源代码
#include "stdafx.h"
#include"stdafx.h"
#include
#include
#include
#include
#include
#include
using namespace std;
void DDALine(int p0_x, int p0_y, int p1_x, int p1_y, COLORREF color){
int dx = p1_x - p0_x, dy = p1_y - p0_y, steps, k;
float xIncrement, yIncrement, xp = p0_x, yp = p0_y;
color = YELLOW;
if (abs(dx) > abs(dy))
steps = abs(dx);
else
steps = abs(dy);
xIncrement = ((float)dx) / steps;
yIncrement = ((float)dy) / steps;
putpixel((int)(xp + 0.5), (int)(yp + 0.5), color);
for (k = 0; k < steps; k++){
xp += xIncrement;
yp += yIncrement;
putpixel((int)(xp + 0.5), (int)(yp + 0.5), color);
}
}
void MidpointCircle(int x0, int y0, int r, int color)
{
int x = 0, y = r;
float d = 5.0 / 4 - r;
color = GREEN;
while (x <= y) {
putpixel(x0 + x, y0 + y, color);
putpixel(x0 + x, y0 - y, color);
putpixel(x0 - x, y0 + y, color);
putpixel(x0 - x, y0 - y, color);
putpixel(x0 + y, y0 + x, color);
putpixel(x0 + y, y0 - x, color);
putpixel(x0 - y, y0 + x, color);
putpixel(x0 - y, y0 - x, color);
if (d<0)
d += x*2.0 + 3;
else {
d += 2.0*(x - y) + 5; y--;
}
x++;
}
}
void elliosePlotPoints(int xCenter, int yCenter, int x, int y, COLORREF color){
putpixel(xCenter + x, yCenter + y, color);
putpixel(xCenter - x, yCenter + y, color);
putpixel(xCenter + x, yCenter - y, color);
putpixel(xCenter - x, yCenter - y, color);
}
void ellipseMidpoint(int xCenter, int yCenter, int rx, int ry, COLORREF color){
int rx2 = rx*rx, ry2 = ry*ry;
int tworx2 = 2 * rx2, twory2 = 2 * ry2;
int p, x = 0, y = ry, px = 0, py = tworx2*y;
elliosePlotPoints(xCenter, yCenter, x, y, color);
p = (int)((ry2 - (rx2*ry) + 0.25*rx2) + 0.5);
while (px < py){
x++;
px += twory2;
if (p < 0)p += ry2 + px;
else{
y--;
py -= tworx2;
p += ry2 + px - py;
}
elliosePlotPoints(xCenter, yCenter, x, y, color);
}
p = (int)((ry2*(x + 0.5)*(x + 0.5) + rx2*(y - 1)*(y - 1) - rx2*ry2) + 0.5);
while (y>0){
y--;
py -= tworx2;
if (p > 0)p += rx2 - py;
else{
x++;
px += twory2;
p += rx2 - py + px;
}
elliosePlotPoints(xCenter, yCenter, x, y, color);
}
}
void main()
{
int x0, y0, x1, y1;
initgraph(640, 480);
DDALine(0, 0, 200, 200, 255);
MidpointCircle(100, 100, 90, 255);
ellipseMidpoint(200, 200, 180, 50, 255);
_getch();
closegraph();
}
测试结果
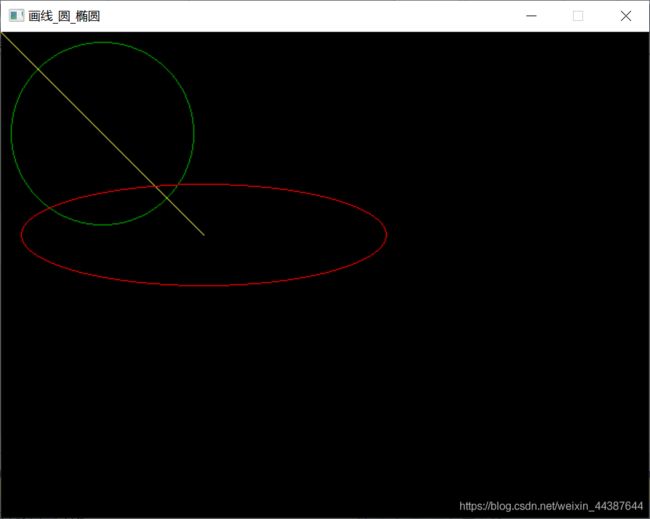