import cv2
import numpy as np
img = cv2.imread("image.jpg")
r1 = cv2.GaussianBlur(img,(7,7),0,0)
gray = cv2.cvtColor(r1,cv2.COLOR_BGR2GRAY)
t,r = cv2.threshold(gray,30,255,cv2.THRESH_BINARY)
kernel = np.ones((5,5),np.uint8)
erosion = cv2.erode(r,kernel,iterations=10)
dilation = cv2.dilate(erosion,kernel,iterations=10)
contours, hierarchy =cv2.findContours(dilation,cv2.RETR_LIST,cv2.CHAIN_APPROX_SIMPLE)
x,y,w,h = cv2.boundingRect(contours[0])
brcnt = np.array([[[x,y]],[[x+w,y]],[[x+w,y+h]],[[x,y+h]]])
cv2.drawContours(img,[brcnt],-1,(255,255),2)
rect = cv2.minAreaRect(contours[0])
point = cv2.boxPoints(rect)
point = np.int0(point)
cv2.drawContours(img,[point],-1,(255,255),2)
(q, p), radius = cv2.minEnclosingCircle(contours[0])
center = (int(q), int(p))
radius = int (radius)
cv2.circle(img, center, radius, (255, 255), 2)
ellipse = cv2.fitEllipse(contours[0])
cv2.ellipse(img, ellipse, (255, 255),2)
area, trl = cv2.minEnclosingTriangle(contours[0])
for i in range(0, 3):
p0 = trl[i, 0]
p1 = [int(j) for j in p0]
p3 = trl[(i + 1) % 3, 0]
p4 = [int(j) for j in p3]
cv2.line(img,tuple(p1),tuple(p4),(255,255),2)
cv2.imshow("result",img)
cv2.waitKey()
cv2.destroyAllWindows()
结果如下:
(1)原图
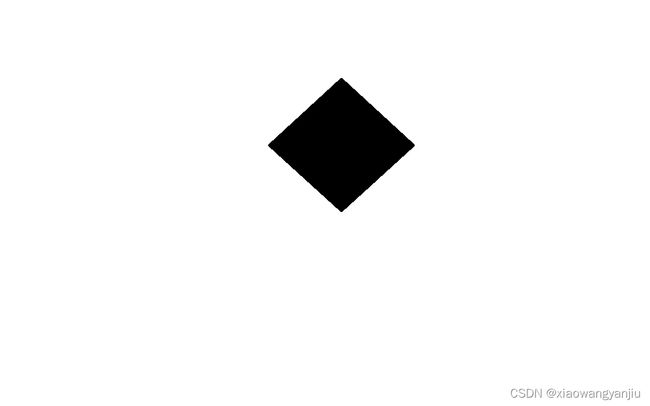
(2)各方法结果图(截取了结果的部分图,所以和原图尺寸不一样)
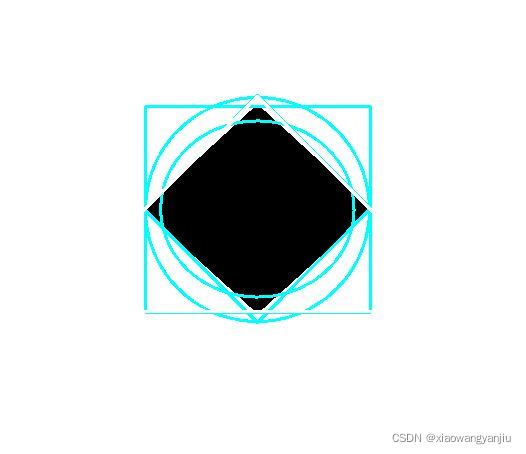