- Python 爬虫实战:DOTA2 比赛数据全量采集(含赛事战报解析与数据库存储
西攻城狮北
python爬虫数据库
一、引言DOTA2作为一款全球知名的多人在线战术竞技游戏,拥有庞大的玩家群体和丰富的比赛数据。这些数据对于电竞分析师、数据研究员、游戏玩家等具有极高的价值。通过爬取DOTA2比赛数据,可以深入了解比赛详情、战队表现、选手数据等信息,为电竞行业提供数据支持。二、开发环境搭建(一)编程语言与工具选择选择Python语言,利用其丰富的库和简洁语法,高效完成爬虫开发任务。搭配PyCharm集成开发环境,享
- Python 爬虫实战:淘宝直播间实时数据抓取(弹幕分析 + 流量监控)
西攻城狮北
python爬虫开发语言
一、引言随着电商直播的迅猛发展,淘宝直播已成为品牌推广和商品销售的重要阵地。通过爬取淘宝直播间的实时数据,包括弹幕互动和流量信息,可以帮助商家深入了解用户行为、优化直播策略,同时为市场分析和商业决策提供数据支持。本文将深入探讨如何利用Python爬虫技术实现对淘宝直播间实时数据的抓取,并进行弹幕分析和流量监控。二、项目背景与目标2.1项目背景淘宝直播作为电商领域的重要流量入口,通过实时视频与用户互
- Python类的基础与高级用法详解
在Python中,类(Class)是面向对象编程(OOP)的核心概念,用于创建对象的蓝图。它定义了对象的属性和行为,支持代码复用、封装、继承和多态。接下来,从多个维度详细解释类的核心概念:一、类的基本结构classMyClass: #类变量(所有实例共享) class_variable="SharedData" #构造方法(初始化实例属性) def__init__(self,name):
- Python训练营-Day41
m0_72314023
python深度学习神经网络
#原始模型(2层卷积)classOriginalCNN(nn.Module):def__init__(self):super().__init__()self.conv1=nn.Conv2d(1,16,3)self.conv2=nn.Conv2d(16,32,3)self.fc=nn.Linear(32*5*5,10)defforward(self,x):x=torch.relu(self.con
- Python训练营-Day40
m0_72314023
python开发语言
importtorchimporttorch.nnasnnimporttorch.optimasoptimfromsklearn.model_selectionimporttrain_test_splitfromsklearn.preprocessingimportMinMaxScalerimporttimeimportmatplotlib.pyplotaspltfromtqdmimporttqd
- Python训练营-Day18
importpandasaspdimportpandasaspdimportnumpyasnpimportmatplotlib.pyplotaspltimportseabornassnsimportwarningswarnings.filterwarnings("ignore")plt.rcParams['font.sans-serif']=['SimHei']plt.rcParams['axes
- Python训练营-Day20
importpandasaspdimportpandasaspdimportnumpyasnpimportmatplotlib.pyplotaspltimportseabornassnsimportwarningswarnings.filterwarnings("ignore")plt.rcParams['font.sans-serif']=['SimHei']plt.rcParams['axes
- Python训练营-Day11
m0_72314023
Python训练营python机器学习深度学习
DAY11常见的调参方式超参数调整专题1知识点回顾1.网格搜索2.随机搜索(简单介绍,非重点实战中很少用到,可以不了解)3.贝叶斯优化(2种实现逻辑,以及如何避开必须用交叉验证的问题)4.time库的计时模块,方便后人查看代码运行时长#LightGBM-网格优化print("\n---3.网格搜索优化LightGBM(训练集->测试集)---")importlightgbmaslgbfromskl
- Python训练营-Day3
DAY3列表、循环和判断语句题目1:列表的基础操作题目:1.创建一个包含三个字符串元素的列表tech_list,元素分别为“Python”,“Java”,“Go”。2.获取列表中的第一个元素,并将其存储在变量first_tech中。3.向tech_list的末尾添加一个新的字符串元素“JavaScript”。4.修改tech_list中的第二个元素(索引为1),将其从“Java”更改为“Ruby”
- python+uniapp基于微信小程序的河湟文化宣传系统nodejs+java
文章目录具体实现截图本项目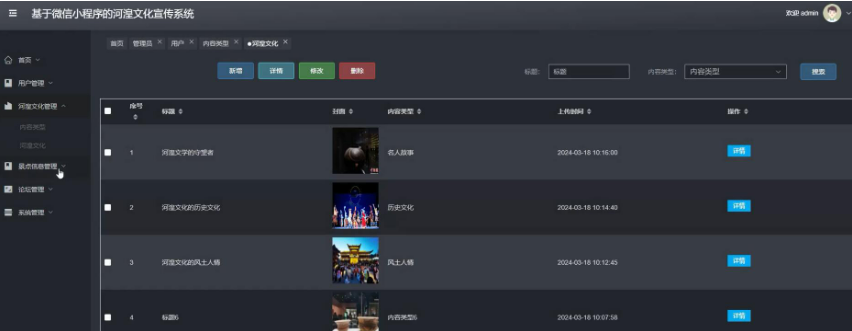源码获取详细视频演示:文章底部获取博主联系方式!!!!本系统开发思路进度安排及各阶段主要任务java类核心代码部分展示主要参考文献:源码获取/详细视频演示##项目介绍摘要随着互联网技术的飞速发展和移动互联网的
- python之海象运算符
youhebuke225
pythonpython
简介海象运算符是一种语法糖,有一个:和一个=构成,语法格式如下:(variable_name:=expression)一般海象运算符有三种用法,如下ifelseifelse中还是比较常用的#if语句中a=10ifa>5:print("hello")ifa:=10>5:print("hello:=")打印hellohello:=他会先进行赋值,然后再进行比较while一般使用while我们会进行无限
- 用Python一键生成PNG图片的PowerPoint幻灯片
在当今的商业环境中,PowerPoint演示是展示和传递信息的常用方式。然而,手动将大量图像插入到幻灯片中往往是一项乏味且耗时的工作。但是,通过Python编程,我们可以轻松自动化这个过程,节省时间和精力。C:\pythoncode\new\folderTOppt.py在本文中,我将介绍如何使用Python、wxPython和python-pptx库编写一个脚本,将指定文件夹中的所有PNG图像逐一
- Python与Dlib库实现人脸技术实战
西域情歌
本文还有配套的精品资源,点击获取简介:本项目详细说明了如何使用Python结合Dlib库实现人脸检测、识别、数量检测和距离检测。利用Dlib提供的机器学习算法和计算机视觉功能,包括HOG特征检测、级联分类器、面部特征向量模型和关键点预测等,项目能够快速准确地在图像中检测和识别人脸。此外,还介绍了如何统计图像中的人脸数量以及如何计算人脸之间的距离。通过实际代码资源,开发者能够掌握实时人脸技术的应用,
- python 海象运算符_python := 海象运算符
伶邪
python海象运算符
最近在做算法题越来越发现python写法真的挺好用的记下来map(lambdax:sum(x))中lambda代表匿名函数re.findall(r'0+|1+',s)是正则表达式:=海象运算符转if(n:=len(a))>10:print(f"Lististoolong({n}elements,expected10:print(f"Lististolong({len(a)}elements,exp
- 零基础学python张志强pdf_零基础学Python
weixin_39707725
前言第一篇Python语言基础第1章进入Python的世界1.1Python的由来1.2Python的特色1.3第一个Python程序1.4搭建开发环境1.4.1Python的下载和安装1.4.2交互式命令行的使用1.5Python的开发工具1.5.1PyCharm的使用1.5.2EclipseIDE的介绍1.5.3EditPlus编辑器环境的配置1.6不同平台下的Python1.7小结1.8习题
- python := 海象运算符
challenge-linge
itit
参考视频教程:**体系课-Go+Python双语言混合开发盯紧技术先机抓紧高薪机遇**最近在做算法题越来越发现python写法真的挺好用的记下来map(lambdax:sum(x))中lambda代表匿名函数re.findall(r’0+|1+’,s)是正则表达式:=海象运算符转背景:python3.8正式版最近更新了,其中PEP572中的海象运算符获得正式python版本的支持.我看了官网的文档
- Python 海象运算符 详细介绍
ys.journey
Pythonpython
海象运算符定义:一个变量名跟一个表达式或者一个值,这个是一种新的赋值运算符。下面看看它的三种用法:一、用于ifelse条件表达式基础写法:x=5ifx10]print(num2)运行结果:海象运算符写法:num1=[1,2,3,4,5]count=1deff(x):globalcountprint(f"f(x)函数运行了{count}次")count+=1returnx**2num2=[nforx
- 【Python】已解决:Traceback (most recent call last): File “C:/python/kfc.py”, line 8, in KfcError: KFC Cra
屿小夏
pythonc语言开发语言
个人简介:某不知名博主,致力于全栈领域的优质博客分享|用最优质的内容带来最舒适的阅读体验!文末获取免费IT学习资料!文末获取更多信息精彩专栏推荐订阅收藏专栏系列直达链接相关介绍书籍分享点我跳转书籍作为获取知识的重要途径,对于IT从业者来说更是不可或缺的资源。不定期更新IT图书,并在评论区抽取随机粉丝,书籍免费包邮到家AI前沿点我跳转探讨人工智能技术领域的最新发展和创新,涵盖机器学习、深度学习、自然
- Python, C++开发社会工作人员学习手册APP
Geeker-2025
pythonc++
#社会工作人员学习手册APP设计方案##系统架构设计```移动端(Flutter/ReactNative)|RESTAPI/gRPC|Go核心服务(Gin/Echo)←───PythonAI服务(FastAPI)|(学习路径规划/智能问答)|Rust高性能模块(数据处理/安全)|PostgreSQL(知识库+用户数据)|Redis(缓存+实时协作)|MinIO(学习资源存储)```##技术分工与优
- Python 开发法律条文咨询APP
Geeker-2025
python
#法律条文咨询APP-Python实现方案我将设计一个基于Python的法律条文咨询应用,提供一个直观的界面让用户轻松查找和浏览法律条文。##设计思路-使用Flask作为后端框架,轻量且高效-SQLite数据库存储法律条文数据-前端使用Bootstrap实现响应式设计-实现关键词搜索和分类浏览功能-提供条文详情展示和书签功能##完整实现代码###文件结构```legal_app/├──app.py
- Python,Go 开发税务CRS 解读概况与实操案例APP
Geeker-2025
pythongolang
以下为基于**Python**与**Go**开发“税务CRS(共同申报准则)系统概况与实操案例APP”的技术方案与实施路径,综合数据处理、合规性保障及高并发需求设计:---###⚙️**一、技术架构与模块分工**|**语言**|**核心模块**|**技术选型与优势**|**应用场景**||-----------|----------------------------|--------------
- Python,C++开发社会游戏规则透视与个人发展实操APP
Geeker-2025
pythonc++
开发一款**社会游戏规则透视与个人发展实操APP**是一个非常有意义的项目,旨在通过数字化手段帮助用户理解社会规则、提升个人能力,并提供实操指导以促进个人发展。该APP可以包括社会规则解析、个人能力评估、发展路径规划、实操指导、社区互动等功能模块。以下是基于Python和C++开发社会游戏规则透视与个人发展实操APP的详细方案。---##**1.功能模块设计**###**1.1社会规则透视**-*
- python : 海象运算符 :=
愚戏师
python基础与机器学习python前端
海象运算符:=在Python3.8中引入的海象运算符(:=,WalrusOperator)是一个语法特性,允许在表达式内部进行变量赋值。它得名于符号:=形似海象的眼睛和獠牙。这一特性旨在简化代码,减少重复计算,同时提升可读性。核心概念语法:变量:=表达式作用:将表达式的值赋给变量,并返回该值。特点:在条件判断、循环、推导式等场景中直接使用赋值结果。避免重复计算同一表达式,提升代码效率。典型使用场景
- python中报错Traceback (most recent call last): File “<stdin>“, line 1, in <module>TypeError: ‘str‘ obj
bk小兔子乖乖
pythonjava前端
>>>len='hello'>>>len('hello')如果运行该代码则会报错:Traceback(mostrecentcalllast):File"",line1,inTypeError:'str'objectisnotcallable出现该错误的原因是在python中有len()该函数,但是由于我们不小心定义了该函数,则会覆盖原来的len函数,此时不论我们怎么使用len函数,都会出现报错,解
- Python, Go 开发全国经济开发区政策查询与实操APP
以下是基于Python和Go开发的全国经济开发区政策查询与实操APP设计方案,结合最新政策动态与技术优势,助力企业精准把握政策红利:---###系统架构设计```移动端/Web端(Flutter/React)|RESTAPI/gRPC|Go核心服务(Gin/Echo)←───Python智能引擎(FastAPI)|(政策匹配/实操分析)PostgreSQL(政策库+企业画像)|Redis(实时缓存
- 内网和外网可以共享一台打印机吗?怎么设置实现跨网电脑远程连接打印
搬码临时工
网络
内网和外网可以通过特定技术手段实现共享一台打印机。实现方式主要包括物理切换器、网络分段映射(如路由设置)、类似nat123内网穿透等技术方案,但需根据网络环境安全等级选择合适方案,并注意数据隔离要求。一、物理切换器方案实现内外网共离同一打印机使用USB打印机共享器或网络切换器,通过物理按钮切换内外网连接。这类设备可实现电路层面的隔离,避免数据泄露风险。例如:采用二进一出的USB切换器连接内
- Ollama实践之:Python代码生成与执行
小村学长毕业设计
python开发语言
Ollama实践之:Python代码生成与执行在人工智能领域,生成式模型正逐渐展现出其强大的潜力。Ollama,作为一个先进的生成式语言模型,不仅能生成连贯的文本,还能生成代码片段,并在某些情况下,生成可执行的代码。本文将详细探讨如何使用Ollama生成Python代码,以及执行这些代码的实践过程。我们不仅会讨论技术细节,还会探讨其应用场景、潜在风险以及未来的发展趋势。一、Ollama简介Olla
- 探索未来科技:Ollama Python 库——Python 与 AI 的无缝对接
尚绮令Imogen
探索未来科技:OllamaPython库——Python与AI的无缝对接项目地址:https://gitcode.com/gh_mirrors/ol/ollama-python在人工智能领域,Python是无可争议的首选语言。而OllamaPythonLibrary正是为了让开发者更加便捷地将Python3.8及以上版本项目与Ollama平台集成,从而解锁更强大的AI功能。这个库以其直观的API设
- HTML表格导出为Excel文件的实现方案
~风清扬~
前端技术htmlexcel前端
1、前端javascript可通过mime类型、blob对象或专业库(如sheetjs)实现html表格导出excel,适用于中小型数据量;2、服务器端方案利用后端语言(如python的openpyxl、java的apachepoi)处理复杂报表和大数据,确保安全性与格式控制;3、常见问题包括数据类型识别错误、样式丢失、大文件卡顿、浏览器兼容性及乱码,需通过设置单元格类型、使用后端样式api、分页
- Ollama-python:调用大模型服务实现代码自动补全,提升编程开发效率!
Ollama是一个优秀的本地部署与管理大模型的框架。通过Ollama,我们可以在本地部署、定制自己的大模型服务。大模型部署在本地后,我们可以有哪些应用呢?本文介绍如何通过Ollama的pythonsdk,调用本地部署的大模型服务,对我们的代码进行自动补全,提升日常的编程开发效率。安装Ollama及其pythonsdk在https://ollama.com/download下载Ollama安装程序并
- 关于旗正规则引擎下载页面需要弹窗保存到本地目录的问题
何必如此
jsp超链接文件下载窗口
生成下载页面是需要选择“录入提交页面”,生成之后默认的下载页面<a>标签超链接为:<a href="<%=root_stimage%>stimage/image.jsp?filename=<%=strfile234%>&attachname=<%=java.net.URLEncoder.encode(file234filesourc
- 【Spark九十八】Standalone Cluster Mode下的资源调度源代码分析
bit1129
cluster
在分析源代码之前,首先对Standalone Cluster Mode的资源调度有一个基本的认识:
首先,运行一个Application需要Driver进程和一组Executor进程。在Standalone Cluster Mode下,Driver和Executor都是在Master的监护下给Worker发消息创建(Driver进程和Executor进程都需要分配内存和CPU,这就需要Maste
- linux上独立安装部署spark
daizj
linux安装spark1.4部署
下面讲一下linux上安装spark,以 Standalone Mode 安装
1)首先安装JDK
下载JDK:jdk-7u79-linux-x64.tar.gz ,版本是1.7以上都行,解压 tar -zxvf jdk-7u79-linux-x64.tar.gz
然后配置 ~/.bashrc&nb
- Java 字节码之解析一
周凡杨
java字节码javap
一: Java 字节代码的组织形式
类文件 {
OxCAFEBABE ,小版本号,大版本号,常量池大小,常量池数组,访问控制标记,当前类信息,父类信息,实现的接口个数,实现的接口信息数组,域个数,域信息数组,方法个数,方法信息数组,属性个数,属性信息数组
}
&nbs
- java各种小工具代码
g21121
java
1.数组转换成List
import java.util.Arrays;
Arrays.asList(Object[] obj); 2.判断一个String型是否有值
import org.springframework.util.StringUtils;
if (StringUtils.hasText(str)) 3.判断一个List是否有值
import org.spring
- 加快FineReport报表设计的几个心得体会
老A不折腾
finereport
一、从远程服务器大批量取数进行表样设计时,最好按“列顺序”取一个“空的SQL语句”,这样可提高设计速度。否则每次设计时模板均要从远程读取数据,速度相当慢!!
二、找一个富文本编辑软件(如NOTEPAD+)编辑SQL语句,这样会很好地检查语法。有时候带参数较多检查语法复杂时,结合FineReport中生成的日志,再找一个第三方数据库访问软件(如PL/SQL)进行数据检索,可以很快定位语法错误。
- mysql linux启动与停止
墙头上一根草
如何启动/停止/重启MySQL一、启动方式1、使用 service 启动:service mysqld start2、使用 mysqld 脚本启动:/etc/inint.d/mysqld start3、使用 safe_mysqld 启动:safe_mysqld&二、停止1、使用 service 启动:service mysqld stop2、使用 mysqld 脚本启动:/etc/inin
- Spring中事务管理浅谈
aijuans
spring事务管理
Spring中事务管理浅谈
By Tony Jiang@2012-1-20 Spring中对事务的声明式管理
拿一个XML举例
[html]
view plain
copy
print
?
<?xml version="1.0" encoding="UTF-8"?>&nb
- php中隐形字符65279(utf-8的BOM头)问题
alxw4616
php中隐形字符65279(utf-8的BOM头)问题
今天遇到一个问题. php输出JSON 前端在解析时发生问题:parsererror.
调试:
1.仔细对比字符串发现字符串拼写正确.怀疑是 非打印字符的问题.
2.逐一将字符串还原为unicode编码. 发现在字符串头的位置出现了一个 65279的非打印字符.
 
- 调用对象是否需要传递对象(初学者一定要注意这个问题)
百合不是茶
对象的传递与调用技巧
类和对象的简单的复习,在做项目的过程中有时候不知道怎样来调用类创建的对象,简单的几个类可以看清楚,一般在项目中创建十几个类往往就不知道怎么来看
为了以后能够看清楚,现在来回顾一下类和对象的创建,对象的调用和传递(前面写过一篇)
类和对象的基础概念:
JAVA中万事万物都是类 类有字段(属性),方法,嵌套类和嵌套接
- JDK1.5 AtomicLong实例
bijian1013
javathreadjava多线程AtomicLong
JDK1.5 AtomicLong实例
类 AtomicLong
可以用原子方式更新的 long 值。有关原子变量属性的描述,请参阅 java.util.concurrent.atomic 包规范。AtomicLong 可用在应用程序中(如以原子方式增加的序列号),并且不能用于替换 Long。但是,此类确实扩展了 Number,允许那些处理基于数字类的工具和实用工具进行统一访问。
 
- 自定义的RPC的Java实现
bijian1013
javarpc
网上看到纯java实现的RPC,很不错。
RPC的全名Remote Process Call,即远程过程调用。使用RPC,可以像使用本地的程序一样使用远程服务器上的程序。下面是一个简单的RPC 调用实例,从中可以看到RPC如何
- 【RPC框架Hessian一】Hessian RPC Hello World
bit1129
Hello world
什么是Hessian
The Hessian binary web service protocol makes web services usable without requiring a large framework, and without learning yet another alphabet soup of protocols. Because it is a binary p
- 【Spark九十五】Spark Shell操作Spark SQL
bit1129
shell
在Spark Shell上,通过创建HiveContext可以直接进行Hive操作
1. 操作Hive中已存在的表
[hadoop@hadoop bin]$ ./spark-shell
Spark assembly has been built with Hive, including Datanucleus jars on classpath
Welcom
- F5 往header加入客户端的ip
ronin47
when HTTP_RESPONSE {if {[HTTP::is_redirect]}{ HTTP::header replace Location [string map {:port/ /} [HTTP::header value Location]]HTTP::header replace Lo
- java-61-在数组中,数字减去它右边(注意是右边)的数字得到一个数对之差. 求所有数对之差的最大值。例如在数组{2, 4, 1, 16, 7, 5,
bylijinnan
java
思路来自:
http://zhedahht.blog.163.com/blog/static/2541117420116135376632/
写了个java版的
public class GreatestLeftRightDiff {
/**
* Q61.在数组中,数字减去它右边(注意是右边)的数字得到一个数对之差。
* 求所有数对之差的最大值。例如在数组
- mongoDB 索引
开窍的石头
mongoDB索引
在这一节中我们讲讲在mongo中如何创建索引
得到当前查询的索引信息
db.user.find(_id:12).explain();
cursor: basicCoursor 指的是没有索引
&
- [硬件和系统]迎峰度夏
comsci
系统
从这几天的气温来看,今年夏天的高温天气可能会维持在一个比较长的时间内
所以,从现在开始准备渡过炎热的夏天。。。。
每间房屋要有一个落地电风扇,一个空调(空调的功率和房间的面积有密切的关系)
坐的,躺的地方要有凉垫,床上要有凉席
电脑的机箱
- 基于ThinkPHP开发的公司官网
cuiyadll
行业系统
后端基于ThinkPHP,前端基于jQuery和BootstrapCo.MZ 企业系统
轻量级企业网站管理系统
运行环境:PHP5.3+, MySQL5.0
系统预览
系统下载:http://www.tecmz.com
预览地址:http://co.tecmz.com
各种设备自适应
响应式的网站设计能够对用户产生友好度,并且对于
- Transaction and redelivery in JMS (JMS的事务和失败消息重发机制)
darrenzhu
jms事务承认MQacknowledge
JMS Message Delivery Reliability and Acknowledgement Patterns
http://wso2.com/library/articles/2013/01/jms-message-delivery-reliability-acknowledgement-patterns/
Transaction and redelivery in
- Centos添加硬盘完全教程
dcj3sjt126com
linuxcentoshardware
Linux的硬盘识别:
sda 表示第1块SCSI硬盘
hda 表示第1块IDE硬盘
scd0 表示第1个USB光驱
一般使用“fdisk -l”命
- yii2 restful web服务路由
dcj3sjt126com
PHPyii2
路由
随着资源和控制器类准备,您可以使用URL如 http://localhost/index.php?r=user/create访问资源,类似于你可以用正常的Web应用程序做法。
在实践中,你通常要用美观的URL并采取有优势的HTTP动词。 例如,请求POST /users意味着访问user/create动作。 这可以很容易地通过配置urlManager应用程序组件来完成 如下所示
- MongoDB查询(4)——游标和分页[八]
eksliang
mongodbMongoDB游标MongoDB深分页
转载请出自出处:http://eksliang.iteye.com/blog/2177567 一、游标
数据库使用游标返回find的执行结果。客户端对游标的实现通常能够对最终结果进行有效控制,从shell中定义一个游标非常简单,就是将查询结果分配给一个变量(用var声明的变量就是局部变量),便创建了一个游标,如下所示:
> var
- Activity的四种启动模式和onNewIntent()
gundumw100
android
Android中Activity启动模式详解
在Android中每个界面都是一个Activity,切换界面操作其实是多个不同Activity之间的实例化操作。在Android中Activity的启动模式决定了Activity的启动运行方式。
Android总Activity的启动模式分为四种:
Activity启动模式设置:
<acti
- 攻城狮送女友的CSS3生日蛋糕
ini
htmlWebhtml5csscss3
在线预览:http://keleyi.com/keleyi/phtml/html5/29.htm
代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>攻城狮送女友的CSS3生日蛋糕-柯乐义<
- 读源码学Servlet(1)GenericServlet 源码分析
jzinfo
tomcatWebservlet网络应用网络协议
Servlet API的核心就是javax.servlet.Servlet接口,所有的Servlet 类(抽象的或者自己写的)都必须实现这个接口。在Servlet接口中定义了5个方法,其中有3个方法是由Servlet 容器在Servlet的生命周期的不同阶段来调用的特定方法。
先看javax.servlet.servlet接口源码:
package
- JAVA进阶:VO(DTO)与PO(DAO)之间的转换
snoopy7713
javaVOHibernatepo
PO即 Persistence Object VO即 Value Object
VO和PO的主要区别在于: VO是独立的Java Object。 PO是由Hibernate纳入其实体容器(Entity Map)的对象,它代表了与数据库中某条记录对应的Hibernate实体,PO的变化在事务提交时将反应到实际数据库中。
实际上,这个VO被用作Data Transfer
- mongodb group by date 聚合查询日期 统计每天数据(信息量)
qiaolevip
每天进步一点点学习永无止境mongodb纵观千象
/* 1 */
{
"_id" : ObjectId("557ac1e2153c43c320393d9d"),
"msgType" : "text",
"sendTime" : ISODate("2015-06-12T11:26:26.000Z")
- java之18天 常用的类(一)
Luob.
MathDateSystemRuntimeRundom
System类
import java.util.Properties;
/**
* System:
* out:标准输出,默认是控制台
* in:标准输入,默认是键盘
*
* 描述系统的一些信息
* 获取系统的属性信息:Properties getProperties();
*
*
*
*/
public class Sy
- maven
wuai
maven
1、安装maven:解压缩、添加M2_HOME、添加环境变量path
2、创建maven_home文件夹,创建项目mvn_ch01,在其下面建立src、pom.xml,在src下面简历main、test、main下面建立java文件夹
3、编写类,在java文件夹下面依照类的包逐层创建文件夹,将此类放入最后一级文件夹
4、进入mvn_ch01
4.1、mvn compile ,执行后会在