package com.spsw.mall.utlis;
import cn.hutool.http.HttpUtil;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.*;
import java.nio.Buffer;
import java.nio.charset.Charset;
import java.util.Base64;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.imageio.ImageIO;
import javax.imageio.stream.ImageOutputStream;
import javax.servlet.http.HttpServletResponse;
import com.huangliang.utils.BaseUtil;
import com.huangliang.utils.HttpUtils;
import com.huangliang.utils.constant.CommonConstant;
import com.huangliang.utils.exception.BaseException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.springframework.core.io.ClassPathResource;
import org.springframework.web.multipart.MultipartFile;
public class ImgUtil {
/**
* 定义二维码图片的宽度
*/
private static final int WIDTH = 125;
/**
* 定义二维码图片的高度
*/
private static final int HEIGHT = 125;
/**
* 定义LOGO图片的宽度
*/
private static final int LOGO_WIDTH = 40;
/**
* 定义LOGO图片的高度
*/
private static final int LOGO_HEIGHT = 40;
final private static String wechatToken = "https://api.weixin.qq.com/cgi-bin/token";
final private static String wechatGetUnlimited = "https://api.weixin.qq.com/wxa/getwxacodeunlimit";
/**
* 生成二维码的方法
*/
public static BufferedImage execute(Integer id) throws Exception {
/** 判断二维码中URL */
String url = null;
if (url == null || "".equals(url)) {
url = "礼好啊奥里给!";
}
/** 定义Map集合封装二维码配置信息 */
Map hints = new HashMap<>();
/** 设置二维码图片的内容编码 */
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");
/** 设置二维码图片的上、下、左、右间隙 */
hints.put(EncodeHintType.MARGIN, 1);
/** 设置二维码的纠错级别 */
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
/**
* 创建二维码字节转换对象
* 第一个参数:二维码图片中的内容
* 第二个参数:二维码格式器
* 第三个参数:生成二维码图片的宽度
* 第四个参数:生成二维码图片的高度
* 第五个参数:生成二维码需要配置信息
* */
BitMatrix matrix = new MultiFormatWriter().encode("想要苹果?想屁吃",
BarcodeFormat.QR_CODE, WIDTH, HEIGHT, hints);
/** 获取二维码图片真正的宽度 */
int matrix_width = matrix.getWidth();
/** 获取二维码图片真正的高度 */
int matrix_height = matrix.getHeight();
/** 定义一张空白的缓冲流图片 */
BufferedImage image = new BufferedImage(matrix_width, matrix_height,
BufferedImage.TYPE_INT_RGB);
/** 把二维码字节转换对象 转化 到缓冲流图片上 */
for (int x = 0; x < matrix_width; x++) {
for (int y = 0; y < matrix_height; y++) {
/** 通过x、y坐标获取一点的颜色 true: 黑色 false: 白色 */
int rgb = matrix.get(x, y) ? 0x000000 : 0xFFFFFF;
image.setRGB(x, y, rgb);
}
}
/** 获取公司logo图片 */
// BufferedImage logo = ImageIO.read(new ClassPathResource("static/img/123.jpg").getInputStream());
/** 获取缓冲流图片的画笔 */
Graphics2D g = (Graphics2D) image.getGraphics();
/** 在二维码图片中间绘制公司logo */
g.drawImage(null, (matrix_width - LOGO_WIDTH) / 2,
(matrix_height - LOGO_HEIGHT) / 2, LOGO_WIDTH, LOGO_HEIGHT, null);
/** 设置画笔的颜色 */
g.setColor(Color.WHITE);
/** 设置画笔的粗细 */
g.setStroke(new BasicStroke(5.0f));
/** 设置消除锯齿 */
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
/** 绘制圆角矩形 */
g.drawRoundRect((matrix_width - LOGO_WIDTH) / 2, (matrix_height - LOGO_HEIGHT) / 2,
LOGO_WIDTH, LOGO_HEIGHT, 10, 10);
return image;
}
/**
* 生成小程序二维码
*/
public static String getUnlimited(Integer id,String type){
Map getToken = new HashMap();
getToken.put("grant_type", "client_credential");
getToken.put("appid", CommonConstant.WX_LOGIN_APPID);
getToken.put("secret", CommonConstant.WX_LOGIN_SECRET);
try {
JSONObject wxTokenRes = JSON.parseObject(HttpUtils.doGet(wechatToken, getToken));
if (BaseUtil.isNotEmpty(wxTokenRes.getString("access_token"))) {
Map param = new HashMap<>();
String wxToken = wxTokenRes.getString("access_token");
String url = "https://api.weixin.qq.com/wxa/getwxacodeunlimit?access_token="+wxToken;
String scene = "id="+String.valueOf(id)+","+"type="+type;
param.put("scene",java.net.URLDecoder.decode(scene,"UTF-8"));
param.put("width",280);
// param.put("page","pages/login/index");
String json = JSON.toJSONString(param);
ByteArrayInputStream inputStream = sendPost(url, json);
//这里判断的是返回的图片还是错误信息,一般错误信息不会大于200
byte[] data = null;
if (inputStream.available() <= 200){
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
int i;
byte[] buffer = new byte[200];
while ((i = inputStream.read(buffer)) != -1){
byteArrayOutputStream.write(buffer,0,i);
}
String str = new String(byteArrayOutputStream.toByteArray());
//错误信息
JSONObject jsonObject = JSONObject.parseObject(str);
if ("41030".equals(jsonObject.getString("errcode"))){
throw new BaseException("所传page页面不存在,或者小程序没有发布");
}else if ("45009".equals(jsonObject.getString("errcode"))){
throw new BaseException("调用分钟频率受限");
}
byteArrayOutputStream.close();
}
ByteArrayOutputStream baos = new ByteArrayOutputStream();
int len = 0;
byte[] b = new byte[1024];
while ((len = inputStream.read(b, 0, b.length)) != -1) {
baos.write(b, 0, len);
}
byte[] buffer = baos.toByteArray();
String base64 = Base64.getEncoder().encodeToString(buffer);
// BufferedImage image = ImageIO.read(inputStream);
return "data:image/jpeg;base64," + base64;
// return image;
}
}catch (IOException e) {
throw new BaseException(e.getMessage());
}
return null;
}
// 二维码和图片的合成
public static final void combineCodeAndPicToFile(Integer id) {
long starttime = System.currentTimeMillis();
System.out.println("开始合成:" + starttime);
try {
//背景图
//BufferedImage big = ImageIO.read(new File(backPicPath));
BufferedImage big = ImageIO.read(new ClassPathResource("static/img/456.jpg").getInputStream());
//二维码的图片
//url扫二维码显示的内容
BufferedImage small = execute(id);
Graphics2D g = big.createGraphics(); //合成的图片
//二维码或小图在大图的左上角坐标
int x = big.getWidth() - small.getWidth()-45; //加是向右,减是向左
int y = (big.getHeight() - small.getHeight()-50); //加是向下,减是向上
//将二维码花在背景图上
g.drawImage(small, x, y, small.getWidth(), small.getHeight(), null);
//结束绘画
g.dispose();
//为了保证大图背景不变色,formatName必须为"png"
ImageIO.write(big, "png", new FileOutputStream("111.jpg"));
System.out.println("结束合成:" + (System.currentTimeMillis() - starttime) / 1000);
} catch (Exception e) {
e.printStackTrace();
}
}
//合成base64响应前台图片数据展示
public static final String combineCodeAndPicToBase64(MultipartFile file,Integer id) {
ImageOutputStream imOut = null;
try {
BufferedImage big = ImageIO.read(file.getInputStream());
// BufferedImage small = ImageIO.read(new File(fillPicPath));
BufferedImage small = execute(id);
Graphics2D g = big.createGraphics();
//左下角位置
int x = big.getWidth() - small.getWidth()-45; //加是向右,减是向左
int y = (big.getHeight() - small.getHeight()-50); //加是向下,减是向上
//右下角位置
/* int x = 45; //加是向右,减是向左
int y = (big.getHeight() - small.getHeight()-50); //加是向下,减是向上*/
//居中位置
// int x = (big.getWidth() - small.getWidth())/2; //加是向右,减是向左
// int y = (big.getHeight() - small.getHeight()-50); //加是向下,减是向上
g.drawImage(small, x, y, small.getWidth(), small.getHeight(), null);
g.dispose();
//为了保证大图背景不变色,formatName必须为"png"
//字节数组流
ByteArrayOutputStream bs = new ByteArrayOutputStream();
//将图片流转换为字节数组流
imOut = ImageIO.createImageOutputStream(bs);
//将合成好的背景图输入到字节数组流中
ImageIO.write(big, "png", imOut);
//将字节数组流转换为二进制流
InputStream in = new ByteArrayInputStream(bs.toByteArray());
byte[] bytes = new byte[in.available()];
//读取数组流中的数据
in.read(bytes);
//转换为base64数据类型
String base64 = Base64.getEncoder().encodeToString(bytes);
System.out.println(base64);
return "data:image/jpeg;base64," + base64;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static ByteArrayInputStream sendPost(String URL, String json) {
InputStream inputStream = null;
ByteArrayInputStream byteArrayInputStream = null;
// 创建默认的httpClient实例.
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建httppost
HttpPost httppost = new HttpPost(URL);
httppost.addHeader("Content-type", "application/json; charset=utf-8");
httppost.setHeader("Accept", "application/json");
try {
StringEntity s = new StringEntity(json, Charset.forName("UTF-8"));
s.setContentEncoding("UTF-8");
httppost.setEntity(s);
HttpResponse response = httpclient.execute(httppost);
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK){
// 获取相应实体
HttpEntity entity = response.getEntity();
inputStream = entity.getContent();
ByteArrayOutputStream outStream = new ByteArrayOutputStream();
// 创建一个Buffer字符串
byte[] buffer = new byte[1024];
// 每次读取的字符串长度,如果为-1,代表全部读取完毕
int len = 0;
// 使用一个输入流从buffer里把数据读取出来
while ((len = inputStream.read(buffer)) != -1) {
// 用输出流往buffer里写入数据,中间参数代表从哪个位置开始读,len代表读取的长度
outStream.write(buffer, 0, len);
}
// 关闭输入流
inputStream.close();
// 把outStream里的数据写入内存
byteArrayInputStream = new ByteArrayInputStream(outStream.toByteArray());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return byteArrayInputStream;
}
}
@Override
public Map geterWeiMaHeC(Integer id,String type) {
Map a = new HashMap();
String s = ImgUtil.getUnlimited(id,type);
a.put("image",s);
return a;
}
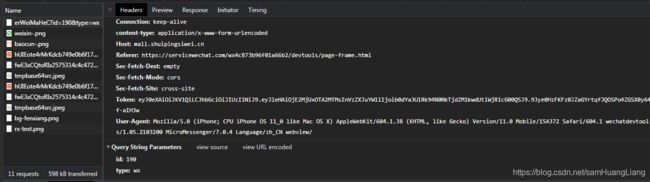
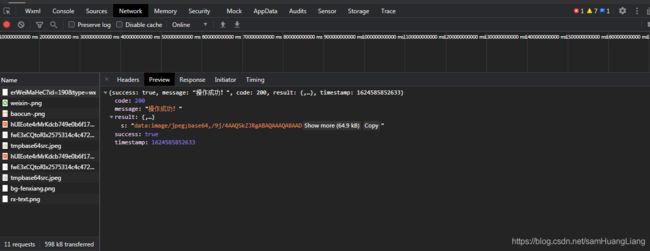
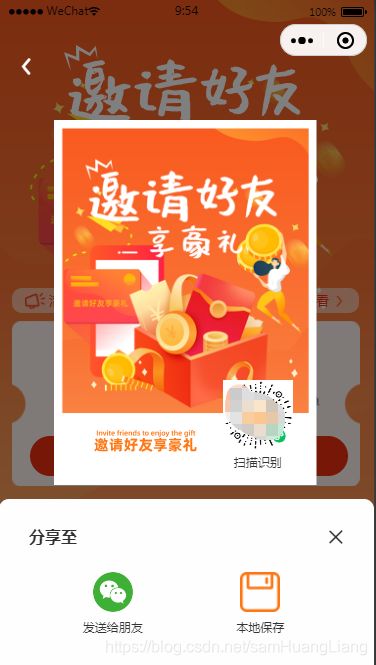