五、条件渲染
1.<template>
2.
3.v-if="isShow">
4.
5. box
6.
7.
8.v-if="(age>20)">
9.
10. {{age}}
11.
12.
13.
14.
15.
16.
17.
18.
19. export default {
20. data() {
21. return {
22. isShow:true,
23. age:12
24. }
25. },
26. methods: {
27. changeShow:function(){
28. this.isShow = !this.isShow;
29. },
30. changeAge:function(){
31. this.age+=3;
32. }
33. }
34. }
35.
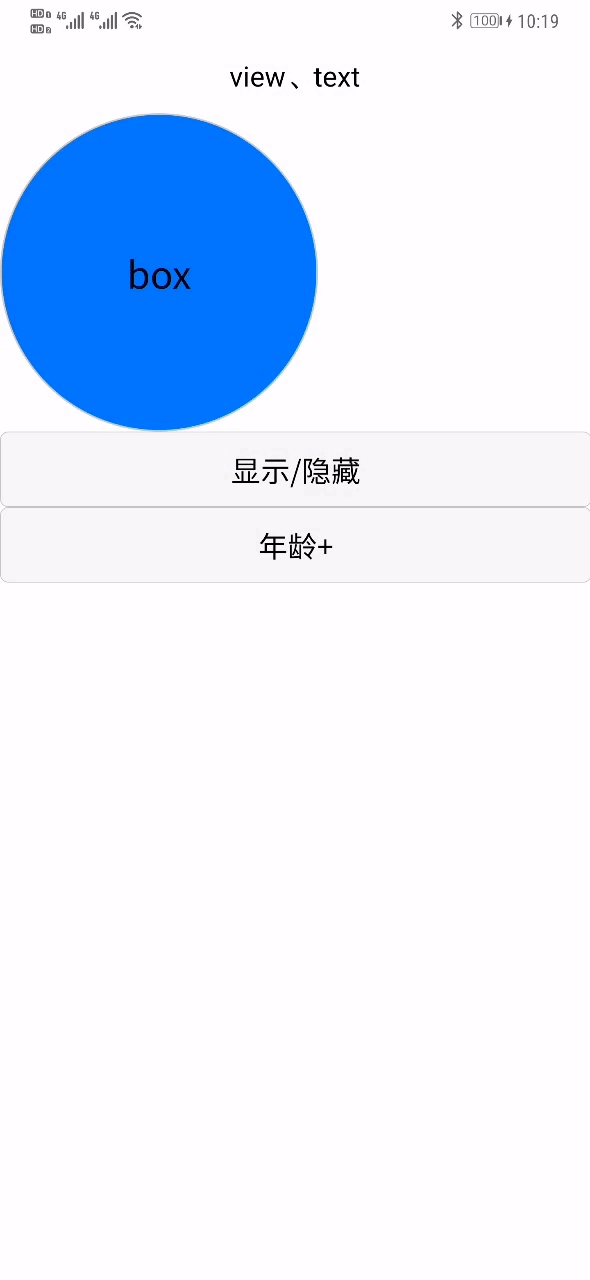
六、列表渲染(key要求值不能相同)
1.<template>
2.
3.
4.
5. {{ball}}-{{item}}
6.
7.
8.
9.
10. {{per}}-{{item1.name}}-{{item1.age}}
11.
12.
13.
14.
15. {{item2.name}}
16.
17.
18. |--{{item3}}
19.
20.
21.
22.
23. {{item4}}
24.
25.
26.
27.
28.
29.
30. export default {
31. data() {
32. return {
33. ballList:["篮球","足球","羽毛球","乒乓球"],
34. persion:[
35. {name:"张三",age:12},
36. {name:"李四",age:15},
37. {name:"王五",age:18}
38. ],
39. address:[
40. {
41. name:"安徽",
42. list:["蒙城","利辛","涡阳"]
43. },
44. {
45. name:"江苏",
46. list:["南京","镇江","连云港"]
47. }
48. ],
49. objList:{
50. name1:"太阳",
51. name2:"地球",
52. name3:"月亮"
53. }
54. }
55. },
56. methods: {
57. }
58. }
59.
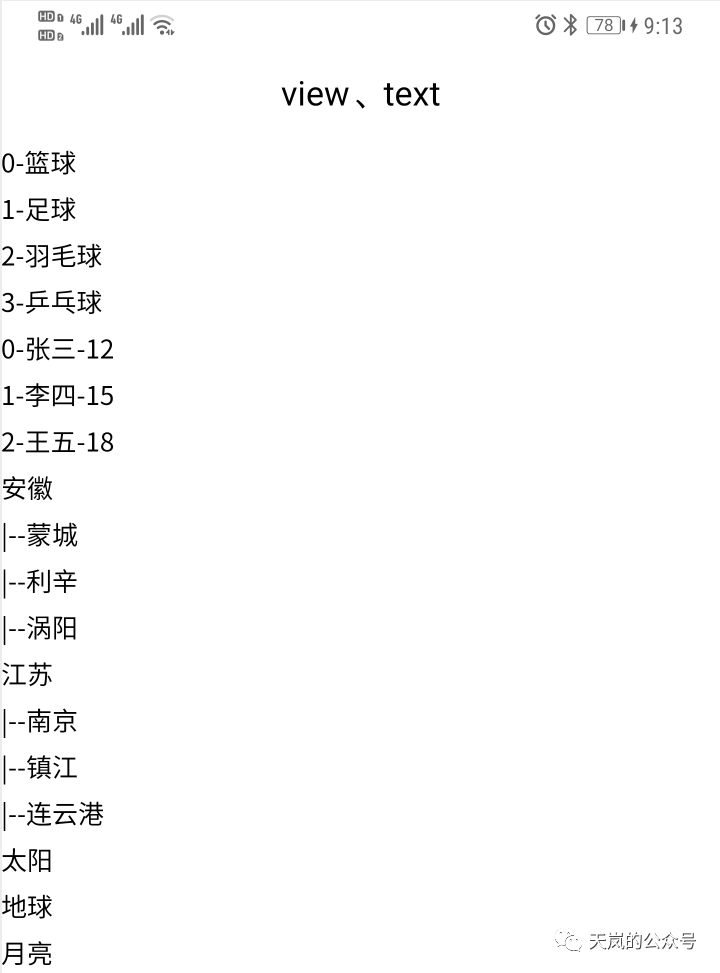
七、事件处理
1.
2.
3.
4.
5. 按钮
6.
7.
8. 外面
9.
10. 里面
11.
12.
13.
14.
15.
16.
17.
18. export default {
19. data() {
20. return {
21. name:"张三"
22. }
23. },
24. methods: {
25. click(){
26. console.log("点击了");
27. this.name="李四";
28. },
29. box1click(){
30. console.log("点击了box1");
31. },
32. box2click(){
33. console.log("点击了box2");
34. }
35. }
36. }
37.
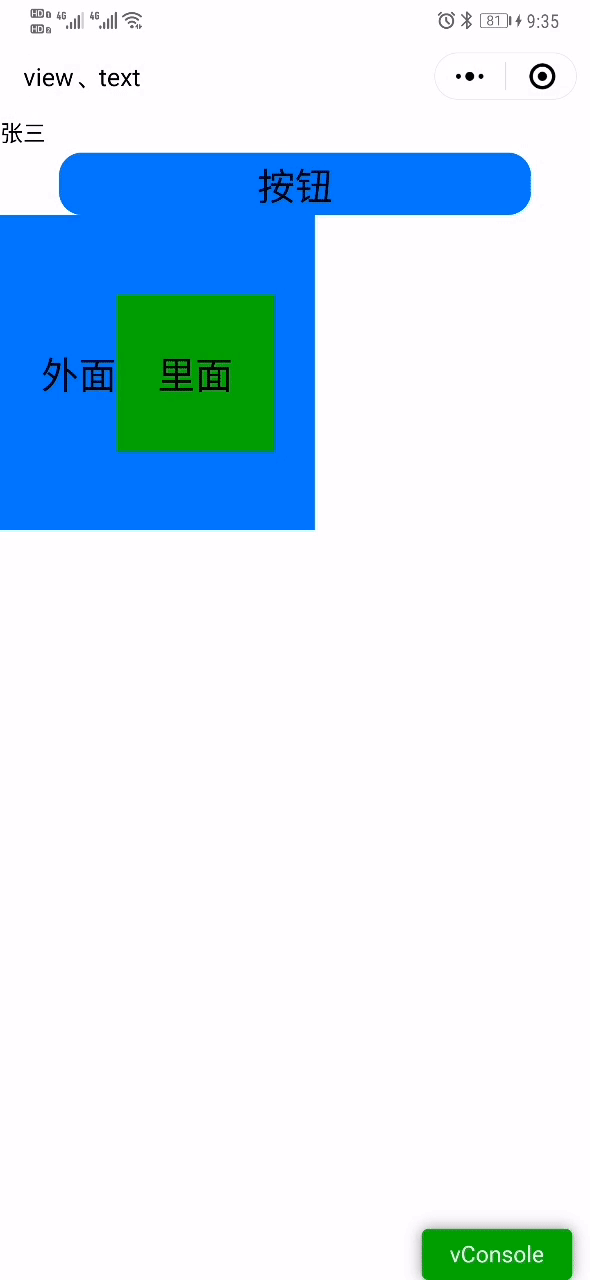
防止内层事件触发外层方法,@tap.stop
1.<template>
2.
3.
4.
5. 按钮
6.
7.
8. 外面
9.
10. 里面
11.
12.
13.
14.
15.
16.
17.
18. export default {
19. data() {
20. return {
21. name:"张三"
22. }
23. },
24. methods: {
25. click(){
26. console.log("点击了");
27. this.name="李四";
28. },
29. box1click(){
30. console.log("点击了box1");
31. },
32. box2click(){
33. console.log("点击了box2");
34. }
35. }
36. }
37.
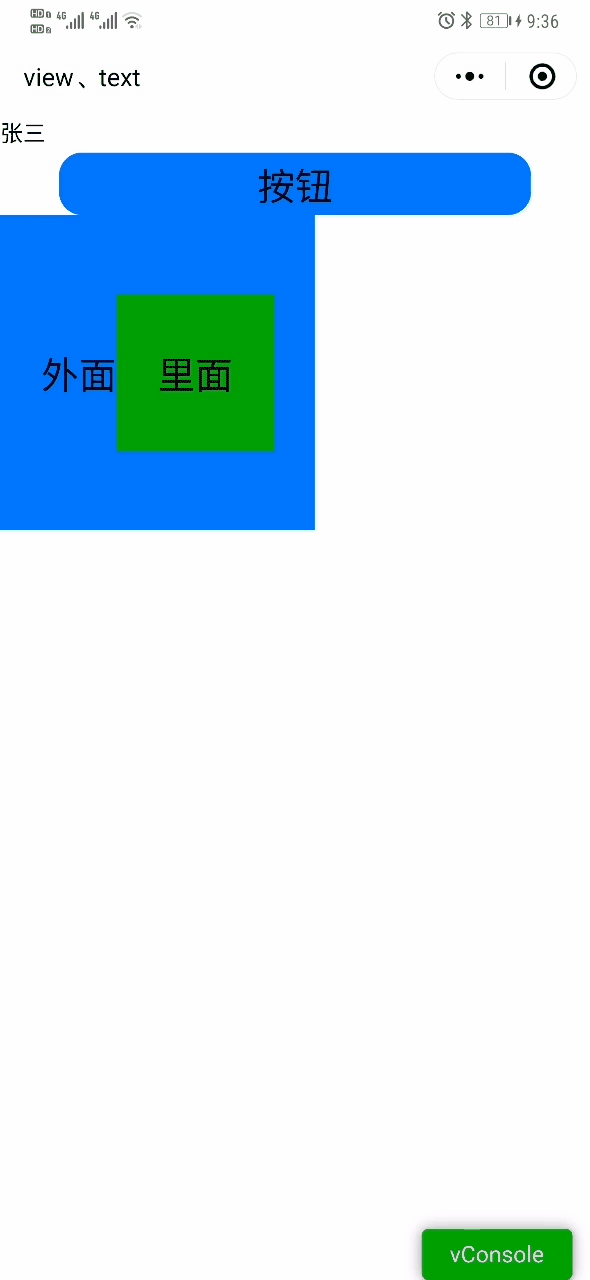
八、监听属性
1.<template>
2.
3.
4.
5. 按钮
6.
7.
8.
9.
10.
11. export default {
12. data() {
13. return {
14. name:"张三",
15. age:12
16. }
17. },
18. watch:{
19. age:function(val){
20. console.log(this.name+"年龄"+val);
21. }
22. },
23. methods: {
24. click(){
25. this.age++;
26. }
27. }
28. }
29.
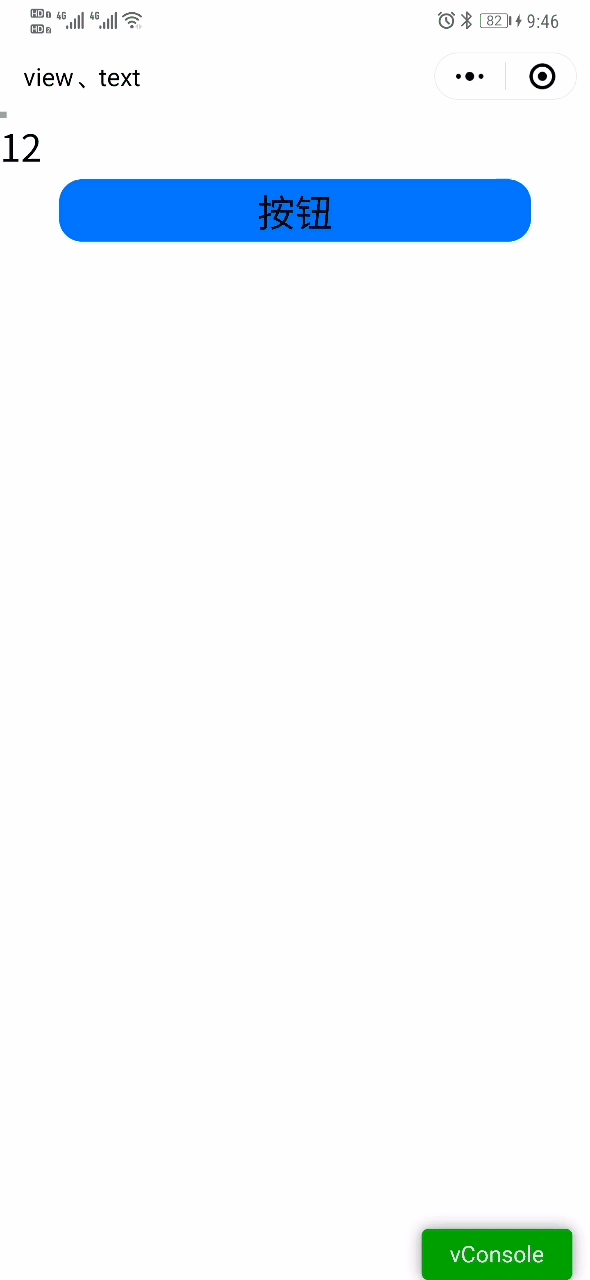
九、计算属性
1.<template>
2.
3.
4.
5. 按钮
6.
7.
8.
9.
10.
11. export default {
12. data() {
13. return {
14. weight:800
15. }
16. },
17. computed:{
18. ZHWeight:function(){
19. return this.weight>=1000?(this.weight/1000)+"kg":this.weight+"g"
20. }
21. },
22. watch:{
23. ZHWeight:function(val){
24. console.log("质量"+val);
25. }
26. },
27. methods: {
28. click(){
29. thisthis.weight=this.weight+100;
30. }
31. }
32. }
33.
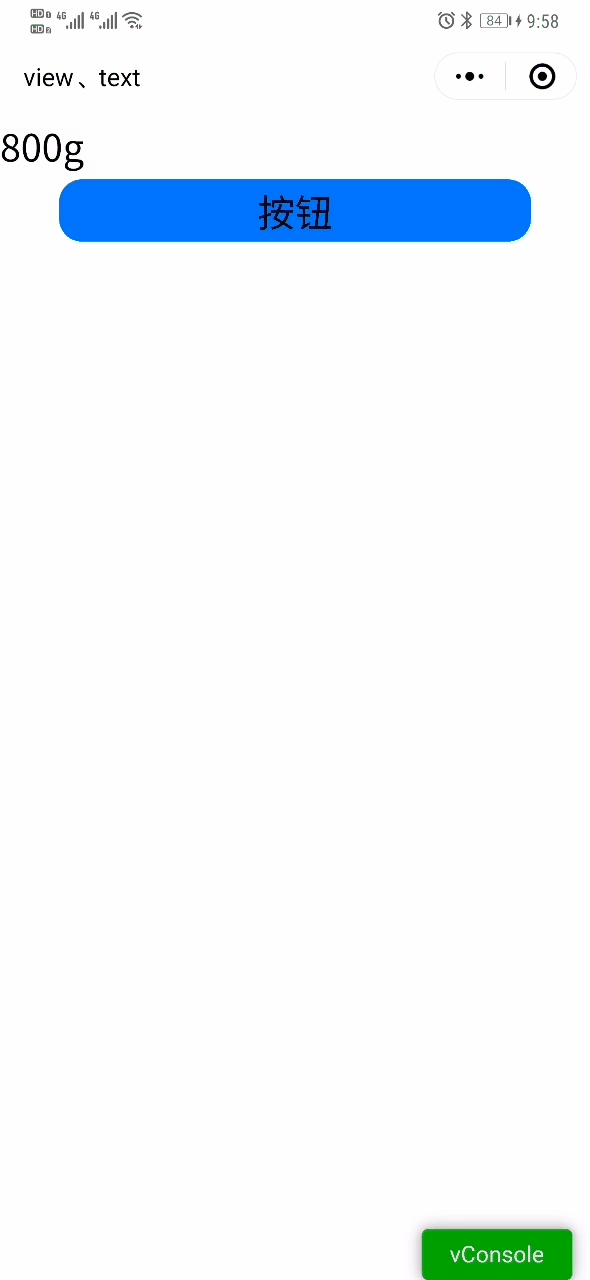