雷达图

import React, { useEffect, useState, useRef } from "react"
import "./chart.less"
import { Chart } from "@antv/g2";
import DataSet from '@antv/data-set';
const Charts = () => {
const fields = new Map()
fields.set(1, "产品功能")
fields.set(2, "服务质量")
fields.set(3, "项目管理")
fields.set(4, "实施过程")
fields.set(5, "整体效果")
fields.set(6, "专业技能")
const data = [
{ item: fields.get(1), a: 2 },
{ item: fields.get(2), a: 4 },
{ item: fields.get(3), a: 6 },
{ item: fields.get(4), a: 8 },
{ item: fields.get(5), a: 7 },
{ item: fields.get(6), a: 5 },
]
const [chart, setChart] = useState(null)
const chartRef = useRef()
const { DataView } = DataSet;
const dv = new DataView().source(data);
dv.transform({
type: 'fold',
fields: ['a'],
key: 'user',
value: 'score',
});
useEffect(() => {
const chart = new Chart({
container: 'c8',
autoFit: true,
height: 300,
});
setChart(() => chart)
}, [])
useEffect(() => {
chart && renderChart()
}, [chart])
const renderChart = () => {
chart.clear()
chart.data(dv.rows);
chart.scale('score', {
min: 0,
max: 10,
tickCount: 6,
nice: true
});
chart.coordinate('polar', {
radius: 0.8,
});
chart.axis('item', {
line: null,
tickLine: null,
grid: {
line: {
style: {
lineDash: null,
},
},
},
label: {
style: {
fontSize: 14,
fill: '#333',
},
offset: 15
}
});
chart.axis('score', {
line: null,
tickLine: null,
grid: {
line: {
type: 'line',
style: {
lineDash: null,
},
},
},
label: {
formatter: () => ""
}
});
chart
.line()
.position('item*score')
.size(2)
chart
.point()
.position('item*score')
.shape('circle')
.size(4)
.label("score", (score) => {
return {
textStyle: {
rotate: 0
},
autoRotate: false,
content: score,
}
})
.style({
stroke: '#fff',
lineWidth: 1,
fillOpacity: 1,
})
chart.tooltip({
showTitle: false,
})
chart
.area()
.position('item*score')
.tooltip("score*item", (score, item) => {
return {
showTitle: false,
name: item,
value: `${score}分`,
}
})
chart.render();
}
return (
<div className={"chart8"}>
<div id="c8" ref={chartRef} />
</div>
)
}
export default Charts
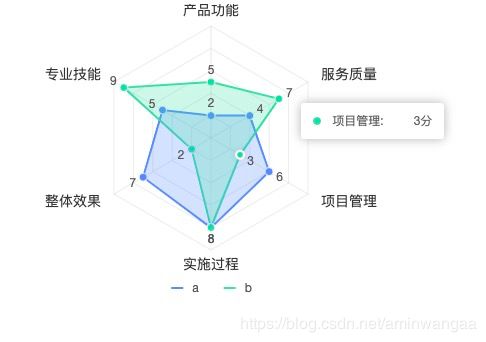
const data = [
{ item: fields.get(1), a: 2, b: 5 },
{ item: fields.get(2), a: 4, b: 7 },
{ item: fields.get(3), a: 6, b: 3 },
{ item: fields.get(4), a: 8, b: 8 },
{ item: fields.get(5), a: 7, b: 2 },
{ item: fields.get(6), a: 5, b: 9 },
]
chart
.line()
.position('item*score')
.size(2)
.color('user')
chart
.point()
.position('item*score')
.shape('circle')
.size(4)
.label("score", (score) => {
return {
textStyle: {
rotate: 0
},
autoRotate: false,
content: score,
}
})
.style({
stroke: '#fff',
lineWidth: 1,
fillOpacity: 1,
})
.color('user')
chart.tooltip({
showTitle: false,
})
chart
.area()
.position('item*score')
.tooltip("score*item", (score, item) => {
return {
showTitle: false,
name: item,
value: `${score}分`,
}
})
.color('user')