springboot+vue实现手机验证码功能
-
榛子云短信平台用户中心注册登录(有免费的一条消息,剩下的需要买)(阿里云个人得备案)
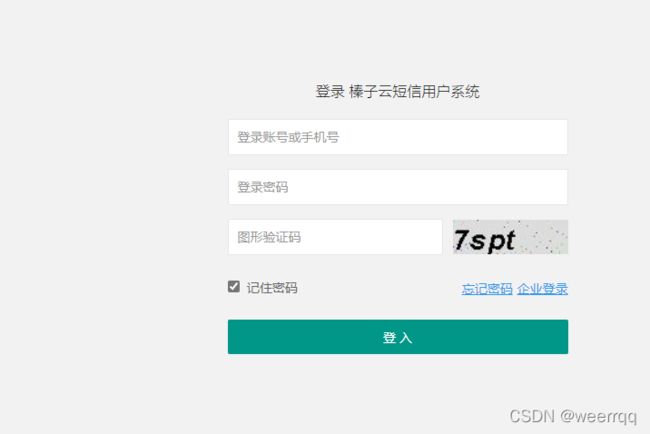
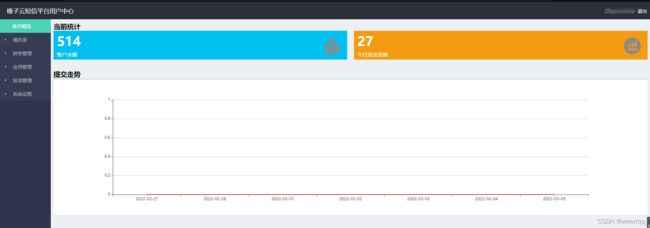
-
在springboot中加入依赖,用到了redis,阿里的fastjson,和短信的平台
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-redisartifactId>
<version>1.4.1.RELEASEversion>
dependency>
<dependency>
<groupId>com.zhenzikjgroupId>
<artifactId>zhenzismsartifactId>
<version>2.0.2version>
dependency>
<dependency>
<groupId>com.alibabagroupId>
<artifactId>fastjsonartifactId>
<version>1.2.49version>
dependency>
- 然后直接编写controller层
import com.alibaba.fastjson.JSONObject;
import com.pro.back.util.RedisUtils;
import com.zhenzi.sms.ZhenziSmsClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpSession;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
import java.util.concurrent.TimeUnit;
@Controller
public class CodeController {
@Autowired
RedisUtils redisUtils;
private String apiUrl = "https://sms_developer.zhenzikj.com";
private String appId = "xxxxxx";
private String appSecret = "xxxxxxxxxxxxxxxxxxx";
@ResponseBody
@GetMapping("/code")
public boolean getCode(@RequestParam("memPhone") String memPhone, HttpSession httpSession){
try {
JSONObject json = null;
String code = String.valueOf(new Random().nextInt(999999));
ZhenziSmsClient client = new ZhenziSmsClient(apiUrl, appId, appSecret);
Map<String, Object> params = new HashMap<String, Object>();
params.put("number", memPhone);
params.put("templateId", "8369");
String[] templateParams = new String[2];
templateParams[0] = code;
templateParams[1] = "2分钟";
params.put("templateParams", templateParams);
String result = client.send(params);
json = JSONObject.parseObject(result);
if (json.getIntValue("code")!=0){
return false;
}
json = new JSONObject();
json.put("memPhone",memPhone);
json.put("code",code);
json.put("createTime",System.currentTimeMillis());
redisUtils.set("verifyCode"+memPhone,json,5L, TimeUnit.MINUTES);
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
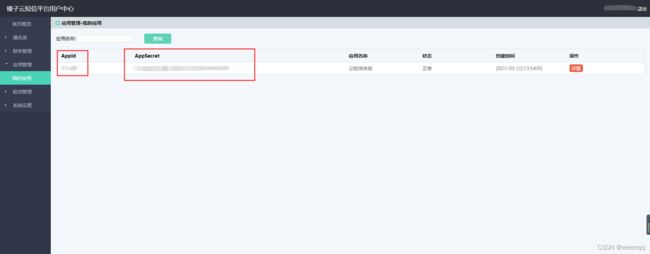
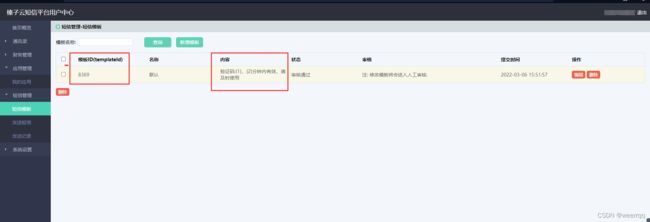
- 编写redisUtils,我用的网上的模板
首先在配置文件写一下(我写的很简单,但是能用就行,redis密码默认没有就不写了)
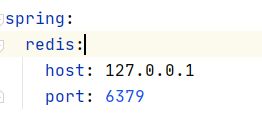
import java.io.Serializable;
import java.util.List;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.HashOperations;
import org.springframework.data.redis.core.ListOperations;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.SetOperations;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.data.redis.core.ZSetOperations;
import org.springframework.stereotype.Service;
@Service
public class RedisUtils {
@Autowired
private RedisTemplate redisTemplate;
public boolean set(final String key, Object value) {
boolean result = false;
try {
ValueOperations<Serializable, Object> operations = redisTemplate.opsForValue();
operations.set(key, value);
result = true;
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public boolean set(final String key, Object value, Long expireTime, TimeUnit timeUnit) {
boolean result = false;
try {
ValueOperations<Serializable, Object> operations = redisTemplate.opsForValue();
operations.set(key, value);
redisTemplate.expire(key, expireTime, timeUnit);
result = true;
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public void remove(final String... keys) {
for (String key : keys) {
remove(key);
}
}
public void removePattern(final String pattern) {
Set<Serializable> keys = redisTemplate.keys(pattern);
if (keys.size() > 0) {
redisTemplate.delete(keys);
}
}
public void remove(final String key) {
if (exists(key)) {
redisTemplate.delete(key);
}
}
public boolean exists(final String key) {
return redisTemplate.hasKey(key);
}
public Object get(final String key) {
Object result = null;
ValueOperations<Serializable, Object> operations = redisTemplate.opsForValue();
result = operations.get(key);
return result;
}
public void hmSet(String key, Object hashKey, Object value) {
HashOperations<String, Object, Object> hash = redisTemplate.opsForHash();
hash.put(key, hashKey, value);
}
public Object hmGet(String key, Object hashKey) {
HashOperations<String, Object, Object> hash = redisTemplate.opsForHash();
return hash.get(key, hashKey);
}
public void lPush(String k, Object v) {
ListOperations<String, Object> list = redisTemplate.opsForList();
list.rightPush(k, v);
}
public List<Object> lRange(String k, long l, long l1) {
ListOperations<String, Object> list = redisTemplate.opsForList();
return list.range(k, l, l1);
}
public void add(String key, Object value) {
SetOperations<String, Object> set = redisTemplate.opsForSet();
set.add(key, value);
}
public Set<Object> setMembers(String key) {
SetOperations<String, Object> set = redisTemplate.opsForSet();
return set.members(key);
}
public void zAdd(String key, Object value, double scoure) {
ZSetOperations<String, Object> zset = redisTemplate.opsForZSet();
zset.add(key, value, scoure);
}
public Set<Object> rangeByScore(String key, double scoure, double scoure1) {
ZSetOperations<String, Object> zset = redisTemplate.opsForZSet();
return zset.rangeByScore(key, scoure, scoure1);
}
}
- 前端vue测试获取,随便把获取验证码60秒内不能重复获取做了
<template>
<div>
<el-form :model="loginForm">
<el-form-item label="手机号">
<el-input type="text" v-model="loginForm.username" style="width: 200px">el-input>
el-form-item>
<div class="login_box">
<el-input type="text" v-model="loginForm.code" aria-placeholder="请输入验证码" style="width: 200px">el-input>
<el-button @click="postCode" :disabled="isClick">{{content}}el-button>
div>
el-form>
div>
template>
<script>
import {getCode, testCode} from "../api/admin/admin";
export default {
name: "Login",
data(){
return{
loginForm: {
code:'',
username:''
},
content: '发送验证码',
totalTime: 60,
isClick:false
}
},
methods:{
postCode(){
getCode(this.loginForm.username).then(res =>{
console.log(res)
})
this.countDown()
},
countDown () {
this.content = this.totalTime + 's后重新发送'
let clock = window.setInterval(() => {
this.totalTime--
this.content = this.totalTime + 's后重新发送'
this.isClick=true
if (this.totalTime < 0) {
window.clearInterval(clock)
this.content = '重新发送验证码'
this.totalTime = 60
this.isClick=false
}
},1000)
console.log(111)
}
},
computed:{
}
}
script>
- 此时,验证码发送到了手机,并且存入到了redis
- 接下来验证,,还是在刚刚那个controller里面写,其实只要提取一下redis里面的数据即可,从前端传来的手机号,通过手机号获取刚刚存入redis的验证码,看看是否一样
@ResponseBody
@PostMapping("/getCode")
public JSONObject getCode(@RequestParam(value = "username") String username,String code){
JSONObject Vcode = (JSONObject)redisUtils.get("verifyCode"+username);
System.out.println("memPhone:"+username);
System.out.println(Vcode);
JSONObject verifyCode = (JSONObject)redisUtils.get("verifyCode"+username);
System.out.println("传入的验证码是:"+code);
if(verifyCode.get("code").equals(code)){
System.out.println("验证码正确");
}
return Vcode;
}