功能需求:
在winform中,每隔几秒就像服务器(局域网内)查看时候连接,如果没连接,则提示用户掉线。按钮功能enable=false
如果掉线后再上线了,则提示上线
有几个需要操作数据库的窗体,我统一建立一个父窗体,代码如下


1
using
System;
2 using System.Collections.Generic;
3 using System.ComponentModel;
4 using System.Data;
5 using System.Drawing;
6 using System.Text;
7 using System.Windows.Forms;
8 using System.IO;
9 using System.Net.NetworkInformation;
10 namespace DeviceInfo
11 {
12
13 ///
14 /// //数据库连接状态
15 ///
16 public enum ConState
17 {
18 ///
19 /// 在线
20 ///
21 OnLine = 0 ,
22 ///
23 /// 掉线
24 ///
25 Fall = 1 ,
26 }
27
28 ///
29 /// 是否提示掉线
30 ///
31 public enum PromptState
32 {
33 ///
34 /// 已提示
35 ///
36 Yes = 1 ,
37 ///
38 /// 未提示
39 ///
40 No = 0
41 }
42 ///
43 /// 是否提示在线
44 ///
45 public enum PromptOnLine
46 {
47 ///
48 /// 已提示
49 ///
50 Yes = 1 ,
51 ///
52 /// 未提示
53 ///
54 No = 0
55 }
56 public class CheckCon : Form
57 {
58 public static System.Windows.Forms.Timer BasicTimer1;
59 // 自定义的定时器事件!(基本单位: 100ms) 来判断数据库连接
60 public static event EventHandler BasicTimerTick1;
61 // 数据库连接状态
62 public static ConState conState;
63 // 是否提示掉线
64 public static PromptState promptState;
65 // 是否提示在线
66 public static PromptOnLine promptOnLine;
67
69 public static void Init()
70 {
71 // 初始化定时器
72 BasicTimer1 = new System.Windows.Forms.Timer();
73 BasicTimer1.Interval = GlobalDefine.TIMER_BASIC_PERIOD1;
74 BasicTimer1.Tick += new System.EventHandler(BasicTimer1_Tick);
75 BasicTimer1.Enabled = true ;
76
77 }
78 /// //
79 /// // 基本定时器!
80 /// //
81 private static void BasicTimer1_Tick( object sender, EventArgs e)
82 {
83 if (BasicTimerTick1 != null )
84 {
85 BasicTimerTick1(sender, EventArgs.Empty);
86
87 }
88 }
89 public CheckCon()
90 : base ()
91 {
92 }
93 protected override void OnLoad(EventArgs e)
94 {
95 base .OnLoad(e);
96 Init();
97 BasicTimerTick1 += new EventHandler(Timer1_Tick);
98 }
99
100
101 public static int NotConnectTime = 0 ;
102
103 public bool m_IsCon = false ;
104 ///
105 /// 判断是否连接
106 ///
107 ///
108 public static bool IsCon()
109 { // 判断是否连接
110 Ping pingSender = new Ping();
111 PingReply reply = null ;
112 reply = pingSender.Send(DataBase.key1, 1000 );
113 if (reply == null || (reply != null && reply.Status != IPStatus.Success))
114 {
115 if (NotConnectTime <= 10 )//如果连接的时候 十次都连接不上,则算掉线
116 {
117 NotConnectTime ++ ;
118
119 return true ;
120 }
121 else
122 {
123 return false ;
124 }
125 }
126 else
127 {
128 NotConnectTime = 0 ;
129 return true ;
130 }
131 }
132
133
134
135
protected
virtual
void
ButtonEnable()
136 {
137
138 }
139 // 判断数据库连接
140 protected void Timer1_Tick( object sender, EventArgs e)
141 {
142 ButtonEnable();
143 try
144 {
145 if ( ! IsCon())
146 {
147 if (promptState == PromptState.No)
148 {
149 promptState = PromptState.Yes; // 提示以后改变状态
150 promptOnLine = PromptOnLine.No;
151 conState = ConState.Fall; // 掉线
152
153 MessageBox.Show( " 数据库连接出错 " , " 数据库连接出错!请稍后再试。 " );
154
155 }
156
157 }
158 else
159 {
160 if (promptOnLine == PromptOnLine.No)
161 {
162 promptState = PromptState.No; // 提示以后改变状态
163 promptOnLine = PromptOnLine.Yes;
164 conState = ConState.OnLine; // 上线
165
166 MessageBox.Show( " 数据库连接成功 " , " 数据库连接成功! " );
167 }
168 }
169 }
170 catch (Exception)
171 {
172
173 }
174 }
175
176
177 }
178 }
179
2 using System.Collections.Generic;
3 using System.ComponentModel;
4 using System.Data;
5 using System.Drawing;
6 using System.Text;
7 using System.Windows.Forms;
8 using System.IO;
9 using System.Net.NetworkInformation;
10 namespace DeviceInfo
11 {
12
13 ///
14 /// //数据库连接状态
15 ///
16 public enum ConState
17 {
18 ///
19 /// 在线
20 ///
21 OnLine = 0 ,
22 ///
23 /// 掉线
24 ///
25 Fall = 1 ,
26 }
27
28 ///
29 /// 是否提示掉线
30 ///
31 public enum PromptState
32 {
33 ///
34 /// 已提示
35 ///
36 Yes = 1 ,
37 ///
38 /// 未提示
39 ///
40 No = 0
41 }
42 ///
43 /// 是否提示在线
44 ///
45 public enum PromptOnLine
46 {
47 ///
48 /// 已提示
49 ///
50 Yes = 1 ,
51 ///
52 /// 未提示
53 ///
54 No = 0
55 }
56 public class CheckCon : Form
57 {
58 public static System.Windows.Forms.Timer BasicTimer1;
59 // 自定义的定时器事件!(基本单位: 100ms) 来判断数据库连接
60 public static event EventHandler BasicTimerTick1;
61 // 数据库连接状态
62 public static ConState conState;
63 // 是否提示掉线
64 public static PromptState promptState;
65 // 是否提示在线
66 public static PromptOnLine promptOnLine;
67
69 public static void Init()
70 {
71 // 初始化定时器
72 BasicTimer1 = new System.Windows.Forms.Timer();
73 BasicTimer1.Interval = GlobalDefine.TIMER_BASIC_PERIOD1;
74 BasicTimer1.Tick += new System.EventHandler(BasicTimer1_Tick);
75 BasicTimer1.Enabled = true ;
76
77 }
78 /// //
79 /// // 基本定时器!
80 /// //
81 private static void BasicTimer1_Tick( object sender, EventArgs e)
82 {
83 if (BasicTimerTick1 != null )
84 {
85 BasicTimerTick1(sender, EventArgs.Empty);
86
87 }
88 }
89 public CheckCon()
90 : base ()
91 {
92 }
93 protected override void OnLoad(EventArgs e)
94 {
95 base .OnLoad(e);
96 Init();
97 BasicTimerTick1 += new EventHandler(Timer1_Tick);
98 }
99
100
101 public static int NotConnectTime = 0 ;
102
103 public bool m_IsCon = false ;
104 ///
105 /// 判断是否连接
106 ///
107 ///
108 public static bool IsCon()
109 { // 判断是否连接
110 Ping pingSender = new Ping();
111 PingReply reply = null ;
112 reply = pingSender.Send(DataBase.key1, 1000 );
113 if (reply == null || (reply != null && reply.Status != IPStatus.Success))
114 {
115 if (NotConnectTime <= 10 )//如果连接的时候 十次都连接不上,则算掉线
116 {
117 NotConnectTime ++ ;
118
119 return true ;
120 }
121 else
122 {
123 return false ;
124 }
125 }
126 else
127 {
128 NotConnectTime = 0 ;
129 return true ;
130 }
131 }
132
133
134
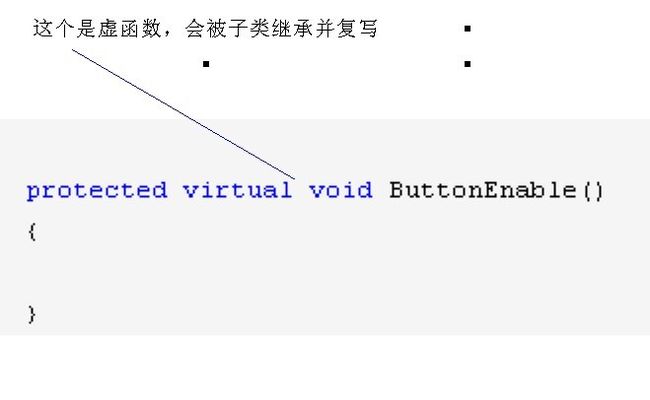
136 {
137
138 }
139 // 判断数据库连接
140 protected void Timer1_Tick( object sender, EventArgs e)
141 {
142 ButtonEnable();
143 try
144 {
145 if ( ! IsCon())
146 {
147 if (promptState == PromptState.No)
148 {
149 promptState = PromptState.Yes; // 提示以后改变状态
150 promptOnLine = PromptOnLine.No;
151 conState = ConState.Fall; // 掉线
152
153 MessageBox.Show( " 数据库连接出错 " , " 数据库连接出错!请稍后再试。 " );
154
155 }
156
157 }
158 else
159 {
160 if (promptOnLine == PromptOnLine.No)
161 {
162 promptState = PromptState.No; // 提示以后改变状态
163 promptOnLine = PromptOnLine.Yes;
164 conState = ConState.OnLine; // 上线
165
166 MessageBox.Show( " 数据库连接成功 " , " 数据库连接成功! " );
167 }
168 }
169 }
170 catch (Exception)
171 {
172
173 }
174 }
175
176
177 }
178 }
179
在需要继承这个窗口的窗体中,加入代码:


1
#region
类构造函数
2 public Form1()
3 {
4 InitializeComponent();
5
6 ButtonBind(); // 因为我无法马上就执行这个,所以先这样写着
7 }
8 #endregion
9 protected void ButtonBind()
10 {
11 if (conState == ConState.Fall)
12 {
13 BarCode.Enabled = false ;
14 BtnOK.Enabled = false ;
15 }
16 else
17 {
18 BarCode.Enabled = true ;
19 BtnOK.Enabled = true ;
20 }
21 }
22 protected override void ButtonEnable()
23 {
24 ButtonBind();
25 }
26
27
2 public Form1()
3 {
4 InitializeComponent();
5
6 ButtonBind(); // 因为我无法马上就执行这个,所以先这样写着
7 }
8 #endregion
9 protected void ButtonBind()
10 {
11 if (conState == ConState.Fall)
12 {
13 BarCode.Enabled = false ;
14 BtnOK.Enabled = false ;
15 }
16 else
17 {
18 BarCode.Enabled = true ;
19 BtnOK.Enabled = true ;
20 }
21 }
22 protected override void ButtonEnable()
23 {
24 ButtonBind();
25 }
26
27
在掉线后再上线,连接数据库的时候会出问题,很奇怪的BUG,我的解决方案是将con重新new一下。。很奇怪的bug,不晓得什么原因。。
try
{
m_SqlConn = new SqlConnection(sqlConnectionDeviceCmd); m_SqlConn.Open();
}
catch (System.Data.SqlClient.SqlException E1)
{
System.Windows.Forms.MessageBox.Show(E1.Message);
return null;
}