文章目录
-
- 10.3
- 10.5
- 10.10
- 10.13
- 10.18
- 10.19
- 10.25
10.3

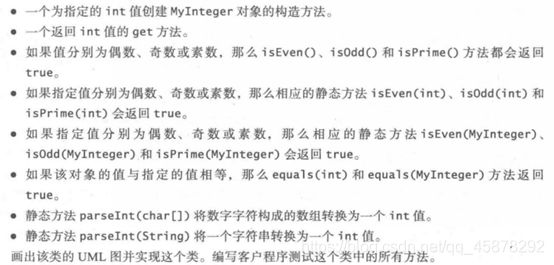
package wwr;
import java.util.Scanner;
class MyInteger {
private int value;
MyInteger(int Value) {
this.value = Value;
}
public int getValue() {
return value;
}
public boolean isEven() {
return isEven(value);
}
public boolean isOdd() {
return isOdd(value);
}
public boolean isPrime() {
return isPrime(value);
}
public static boolean isEven(int value) {
if(value % 2 == 0)
return true;
else
return false;
}
public static boolean isOdd(int value) {
if(value % 2 != 0)
return true;
else
return false;
}
public static boolean isPrime(int value) {
for(int i = 2; i < value - 1; i++)
if(value % i == 0)
return false;
return true;
}
public static boolean isEven(MyInteger m) {
if(m.getValue() % 2 == 0)
return true;
else
return false;
}
public static boolean isOdd(MyInteger m) {
if(m.getValue() % 2 != 0)
return true;
else
return false;
}
public static boolean isPrime(MyInteger m) {
for(int i = 2; i < m.getValue() - 1; i++)
if(m.getValue() % i == 0)
return false;
return true;
}
public boolean equals(int value) {
if(this.value == value)
return true;
else
return false;
}
public boolean equals(MyInteger m) {
if(this.value == m.getValue())
return true;
else
return false;
}
public static int parseInt(char[] c) {
int tmp = 1, num = 0;
for(int i = c.length; i >= 0; i--) {
num += c[i] * tmp;
tmp *= 10;
}
return num;
}
public static int parseInt(String s) {
int tmp = 1, num = 0;
for(int i = s.length(); i >= 0; i--) {
num += s.charAt(i) * tmp;
tmp *= 10;
}
return num;
}
}
public class Demo {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter n1: ");
int n1 = input.nextInt();
MyInteger N1 = new MyInteger(n1);
System.out.println("n1 is " + N1.getValue());
System.out.println("Is n1 an even number?" + '\t' + N1.isEven());
System.out.println("Is n1 an odd number?" + '\t' + N1.isOdd());
System.out.println("Is n1 a prime?" + '\t' + N1.isPrime());
System.out.println("Is n1 an even number?" + '\t' + MyInteger.isEven(n1));
System.out.println("Is n1 an odd number?" + '\t' + MyInteger.isOdd(n1));
System.out.println("Is n1 a prime?" + '\t' + MyInteger.isPrime(n1));
System.out.println("Is n1 an even number?" + '\t' + MyInteger.isEven(N1));
System.out.println("Is n1 an odd number?" + '\t' + MyInteger.isOdd(N1));
System.out.println("Is n1 a prime?" + '\t' + MyInteger.isPrime(N1));
System.out.print("Enter n2: ");
int n2 = input.nextInt();
MyInteger N2 = new MyInteger(n2);
System.out.println("Is n1 equal to n2?" + '\t' + N1.equals(n2));
System.out.println("Is n1 equal to n2?" + '\t' + N1.equals(N2));
}
}
10.5

package wwr;
import java.util.Scanner;
import java.util.Arrays;
class StackOfIntegers {
private int integer;
public StackOfIntegers(int integer) {
this.integer = integer;
}
private static int k = 0;
private static int l = 0;
public int[] GetPrime() {
int[] getPrime = new int[this.integer];
boolean isPrime = true;
for (int i = 2; i < this.integer; i++) {
for (int h = 2; h < i; h++) {
if (i % h == 0) {
isPrime = false;
break;
}
}
if (isPrime) {
getPrime[k] = i;
k++;
} else isPrime = true;
}
return getPrime;
}
public int[] Prime() {
int[] get = GetPrime();
int[] prime1 = new int[k];
boolean judge = false;
while (this.integer != 0) {
for (int a = k - 1; a >= 0; a--) {
if ((int) (this.integer / get[a]) == (double) this.integer / get[a]) {
prime1[l] = get[a];
this.integer /= get[a];
l++;
}
if (integer == get[a] || integer == 1) {
prime1[l] = get[a];
judge = true;
break;
}
}
if (judge)
break;
}
int[] prime = new int[l + 1];
for (int i = 0; i < l + 1; i++){
prime[i] = prime1[i];
}
return prime;
}
}
public class Demo {
public static void main(String[] args) {
System.out.print("Please input a integer: ");
Scanner input = new Scanner(System.in);
int integer=input.nextInt();
StackOfIntegers stackOfIntegers = new StackOfIntegers(integer);
int[] a=stackOfIntegers.Prime();
System.out.print("All the prime factors of the "+ integer + " are :");
System.out.print(Arrays.toString(a));
input.close();
}
}
10.10
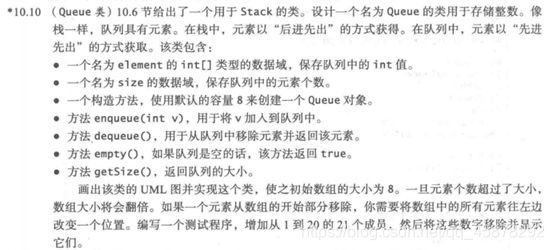
package wwr;
import javax.management.RuntimeErrorException;
class Queue{
private int[] element;
public static final int capacity = 8;
private int size = 0;
public Queue() {
element = new int[capacity];
}
public void enqueue(int v) {
if(size >= element.length) {
int[] temp = new int[element.length * 2];
System.arraycopy(element, 0, temp, 0, element.length);
element = temp;
}
element[size++] = v;
}
public int dequeue() {
if (empty()) {
throw new RuntimeException("error");
}
else {
int temp = element[0];
for (int i = 0; i < element.length - 1; i++) {
element[i] = element[i + 1];
}
size--;
return temp;
}
}
public boolean empty() {
return size == 0;
}
public int getSize() {
return size;
}
}
public class Demo {
public static void main(String[] args) {
Queue queue = new Queue();
for (int i = 1; i <= 20; i++) {
queue.enqueue(i);
}
System.out.println("队列实际长度:" + queue.getSize());
for (int i = 0; i < 20; i++) {
System.out.println("元素 "+ queue.dequeue() + "出队列");
}
}
}
10.13

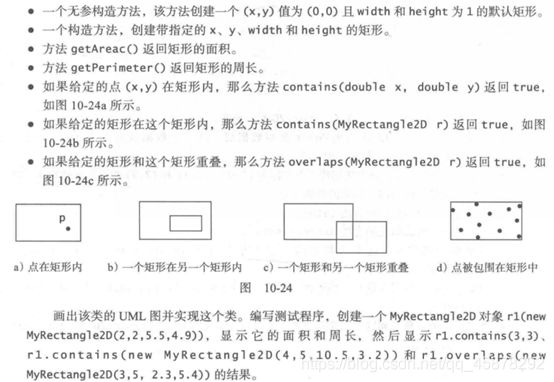
package wwr;
import java.util.*;
class MyRectangle2D {
public MyRectangle2D() {
this.x = 0;
this.y = 0;
this.height = 1;
this.width = 1;
}
private double x;
private double y;
private double width;
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
private double height;
public MyRectangle2D(double x, double y, double width, double height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public double getArea() {
return height * width;
}
public double getPerimeter() {
return (height + width) * 2;
}
public boolean contains(double x, double y) {
return Math.abs(x - this.x) < width / 2 && Math.abs(y - this.y) < height / 2;
}
public boolean contains(MyRectangle2D r) {
return Math.abs(this.x - r.getX()) <= Math.abs((this.width - r.width) / 2)
&& Math.abs(this.y - r.y ) <= Math.abs((this.height - r.height) / 2);
}
public boolean overLaps(MyRectangle2D r) {
boolean overlaps1 = Math.abs(this.x - r.getX()) > Math.abs((this.width - r.width) / 2)
&& Math.abs(this.y - r.y )> Math.abs((this.height - r.height) / 2);
boolean overlaps2 = Math.abs(this.x - r.getX()) < Math.abs((this.width + r.width) / 2)
&& Math.abs(this.y - r.y )< Math.abs((this.height + r.height) / 2);
return overlaps1 && overlaps2;
}
}
public class Demo {
public static void main(String[] args) {
MyRectangle2D r1 = new MyRectangle2D(2, 2, 5.5, 4.9);
System.out.println("The r1's area is " + r1.getArea());
System.out.println("The r1's perimeter is " + r1.getPerimeter());
System.out.println("The point contain is " + r1.contains(3, 3));
System.out.println("The new myRectangle contain is " + r1.contains(new MyRectangle2D(4, 5, 10.5, 3.2)));
System.out.println("The new myRectangle overLaps is " + r1.overLaps(new MyRectangle2D(3, 5, 2.3, 5.4)));
}
}
10.18

package wwr;
import java.math.BigInteger;
public class Demo {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
BigInteger bigNum = new BigInteger(Long.MAX_VALUE + "");
bigNum = bigNum.add(BigInteger.ONE);
int count = 1;
while (count <= 5) {
if (isPrime(bigNum)) {
System.out.println(bigNum);
count++;
}
bigNum = bigNum.add(BigInteger.ONE);
}
System.out.println(bigNum.toString());
}
public static boolean isPrime(BigInteger num) {
return true;
}
}
10.19
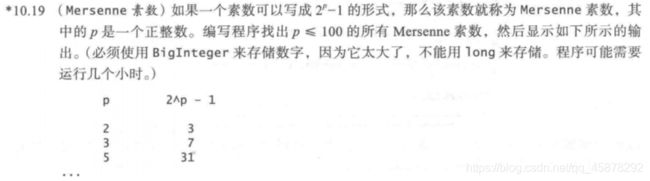
package wwr;
import java.math.BigInteger;
public class Demo {
public static void Check(BigInteger result, int i) {
if(result.isProbablePrime(1))
System.out.println(i + "\t" + result);
}
public static void main(String[] args) {
BigInteger num1 = BigInteger.ONE;
System.out.println("p\t2^p-1");
System.out.println("");
for(int i = 0; i <= 100;i++) {
num1 = num1.multiply(new BigInteger("2"));
BigInteger result = num1.subtract(new BigInteger("1"));
Check(result, i);
}
}
}
10.25
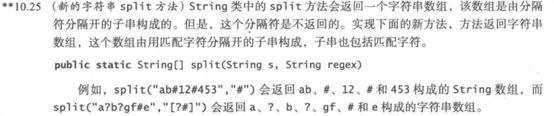
package wwr;
import java.util.Scanner;
import java.util.ArrayList;
import java.util.regex.*;
public class Demo {
public static String[] split(String s, String regex) {
ArrayList<String> matchList = new ArrayList<String>();
Pattern pat = Pattern.compile(regex);
Matcher mat = pat.matcher(s);
int index = 0;
while(mat.find()) {
String match = s.subSequence(index, mat.start()).toString();
matchList.add(match);
String match2 = s.subSequence(mat.start(),mat.end()).toString();
matchList.add(match2);
index = mat.end();
}
String match = s.subSequence(index,s.length()).toString();
matchList.add(match);
int resultSize = matchList.size();
while (resultSize > 0 && matchList.get(resultSize-1).equals(""))
resultSize--;
String[] result = new String[resultSize];
return matchList.subList(0, resultSize).toArray(result);
}
public static void main(String[] args) {
String[] ans=split("ab#123#453","#");
System.out.println("ab#123#453");
for(int i=0;i<ans.length;i++) {
System.out.println(ans[i]);
}
String[] ans2=split("a?b?gf#e","[?#]");
System.out.println("a?b?gf#e");
for(int i=0;i<ans2.length;i++) {
System.out.println(ans2[i]);
}
}
}