目录
- 3.Circuits
- 3.1Combinational Logic
- 3.1.1Basic Gates
- 3.1.2 Multiplexers
- 3.1.3 Arithmetic Circuits
- 3.1.4 Karnaugh Map to Circuit
3.Circuits
3.1Combinational Logic
3.1.1Basic Gates
module top_module (
input in,
output out);
assign out = in;
endmodule
module top_module (
output out);
assign out = 1'b0;
endmodule
module top_module (
input in1,
input in2,
output out);
assign out =~(in1 | in2);
endmodule
module top_module (
input in1,
input in2,
output out);
assign out = in1 & (~in2);
endmodule
module top_module (
input in1,
input in2,
input in3,
output out);
assign out = in3 ^ ~(in1^in2);
endmodule
module top_module(
input a, b,
output out_and,
output out_or,
output out_xor,
output out_nand,
output out_nor,
output out_xnor,
output out_anotb
);
assign out_and = a & b;
assign out_or = a | b;
assign out_xor = a ^ b;
assign out_nand = ~ (a & b);
assign out_nor = ~ (a | b);
assign out_xnor = ~ (a ^ b);
assign out_anotb = a & ~b;
endmodule
module top_module (
input p1a, p1b, p1c, p1d,
output p1y,
input p2a, p2b, p2c, p2d,
output p2y );
assign p2y = ~(p2a & p2b & p2c & p2d);
assign p1y = ~(p1a & p1b & p1c & p1d);
endmodule
- Truth tables
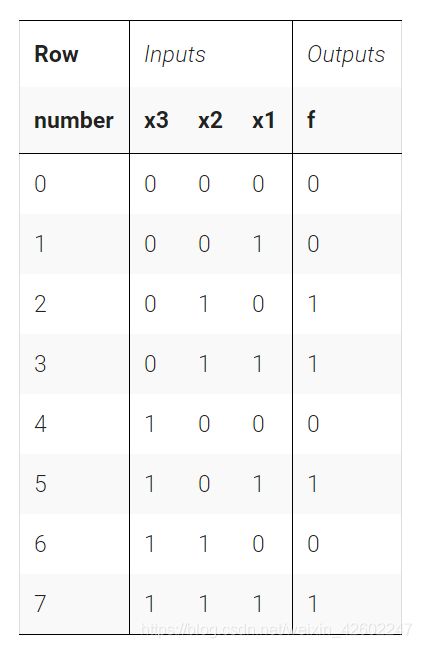
module top_module(
input x3,
input x2,
input x1, // three inputs
output f // one output
);
wire [2:0]mid;
assign mid = {x3,x2,x1};
always@(*)
begin
case(mid)
3'b000,3'b001,3'b100,3'b110: f = 0;
3'b010,3'b011,3'b101,3'b111: f = 1;
default:;
endcase
end
endmodule
module top_module ( input [1:0] A, input [1:0] B, output z );
assign z = (A == B)?1:0;
endmodule
module top_module (input x, input y, output z);
assign z = (x^y) & x;
endmodule
module top_module ( input x, input y, output z );
assign z = ~(x ^ y);
endmodule
- Combine circuits A and B
task相关使用:https://www.cnblogs.com/lianjiehere/p/4018926.html,有代码使用task解决了此问题。
module top_module (input x, input y, output z);
wire z1,z2,z3,z4;
A IA1(.x(x), .y(y), .z(z1));
B IB1(.x(x), .y(y), .z(z2));
A IA2(.x(x), .y(y), .z(z3));
B IB2(.x(x), .y(y), .z(z4));
assign z = (z1 | z2) ^ (z3 & z4);
endmodule
module A (input x, input y, output z);
assign z = (x^y) & x;
endmodule
module B ( input x, input y, output z );
assign z = ~(x ^ y);
endmodule
module top_module (
input ring,
input vibrate_mode,
output ringer, // Make sound
output motor // Vibrate
);
assign ringer = ring & ~vibrate_mode;
assign motor = ring & vibrate_mode;
endmodule
module top_module (
input too_cold,
input too_hot,
input mode,
input fan_on,
output heater,
output aircon,
output fan
);
assign fan = fan_on | aircon | heater;
assign aircon = ~mode & too_hot;
assign heater = mode & too_cold;
endmodule
module top_module(
input [2:0] in,
output [1:0] out );
always @(*) begin
out = 2'b0;
for(integer i=0; i<3; i=i+1) begin //这里i<3,而不是8
if (in[i])
out = out + 1'b1;
else
out = out + 1'b0;
end
end
endmodule
module top_module(
input [3:0] in,
output [2:0] out_both,
output [3:1] out_any,
output [3:0] out_different );
always@(*) begin
for(integer i=0;i<3;i=i+1) begin
out_both[i] = in[i] & in[i+1];
out_any[i+1] = in[i+1] | in[i];
out_different[i] = in[i] ^ in[i+1];
end
out_different[3] = in[3] ^ in[0];
end
endmodule
module top_module(
input [99:0] in,
output [98:0] out_both,
output [99:1] out_any,
output [99:0] out_different );
always@(*) begin
for(integer i=0;i<99;i=i+1) begin
out_both[i] = in[i] & in[i+1];
out_any[i+1] = in[i+1] | in[i];
out_different[i] = in[i] ^ in[i+1];
end
out_different[99] = in[99] ^ in[0];
end
endmodule
3.1.2 Multiplexers
module top_module(
input a, b, sel,
output out );
assign out = sel?b:a;
endmodule
module top_module(
input [99:0] a, b,
input sel,
output [99:0] out );
assign out = sel?b[99:0]:a[99:0];
endmodule
module top_module(
input [15:0] a, b, c, d, e, f, g, h, i,
input [3:0] sel,
output [15:0] out );
always@ (*) begin
case(sel)
4'd0: out=a;
4'd1: out=b;
4'd2: out=c;
4'd3: out=d;
4'd4: out=e;
4'd5: out=f;
4'd6: out=g;
4'd7: out=h;
4'd8: out=i;
default: out=16'hffff;
endcase
end
endmodule
- 256-to-1 multiplexer //256-1mux
module top_module(
input [255:0] in,
input [7:0] sel,
output out );
assign out = in[sel];
endmodule
- 256-to-1 4-bit multiplexer
[ ]的常数表达:[base -: width]
module top_module(
input [1023:0] in,
input [7:0] sel,
output [3:0] out );
assign out = in[sel*4+3 -: 4];
// assign out = in[sel*4+3:sel*4];这一句是错误的,assign[必须是常数]
endmodule
截图来自百度:
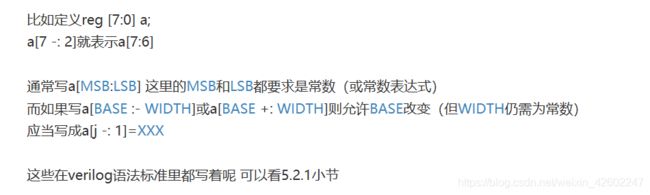
3.1.3 Arithmetic Circuits
module top_module(
input a, b,
output cout, sum );
assign {cout,sum} = a +b;
endmodule
module top_module(
input a, b, cin,
output cout, sum );
assign sum = a ^ b ^ cin;
assign cout = a & b | a & cin | cin & b;
endmodule
module top_module(
input [2:0] a, b,
input cin,
output [2:0] cout,
output [2:0] sum );
reg [2:0] Rcout,Rsum;
task fa;
input a,b,cin;
output reg Rcout,Rsum;
begin
Rcout = a & b| a&cin |b&cin;
Rsum = a ^ b ^ cin;
end
endtask
always@(*) begin
fa (a[0],b[0],cin,Rcout[0],Rsum[0]); //task输出必须是reg
fa (a[1],b[1],cout[0],Rcout[1],Rsum[1]);
fa (a[2],b[2],cout[1],Rcout[2],Rsum[2]);
sum = {Rsum[2],Rsum[1],Rsum[0]};
cout = {Rcout[2],Rcout[1],Rcout[0]};
end
endmodule
module top_module (
input [3:0] x,
input [3:0] y,
output [4:0] sum);
wire cin;
wire [3:0]cout;
assign cin = 1'b0;
assign sum[0] = x[0] ^ y[0] ^ cin;
assign cout[0] = x[0] & y[0];
always@(*) begin
for(integer i =1;i<4;i=i+1) begin
sum[i] = x[i] ^ y[i] ^ cout[i-1];
cout[i] = x[i] & y[i] | x[i] & cout[i-1] | y[i] & cout[i-1];
end
end
assign sum[4:0] = {cout[3],sum[3:0]};
endmodule
module top_module (
input [7:0] a,
input [7:0] b,
output [7:0] s,
output overflow
);
assign s = a + b;
assign overflow = ((a[7]==b[7])&(s[7]!=a[7]))?1'b1:1'b0;
//当最高位(符号位)和次高位carry项结果不同时,溢出
endmodule
module top_module(
input [99:0] a, b,
input cin,
output cout,
output [99:0] sum );
assign {cout,sum[99:0]} = a[99:0] + b[99:0] + cin;
endmodule
- 4-digit BCD adder
再说亿遍!always块里不可以例化block
module top_module(
input [15:0] a, b,
input cin,
output cout,
output [15:0] sum );
wire carry[15:3];
bcd_fadd a_0(.a(a[3:0]), .b(b[3:0]), .cin(cin), .cout(carry[3]), .sum(sum[3:0]));
generate
genvar i;
for(i=7;i<16;i=i+4) begin:GO
bcd_fadd a_i (.a(a[i-:4]), .b(b[i-:4]), .cin(carry[i-4]), .cout(carry[i]), .sum(sum[i-:4]));
end
endgenerate
assign cout = carry[15];
endmodule
3.1.4 Karnaugh Map to Circuit
- 3-variable
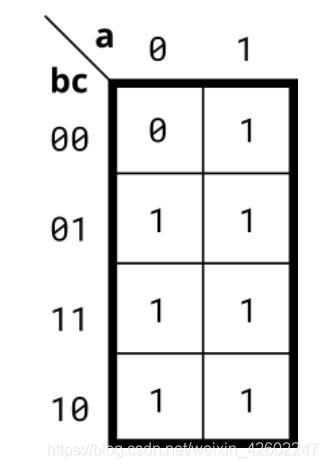
module top_module(
input a,
input b,
input c,
output out );
assign out = a | b | c;
endmodule
module top_module(
input a,
input b,
input c,
input d,
output out );
assign out = ~((a & c & ~d) | (a & b & ~c) | (~a & b & ~c & d) | (~a & ~b & c & d));
endmodule
module top_module(
input a,
input b,
input c,
input d,
output out );
assign out = a | (~a & ~b & c);
endmodule
module top_module(
input a,
input b,
input c,
input d,
output out );
assign out = (~c & ~d & (~a & b | a & ~b)) | (~c & d & (~a & ~b | a & b)) | (c & d & (~a & b | a & ~b)) | (c & ~d & (~a&~b | a&b));
endmodule
module top_module (
input a,
input b,
input c,
input d,
output out_sop,
output out_pos
);
assign out_sop = c & d | ~a & ~b & c & ~d;
assign out_pos = out_sop;
endmodule
module top_module (
input [4:1] x,
output f );
assign f = ~x[3] & x[1] & x[2] | ~x[1] & x[3];
endmodule
- Karnaugh map
SOP是1的情况或,POS是0的情况与。
module top_module (
input [4:1] x,
output f
);
assign f = (~x[1] & x[3]) | (~x[4] & x[1] & ~x[2]) | (x[1] & x[2] & x[3] & x[4]) | (~x[1] & ~x[2] & ~x[3] & ~x[4]);
endmodule
- K-map implemented with a multiplexer//用mux实现卡诺图
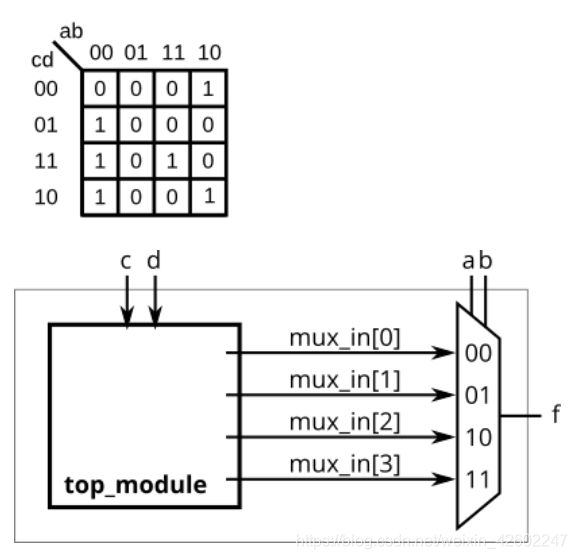
module top_module (
input c,
input d,
output [3:0] mux_in
);
assign mux_in[0] = c | d;
assign mux_in[1] = 1'b0;
assign mux_in[2] = ~c & ~d | c & ~d;
assign mux_in[3] = c & d;
endmodule