Spring MVC 返回统一JSON格式
-
- 格式
- 1、创建一个存放公共返回码的枚举类型
- 2、创建一个Result类封装返回数据
- 3、分页展示工具类
- 4、案例展示
格式
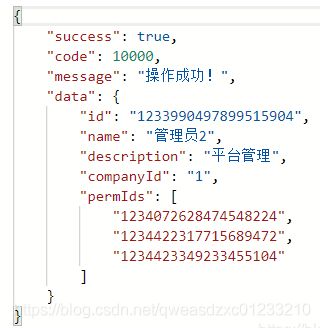
1、创建一个存放公共返回码的枚举类型
public enum ResultCode {
SUCCESS(true,10000,"操作成功!"),
FAIL(false,10001,"操作失败"),
UNAUTHENTICATED(false,10002,"您还未登录"),
UNAUTHORISE(false,10003,"权限不足"),
SERVER_ERROR(false,99999,"抱歉,系统繁忙,请稍后重试!"),
MOBILEORPASSWORDERROR(false,20001,"用户名或密码错误");
boolean success;
int code;
String message;
ResultCode(boolean success,int code, String message){
this.success = success;
this.code = code;
this.message = message;
}
public boolean success() {
return success;
}
public int code() {
return code;
}
public String message() {
return message;
}
}
2、创建一个Result类封装返回数据
@Data
@NoArgsConstructor
public class Result {
private boolean success;
private Integer code;
private String message;
private Object data;
public Result(ResultCode code) {
this.success = code.success;
this.code = code.code;
this.message = code.message;
}
public Result(ResultCode code,Object data) {
this.success = code.success;
this.code = code.code;
this.message = code.message;
this.data = data;
}
public Result(Integer code,String message,boolean success) {
this.code = code;
this.message = message;
this.success = success;
}
public static Result SUCCESS(){
return new Result(ResultCode.SUCCESS);
}
public static Result ERROR(){
return new Result(ResultCode.SERVER_ERROR);
}
public static Result FAIL(){
return new Result(ResultCode.FAIL);
}
}
3、分页展示工具类
@Data
@AllArgsConstructor
@NoArgsConstructor
public class PageResult<T> {
private Long total;
private List<T> rows;
}
4、案例展示
- 带有返回数据
@RestController
public class CompanyController {
@Autowired
private CompanyService companyService;
@GetMapping("/get/{id}")
public Result getCompany(@PathVariable("id") Long id) {
Company company = companyService.getById(id);
return new Result(ResultCode.SUCCESS,company);
}
}
- 无返回数据
@RestController
public class CompanyController {
@Autowired
private CompanyService companyService;
@PostMapping("/add")
public Result addCompany(@RequestBody Company company) {
companyService.addCompany(company);
return new Result(ResultCode.SUCCESS);
}
}
- 分页数据
@RestController
public class CompanyController {
@Autowired
private CompanyService companyService;
@GetMapping("/pageList")
public Result getCompanies(@RequestParam("page") Long page,
@RequestParam("limit") Long limit) {
Page<Company> pageCompany = new Page<>(page,limit);
companyService.page(pageCompany,null);
long total = pageCompany.getTotal();
List<Company> records = pageCompany.getRecords();
PageResult<Company> pr = new PageResult<>(total,records);
return new Result(ResultCode.SUCCESS,pr);
}
}
- 返回数据展示
{
"success": true,
"code": 10000,
"message": "操作成功!",
"data": {
"total": 14,
"rows": [
{
"id": 1239786045768237058,
"name": "dfasf0",
"managerId": "1231241240",
},
{
"id": 1239786045768237058,
"name": "dfasf1",
"managerId": "1231241241",
},
{
"id": 1239786045768237058,
"name": "dfasf2",
"managerId": "1231241242",
},
{
"id": 1239786045768237058,
"name": "dfasf3",
"managerId": "1231241243",
}
]
}
}