python-对象测量
-
- 一、多边形拟合
- 二、凸包拟合
- 三、矩形包围框拟合
- 四、圆形包围框拟合
- 五、圆形包围框拟合
一、多边形拟合
"""
多边形拟合:以特定的精度逼近多边形曲线
方法:从轮廓中找到距离最远的两个点,并将两个点相连,在轮廓上找到一个距离该线最远的点,并
将该点与原有直线连接成为一个封闭多边形,此时找到一个三角形,将上述过程不断迭代,将新找到的
距离当前多边形最远距离的点加入到结果中,当轮廓上的点到当前的多边形距离低于epsilon时,停止迭代
approxCurve=cv2.approxPoly(curve,epsilon,closed[,approxCure])
approxCurve:近似轮廓
curve:2D向量点
epsilon:精度,原始曲线与近似曲线的最大距离 (一般设为多边形总长的百分比)
closed:曲线是否闭合
"""
import cv2
import matplotlib.pyplot as plt
img = cv2.imread('pic1.jpg',1)
img_gray=cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
ret,thresh=cv2.threshold(img_gray,180,255,0)
contours,hierarchy=cv2.findContours(thresh,cv2.RETR_EXTERNAL,cv2.CHAIN_APPROX_SIMPLE)
cnt = contours[0]
epsilon1 = 0.2*cv2.arcLength(cnt,True)
approx1=cv2.approxPolyDP(cnt,epsilon1,True)
epsilon2 = 0.003*cv2.arcLength(cnt,True)
approx2=cv2.approxPolyDP(cnt,epsilon2,True)
img1=img.copy()
approx_1=cv2.drawContours(img1,[approx1],-1,(0,255,0),2)
img2=img.copy()
approx_2=cv2.drawContours(img2,[approx2],-1,(0,255,0),2)
plt.subplot(2,2,1)
plt.imshow(cv2.cvtColor(img,cv2.COLOR_BGR2RGB))
plt.title("img")
plt.subplot(2,2,2)
plt.imshow(cv2.cvtColor(img_gray,cv2.COLOR_BGR2RGB))
plt.title("img_gray")
plt.subplot(2,2,3)
plt.imshow(cv2.cvtColor(approx_1,cv2.COLOR_BGR2RGB))
plt.title("approx1")
plt.subplot(2,2,4)
plt.imshow(cv2.cvtColor(approx_2,cv2.COLOR_BGR2RGB))
plt.title("approx2")
plt.show()
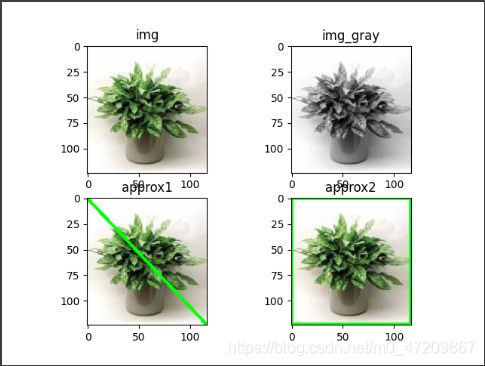
二、凸包拟合
"""
凸包拟合
类似于多边形轮廓拟合,凸包的特点是任意链接两点都在凸包的内部,
hull=cv2.convexHull(points[,hull[,clockwise[,returnPoints]]])
points:轮廓
clockwise:布尔型值,该值为True,凸包角点按顺时针排列
returnPoints:布尔型值,默认为True,返回坐标,当False返回索引
"""
import cv2
import matplotlib.pyplot as plt
img = cv2.imread('pic1.jpg',1)
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
ret,threshold = cv2.threshold(img_gray,230,255,0)
contours,hierarchy=cv2.findContours(threshold,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
cnt=contours[6]
hull = cv2.convexHull(cnt)
img1 = img.copy()
img2 = cv2.drawContours(img1,[hull],-1,(0,255,0),3)
plt.imshow(cv2.cvtColor(img2,cv2.COLOR_BGR2RGB))
plt.title("img2")
plt.show()
三、矩形包围框拟合
"""
矩形包围框拟合
x,y,w,h=cv2.boundingRect(cnt)
x:矩形边界左上角定点的x坐标
y:矩形边界左上角定点的y坐标
w:矩形边界x方向上的长度
h:矩形边界y方向上的长度
"""
import cv2
import matplotlib.pyplot as plt
img = cv2.imread('pic1.jpg',1)
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
ret,threshold = cv2.threshold(img_gray,230,255,0)
contours,hierarchy=cv2.findContours(threshold,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
cnt=contours[6]
x,y,w,h=cv2.boundingRect(cnt)
img1 = img.copy()
img2 = cv2.rectangle(img1,(x,y),(x+w,y+h),(0,255,0),2)
plt.imshow(cv2.cvtColor(img2,cv2.COLOR_BGR2RGB))
plt.title("img2")
plt.show()
四、圆形包围框拟合
"""
旋转包围框拟合
绘制轮廓的最小包围矩形
retval = cv2.minAreaRect(points)
retval:矩形特征信息,中心(x,y),(宽度,高度),旋转角度
points:轮廓
"""
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread('pic1.jpg',1)
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
ret,threshold = cv2.threshold(img_gray,230,255,0)
contours,hierarchy=cv2.findContours(threshold,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
cnt=contours[6]
rect = cv2.minAreaRect(cnt)
box=cv2.boxPoints(rect)
box=np.int0(box)
img1 = img.copy()
img2 = cv2.drawContours(img1,[box],0,(0,255,0),4)
plt.imshow(cv2.cvtColor(img2,cv2.COLOR_BGR2RGB))
plt.title("img2")
plt.show()
五、圆形包围框拟合
"""
圆形包围框拟合:
通过迭代算法构造一个最小的圆形
center,radius=cv2.minEnclosingCircle(points)
center:返回值,圆形的中心
radius:返回值,半径
points:参数,轮廓
"""
import cv2
import matplotlib.pyplot as plt
img = cv2.imread('pic1.jpg',1)
img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
ret,threshold = cv2.threshold(img_gray,230,255,0)
contours,hierarchy=cv2.findContours(threshold,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
cnt=contours[6]
(x,y),radius=cv2.minEnclosingCircle(cnt)
center=(int(x),int(y))
radius =int(radius)
img1 = img.copy()
img2 = cv2.circle(img1,center,radius,(255,255,0),3)
plt.imshow(cv2.cvtColor(img2,cv2.COLOR_BGR2RGB))
plt.title("img2")
plt.show()