#include
#include
using namespace std;
#define LIST_INIT_SIZE 100
#define LISTINCREMENT 10
typedef int ElemType;
typedef int Status;
typedef struct
{
ElemType *elem;
ElemType lenth;
ElemType listsize;
}List;
Status DestroyList(List& L);
Status ClearList(List& L);
Status ListEmpty(List L);
int ListLenth(List L);
void GetElem(List L,int i,int&e);
Status compare(ElemType e,ElemType a);
void ShowList(List L);
Status InputList(List& L,int lenth);
void unions(List &La,List Lb);
void MergeList1(List La,List Lb,List &Lc);
Status InitList(List& L);
Status ListInsert(List &L,int i,ElemType e);
Status ListDelete(List& L,int i,ElemType &e);
int LocateElem(List& L,ElemType e,Status(*compare)(ElemType,ElemType));
void MergeList2(List La,List Lb,List &Lc);
int main()
{
List a,b;
cout<<'\n'<<"开始测试"<<"##算法2.3##"<<endl;
if(InitList(a))cout<<"表a初始化成功"<<endl;
if(InitList(b))cout<<"表b初始化成功"<<endl;
cout<<"测试完毕"<<"##算法2.3##"<<endl;
if(InputList(a,4))cout<<"数据导入成功!";
if(InputList(b,7))cout<<"数据导入成功!";
cout<<endl;
cout<<"表格a存储的数据为:"<<endl;ShowList(a);
cout<<"表格b存储的数据为:"<<endl;ShowList(b);
cout<<'\n'<<"开始测试"<<"##算法2.6##"<<endl;
if(LocateElem(a,8,compare))cout<<"元素‘8’位序排在第"<<LocateElem(a,8,compare)<<"位"<<endl;
cout<<"测试完毕"<<"##算法2.6##"<<endl;
cout<<'\n'<<"开始测试"<<"##算法2.7##"<<endl;
List c;InitList(c);
MergeList2(a,b,c);
ShowList(c);
cout<<"测试完毕"<<"##算法2.7##"<<endl;
cout<<'\n'<<"开始测试"<<"##算法2.2##"<<endl;
List d;InitList(d);
MergeList1(a,b,d);
ShowList(d);
cout<<"测试完毕"<<"##算法2.2##"<<endl;
cout<<'\n'<<"开始测试"<<"##算法2.4##"<<endl;
cout<<"开始表格c存储的数据为:"<<endl;ShowList(c);
ListInsert(c,1,1);
ListInsert(c,4,4);
cout<<"结束表格c存储的数据为:"<<endl;ShowList(c);
cout<<"测试完毕"<<"##算法2.4##"<<endl;
cout<<'\n'<<"开始测试"<<"##算法2.5##"<<endl;
cout<<"开始表格c存储的数据为:"<<endl;ShowList(c);
int i=0,j=0;
ListDelete(c,1,i);
ListDelete(c,4,j);
cout<<"结束表格c存储的数据为:"<<endl;ShowList(c);
cout<<"删除的数据为"<<i<<"和"<<j<<endl;
cout<<"测试完毕"<<"##算法2.5##"<<endl;
cout<<'\n'<<"开始测试"<<"##算法2.1##"<<endl;
cout<<"开始表格a存储的数据为:"<<endl;ShowList(a);
unions(a,b);
cout<<"结束表格a存储的数据为:"<<endl;ShowList(a);
cout<<"测试完毕"<<"##算法2.1##"<<endl;
return 0;
}
Status DestroyList(List& L)
{
free(L.elem);
if(!L.elem)return 1;
}
Status ClearList(List& L)
{
L.lenth=0;
return 1;
}
Status ListEmpty(List L)
{
if(L.lenth==0)return true;
return false;
}
int ListLenth(List L)
{
return L.lenth;
}
void GetElem(List L,int i,int&e)
{
if(i>=1&&i<=ListLenth(L))
e=L.elem[i-1];
}
Status compare(ElemType e,ElemType a)
{
if(e==a)return true;
else return false;
}
void ShowList(List L)
{
for(int i=0;i<L.lenth;i++)
{
cout<<L.elem[i]<<" ";
}
cout<<endl;
}
void SortList(List& L)
{
int i=0,j;
for(i;i<L.lenth-1;i++)
{
for(j=i+1;j<L.lenth;j++)
{
if(L.elem[i]<L.elem[j])
{
int temp=L.elem[i];
L.elem[i]=L.elem[j];
L.elem[j]=temp;
}
}
}
}
Status InputList(List& L,int lenth)
{
cout<<"输入元素:"<<endl;
if(lenth<=L.listsize)
{
for(int i=0;i<lenth;i++)
{
cin>>L.elem[i];
L.lenth++;
}
return 1;
}
else return 0;
}
void unions(List &La,List Lb)
{
int La_len=ListLenth(La),Lb_len=ListLenth(Lb),e;
for(int i=1;i<=Lb_len;i++)
{
GetElem(Lb,i,e);
if(!(LocateElem(La,e,compare)))ListInsert(La,++La_len,e);
}
}
void MergeList1(List La,List Lb,List &Lc)
{
InitList(Lc);
int i=1,j=1,k=0,ai=0,bj=0;
int La_len=ListLenth(La);int Lb_len=ListLenth(Lb);
while((i<=La_len)&&(j<=Lb_len))
{
GetElem(La,i,ai);GetElem(Lb,j,bj);
if(ai<=bj)
{
ListInsert(Lc,++k,ai);++i;
}
else
{
ListInsert(Lc,++k,bj);++j;
}
}
while(i<=La_len)
{
GetElem(La,i++,ai);ListInsert(Lc,++k,ai);
}
while(j<=Lb_len)
{
GetElem(Lb,j++,bj);ListInsert(Lc,++k,bj);
}
}
Status InitList(List& L)
{
L.elem=(ElemType*)malloc(sizeof(ElemType)*LIST_INIT_SIZE);
if(!L.elem)exit(OVERFLOW);
L.lenth=0;
L.listsize=LIST_INIT_SIZE;
return 1;
}
Status ListInsert(List &L,int i,ElemType e)
{
if(i<1||i>L.lenth+1)return 0;
int*q=NULL;
if(L.lenth>=L.listsize)
{
ElemType* newbase=(ElemType*)realloc(L.elem,(L.listsize+LISTINCREMENT)*sizeof(ElemType));
if(!newbase)exit(OVERFLOW);
L.elem=newbase;
L.listsize+=LISTINCREMENT;
}
q=&(L.elem[i-1]);
for(ElemType* p=&(L.elem[L.lenth-1]);p>=q;--p)
*(p+1)=*p;
*q=e;
++L.lenth;
return 1;
}
Status ListDelete(List& L,int i,ElemType &e)
{
if((i<1)||(i>L.lenth))return 0;
ElemType* p=&(L.elem[i-1]);
e=*p;
ElemType*q=L.elem+L.lenth-1;
for(++p;p<=q;++p)
*(p-1)=*p;
--L.lenth;
return 1;
}
int LocateElem(List& L,ElemType e,Status(*compare)(ElemType,ElemType))
{
int i=1;
int *p=L.elem;
while(i<=L.listsize&&!((*compare)(*p++,e)))++i;
if(i<=L.listsize) return i;
else return 0;
}
void MergeList2(List La,List Lb,List &Lc)
{
ElemType* pa=La.elem,*pb=Lb.elem;
Lc.listsize=Lc.lenth=La.lenth+Lb.lenth;
ElemType* pc=Lc.elem=(ElemType*)malloc(Lc.listsize*sizeof(ElemType));
if(!Lc.elem)exit(OVERFLOW);
int*pa_last=La.elem+La.lenth-1;
int*pb_last=Lb.elem+Lb.lenth-1;
while(pa<=pa_last&&pb<=pb_last)
{
if(*pa<=*pb) *pc++=*pa++;
else*pc++=*pb++;
}
while(pa<=pa_last)*pc++=*pa++;
while(pb<=pb_last)*pc++=*pb++;
}
结果:
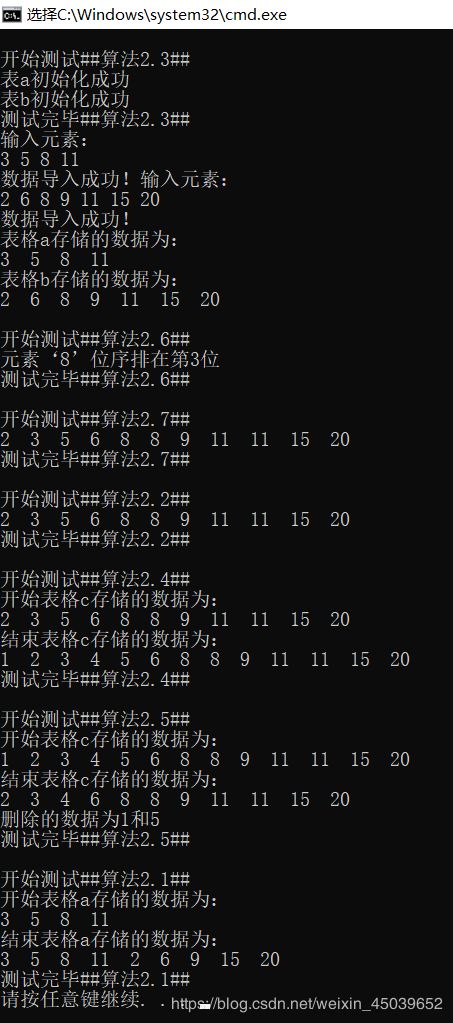