参考博客
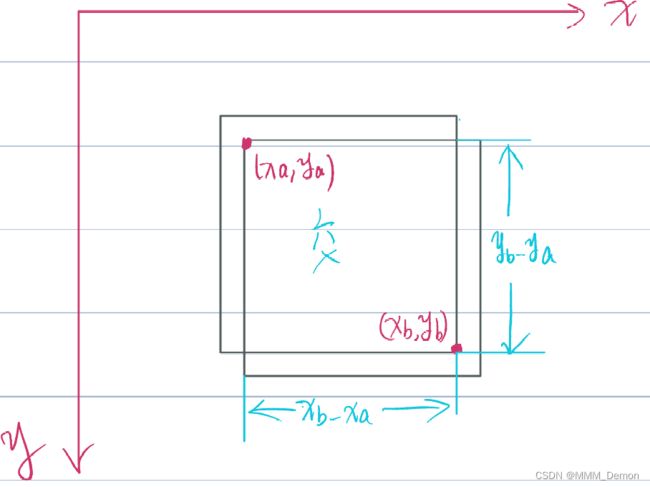
- for循环
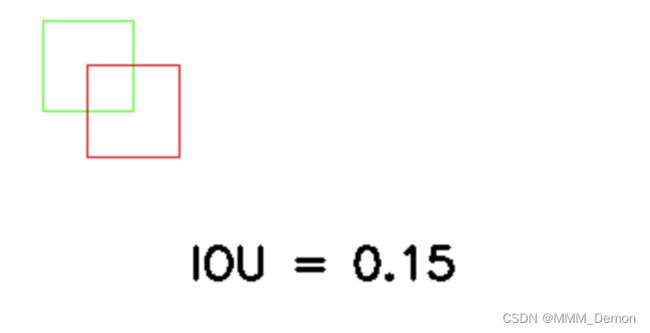
import cv2
import numpy as np
def CountIOU(recA, recB):
xA = max(recA[0], recB[0])
yA = max(recA[1], recB[1])
xB = min(recA[2], recA[2])
yB = min(recA[3], recA[3])
interArea = max(0, xB - xA + 1) * max(0, yB - yA + 1)
recA_Area = (recA[2] - recA[0] + 1) * (recA[3] - recA[1] + 1)
recB_Area = (recB[2] - recB[0] + 1) * (recB[3] - recB[1] + 1)
iou = interArea / float(recA_Area + recB_Area - interArea)
return iou
img = np.zeros((512, 512, 3))
img.fill(255)
recA = [50, 50, 99, 99]
recB = [74, 74, 124, 124]
cv2.rectangle(img, (recA[0], recA[1]), (recA[2], recA[3]), (0, 255, 0), 1)
cv2.rectangle(img, (recB[0], recB[1]), (recB[2], recB[3]), (0, 0, 255), 1)
IOU = CountIOU(recA, recB)
cv2.putText(img, "IOU = %.2f" % IOU, (130, 190), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 0, 0), 2)
cv2.imshow("img", img)
cv2.waitKey()
cv2.destroyAllWindows()
- 向量
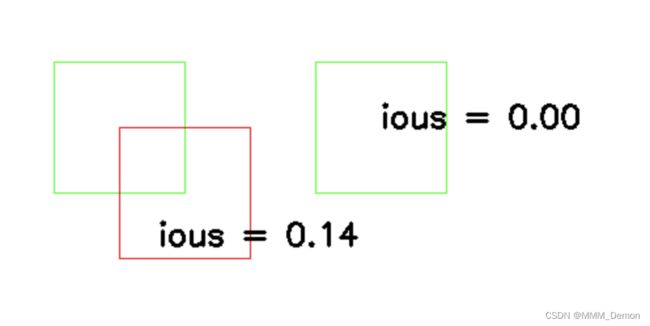
import cv2
import numpy as np
anchors = np.array([[50, 50, 150, 150],
[250, 50, 350, 150]])
ground_truth = np.array([[100, 100, 200, 200],
[400, 50, 500, 150]])
top_left = np.maximum(anchors[:, :2], ground_truth[:, :2])
bottom_right = np.minimum(anchors[:, 2:], ground_truth[:, 2:])
print('top_left:\n', top_left)
print('bottom_right:\n', bottom_right)
area_overlap = np.prod(bottom_right - top_left, axis=1) * (top_left < bottom_right).all(axis=1)
print('area_overlap:\n', area_overlap)
area_anchors = np.prod(anchors[:, 2:] - anchors[:, :2], axis=1)
print('area_anchors:\n', area_anchors)
area_ground_truth = np.prod(ground_truth[:, 2:] - ground_truth[:, :2], axis=1)
print('area_ground_truth:\n', area_ground_truth)
ious = (area_overlap / (area_anchors + area_ground_truth - area_overlap))
print('ious:\n', ious)
img = np.zeros((512, 512, 3))
img.fill(255)
for index in anchors:
cv2.rectangle(img, (index[0], index[1]), (index[2], index[3]), (0, 255, 0), 1)
cv2.rectangle(img, (ground_truth[0][0], ground_truth[0][1]), (ground_truth[0][2], ground_truth[0][3]), (0, 0, 255), 1)
cv2.putText(img, "ious = %.2f" % ious[0], (130, 190), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 0, 0), 2)
cv2.putText(img, "ious = %.2f" % ious[1], (300, 100), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 0, 0), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()