@[Python][ch01]
教6个月前的自己学Python
Python 数据类型
- python3有六大类数据 Number(数字)、String(字符串/廖雪峰)、List(列表)、Tuple(元组)、Sets(集合)、Dictionary(字典)
数据类型练习:
- Dictionary
name = {1:'zhang', 3:'nai', 'a': 'tao', 3: 'neil', 4: 'tao', 1: 'Neil'}
print (name)
print (name.values())
print (name.keys())
b = set(name.values())
print (b)
c = set(name.keys())
print (c)
d = b&c
print (d)
diff = b^c
print (diff)
- Dictionary练习结果输出:
{1: 'Neil', 3: 'neil', 'a': 'tao', 4: 'tao'}
dict_values(['Neil', 'neil', 'tao', 'tao'])
dict_keys([1, 3, 'a', 4])
{'neil', 'Neil', 'tao'}
{1, 3, 'a', 4}
set()
{1, 'tao', 3, 'a', 4, 'neil', 'Neil'}
- List : for ch0 for random 4 different numbers
# random 4 different numbers - 02
# -*- coding: utf-8 -*-
import random
guess_number = []
list = [i for i in range(10)]
print ("Original list =", list)
# compare above out put style,
for i in range(4):
print (("i = %s") % i)
j = random.randint(0, len(list) - 1)
print (("j = %s") % j)
guess_number.append(list[j])
print(("list['j' = %s] = %s ") % (j, list[j]))
print("guess_number = %s " % guess_number)
# you may can optimize above output code.
print(("list.pop('j' = %s) = %s") % (j,list.pop(j)))
## list.pop(j)
# It very import to cancel above code, because list.pop(j) already excute in code "print.....
print(("list after pop ['j' = %s]) = %s") % (j, list))
print ("*"*10)
print ("*"*20)
print(f'method 2 (list): guess_number = {guess_number}')
print (len(list)-1)
print (list.pop(j))
# you can guess why abve list.pop(j) = 7?
print (guess_number[1:3])
- List练习结果输出 : ch0 for random 4 different numbers
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07> py36 ex10.py
Original list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
i = 0
j = 5
list['j' = 5] = 5
guess_number = [5]
list.pop('j' = 5) = 5
list after pop ['j' = 5]) = [0, 1, 2, 3, 4, 6, 7, 8, 9]
**********
********************
i = 1
j = 2
list['j' = 2] = 2
guess_number = [5, 2]
list.pop('j' = 2) = 2
list after pop ['j' = 2]) = [0, 1, 3, 4, 6, 7, 8, 9]
**********
********************
i = 2
j = 6
list['j' = 6] = 8
guess_number = [5, 2, 8]
list.pop('j' = 6) = 8
list after pop ['j' = 6]) = [0, 1, 3, 4, 6, 7, 9]
**********
********************
i = 3
j = 4
list['j' = 4] = 6
guess_number = [5, 2, 8, 6]
list.pop('j' = 4) = 6
list after pop ['j' = 4]) = [0, 1, 3, 4, 7, 9]
**********
********************
method 2 (list): guess_number = [5, 2, 8, 6]
5
7
[2, 8]
- List code2
# random 4 differernt numbers-01
import random
guess_number = []
list = [i for i in range(10)]
guess_number = random.sample(list, 4)
# for test
print (guess_number)
while guess_number[0] == 0:
guess_number = random.sample(list, 4)
# for test
print("new_guess_number", guess_number)
print (f'method 1(random.sample): guess_number = {guess_number}')
# for test different print code
print (guess_number)
- code2 输出结果
For comparing the code 1 and 2 , which achieve the sam function, and code 2 even better on functions, for example, code 2 sucessfully avoid the first num = 0
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07> py36 ex09.py
[5, 7, 9, 2]
method 1(random.sample): guess_number = [5, 7, 9, 2]
[5, 7, 9, 2]
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07> py36 ex09.py
[4, 1, 9, 6]
method 1(random.sample): guess_number = [4, 1, 9, 6]
[4, 1, 9, 6]
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07> py36 ex09.py
[9, 4, 6, 8]
method 1(random.sample): guess_number = [9, 4, 6, 8]
[9, 4, 6, 8]
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07> py36 ex09.py
[0, 4, 7, 2]
new_guess_number [2, 7, 8, 9]
method 1(random.sample): guess_number = [2, 7, 8, 9]
[2, 7, 8, 9]
PS C:\Users\CNNEZHA2\mystuff\Python-exercises_2017_07>
函数
菜鸟教程:
文本记录(Markdown)
1. 目前文档发布流程 (使用maxiang 编辑,发布)
流程图(maxing代码):
st=>start: 在maxiang编辑
op1=>operation: maxiang直接同步到evernote
cond=>condition: 检查,没有错误
op2=>operation: 复制maxing代码到发布
e2=>end: 最终结束所有发布与保存!
st->op1->cond
cond(yes)->op2->e2
cond(no)->op1
- 流程图如下:
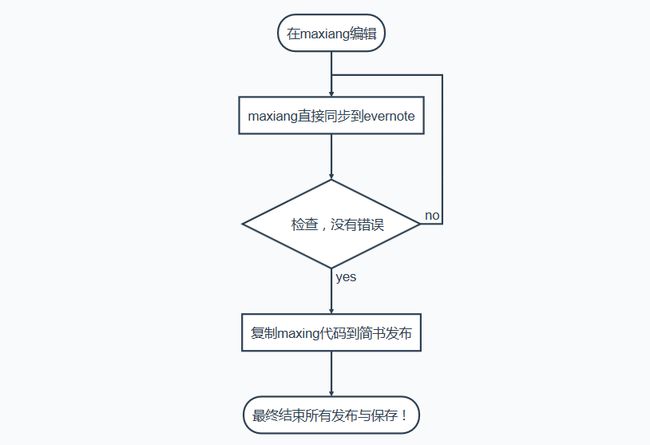
2. 目前 maxiang 编辑中的代码编辑流程
- 要在maxing中加入整片的代码段,代码段的拷贝来源要从MarkdownPad2来,Atom上直接拷贝始终会出现顺序行的问题, 相关流程如下:
流程图(maxing代码):
st=>start: 从Atom拷贝到MarkdownPad2
op1=>start: 拷贝代码从MarkdownPad2 到 maxing
e1=>end: 检查相关代码间距
e2=>end: 发布
MarkdwonPad2 => maxing
st->op1->e1->e2
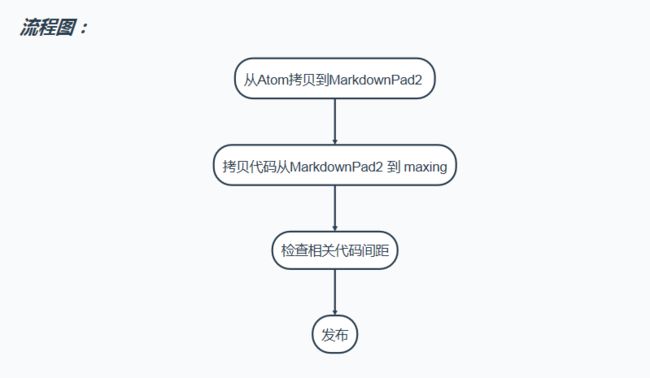
Class 类.....累 /面向对象编程......对象
定义:
Class是用来描述具有相同的属性和方法的对象的集合。它定义了该集合中每个对象所共有的属性和方法。
对象是类的实例 & 类是抽象的模板
实例变量:定义在方法中的变量,只作用于当前实例的类
方法:类中定义的函数。
-
对象:通过类定义的数据结构实例。对象包括两个数据成员(类变量和实例变量)和方法
面向对象 (Object Oriented Programming) 的设计思想是从自然界中来的,因为在自然界中,类(Class)和实例(Instance)的概念是很自然的。Class是一种抽象概念,比如我们定义的Class——Student,是指学生这个概念,而实例(Instance)则是一个个具体的Student,比如,Bart Simpson和Lisa Simpson是两个具体的Student。所以,面向对象的设计思想是抽象出Class,根据Class创建Instance。面向对象的抽象程度又比函数要高,因为一个Class既包含数据,又包含操作数据的方法。
实例:
实例化:创建一个类的实例,类的具体对象。灰常重要,类始终是要服务与对象,也就是要实例化。
第一种方法init()方法是一种特殊的方法,被称为类的构造函数或初始化方法,当创建了这个类的实例时就会调用该方法. 也就是说在实例代码出来时,马上就对对象按照init() (方法)进行了初始化。
init方法的第一个参数永远是self,表示创建的实例本身,因此,在init方法内部,就可以把各种属性绑定到self,因为self就指向创建的实例本身
Generator functions A function or method which uses the yield statement (see section The yield statement) is called a generator function. Such a function, when called, always returns an iterator object which can be used to execute the body of the function: calling the iterator’s iterator.next() method will cause the function to execute until it provides a value using the yield statement. When the function executes a return statement or falls off the end, a StopIteration exception is raised and the iterator will have reached the end of the set of values to be returned.
self : init方法的第一个参数
类的方法与普通的函数只有一个特别的区别——它们必须有一个额外的第一个参数名称, 按照惯例它的名称是 self.
self 代表类的实例,self 在定义类的方法时是必须有的,虽然在调用时不必传入相应的参数。
self 代表的是类的实例,代表当前对象的地址,而 self.class 则指向类。self 不是 python 关键字,我们把他换成 runoob 也是可以正常执行的.
When an instance method object is called, the underlying function (func) is called, inserting the class instance (self) in front of the argument list. For instance, when C is a class which contains a definition for a function f(), and x is an instance of C, calling x.f(1) is equivalent to calling C.f(x, 1).
Python内置类属性:
- dict : 类的属性(包含一个字典,由类的数据属性组成)
- doc : 类的文档字符串
- name: 类名
- module: 类定义所在的模块(类的全名是 main.className,如果类位于一个导入模块mymod中,那么className.module 等于 mymod)
- bases : 类的所有父类构成元素(包含了一个由所有父类组成的元组)
类的继承:
- 继承语法 class 派生类名(基类名)://... 基类名写在括号里,基本类是在类定义的时候,在元组之中指明的
- 在继承中基类的构造(init()方法)不会被自动调用,它需要在其派生类的构造中亲自专门调用
- 在调用基类的方法时,需要加上基类的类名前缀,且需要带上self参数变量。区别于在类中调用普通函数时并不需要带上self参数
- Python总是首先查找对应类型的方法,如果它不能在派生类中找到对应的方法,它才开始到基类中逐个查找。(先在本类中查找调用的方法,找不到才去基类中找)。
- 通常,如果没有合适的继承类,就使用object类,这是所有类最终都会继承的类.
类的私有属性:
- __private_attrs:两个下划线开头,声明该属性为私有,不能在类的外部被使用或直接访问。在类内部的方法中使用时 self.__private_attrs
- Python不允许实例化的类访问私有数据,但你可以使用 object._className__attrName 访问属性
python对象销毁(垃圾回收):
Python 使用了引用计数这一简单技术来跟踪和回收垃圾。在 Python 内部记录着所有使用中的对象各有多少引用。一个内部跟踪变量,称为一个引用计数器。当对象被创建时, 就创建了一个引用计数, 当这个对象不再需要时, 也就是说, 这个对象的引用计数变为 0 时, 它被垃圾回收。但是回收不是"立即"的, 由解释器在适当的时机,将垃圾对象占用的内存空间回收。
垃圾回收机制不仅针对引用计数为0的对象,同样也可以处理循环引用的情况。循环引用指的是,两个对象相互引用,但是没有其他变量引用他们。这种情况下,仅使用引用计数是不够的。Python 的垃圾收集器实际上是一个引用计数器和一个循环垃圾收集器。作为引用计数的补充, 垃圾收集器也会留心被分配的总量很大(及未通过引用计数销毁的那些)的对象。 在这种情况下, 解释器会暂停下来, 试图清理所有未引用的循环。
Python code style
1. Immediately inside parentheses, brackets or braces.
Yes: spam(ham[1], {eggs: 2})
No: spam( ham[ 1 ], { eggs: 2 } )
2. Immediately before a comma, semicolon, or colon:
Yes: if x == 4: print x, y; x, y = y, x
No: if x == 4 : print x , y ; x , y = y , x
3. However, in a slice the colon acts like a binary operator, and should have equal amounts on either side (treating it as the operator with the lowest priority). In an extended slice, both colons must have the same amount of spacing applied. Exception: when a slice parameter is omitted, the space is omitted
Yes:
ham[1:9], ham[1:9:3], ham[:9:3], ham[1::3], ham[1:9:]
ham[lower:upper], ham[lower:upper:], ham[lower::step]
ham[lower+offset : upper+offset]
ham[: upper_fn(x) : step_fn(x)], ham[:: step_fn(x)]
ham[lower + offset : upper + offset]
No:
ham[lower + offset:upper + offset]
ham[1: 9], ham[1 :9], ham[1:9 :3]
ham[lower : : upper]
ham[ : upper]