本文系转载,原文地址为iOS触摸事件全家桶
关于手势识别器即 UIGestureRecognizer
本身的使用不是本文要所讨论的内容,按下不表。此处要探讨的是:手势识别器与UIResponder的联系。
附:
UIGestureRecognizer
子类
离散型手势
- UITapGestureRecognizer
- UISwipeGestureRecognizer
持续性手势
- UIPinchGestureRecognizer
- UIRotationGestureRecognizer
- UIPanGestureRecognizer
- UIScreenEdgePanGestureRecognizer
- UILongPressGestureRecognizer
事实上,手势分为离散型手势(discrete gestures)和持续型手势(continuous gesture)。系统提供的离散型手势包括点按手势(UITapGestureRecognizer
)和轻扫手势(UISwipeGestureRecognizer
),其余均为持续型手势。
两者主要区别在于状态变化过程:
离散型:
识别成功:Possible —> Recognized
识别失败:Possible —> Failed持续型:
完整识别:Possible —> Began —> [Changed] —> Ended
不完整识别:Possible —> Began —> [Changed] —> Cancel
离散型手势
先抛开上面的场景,看一个简单的demo。
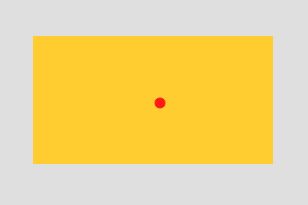
控制器的视图上add了一个View记为YellowView,并绑定了一个单击手势识别器。
// LXFViewController
- (void)viewDidLoad {
[super viewDidLoad];
UITapGestureRecognizer *tap = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(actionTap)];
[self.view addGestureRecognizer:tap];
}
- (void)actionTap{
NSLog(@"View Taped");
}
单击YellowView,日志打印如下:
-[YellowView touchesBegan:withEvent:]
View Taped
-[YellowView touchesCancelled:withEvent:]
从日志上看出YellowView最后Cancel了对触摸事件的响应,而正常应当是触摸结束后,YellowView的 touchesEnded:withEvent:
的方法被调用才对。另外,期间还执行了手势识别器绑定的action 。我从官方文档找到了这样的解释:
A window delivers touch events to a gesture recognizer before it delivers them to the hit-tested view attached to the gesture recognizer. Generally, if a gesture recognizer analyzes the stream of touches in a multi-touch sequence and doesn’t recognize its gesture, the view receives the full complement of touches. If a gesture recognizer recognizes its gesture, the remaining touches for the view are cancelled.The usual sequence of actions in gesture recognition follows a path determined by default values of the cancelsTouchesInView, delaysTouchesBegan, delaysTouchesEnded properties.
大致理解是,Window在将事件传递给hit-tested view之前,会先将事件传递给相关的手势识别器并由手势识别器优先识别。若手势识别器成功识别了事件,就会取消hit-tested view对事件的响应;若手势识别器没能识别事件,hit-tested view才完全接手事件的响应权。
一句话概括:手势识别器比UIResponder具有更高的事件响应优先级!!
按照这个解释,Window在将事件传递给hit-tested view即YellowView之前,先传递给了控制器根视图上的手势识别器。手势识别器成功识别了该事件,通知Application取消YellowView对事件的响应。
然而看日志,却是YellowView的 touchesBegan:withEvent:
先调用了,既然手势识别器先响应,不应该上面的action先执行吗,这又怎么解释?事实上这个认知是错误的。手势识别器的action的调用时机(即此处的 actionTap
)并不是手势识别器接收到事件的时机,而是手势识别器成功识别事件后的时机,即手势识别器的状态变为UIGestureRecognizerStateRecognized。因此从该日志中并不能看出事件是优先传递给手势识别器的,那该怎么证明Window先将事件传递给了手势识别器?
要解决这个问题,只要知道手势识别器是如何接收事件的,然后在接收事件的方法中打印日志对比调用时间先后即可。说起来你可能不信,手势识别器对于事件的响应也是通过这4个熟悉的方法来实现的。
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event;
需要注意的是,虽然手势识别器通过这几个方法来响应事件,但它并不是UIResponder的子类,相关的方法声明在 UIGestureRecognizerSubclass.h
中。
这样一来,我们便可以自定义一个单击手势识别器的类,重写这几个方法来监听手势识别器接收事件的时机。创建一个UITapGestureRecognizer的子类,重写响应事件的方法,每个方法中调用父类的实现,并替换demo中的手势识别器。另外需要在.m文件中引入 import
,因为相关方法声明在该头文件中。
// LXFTapGestureRecognizer (继承自UITapGestureRecognizer)
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"%s",__func__);
[super touchesBegan:touches withEvent:event];
}
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"%s",__func__);
[super touchesMoved:touches withEvent:event];
}
- (void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"%s",__func__);
[super touchesEnded:touches withEvent:event];
}
- (void)touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"%s",__func__);
[super touchesCancelled:touches withEvent:event];
}
现在,再次点击YellowView,日志如下:
-[LXFTapGestureRecognizer touchesBegan:withEvent:]
-[YellowView touchesBegan:withEvent:]
-[LXFTapGestureRecognizer touchesEnded:withEvent:]
View Taped
-[YellowView touchesCancelled:withEvent:]
很明显,确实是手势识别器先接收到了事件。之后手势识别器成功识别了手势,执行了action,再由Application取消了YellowView对事件的响应。
Window怎么知道要把事件传递给哪些手势识别器?
之前探讨过Application怎么知道要把event传递给哪个Window,以及Window怎么知道要把event传递给哪个hit-tested view的问题,答案是这些信息都保存在event所绑定的touch对象上。手势识别器也是一样的,event绑定的touch对象上维护了一个手势识别器数组,里面的手势识别器毫无疑问是在hit-testing的过程中收集的。打个断点看一下touch上绑定的手势识别器数组:
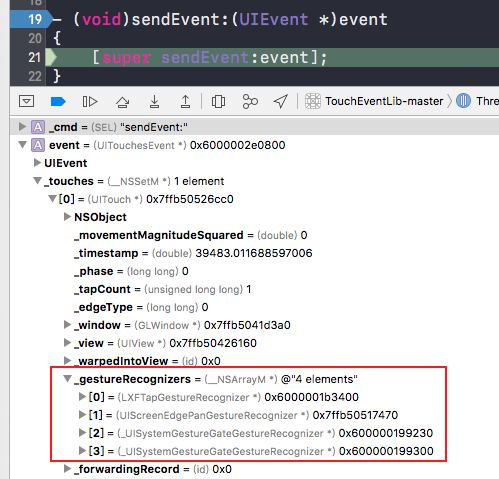
Window先将事件传递给这些手势识别器,再传给hit-tested view。一旦有手势识别器成功识别了手势,Application就会取消hit-tested view对事件的响应。
持续型手势
将上面Demo中视图绑定的单击手势识别器用滑动手势识别器(UIPanGestureRecognizer)替换。
- (void)viewDidLoad {
[super viewDidLoad];
UIPanGestureRecognizer *pan = [[UIPanGestureRecognizer alloc] initWithTarget:self action:@selector(actionPan)];
[self.view addGestureRecognizer:pan];
}
- (void)actionPan{
NSLog(@"View panned");
}
在YellowView上执行一次滑动:
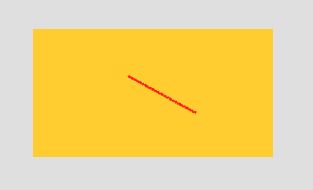
日志打印如下:
-[YellowView touchesBegan:withEvent:]
-[YellowView touchesMoved:withEvent:]
-[YellowView touchesMoved:withEvent:]
-[YellowView touchesMoved:withEvent:]
View panned
-[YellowView touchesCancelled:withEvent:]
View panned
View panned
View panned
...
在一开始滑动的过程中,手势识别器处在识别手势阶段,滑动产生的连续事件既会传递给手势识别器又会传递给YellowView,因此YellowView的 touchesMoved:withEvent:
在开始一段时间内会持续调用;当手势识别器成功识别了该滑动手势时,手势识别器的action开始调用,同时通知Application取消YellowView对事件的响应。之后仅由滑动手势识别器接收事件并响应,YellowView不再接收事件。
另外,在滑动的过程中,若手势识别器未能识别手势,则事件在触摸滑动过程中会一直传递给hit-tested view,直到触摸结束。读者可自行验证。
先总结一下手势识别器与UIResponder对于事件响应的联系:
- 当触摸发生或者触摸的状态发生变化时,Window都会传递事件寻求响应。
- Window先将绑定了触摸对象的事件传递给触摸对象上绑定的手势识别器,再发送给触摸对象对应的hit-tested view。
- 手势识别器识别手势期间,若触摸对象的触摸状态发生变化,事件都是先发送给手势识别器再发送给hit-test view。
- 手势识别器若成功识别了手势,则通知Application取消hit-tested view对于事件的响应,并停止向hit-tested view发送事件;
- 若手势识别器未能识别手势,而此时触摸并未结束,则停止向手势识别器发送事件,仅向hit-test view发送事件。
- 若手势识别器未能识别手势,且此时触摸已经结束,则向hit-tested view发送end状态的touch事件以停止对事件的响应。
手势识别器的3个属性
@property(nonatomic) BOOL cancelsTouchesInView;
@property(nonatomic) BOOL delaysTouchesBegan;
@property(nonatomic) BOOL delaysTouchesEnded;
cancelsTouchesInView
默认为YES
。表示当手势识别器成功识别了手势之后,会通知Application取消响应链对事件的响应,并不再传递事件给hit-test view
。
若设置成NO
,表示手势识别成功后不取消响应链对事件的响应,事件依旧会传递给hit-test view
。
demo中设置:pan.cancelsTouchesInView = NO
滑动时日志如下:
-[YellowView touchesBegan:withEvent:]
-[YellowView touchesMoved:withEvent:]
-[YellowView touchesMoved:withEvent:]
-[YellowView touchesMoved:withEvent:]
View panned
-[YellowView touchesMoved:withEvent:]
View panned
View panned
-[YellowView touchesMoved:withEvent:]
View panned
-[YellowView touchesMoved:withEvent:]
即便滑动手势识别器识别了手势,Application也会依旧发送事件给YellowView。
delaysTouchesBegan
默认为 NO
。默认情况下手势识别器在识别手势期间,当触摸状态发生改变时,Application都会将事件传递给手势识别器和hit-tested view
;
若设置成YES
,则表示手势识别器在识别手势期间,截断事件,即不会将事件发送给hit-tested view
。
设置pan.delaysTouchesBegan = YES
日志如下:
View panned
View panned
View panned
View panned
因为滑动手势识别器在识别期间,事件不会传递给YellowView,因此期间YellowView的 touchesBegan:withEvent:
和 touchesMoved:withEvent:
都不会被调用;而后滑动手势识别器成功识别了手势,也就独吞了事件,不会再传递给YellowView。因此只打印了手势识别器成功识别手势后的action调用。
delaysTouchesEnded
默认为YES
。当手势识别失败时,若此时触摸已经结束,会延迟
一小段时间(0.15s)再调用响应者的 touchesEnded:withEvent:;
若设置成NO
,则在手势识别失败时会立即
通知Application发送状态为end的touch事件给hit-tested view以调用 touchesEnded:withEvent: 结束事件响应。
总结:手势识别器比响应链具有更高的事件响应优先级。