE);
- progress.setVisibility(View.VISIBLE);
- tip.setText("正在刷新...");
- arrow.clearAnimation();
- break;
- }
- }
-
-
-
-
- public void refreshComplete() {
- state = NONE;
- isRemark = false;
- refreshViewByState();
- TextView lastupdatetime = (TextView) header
- .findViewById(R.id.lastupdate_time);
- SimpleDateFormat format = new SimpleDateFormat("yyyy年MM月dd日 hh:mm:ss");
- Date date = new Date(System.currentTimeMillis());
- String time = format.format(date);
- lastupdatetime.setText(time);
- }
-
- public void setInterface(IRefreshListener iRefreshListener){
- this.iRefreshListener = iRefreshListener;
- }
-
-
-
-
-
- public interface IRefreshListener{
- public void onRefresh();
- }
- }
MainActivity: (添加了接口回调,即在listview中调用main的添加数据的方法)
- public class MainActivity extends Activity implements IRefreshListener {
- private RefreshListView listView;
- private SimpleAdapter simple_adapter;
- private List
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- listView = (RefreshListView) findViewById(R.id.listview);
- iniData();
-
-
-
-
-
-
-
-
-
-
- simple_adapter = new SimpleAdapter(MainActivity.this, list,
- R.layout.listview_item, new String[] { "image", "text" },
- new int[] { R.id.image, R.id.text });
-
- listView.setAdapter(simple_adapter);
-
- listView.setInterface(this);
- }
-
-
- private List
- list = new ArrayList
- for (int i = 0; i < 20; i++) {
- Map map = new HashMap();
-
- map.put("text", i);
- map.put("image", R.drawable.ic_launcher);
- list.add(map);
- }
-
- return list;
- }
-
- @Override
- public boolean onCreateOptionsMenu(Menu menu) {
-
- getMenuInflater().inflate(R.menu.main, menu);
- return true;
- }
-
-
-
-
- @Override
- public void onRefresh() {
-
- Handler handler = new Handler();
- handler.postDelayed(new Runnable() {
- @Override
- public void run() {
-
- Map map = new HashMap();
- map.put("text", "滚动添加 ");
- map.put("image", R.drawable.ic_launcher);
- list.add(0, map);
- listView.setAdapter(simple_adapter);
- simple_adapter.notifyDataSetChanged();
- listView.refreshComplete();
- }
- }, 2000);
- }
-
- }