一直在学习关注大访问量网站的缓存是如何实现,之前看过Memcached的资料,忙于没有时间来真正测试一下,今天测试下分布式缓存Memcached
首先要在缓存服务器上安装安装:memcached(1.2.6 for Win32)
测试程序部署到本地环境,开发工具VS2008 .NET3.5
使用到memcached 1.2.6 for Win32下载地址:
memcached-1.2.6-win32-bin.zip
好了,下面我们按步骤来测试:
第一、首先到把下载好的memcached 1.2.6解压到C:\memcached目录,分别复制到两台服务器中。
第二、安装memcached服务,在命令提示符输入CD c:\memcached进入到memcached目录,如下图:
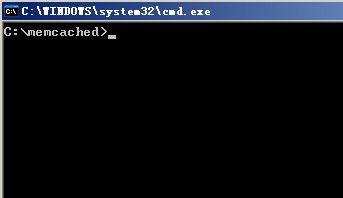
之后输入memcached -h 回车,看帮助说明,接下来输入memcached -d install 回车即可自动安装
memcached服务了,如下图:
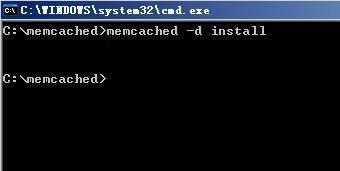
安装好安装memcached服务后,输入memcached -d start 回车启动memcached服务,如下图:
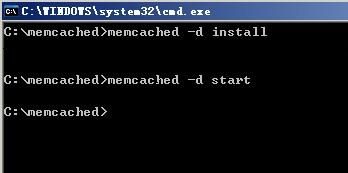
在两台电脑都按以上操作来安装启动memcached。
第三、下载.NET版memcached客户端API组件来写测试程序。
使用memcacheddotnet,下载地址如下:
http://sourceforge.net/projects/memcacheddotnet/
下载好之后把这些文件Commons.dll,ICSharpCode.SharpZipLib.dll,log4net.dll,
Memcached.ClientLibrary.dll放到bin目录(少一个都不行),之后再到测试项目开发环境引用
Memcached.ClientLibrary.dll,如下图
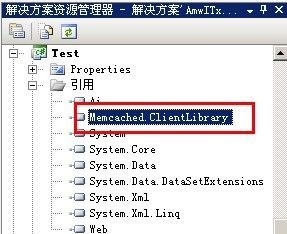
第四、测试程序:
Memcached.cs
--------------------------------------
1
using
System;
2
using
System.Collections.Generic;
3
using
System.Linq;
4
using
System.Text;
5
using
Memcached.ClientLibrary;
6
using
System.Collections;
7
8
namespace
Test {
9
public
partial
class
Memcached {
10
private
static
Memcached _instance
=
new
Memcached();
11
public
static
Memcached _ {
12
get
{
13
return
_instance;
14
}
15
}
16
17
string
[] servers
=
{
"
192.168.1.10:11211
"
,
"
192.168.1.11:11211
"
};
18
SockIOPool pool;
19
MemcachedClient mc;
20
21
public
Memcached() {
22
//
初始化池
23
pool
=
SockIOPool.GetInstance();
24
pool.SetServers(servers);
25
26
pool.InitConnections
=
3
;
27
pool.MinConnections
=
3
;
28
pool.MaxConnections
=
1000
;
29
30
pool.SocketConnectTimeout
=
1000
;
31
pool.SocketTimeout
=
3000
;
32
33
pool.MaintenanceSleep
=
30
;
34
pool.Failover
=
true
;
35
36
pool.Nagle
=
false
;
37
pool.Initialize();
38
39
mc
=
new
MemcachedClient();
40
mc.EnableCompression
=
false
;
41
}
42
43
public
void
Remove(
string
key) {
44
mc.Delete(key);
45
}
46
47
public
bool
Set(
string
key,
object
value) {
48
return
mc.Set(key, value);
49
}
50
51
public
bool
Set(
string
key,
object
value,
int
minute) {
52
return
mc.Set(key, value, DateTime.Now.AddMinutes(minute));
53
}
54
55
public
Hashtable Stats() {
56
return
mc.Stats();
57
}
58
59
public
object
Get(
string
key) {
60
return
mc.Get(key);
61
}
62
63
public
bool
ContainsKey(
string
key) {
64
return
mc.KeyExists(key);
65
}
66
}
67
public
class
MemcachedTest {
68
///
<summary>
69
///
测试缓存
70
///
</summary>
71
public
void
test() {
72
//
写入缓存
73
Console.WriteLine(
"
写入缓存测试:
"
);
74
Console.WriteLine(
"
_______________________________________
"
);
75
if
(Memcached._.ContainsKey(
"
cache
"
)) {
76
Console.WriteLine(
"
缓存cache已存在
"
);
77
}
78
else
{
79
Memcached._.Set(
"
cache
"
,
"
写入缓存时间:
"
+
DateTime.Now.ToString());
80
Console.WriteLine(
"
缓存已成功写入到cache
"
);
81
}
82
Console.WriteLine(
"
_______________________________________
"
);
83
Console.WriteLine(
"
读取缓存内容如下:
"
);
84
Console.WriteLine(Memcached._.Get(
"
cache
"
).ToString());
85
86
//
测试缓存过期
87
Console.WriteLine(
"
_______________________________________
"
);
88
if
(Memcached._.ContainsKey(
"
endCache
"
)) {
89
Console.WriteLine(
"
缓存endCache已存在,过期时间为:
"
+
Memcached._.Get
90
91
(
"
endCache
"
).ToString());
92
}
93
else
{
94
Memcached._.Set(
"
endCache
"
, DateTime.Now.AddMinutes(
1
).ToString(),
1
);
95
Console.WriteLine("缓存已更新写入到endCache");
96 Console.WriteLine("写入时间:" + DateTime.Now.ToString());
97 Console.WriteLine("过期时间:" + DateTime.Now.AddMinutes(1).ToString());
98
}
99
100
//
分析缓存状态
101
Hashtable ht
=
Memcached._.Stats();
102
Console.WriteLine(
"
_______________________________________
"
);
103
104
Console.WriteLine(
"
Memcached Stats:
"
);
105
Console.WriteLine(
"
_______________________________________
"
);
106
foreach
(DictionaryEntry de
in
ht) {
107
Hashtable info
=
(Hashtable)de.Value;
108
foreach
(DictionaryEntry de2
in
info) {
109
Console.WriteLine(de2.Key.ToString()
+
"
:
"
+
de2.Value.ToString()
+
"
"
);
110
}
111
}
112
}
113
}
114
}
115
Program.cs
--------------------------------------
1
using
System;
2
using
System.Collections.Generic;
3
using
System.Linq;
4
using
System.Text;
5
using
System.IO;
6
7
namespace
Test {
8
class
Program {
9
static
void
Main(
string
[] args) {
10
MemcachedTest mt
=
new
MemcachedTest();
11
mt.test();
12
Console.ReadLine();
13
}
14
}
15
}
首次执行效果图:
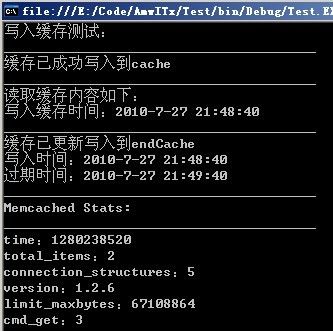
第二次便从缓存中读取:
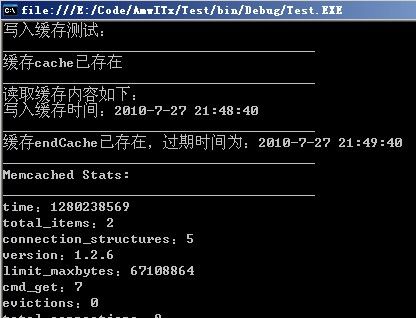