此实现工分三个文件,即:CreatImage.java 生成图像流的类,提供用于生成图片流的方法;images.jsp 图片包装,用于将图片加入到response输出流中;login.jsp 调用前面的jsp,显示验证码。
下面是源代码:
1
package
com;
2
3
import
java.awt.Color;
4
import
java.awt.Font;
5
import
java.awt.Graphics;
6
import
java.awt.Image;
7
import
java.awt.image.BufferedImage;
8
import
java.util.Random;
9
10
import
javax.servlet.http.HttpServletRequest;
11
import
javax.servlet.http.HttpServletResponse;
12
import
javax.servlet.http.HttpSession;
13
14
public
class
CreatImage{
15
16
private
static
final
long
serialVersionUID
=
1L
;
17
Image image
=
null
;
18
HttpServletRequest request
=
null
;
19
HttpServletResponse response
=
null
;
20
21
public
CreatImage(HttpServletRequest request, HttpServletResponse response){
22
this
.request
=
request;
23
this
.response
=
response;
24
}
25
26
public
Color getRandColor(
int
fc,
int
bc){
//
给定范围获得随机颜色
27
Random random
=
new
Random();
28
if
(fc
>
255
) fc
=
255
;
29
if
(bc
>
255
) bc
=
255
;
30
int
r
=
fc
+
random.nextInt(bc
-
fc);
31
int
g
=
fc
+
random.nextInt(bc
-
fc);
32
int
b
=
fc
+
random.nextInt(bc
-
fc);
33
return
new
Color(r,g,b);
34
}
35
36
public
BufferedImage creatImage(){
37
int
width
=
100
, height
=
26
;
38
//
生成随机类
39
Random random
=
new
Random();
40
BufferedImage image
=
new
BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
41
//
获取图形上下文
42
Graphics g
=
image.getGraphics();
43
//
设定背景色
44
g.setColor(getRandColor(
200
,
250
));
45
g.fillRect(
0
,
0
, width, height);
46
//
设定字体
47
g.setFont(
new
Font(
"
Times New Roman
"
,Font.PLAIN,
22
));
48
49
//
画边框
50
//
g.setColor(getRandColor(200,250));
51
//
g.drawRect(0,0,width,height);
52
53
//
随机产生155条干扰线,使图象中的认证码不易被其它程序探测到
54
g.setColor(getRandColor(
160
,
200
));
55
for
(
int
i
=
0
;i
<
155
;i
++
){
56
int
x
=
random.nextInt(width);
57
int
y
=
random.nextInt(height);
58
int
xl
=
random.nextInt(
12
);
59
int
yl
=
random.nextInt(
12
);
60
g.drawLine(x,y,x
+
xl,y
+
yl);
61
}
62
//
取随机产生的认证码(4位数字)
63
//
String rand = request.getParameter("rand");
64
//
rand = rand.substring(0,rand.indexOf("."));
65
String str1
=
randomStr(
4
);
//
得到随机字符
66
HttpSession session
=
request.getSession();
67
session.setAttribute(
"
validatenumber
"
,str1);
68
//
System.out.println(str1);
69
for
(
int
i
=
0
;i
<
4
;i
++
){
70
String rand
=
str1.substring(i,i
+
1
);
71
//
将认证码显示到图象中
72
g.setColor(
new
Color(
20
+
random.nextInt(
110
),
20
+
random.nextInt(
110
),
20
+
random.nextInt(
110
)));
73
//
调用函数出来的颜色相同,可能是因为种子太接近,所以只能直接生成
74
g.drawString(rand,
20
*
i
+
12
,
20
);
75
}
76
//
图象生效
77
g.dispose();
78
return
image;
79
}
80
81
//
得到随机字符
82
public
String randomStr(
int
n) {
83
String str1
=
"
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz1234567890
"
;
84
String str2
=
""
;
85
int
len
=
str1.length()
-
1
;
86
double
r;
87
for
(
int
i
=
0
; i
<
n; i
++
) {
88
r
=
(Math.random())
*
len;
89
str2
=
str2
+
str1.charAt((
int
) r);
90
}
91
return
str2;
92
}
93
}
1
<%
@ page language
=
"
java
"
contentType
=
"
image/jpeg ; charset=UTF-8
"
2
pageEncoding
=
"
UTF-8
"
import
=
"
java.awt.*,java.awt.image.*,javax.imageio.*,java.io.*
"
%>
3
<%
@ page import
=
"
com.CreatImage
"
%>
4
<!
DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"
>
5
<
html
>
6
<
head
>
7
<
meta
http-equiv
="Content-Type"
content
="text/html; charset=UTF-8"
>
8
<
title
>
Insert title here
</
title
>
9
</
head
>
10
<
body
>
11
<%
12
response.setHeader(
"
Pragma
"
,
"
No-cache
"
);
13
response.setHeader(
"
Cache-Control
"
,
"
no-cache
"
);
14
response.setDateHeader(
"
Expires
"
,
0
);
15
16
CreatImage c
=
new
CreatImage(request,response);
17
BufferedImage image
=
c.creatImage();
18
OutputStream b
=
response.getOutputStream();
19
%>
20
<%
21
ImageIO.write(image,
"
JPEG
"
, response.getOutputStream());
22
//
必须添加以下两行否则tomcat下jsp出现getOutputStream() has already been called
for
this response异常
23
out.clear();
24
out
=
pageContext.pushBody();
25
%>
26
</
body
>
27
</
html
>
注意 contentType="image/jpeg ; charset=UTF-8"
1
<%
@ page language
=
"
java
"
contentType
=
"
text/html; charset=UTF-8
"
2
pageEncoding
=
"
UTF-8
"
%>
3
<%
4
String
path
=
request.getContextPath();
5
%>
6
<!
DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"
>
7
<
html
>
8
<
head
>
9
<
meta
http-equiv
="Content-Type"
content
="text/html; charset=UTF-8"
>
10
<
title
>
Insert title here
</
title
>
11
<
script
>
12
function
changeCode()
13
{
14
document.getElementById(
"
cc
"
).src
=
"
<%=path%>/images.jsp?a=
"
+
Math.random();
15
}
16
</
script
>
17
</
head
>
18
<
body
>
19
<
form
action
=""
method
="post"
>
20
<
table
cellspacing
="2px"
cellpadding
="2px"
align
="center"
>
21
<
tr
>
22
<
td
>
用户名:
</
td
>
23
<
td
><
input
type
="text"
id
="name"
value
=""
/></
td
>
24
</
tr
>
25
<
tr
>
26
<
td
>
密
码:
</
td
>
27
<
td
><
input
type
="password"
id
="password"
value
=""
/></
td
>
28
</
tr
>
29
<
tr
height
="26px"
>
30
<
td
>
验证码:
</
td
><
td
valign
="middle"
><
input
type
="text"
id
="validate"
></
td
>
31
<
td
><
a
href
="#"
onclick
="changeCode();"
><
img
alt
=""
border
="0"
id
="cc"
src
="<%=path %>/images.jsp"
/></
a
></
td
>
32
</
tr
>
33
<
tr
>
34
<
td
colspan
="2"
align
="center"
><
input
type
="button"
name
="button"
value
="submit"
/></
td
>
35
</
tr
>
36
</
table
>
37
</
form
>
38
</
body
>
39
</
html
>
40
41
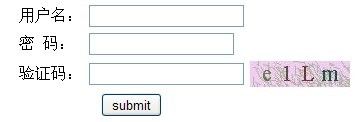
应各位的请求,我把效果图和源文件都放了上来,这样的实现方式我也是参考了别人的博客,不妥之处,还请各位指摘。
validation demo