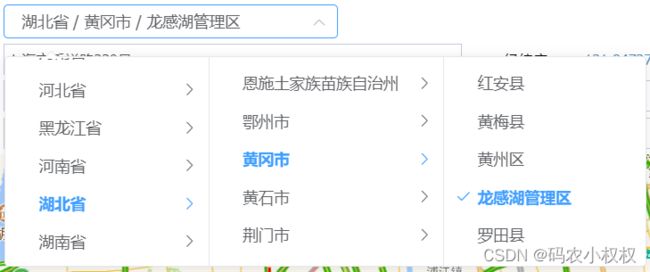
<template>
<div style="display: flex; align-items: flex-end; width: 100%" class="box">
<el-cascader
:key="new Date()"
ref="cascaderAddr"
:options="options"
:props="props"
:style="{ width: inputwidth + 'px' }"
v-model="chooseAddressList"
:placeholder="defaultaddress"
:collapse-tags="iscollapsetag"
@change="visibleChange"
></el-cascader>
<div v-if="isshowtitle" style="color: red; margin-left: 10px">
{{ title }}
</div>
</div>
</template>
<script>
import 'element-ui/lib/theme-chalk/index.css'
export default {
props: {
inputwidth: {
type: Number,
default: 260,
},
addresstype: {
type: Number,
default: 0,
},
addresslevel: {
type: String,
default: 'STREET',
},
isshowtitle: {
type: Boolean,
default: true,
},
currentaddresscode: {
type: String,
default: '',
},
currentaddresscodelist: {
type: Array,
default: [],
},
ismultiple: {
type: Boolean,
default: false,
},
iscollapsetag: {
type: Boolean,
default: false,
},
},
data() {
return {
options: [],
title: '',
chooseAddressList: [],
props: {},
}
},
mounted() {
this.getTitle()
},
methods: {
visibleChange() {
let areaInfo = this.$refs['cascaderAddr'].getCheckedNodes()
if (areaInfo.length !== 0) {
if (this.addresstype !== 0 && areaInfo[0].level < this.addresstype) {
_.ui.notify({
type: 'warning',
message: this.getLevelInfo(),
})
this.chooseAddressList = []
this.$refs.cascaderAddr.dropDownVisible = false
console.log(this.$refs.cascaderAddr.dropDownVisible)
} else {
let addressInfo
if (this.ismultiple) {
addressInfo = []
areaInfo.forEach((e) => {
addressInfo.push(e.data)
})
} else {
addressInfo = areaInfo[0].data
}
if (this.ismultiple) {
addressInfo.forEach((e, i) => {
e.parentList = []
areaInfo[i].pathNodes.forEach((t, j) => {
if (j < areaInfo[i].pathNodes.length - 1)
e.parentList.push(t.data)
})
})
} else {
addressInfo.parentList = []
areaInfo[0].pathNodes.forEach((t, j) => {
if (j < areaInfo[0].pathNodes.length - 1)
addressInfo.parentList.push(t.data)
})
}
this.$emit('getaddressinfo', addressInfo)
console.log(
this.chooseAddressList,
areaInfo,
addressInfo,
'selectedOptions'
)
}
}
},
handleChange(value) {
},
init() {
console.log(this.currentaddresscode)
let that = this
if (this.ismultiple) {
this.props = {
checkStrictly: true,
value: 'code',
label: 'name',
multiple: true,
}
} else {
this.props = { checkStrictly: false, value: 'code', label: 'name' }
}
_.util.ajax_post_factory(
'gw/gis-district-serve/district/getRegionNode',
{
parentCode: '0',
level: this.addresslevel,
},
function (res) {
if (res.success == true) {
that.options = res.data
if (
that.currentaddresscode !== '' &&
that.currentaddresscode !== undefined
) {
that.chooseAddressList = that.getTreeDeepArr(
that.currentaddresscode,
that.options
)
} else {
that.chooseAddressList = []
}
if (that.ismultiple) {
if (that.currentaddresscodelist.length !== 0) {
that.chooseAddressList = []
that.currentaddresscodelist.forEach((e) => {
that.chooseAddressList.push(
that.getTreeDeepArr(e, that.options)
)
})
} else {
that.chooseAddressList = []
}
}
}
}
)
},
getTitle() {
switch (this.addresstype) {
case 4:
this.title = '(省、市、区、街道为必选项)'
break
case 3:
this.title = '(省、市、区为必选项)'
break
case 2:
this.title = '(省、市为必选项)'
break
}
},
getLevelInfo() {
switch (this.addresstype) {
case 4:
return '请选择省、市、区、街道'
case 3:
return '请选择省、市、区'
case 2:
return '请选择省、市'
}
},
getTreeDeepArr(key, treeData) {
let arr = []
let returnArr = []
let depth = 0
function childrenEach(childrenData, depthN) {
for (var j = 0; j < childrenData.length; j++) {
depth = depthN
arr[depthN] = childrenData[j].code
if (childrenData[j].code == key) {
returnArr = arr.slice(0, depthN + 1)
break
} else {
if (childrenData[j].children) {
depth++
childrenEach(childrenData[j].children, depth)
}
}
}
return returnArr
}
return childrenEach(treeData, depth)
},
},
}
</script>
<style>
.el-cascader-panel .el-radio {
width: 100%;
height: 100%;
z-index: 10;
position: absolute;
top: 10px;
right: 10px;
}
.el-cascader-panel .el-radio__input {
visibility: hidden;
}
.el-cascader-panel .el-cascader-node__postfix {
top: 10px;
}
.el-cascader .el-input .el-input__inner {
height: 30px;
}
</style>