- Spring 生态创新应用:微服务架构设计与前沿技术融合实践
七夜zippoe
#Javaspring微服务java
在数字化转型的深水区,企业级应用正面临从“单体架构”向“分布式智能架构”的根本性跃迁。Spring生态以其二十年技术沉淀形成的生态壁垒,已成为支撑这场变革的核心基础设施。从2002年RodJohnson发布《ExpertOne-on-OneJ2EEDesignandDevelopment》奠定的理论基础,到如今覆盖从开发到运维全链路的技术矩阵,Spring始终以“简化开发”为初心,构建出适配不同业
- 排序的艺术:Spring Data JPA 如何玩转关联实体排序 (. 运算符的奥秘) ✨
小丁学Java
SpringDataJPAjpa
这次我们来深入探讨SpringDataJPA分页排序中一个非常实用但又容易混淆的技巧:如何优雅地对关联实体(或嵌套属性)进行排序。排序的艺术:SpringDataJPA如何玩转关联实体排序(.运算符的奥秘)你好,我是坚持哥!在构建Web应用时,分页查询是家常便饭。SpringDataJPA(JavaPersistenceAPI)提供了强大的Pageable接口,让分页和排序变得异常简单。但当你的排
- Hutool TreeUtil快速构建树形数据结构
yifanghub
工具类java
在管理菜单、部门结构等场景时,我们经常需要将数据库中的层级数据转换为树形结构。本文将通过Hutool的TreeUtil工具类,实现零递归快速构建树形结构。一、环境准备JDK1.8+SpringBoot2.xHutool5.8.16MySQL8.0二、数据准备--创建部门表CREATETABLE`sys_dept`(`id`intNOTNULLAUTO_INCREMENT,`dept_name`va
- 4.服务注册发现:微服务的神经系统
在微服务架构中,服务之间不再是固定连接,而是高度动态、短暂存在的。如何让每个服务准确找到彼此,是分布式系统治理的核心问题之一。服务注册发现机制,正如神经系统之于人体,承担着连接、协调、感知变化的关键角色。本文将围绕Netflix开源的服务注册发现组件Eureka展开,深入剖析其原理,并以SpringCloud实战为导向,帮助你掌握服务治理的第一步。一、为什么需要服务注册发现?在单体架构中,服务调用
- 2.Spring Cloud生态全景解析:核心组件、能力边界与定位
碎风影
SpringCloud深度解析springcloudspring后端
导语:SpringCloud并非单一框架,而是基于SpringBoot构建的分布式系统工具集。它通过标准化封装,将服务发现、配置管理、熔断限流等复杂基础设施转化为开箱即用的组件,让开发者聚焦业务逻辑。本文将系统解析其核心组成、与SpringBoot的共生关系,并客观审视其能力边界,助您构建清晰的微服务技术选型地图。一、核心基石:SpringBoot与SpringCloud的共生关系关键结论:Spr
- SpringBoot3+JPA+MySQL实现多数据源的读写分离(基于EntityManagerFactory)
没刮胡子
java软件开发技术实战专栏SpringBoot3JPAMySQL多数据源读写分离
1、简介在SpringBoot中配置多个数据源并实现自动切换EntityManager,这里我编写了一个RoutingEntityManagerFactory和AOP(面向切面编程)的方式来实现。这里我配置了两个数据源:primary和secondary,其中primary主数据源用来写入数据,secondary从数据源用来读取数据。注意1:使用Springboot3的读写分离,首先要保证主库和从
- 微服务世界的“导航仪”!Spring Cloud五大注册中心选型指南,从此不再迷路!
码农技术栈
微服务微服务springcloud架构springbootjava后端
引言:为什么微服务需要“导航仪”?想象一下,你走进一座巨大的迷宫(微服务集群),里面有成百上千个房间(服务实例),每个房间都在动态变化位置(服务扩缩容)。注册中心就像迷宫里的导航仪,实时记录所有房间的位置,告诉你怎么最快找到目标。没有它?你可能会永远迷失在“服务调用”的迷宫里!注册中心的核心作用服务注册:服务启动时,主动上报自己的地址和状态。服务发现:调用方通过注册中心查询目标服务的位置。健康监测
- spring-data-jpa+spring+hibernate+druid配置
参考链接:http://doc.okbase.net/liuyitian/archive/109276.htmlhttp://my.oschina.net/u/1859292/blog/312188最新公司的web项目需要用到spring-data-jpa作为JPA的实现框架,同时使用阿里巴巴的开源数据库连接池druid。关于这两种框架的介绍我在这里就不多赘述。直接进入配置页面:spring的配置
- Spring Data Jpa +alibaba druid+query dsl 实现多数据源
下海揽月
springdatajpajava
SpringDataJpa+alibabadruid+querydsl实现多数据源,主要通过配置来实现多个数据源的操作,无需动态切换1.maven配置org.springframework.bootspring-boot-starter-data-jpa2.3.12.RELEASEcom.alibabadruid-spring-boot-starter1.1.24com.querydslquery
- Spring Boot + Spring JPA + JDBC + Druid实现动态数据源切换
Apr01Chell
代码片段springjava数据库
SpringBoot+SpringJPA+JDBC+Druid实现动态数据源切换目录SpringBoot+SpringJPA+JDBC+Druid实现动态数据源切换AbstractRoutingDataSource源码分析需求代码实现DynamicDataSourceDBContextHolderDruidDbConfigDataSourcePropertiesAllDataSourcesExec
- Spring框架中的Component与Bean注解
SpringBoot中的@Bean与@Component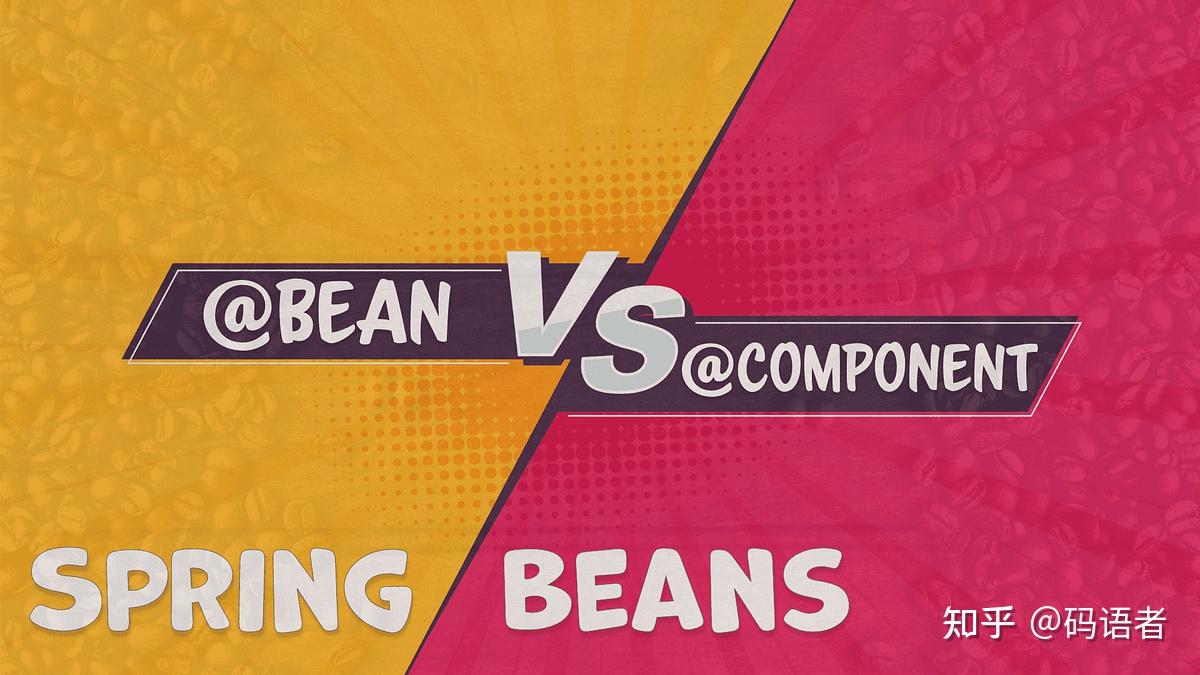Spring的@Component和@Bean注解的关键区别在于:@Bean注解可用于暴露您自己编写的JavaBeans,而@Component注解可用于暴露源代码由他人维护的JavaBeans。Sprin
- Flex与Spring集成
hkmw
Flex配置springflexapplicationdependenciescomponentsaccess
Flex与Spring集成UsingFlexwithSpringUPDATE(1/12/2007):IputtogetheraTomcat-basedTestDriveServerthatincludesthesamplesdescribedbelowrunningout-of-thebox.Readthispostformoreinfo.WhatisSpring?Springisoneofthe
- 【视频观看系统】- 技术与架构选型
✅项目技术选型方案一、整体架构风格项目层级技术选型说明架构风格微服务架构(SpringCloud)独立部署、易扩展、易维护服务通信HTTP(RestTemplate或Feign)+RocketMQ同步调用+异步事件注册中心Nacos服务注册、发现、配置中心配置中心Nacos配置管理多服务统一配置API网关SpringCloudGateway路由转发、权限验证、限流服务监控SpringBootAdm
- SpringBoot ThreadLocal 全局动态变量设置
xdscode
springbootjavaThreadLocal
需求说明:现有一个游戏后台管理系统,该系统可管理多个大区的数据,但是需要使用大区id实现数据隔离,并且提供了大区选择功能,先择大区后展示对应的数据。需要实现一下几点:1.前端请求时,area_id是必传的1.数据隔离,包括查询及增删改:使用mybatis拦截器实现2.多个用户同时操作互不影响3.非前端调用场景的处理:定时任务、mq1.前端决定area_id为了解决多个用户可以互不影响的使用不同的a
- 实操 SpringBoot+MCP!
清风孤客
springboot后端java人工智能
引言随着人工智能的飞速发展,大语言模型(LLM)正在革命性地重塑用户与软件的交互范式。想象一下这样的场景:用户无需钻研复杂的API文档或者在繁琐的表单间来回切换,只需通过自然语言直接与系统对话——“帮我查找所有2023年出版的图书”、“创建一个新用户叫张三,邮箱是
[email protected]”。这种直观、流畅的交互方式不仅能显著降低新用户的学习曲线,更能大幅削减B端系统的培训成本和实施
- 【SpringBoot】Spring Boot 高并发优化终极指南,涵盖线程模型、JVM 调优、数据库访问、缓存策略等 15+ 核心模块
夜雨hiyeyu.com
javaspringbootjvmspringjava后端性能优化系统架构
SpringBoot高并发优化终极指南,涵盖线程模型、JVM调优、数据库访问、缓存策略等15+核心模块一、线程模型深度调优(核心瓶颈突破)1.Tomcat线程池原子级配置2.异步任务线程池隔离策略二、JVM层终极调参(G1GC深度优化)1.内存分配策略2.GC日志分析技巧三、缓存策略原子级优化1.三级缓存架构实现2.缓存穿透/雪崩防护四、数据库访问极致优化1.连接池死亡参数配置2.分页查询深度优化
- SpringBoot+AOP+自定义注解,实现日志记录
一.定义自定义注解importjava.lang.annotation.*;/***@authorawen*定义注解目的想让他当作切点*/@Target({ElementType.METHOD})@Retention(RetentionPolicy.RUNTIME)//.java.class字节码@Documentedpublic@interfaceLog{/***处理类型**@return{@l
- spring boot使用mybatis-plus实现分页功能
kong@react
springbootmybatis后端
使用MyBatis-Plus实现分页功能MyBatis-Plus提供了内置的分页插件,可以轻松实现分页查询功能。以下是实现分页的具体方法:配置分页插件在SpringBoot项目中,需要在配置类中注册分页插件:@ConfigurationpublicclassMybatisPlusConfig{@BeanpublicMybatisPlusInterceptormybatisPlusIntercept
- springboot通过aop实现全局日志(是否自定义注解都可以)
甜无能
springbootjava#aopspringbootjavaaop全局日志自定义注解
内容参考自以下两个链接1、springboot中使用AOP切面完成全局日志_aop全局日志_邹飞鸣的博客-CSDN博客使用AOP记录日志_aop日志_trusause的博客-CSDN博客第一个链接思路很清晰,讲的也很详细,第二个链接讲了自定义注解为了便于自己理解做了以下整理目录1.aspectj基本概念2.添加aop依赖3.进行切面处理(1)切面类(2)自定义注解(3)controller和ser
- SpringBoot AOP+注解 全局日志记录
xdscode
springbootjavaAOP
一、需求描述如何优雅地记录用户操作日志?网站后台,功能开发完成后,新增了一个需求,即需要记录用户的各种操作记录。由于是在开发后期,如果针对每一个功能都去添加一段记录日志的代码,工作量较大、代码侵入性太强,因此采用AOP+注解的方式实现。可读性大大提高,且便于维护和扩展。AOP:面向切面编程,在不修改现有逻辑代码的情况下,增强功能,恰好体现了spring的理念:无入侵式自定义注解:当被注解的方法执行
- DolphinScheduler 3.2.0 Master启动核心源码解析
目录1.手动调度工作流触发原理2.MasterServer启动入口与整体流程3.MasterRPC服务启动3.1启动RPCServer3.2启动RPCClient4.插件加载机制5.注册中心客户端初始化与心跳维护6.核心调度引擎启动6.1恢复Command6.2事件循环6.3任务派发7.事件处理服务8.故障转移线程8.1MasterFailover8.2WorkerFailover9.Quartz
- C#实现SVM支持向量机(附完整源码)
源代码大师
C#实战教程c#支持向量机开发语言
C#实现SVM支持向量机下面是使用C#实现支持向量机(SVM)的示例代码:usingSystem;usingAccord.MachineLearning.VectorMachines;usingAccord.MachineLearning.VectorMachines.Learning;usingAccord
- Spring Bean 生命周期 SmartLifecycle接口介绍和使用场景 和 Lifecycle对比
极光雨雨
#Spring全家springjava
在SpringBoot中,SmartLifecycle是org.springframework.context.Lifecycle接口的一个扩展接口,它提供了更细粒度的控制生命周期的方法。Spring容器管理Bean的生命周期时,可以通过实现SmartLifecycle接口来定义自定义的启动和关闭逻辑。一、使用前提需要在Spring容器启动完成后执行某些初始化操作。需要在应用关闭前做一些清理工作(
- Java程序设计(二十七):基于SSM框架的OA办公自动化管理平台的设计与实现
人工智能_SYBH
2025年java程序设计java数据挖掘开发语言vue.js后端人工智能springboot
1.项目概述办公自动化(OA,OfficeAutomation)管理平台是企业实现内部管理信息化的重要工具。本文提出并实现了一个基于Java的OA办公自动化管理平台。该平台基于SSM架构(Spring+SpringMVC+MyBatis),数据库采用MySQL,并通过HTML、CSS、JavaScript等技术实现用户界面。1.1平台功能简介平台提供了管理员、普通用户和部门三类角色,分别具有不同的
- Android网络层架构:统一错误处理的问题分析到解决方案与设计实现
wzj_what_why_how
Android#Android——架构和设计android架构
前言在Android项目开发中,我们经常遇到需要统一处理某些特定状态码的场景。本文分享一个项目中遇到的4406状态码(实名认证)处理不统一问题,从问题分析到完整解决方案,提供一套可复用的架构设计模式。目录前言问题分析不同框架的回调处理机制解决方案关键技术细节添加应用拦截器循环依赖问题与回调接口模式问题分析解决方案:回调接口模式ResponseBody流管理问题现象原因总结源码分析总结其设计原理重复
- CTFSHOW-WEB-36D杯
wyjcxyyy
前端android
给你shell这道题对我这个新手还是有难度的,花了不少时间。首先f12看源码,看到?view_source,点进去看源码location.href=\'./index.php\'');if(!isset($_GET['code'])){show_source(__FILE__);exit();}else{$code=$_GET['code'];if(!preg_match($secret_waf,
- firecrawl本地docker部署(WSL虚拟机Ubuntu24)
firecrawl本地docker部署下载源码github下载地址部署按照firecrawl目录下SELF_HOST.md文档进行操作即可。本次生成的镜像在后面提供了百度网盘下载。创建.env文件将firecrawl\apps\api.env.example文件拷贝到firecrawl目录下(和docker-compose.yaml同一目录下),修改文件名为.env#=====RequiredEN
- 【Java源码阅读系列44】深度解读Java NIO ByteBuffer 源码
·云扬·
源码阅读系列之Javajavanio开发语言
JavaNIO(NewInput/Output)中的ByteBuffer是Buffer抽象类的具体子类,专门用于处理字节数据的高效读写。作为NIO的核心组件,ByteBuffer支持堆内存(Heap)和直接内存(Direct)两种存储方式,广泛应用于网络通信、文件IO等场景。本文将结合源码,深入解析ByteBuffer的核心机制、关键方法及设计模式的应用。一、ByteBuffer的核心特性与存储方
- OpenWebUI(8)源码学习-后端utils/telemetry追踪遥测模块
目录目录结构说明`constants.py`核心作用:主要功能:示例代码片段:`exporters.py`核心作用:主要类:`LazyBatchSpanProcessor`特点:技术亮点:`instrumentors.py`核心作用:插桩对象包括:钩子函数(Hooks):Instrumentor类:插桩流程:`setup.py`核心作用:主要功能:典型调用方式:✨总体架构与价值技术亮点总结✅开发建
- 【人工智能】Spring AI Alibaba,一个面向 Java 开发者的开源框架,它旨在简化将人工智能(AI)功能集成到应用程序中的过程。
本本本添哥
A-AIGC人工智能大模型人工智能javaspring
一、SpringAIAlibaba介绍SpringAIAlibaba是一个面向Java开发者的开源框架,它旨在简化将人工智能(AI)功能集成到应用程序中的过程。该项目基于SpringAI构建,并且是阿里云通义系列模型及服务在JavaAI应用开发领域的最佳实践。SpringAIAlibaba的目标是为开发者提供一套高层次的AIAPI抽象以及与云原生基础设施的深度集成方案,从而帮助他们快速构建智能应用
- 用MiddleGenIDE工具生成hibernate的POJO(根据数据表生成POJO类)
AdyZhang
POJOeclipseHibernateMiddleGenIDE
推荐:MiddlegenIDE插件, 是一个Eclipse 插件. 用它可以直接连接到数据库, 根据表按照一定的HIBERNATE规则作出BEAN和对应的XML ,用完后你可以手动删除它加载的JAR包和XML文件! 今天开始试着使用
- .9.png
Cb123456
android
“点九”是andriod平台的应用软件开发里的一种特殊的图片形式,文件扩展名为:.9.png
智能手机中有自动横屏的功能,同一幅界面会在随着手机(或平板电脑)中的方向传感器的参数不同而改变显示的方向,在界面改变方向后,界面上的图形会因为长宽的变化而产生拉伸,造成图形的失真变形。
我们都知道android平台有多种不同的分辨率,很多控件的切图文件在被放大拉伸后,边
- 算法的效率
天子之骄
算法效率复杂度最坏情况运行时间大O阶平均情况运行时间
算法的效率
效率是速度和空间消耗的度量。集中考虑程序的速度,也称运行时间或执行时间,用复杂度的阶(O)这一标准来衡量。空间的消耗或需求也可以用大O表示,而且它总是小于或等于时间需求。
以下是我的学习笔记:
1.求值与霍纳法则,即为秦九韶公式。
2.测定运行时间的最可靠方法是计数对运行时间有贡献的基本操作的执行次数。运行时间与这个计数成正比。
- java数据结构
何必如此
java数据结构
Java 数据结构
Java工具包提供了强大的数据结构。在Java中的数据结构主要包括以下几种接口和类:
枚举(Enumeration)
位集合(BitSet)
向量(Vector)
栈(Stack)
字典(Dictionary)
哈希表(Hashtable)
属性(Properties)
以上这些类是传统遗留的,在Java2中引入了一种新的框架-集合框架(Collect
- MybatisHelloWorld
3213213333332132
//测试入口TestMyBatis
package com.base.helloworld.test;
import java.io.IOException;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibat
- Java|urlrewrite|URL重写|多个参数
7454103
javaxmlWeb工作
个人工作经验! 如有不当之处,敬请指点
1.0 web -info 目录下建立 urlrewrite.xml 文件 类似如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE u
- 达梦数据库+ibatis
darkranger
sqlmysqlibatisSQL Server
--插入数据方面
如果您需要数据库自增...
那么在插入的时候不需要指定自增列.
如果想自己指定ID列的值, 那么要设置
set identity_insert 数据库名.模式名.表名;
----然后插入数据;
example:
create table zhabei.test(
id bigint identity(1,1) primary key,
nam
- XML 解析 四种方式
aijuans
android
XML现在已经成为一种通用的数据交换格式,平台的无关性使得很多场合都需要用到XML。本文将详细介绍用Java解析XML的四种方法。
XML现在已经成为一种通用的数据交换格式,它的平台无关性,语言无关性,系统无关性,给数据集成与交互带来了极大的方便。对于XML本身的语法知识与技术细节,需要阅读相关的技术文献,这里面包括的内容有DOM(Document Object
- spring中配置文件占位符的使用
avords
1.类
<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.o
- 前端工程化-公共模块的依赖和常用的工作流
bee1314
webpack
题记: 一个人的项目,还有工程化的问题嘛? 我们在推进模块化和组件化的过程中,肯定会不断的沉淀出我们项目的模块和组件。对于这些沉淀出的模块和组件怎么管理?另外怎么依赖也是个问题? 你真的想这样嘛? var BreadCrumb = require(‘../../../../uikit/breadcrumb’); //真心ugly。
- 上司说「看你每天准时下班就知道你工作量不饱和」,该如何回应?
bijian1013
项目管理沟通IT职业规划
问题:上司说「看你每天准时下班就知道你工作量不饱和」,如何回应
正常下班时间6点,只要是6点半前下班的,上司都认为没有加班。
Eno-Bea回答,注重感受,不一定是别人的
虽然我不知道你具体从事什么工作与职业,但是我大概猜测,你是从事一项不太容易出现阶段性成果的工作
- TortoiseSVN,过滤文件
征客丶
SVN
环境:
TortoiseSVN 1.8
配置:
在文件夹空白处右键
选择 TortoiseSVN -> Settings
在 Global ignote pattern 中添加要过滤的文件:
多类型用英文空格分开
*name : 过滤所有名称为 name 的文件或文件夹
*.name : 过滤所有后缀为 name 的文件或文件夹
--------
- 【Flume二】HDFS sink细说
bit1129
Flume
1. Flume配置
a1.sources=r1
a1.channels=c1
a1.sinks=k1
###Flume负责启动44444端口
a1.sources.r1.type=avro
a1.sources.r1.bind=0.0.0.0
a1.sources.r1.port=44444
a1.sources.r1.chan
- The Eight Myths of Erlang Performance
bookjovi
erlang
erlang有一篇guide很有意思: http://www.erlang.org/doc/efficiency_guide
里面有个The Eight Myths of Erlang Performance: http://www.erlang.org/doc/efficiency_guide/myths.html
Myth: Funs are sl
- java多线程网络传输文件(非同步)-2008-08-17
ljy325
java多线程socket
利用 Socket 套接字进行面向连接通信的编程。客户端读取本地文件并发送;服务器接收文件并保存到本地文件系统中。
使用说明:请将TransferClient, TransferServer, TempFile三个类编译,他们的类包是FileServer.
客户端:
修改TransferClient: serPort, serIP, filePath, blockNum,的值来符合您机器的系
- 读《研磨设计模式》-代码笔记-模板方法模式
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
- 配置心得
chenyu19891124
配置
时间就这样不知不觉的走过了一个春夏秋冬,转眼间来公司已经一年了,感觉时间过的很快,时间老人总是这样不停走,从来没停歇过。
作为一名新手的配置管理员,刚开始真的是对配置管理是一点不懂,就只听说咱们公司配置主要是负责升级,而具体该怎么做却一点都不了解。经过老员工的一点点讲解,慢慢的对配置有了初步了解,对自己所在的岗位也慢慢的了解。
做了一年的配置管理给自总结下:
1.改变
从一个以前对配置毫无
- 对“带条件选择的并行汇聚路由问题”的再思考
comsci
算法工作软件测试嵌入式领域模型
2008年上半年,我在设计并开发基于”JWFD流程系统“的商业化改进型引擎的时候,由于采用了新的嵌入式公式模块而导致出现“带条件选择的并行汇聚路由问题”(请参考2009-02-27博文),当时对这个问题的解决办法是采用基于拓扑结构的处理思想,对汇聚点的实际前驱分支节点通过算法预测出来,然后进行处理,简单的说就是找到造成这个汇聚模型的分支起点,对这个起始分支节点实际走的路径数进行计算,然后把这个实际
- Oracle 10g 的clusterware 32位 下载地址
daizj
oracle
Oracle 10g 的clusterware 32位 下载地址
http://pan.baidu.com/share/link?shareid=531580&uk=421021908
http://pan.baidu.com/share/link?shareid=137223&uk=321552738
http://pan.baidu.com/share/l
- 非常好的介绍:Linux定时执行工具cron
dongwei_6688
linux
Linux经过十多年的发展,很多用户都很了解Linux了,这里介绍一下Linux下cron的理解,和大家讨论讨论。cron是一个Linux 定时执行工具,可以在无需人工干预的情况下运行作业,本文档不讲cron实现原理,主要讲一下Linux定时执行工具cron的具体使用及简单介绍。
新增调度任务推荐使用crontab -e命令添加自定义的任务(编辑的是/var/spool/cron下对应用户的cr
- Yii assets目录生成及修改
dcj3sjt126com
yii
assets的作用是方便模块化,插件化的,一般来说出于安全原因不允许通过url访问protected下面的文件,但是我们又希望将module单独出来,所以需要使用发布,即将一个目录下的文件复制一份到assets下面方便通过url访问。
assets设置对应的方法位置 \framework\web\CAssetManager.php
assets配置方法 在m
- mac工作软件推荐
dcj3sjt126com
mac
mac上的Terminal + bash + screen组合现在已经非常好用了,但是还是经不起iterm+zsh+tmux的冲击。在同事的强烈推荐下,趁着升级mac系统的机会,顺便也切换到iterm+zsh+tmux的环境下了。
我为什么要要iterm2
切换过来也是脑袋一热的冲动,我也调查过一些资料,看了下iterm的一些优点:
* 兼容性好,远程服务器 vi 什么的低版本能很好兼
- Memcached(三)、封装Memcached和Ehcache
frank1234
memcachedehcachespring ioc
本文对Ehcache和Memcached进行了简单的封装,这样对于客户端程序无需了解ehcache和memcached的差异,仅需要配置缓存的Provider类就可以在二者之间进行切换,Provider实现类通过Spring IoC注入。
cache.xml
<?xml version="1.0" encoding="UTF-8"?>
- Remove Duplicates from Sorted List II
hcx2013
remove
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.
For example,Given 1->2->3->3->4->4->5,
- Spring4新特性——注解、脚本、任务、MVC等其他特性改进
jinnianshilongnian
spring4
Spring4新特性——泛型限定式依赖注入
Spring4新特性——核心容器的其他改进
Spring4新特性——Web开发的增强
Spring4新特性——集成Bean Validation 1.1(JSR-349)到SpringMVC
Spring4新特性——Groovy Bean定义DSL
Spring4新特性——更好的Java泛型操作API
Spring4新
- MySQL安装文档
liyong0802
mysql
工作中用到的MySQL可能安装在两种操作系统中,即Windows系统和Linux系统。以Linux系统中情况居多。
安装在Windows系统时与其它Windows应用程序相同按照安装向导一直下一步就即,这里就不具体介绍,本文档只介绍Linux系统下MySQL的安装步骤。
Linux系统下安装MySQL分为三种:RPM包安装、二进制包安装和源码包安装。二
- 使用VS2010构建HotSpot工程
p2p2500
HotSpotOpenJDKVS2010
1. 下载OpenJDK7的源码:
http://download.java.net/openjdk/jdk7
http://download.java.net/openjdk/
2. 环境配置
▶
- Oracle实用功能之分组后列合并
seandeng888
oracle分组实用功能合并
1 实例解析
由于业务需求需要对表中的数据进行分组后进行合并的处理,鉴于Oracle10g没有现成的函数实现该功能,且该功能如若用JAVA代码实现会比较复杂,因此,特将SQL语言的实现方式分享出来,希望对大家有所帮助。如下:
表test 数据如下:
ID,SUBJECTCODE,DIMCODE,VALUE
1&nbs
- Java定时任务注解方式实现
tuoni
javaspringjvmxmljni
Spring 注解的定时任务,有如下两种方式:
第一种:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http
- 11大Java开源中文分词器的使用方法和分词效果对比
yangshangchuan
word分词器ansj分词器Stanford分词器FudanNLP分词器HanLP分词器
本文的目标有两个:
1、学会使用11大Java开源中文分词器
2、对比分析11大Java开源中文分词器的分词效果
本文给出了11大Java开源中文分词的使用方法以及分词结果对比代码,至于效果哪个好,那要用的人结合自己的应用场景自己来判断。
11大Java开源中文分词器,不同的分词器有不同的用法,定义的接口也不一样,我们先定义一个统一的接口:
/**
* 获取文本的所有分词结果, 对比