1. EL表达式
1.1 介绍
- EL(Expression Lannguage):表达式语言
- 在JSP2.0规范中加入的内容,也是Servlet规范的一部分
- 作用
- 在JSP页面获取数据,让我们的JSP脱离Java代码块和JSP表达式
- 不需要关心是哪个对象,只需要关心名称就行了
- 语法
- 只能从域对象中获取,即四大域对象
1.2 入门案例
<head>
<title>el快速入门title>
head>
<body>
<% request.setAttribute("username","zhangsan");%>
${username}
body>
html>
1.3 功能
1.3.1 获取数据
- 获取普通类型数据
- 自定义对象类型
- ${数据的名称} ==> 获取到的是对象
- ${数据名称.属性名}
- list集合
- map集合
- ${数据名称.map集合key的名称}
- ${数据名称[“map集合key的名称”]}
<body>
<%--基本数据类型--%>
<%pageContext.setAttribute("num",10);%>
${num}<br/>
<%--自定义对象--%>
<%
Student stu = new Student("张三","23");
pageContext.setAttribute("stu",stu);
%>
${stu}<br/>
${stu.name}
${stu.age}
<%--数组类型--%>
<%
String[] arr = {"hello","world"};
pageContext.setAttribute("arr",arr);
%>
${arr} <br/>
${arr[0]}<br/>
${arr[1]}<br/>
<%--List集合--%>
<%
ArrayList<String> list = new ArrayList<>();
list.add("hello");
list.add("world");
pageContext.setAttribute("list",list);
%>
${list}<br/>
${list[0]}<br/>
${list[1]}<br/>
<%--Map集合--%>
<%
HashMap<String,Student> map = new HashMap<>();
map.put("hm1",new Student("张三","23"));
map.put("hm2",new Student("李四","24"));
pageContext.setAttribute("map",map);
%>
${map}<br/>
${map.hm1}<br/>
${map.hm2}<br/>
body>
1.3.2 运算符
运算符 |
作用 |
示例 |
结果 |
==或eq |
等于 |
5 = = 5 或 {5 == 5}或 5==5或{5 eq 5} |
true |
!=或ne |
不等于 |
5 ! = 5 或 {5 != 5}或 5!=5或{5 ne 5} |
false |
<或It |
小于 |
3 < 5 或 {3 < 5}或 3<5或{3 It 5} |
true |
> 或gt |
大于 |
3 > 5 或 {3 > 5}或 3>5或{3 gt 5} |
false |
<=或le |
小于等于 |
3 < = 5 或 {3 <= 5}或 3<=5或{3 le 5} |
true |
>=或ge |
大于等于 |
3 > = 5 或 {3 >= 5}或 3>=5或{3 ge 5} |
false |
运算符 |
作用 |
示例 |
结果 |
&&或and |
并且 |
{A&&B}或{A and B} |
true/false |
||或or |
或者 |
A ∥ ∥ B 或 {A\|\|B}或 A∥∥B或{A or B} |
true/false |
!或not |
取反 |
! A 或 {! A}或 !A或{not A} |
true/false |
运算符 |
作用 |
empty |
判断对象是否为Null,判断字符串是否为空字符串,判断集合长度是否为0 |
条件?表达式1:表达式2 |
三元运算符 |
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@ page import="com.itheima.domain.User" %>
<html>
<head>
<title>EL两个特殊的运算符title>
head>
<body>
<%--empty运算符:
它会判断:对象是否为null,字符串是否为空字符串,集合中元素是否是0个
--%>
<% String str = null;
String str1 = "";
List<String> slist = new ArrayList<String>();
pageContext.setAttribute("str", str);
pageContext.setAttribute("str1", str1);
pageContext.setAttribute("slist", slist);
%>
${empty str}============当对象为null返回true<br/>
${empty str1 }==========当字符串为空字符串是返回true(注意:它不会调用trim()方法)<br>
${empty slist}==========当集合中的元素是0个时,是true
<hr/>
<%--三元运算符
条件?真:假
--%>
<% request.setAttribute("gender", "female"); %>
<input type="radio" name="gender" value="male" ${gender eq "male"?"checked":""} >男
<input type="radio" name="gender" value="female" ${gender eq "female"?"checked":""}>女
body>
html>
- 运行结果
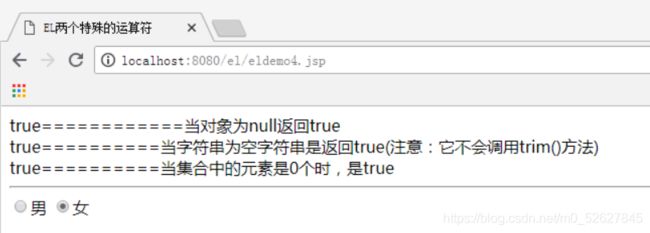
1.4 注意事项
- EL表达式中没有空指针异常
- EL表达式中没有索引越界异常
- EL表达式中没有字符串拼接的效果
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>EL表达式的注意事项title>
head>
<body>
<%--EL表达式的三个没有--%>
第一个:没有空指针异常<br/>
<% String str = null;
request.setAttribute("testNull",str);
%>
${testNull}
<hr/>
第二个:没有数组下标越界<br/>
<% String[] strs = new String[]{"a","b","c"};
request.setAttribute("strs",strs);
%>
取第一个元素:${strs[0]}
取第六个元素:${strs[5]}
<hr/>
第三个:没有字符串拼接<br/>
<%--${strs[0]+strs[1]}--%>
${strs[0]}+${strs[1]}
body>
html>
1.5 使用细节
- EL表达式能够获取四大域对象的数据,
- EL表达式能够获取JSP中其他的八个隐式对象,并且可以调用对应对象的对应方法
- ${pageContext.request.方法名}
- EL表达式中共有11个隐式对象
EL中的隐式对象 |
类型 |
对应JSP隐式对象 |
备注 |
PageContext |
Javax.serlvet.jsp.PageContext |
PageContext |
完全一样 |
ApplicationScope |
Java.util.Map |
没有 |
应用层范围 |
SessionScope |
Java.util.Map |
没有 |
会话范围 |
RequestScope |
Java.util.Map |
没有 |
请求范围 |
PageScope |
Java.util.Map |
没有 |
页面层范围 |
Header |
Java.util.Map |
没有 |
请求消息头key,值是value(一个) |
HeaderValues |
Java.util.Map |
没有 |
请求消息头key,值是数组(一个头多个值) |
Param |
Java.util.Map |
没有 |
请求参数key,值是value(一个) |
ParamValues |
Java.util.Map |
没有 |
请求参数key,值是数组(一个名称多个值) |
InitParam |
Java.util.Map |
没有 |
全局参数,key是参数名称,value是参数值 |
Cookie |
Java.util.Map |
没有 |
Key是cookie的名称,value是cookie对象 |
2. JSTL标签库
2.1 介绍
- JSTL(Java Server Pages Standarded Tag Library):JSP标准标签库
- 主要提供给开发人员一个标准通用的标签库
- 开发人员可以利用这些标签取代JSP中的Java代码
组成 |
作用 |
说明 |
core |
核心标签库 |
通用的逻辑处理 |
fmt |
国际化 |
不同地域显示不同语言 |
functions |
EL函数 |
EL表达式可以使用的方法 |
sql |
操作数据库 |
可以直接从页面上实现数据库操作的功能 |
xml |
操作XML |
能轻松的读取xml文件的内容 |
2.2 核心标签库(core)
标签名称 |
功能分类 |
属性 |
作用 |
<标签名:if> |
流程控制 |
核心标签库 |
用于条件判断 |
<标签名:choose><标签名:when><标签名:otherwise> |
流程控制 |
核心标签库 |
用于多条件判断 |
<标签名:forEach> |
迭代遍历 |
核心标签库 |
用于循环遍历 |
2.3 常用标签
2.3.1 if标签
<%--向域对象中添加成绩数据--%>
${pageContext.setAttribute("score","A")}
<%--对成绩进行判断--%>
<c:if test="${score == 'A'} ">
优秀
c:if>
2.3.2 choose标签
<%--向域对象中添加成绩数据--%>
${pageContext.setAttribute("score","T")}
<%--对成绩进行多条件判断--%>
<c:choose>
<c:when test="${score eq 'A'}">优秀c:when>
<c:when test="${score eq 'B'}">良好c:when>
<c:when test="${score eq 'C'}">及格c:when>
<c:when test="${score eq 'D'}">较差c:when>
<c:otherwise>成绩非法c:otherwise>
c:choose>
2.3.3 forEach标签
- items属性: 集合的名称
- var属性 : 集合中每一个元素的名称
<%--向域对象中添加集合--%>
<%
ArrayList<String> list = new ArrayList<>();
list.add("aa");
list.add("bb");
list.add("cc");
list.add("dd");
pageContext.setAttribute("list",list);
%>
<%--遍历集合--%>
<c:forEach items="${list}" var="str">
${str} <br>
c:forEach>
2.4 JSTL基本使用
- 创建web项目
- 在web目录下创建一个WEB-INF目录
- 在WEB-INF中创建lib文件夹
- 将jar包导入到lib文件夹中
- 在web文件夹中创建一个jsp文件
- 在jsp文件开头第二行引入核心标签库
- <%@ taglib uri = “路径” prefix =“标签名” %>
- 文件中书写代码可以正常使用标签
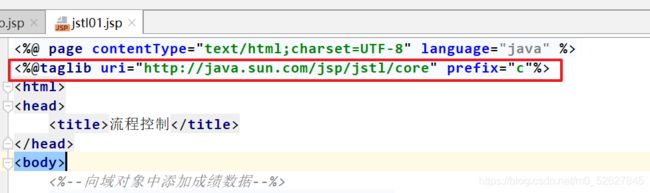
3. 过滤器(Filter)
3.1 介绍
- 在程序中访问服务器资源时,当一个请求到来,服务器首先判断是否有过滤器与请求资源相关联,如果有,过滤器可以将请求拦截下来,完成一些特定的功能,再由过滤器决定是否交给请求资源,如果没有则像之前那样直接请求资源了,响应也是类似的
- 功能
- web阶段的三大组件:servlet 过滤器 监听器
3.2 Filter介绍
- 是一个接口,想要实现过滤器的功能,需要实现该接口,然后进行相关配置
- Filter_API
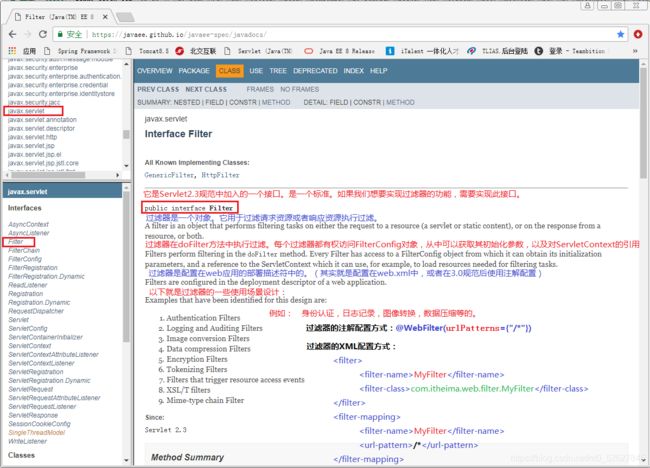
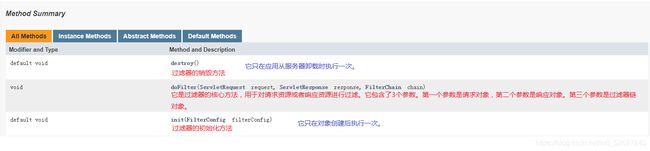
3.3 核心方法
方法 |
说明 |
init(FilterCofig config) |
初始化 |
doFilter(ServletRequset Request,ServletResponse Response,FilterChain ) |
执行服务 |
destroy() |
销毁 |
3.4 FilterChain介绍
- FilterChain是一个接口,代表过滤器链对象,由Servlet容器提供实现类对象,可以直接使用
- 过滤器可以定义多个,就会组成过滤器链对象
- FilterChain_API
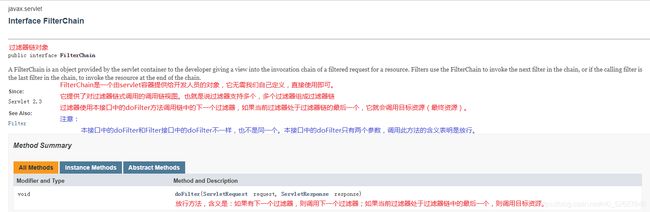
- 核心方法
方法 |
作用 |
doFilter(ServletRequset Request,ServletResponse Response) |
放行方法 |
- 如果有多个过滤器,在第一个过滤器中调用下一个过滤器,以此类推,直到到达最终访问资源,如果只有一个过滤器,放行时,就会直接到达最终访问资源
3.5 案例
- 创建一个web项目
- 创建一个servlet文件
- 定义一个过滤器(创建文件实现Filter接口,重写doFilter方法)
public class FilterDemo1 implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
System.out.println("我是filterDemo1过滤器");
chain.doFilter(request,response);
}
}
- 配置文件
<filter>
<filter-name>demo1filter-name>
<filter-class>com.itheima.filter.FilterDemo1filter-class>
filter>
<filter-mapping>
<filter-name>demo1filter-name>
<url-pattern>/*url-pattern>
filter-mapping>
- 注解的配置方式
- 在过滤器类上添加@WebFilter("/拦截路径")
3.6 拦截路径的配置
3.7 使用细节
3.8 过滤器的生命周期
- 创建
- 当应用加载时实例化对象并执行init初始化方法
- init(FilterConfig filterConfig),需要一个FilterConfig参数
- 服务
- 销毁
- 当应用卸载时或服务器停止时对象销毁,执行destroy方法
3.9 FilterConfig
- 接口,代表过滤器的配置对象,可以加载一些初始化参数
- 把字符集在配置文件中配置,可以通过读取配置文件的方式得到字符集
- FilterConfig_API
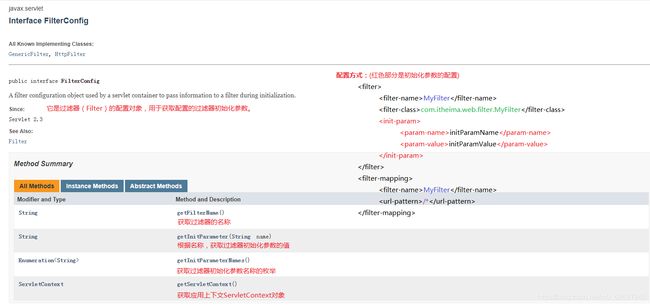
3.10 过滤器的四种拦截行为
- 我们的过滤器目前拦截的是请求,但是在实际开发中,我们还有请求转发和请求包含,以及由服务器触发调用的全局错误页面。默认情况下过滤器是不参与过滤的,要想使用,需要我们配置。配置的方式如下:
<filter>
<filter-name>FilterDemo1filter-name>
<filter-class>com.itheima.web.filter.FilterDemo1filter-class>
<async-supported>trueasync-supported>
filter>
<filter-mapping>
<filter-name>FilterDemo1filter-name>
<url-pattern>/ServletDemo1url-pattern>
<dispatcher>REQUESTdispatcher>
<dispatcher>ERRORdispatcher>
<dispatcher>FORWARDdispatcher>
<dispatcher>INCLUDEdispatcher>
<dispatcher>ASYNCdispatcher>
filter-mapping>
3.11 过滤器与Servlet的区别
方法/类型 |
Servlet |
Filter |
备注 |
初始化 方法 |
void init(ServletConfig); |
void init(FilterConfig); |
几乎一样,都是在web.xml中配置参数,用该对象的方法可以获取到。 |
提供服务方法 |
void service(request,response); |
void dofilter(request,response,FilterChain); |
Filter比Servlet多了一个FilterChain,它不仅能完成Servlet的功能,而且还可以决定程序是否能继续执行。所以过滤器比Servlet更为强大。 在Struts2中,核心控制器就是一个过滤器。 |
销毁方法 |
void destroy(); |
void destroy(); |
|
4. 监听器(Listener)
4.1 介绍
- 观察者设计模式,所有的监听器都是基于观察者设计模式的
- 三个组成部分
- 事件源:触发事件的对象
- 事件:触发的动作,封装了事件源
- 监听器:当事件源触发事件后,可以完成功能
- 在程序当中,我们可以对:对象的创建销毁、域对象中属性的变化、会话的相关内容进行监听
- Servlet中共计有8个监听器
4.2 监听对象的监听器
- ServletContextListener:用于监听ServletContext域对象的创建和销毁
- 核心方法
方法名 |
作用 |
contextInitialized(ServletContextEvent sce) |
对象创建时执行该方法 |
contextDestroyed(ServletContextEvent sce) |
对象销毁时执行该方法 |
- 参数介绍
- ServletContextEvent代表事件对象
- 事件对象中封装了事件源,也就是ServletContext
- 真正的事件指的是创建或销毁ServletContext对象的操作
public interface ServletContextListener extends EventListener {
public default void contextInitialized(ServletContextEvent sce) {
}
public default void contextDestroyed(ServletContextEvent sce) {
}
}
- HttpSessionListener:用于监听HttpSession对象的创建和销毁
- 核心方法
方法名 |
作用 |
sessionCreated(HttpSessionEvent se) |
对象创建时执行该方法 |
sessionDestroyed(HttpSessionEvent se) |
对象销毁时执行该方法 |
- 参数介绍
- HttpSessionEvent代表事件对象
- 事件对象中封装了事件源,也就是HttpSession
- 真正的事件指的是创建或销毁HttpSession对象的操作
public interface HttpSessionListener extends EventListener {
public default void sessionCreated(HttpSessionEvent se) {
}
public default void sessionDestroyed(HttpSessionEvent se) {
}
}
- ServletRequestListener:用于监听ServletRequest对象的创建和销毁
- 核心方法
方法 |
作用 |
requestInitialized(ServletRequestEvent sre) |
对象创建时执行该方法 |
requestDestroyed(ServletRequestEvent sre) |
对象销毁时执行该方法 |
- 参数介绍
- ServletRequestEvent代表事件对象
- 事件对象中封装了事件源,也就是ServletRequest
- 真正的事件指的是创建或销毁ServletRequest对象的操作
public interface ServletRequestListener extends EventListener {
public default void requestInitialized (ServletRequestEvent sre) {
}
public default void requestDestroyed (ServletRequestEvent sre) {
}
}
4.3 监听域对象属性变化的监听器
- ServletContextAttributeListener:用于监听ServletContext应用域中属性的变化
- 核心方法
方法 |
作用 |
attributeAdded(ServletContextAttributeEvent scae) |
域中添加属性时执行该方法 |
attributeReplaced(ServletContextAttributeEvent scae) |
域中替换属性时执行该方法 |
attributeRemoved(ServletContextAttributeEvent scae) |
域中移除属性时执行该方法 |
- 参数介绍
- ServletContextAttributeEvent代表事件对象
- 事件对象中封装了事件源,也就是ServletContext
- 真正的事件指的是添加、移除、替换应用域中属性的操作
public interface ServletContextAttributeListener extends EventListener {
public default void attributeAdded(ServletContextAttributeEvent scae) {
}
public default void attributeRemoved(ServletContextAttributeEvent scae) {
}
public default void attributeReplaced(ServletContextAttributeEvent scae) {
}
}
- HttpSessionAttributeListener:用于监听HttpSession会话域中属性的变化
- 核心方法
方法 |
作用 |
attributeAdded(HttpSessionBindingEvent se) |
域中添加属性时执行该方法 |
attributeRemoved(HttpSessionBindingEvent se) |
域中移除属性时执行该方法 |
attributeReplaced(HttpSessionBindingEvent se) |
域中替换属性时执行该方法 |
- 参数介绍
- HttpSessionBindingEvent代表事件对象
- 事件对象中封装了事件源,也就是HttpSession
- 真正的事件指的是添加、移除、替换会话域中属性的操作
public interface HttpSessionAttributeListener extends EventListener {
public default void attributeAdded(HttpSessionBindingEvent se) {
}
public default void attributeRemoved(HttpSessionBindingEvent se) {
}
public default void attributeReplaced(HttpSessionBindingEvent se) {
}
}
- ServletRequestAttributeListener:用于监听ServletRequest请求域中属性的变化
- 核心方法
方法 |
作用 |
attributeAdded(ServletRequestAttributeEvent srae) |
域中添加属性时执行该方法 |
attributeRemoved(ServletRequestAttributeEvent srae) |
域中移除属性时执行该方法 |
attributeReplaced(ServletRequestAttributeEvent srae) |
域中替换属性时执行该方法 |
- 参数介绍
- ServletRequestAttributeEvent代表事件对象
- 事件对象中封装了事件源,也就是ServletRequest
- 真正的事件指的是添加、移除、替换请求域中属性的操作
public interface ServletRequestAttributeListener extends EventListener {
public default void attributeAdded(ServletRequestAttributeEvent srae) {
}
public default void attributeRemoved(ServletRequestAttributeEvent srae) {
}
public default void attributeReplaced(ServletRequestAttributeEvent srae) {
}
}
4.4 监听会话相关的感知型监听器
- HttpSessionBindingListener:用于感知对象和会话域绑定的监听器
- 核心方法
方法 |
作用 |
valueBound(HttpSessionBindingEvent event) |
数据添加到会话域中(绑定)时执行该方法 |
valueUnbound(HttpSessionBindingEvent event) |
数据从会话域中(解绑)时执行该方法 |
- 参数介绍
- HttpSessionBindingEvent代表事件对象
- 事件对象中封装了事件源,也就是HttpSession
- 真正的事件指的是添加、移除会话域数据的操作
public interface HttpSessionBindingListener extends EventListener {
public default void valueBound(HttpSessionBindingEvent event) {
}
public default void valueUnbound(HttpSessionBindingEvent event) {
}
}
- HttpSessionActivationListener:用于感知会话域中对象钝化和活化的监听器
- 核心方法
方法 |
作用 |
sessionWillPassivate(HttpSessionEvent se) |
会话域中数据钝化时执行该方法 |
sessionDidActivate(HttpSessionEvent se) |
会话域中数据活化时执行该方法 |
- 参数介绍
- HttpSessionEvent代表事件对象
- 事件对象中封装了事件源,也就是HttpSession
- 真正的事件指的是会话域中数据钝化、活化的操作
public interface HttpSessionActivationListener extends EventListener {
public default void sessionWillPassivate(HttpSessionEvent se) {
}
public default void sessionDidActivate(HttpSessionEvent se) {
}
}
4.5 监听器的使用
public class ServletContextListenerDemo implements ServletContextListener {
@Override
public void contextInitialized(ServletContextEvent sce) {
System.out.println("监听到了对象的创建");
ServletContext servletContext = sce.getServletContext();
System.out.println(servletContext);
}
@Override
public void contextDestroyed(ServletContextEvent sce) {
System.out.println("监听到了对象的销毁");
}
}
<listener>
<listener-class>com.itheima.web.listener.ServletContextListenerDemolistener-class>
listener>
- 测试结果
