最近项目有用到价格标图,就自已定义了一个,现在分享出来,供大家学习了解,好了废话不多说,直接看图吧!
gif_anime.gif
源代码请看:
package single.electronic.sz.com.pircetagdemo;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Rect;
import android.graphics.RectF;
import android.util.AttributeSet;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
/**
* Created by men on 2017/11/30.
* 邮箱:[email protected]
*/
public class SlidingPriceBarView extends View {
private Bitmap gray_bg;//灰色价格标
private Bitmap green_bg;//绿色价格标
private Bitmap greatRound;//大圆
private Bitmap priceNumber;//左侧价格底图
private int price_h;//高价
private int price_d;//低价
//价格区间
private final int price_0 = 0;
private final int price_200 = 200;
private final int price_500 = 500;
private final int price_1000 = 1000;
private final int price_10000 = 10000;
private int bg_width;
private int bg_height;
private float scale;//压缩比例
private int danHeight;//分成四段的长度
private float half_round;
private Paint mPaint;
private float greatRoundX;
private float y_h;
private float y_d;
private int PRICE_PADDING = 15;
private float bg_x;
private boolean isUpTouched=false;
private boolean isDownTouched=false;
public SlidingPriceBarView(Context context, AttributeSet attrs) {
super(context, attrs);
init(context, attrs);
}
private void init(Context context, AttributeSet attrs) {
gray_bg = BitmapFactory.decodeResource(getResources(), R.drawable.axis_before);
green_bg = BitmapFactory.decodeResource(getResources(), R.drawable.axis_after);
greatRound = BitmapFactory.decodeResource(getResources(), R.drawable.btn);
priceNumber = BitmapFactory.decodeResource(getResources(), R.drawable.bg_number);
//得到自定义价格
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.SlidingPriceBarView);
price_h = typedArray.getInteger(R.styleable.SlidingPriceBarView_price_h, 1000);
price_d = typedArray.getInteger(R.styleable.SlidingPriceBarView_price_d, 200);
mPaint = new Paint();
mPaint.setColor(Color.BLACK);
typedArray.recycle();
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
//super.onMeasure(widthMeasureSpec, heightMeasureSpec);
int heightMode = MeasureSpec.getMode(heightMeasureSpec);
int heightSize = MeasureSpec.getSize(heightMeasureSpec);
bg_width = gray_bg.getWidth();
bg_height = gray_bg.getHeight();
//根据父类给出的尺寸测量出
int measureHeight = (heightMode == MeasureSpec.EXACTLY) ? heightSize : bg_height;
measureHeight = Math.max(measureHeight, heightSize);
int measureWidth = measureHeight * 2 / 3;
scale = measureHeight / (float) bg_height;
//分成四段的长度
danHeight = (bg_height - bg_width) / 4;
//小圆的半径
half_round = bg_height * (1 - 0.95f) / 2;
//设置自己测量的宽高
setMeasuredDimension(measureWidth, measureHeight);
}
/**
* @param canvas
*/
@Override
protected void onDraw(Canvas canvas) {
//保存当前的画布
canvas.save();
//对画布进行缩放
canvas.scale(scale, scale);
//绘制灰色底图
bg_x = (this.getWidth() / scale - gray_bg.getWidth()) / 2;
canvas.drawBitmap(gray_bg, bg_x, 0, mPaint);
//绘制右侧的数字
mPaint.setTextSize(20 / scale);
String[] number = new String[]{
"不限",
String.valueOf(price_1000),
String.valueOf(price_500),
String.valueOf(price_200),
String.valueOf(price_0)
};
for (int i = 0; i < number.length; i++) {
int startX = (int) (bg_x * 5 / 4);
int endX = (int) (i * danHeight + bg_width / 2 - (mPaint.ascent() + mPaint.descent()) / 2);
canvas.drawText(number[i].toString(), startX, endX, mPaint);
}
//画大圆(上下圆)
greatRoundX = (this.getWidth() / scale - greatRound.getWidth()) / 2;
y_h = getBtnYLocationByPrice(price_h);
canvas.drawBitmap(greatRound,greatRoundX,y_h-greatRound.getHeight()/2,mPaint);
y_d = getBtnYLocationByPrice(price_d);
canvas.drawBitmap(greatRound,greatRoundX,y_d-greatRound.getHeight()/2,mPaint);
//画中间的绿色部分
Rect src=new Rect(0,(int)(y_h+greatRound.getHeight()/2),(int)green_bg.getWidth(),(int)y_d-greatRound.getHeight()/2);
Rect dst=new Rect((int) bg_x,(int)y_h+greatRound.getHeight()/2,(int)(bg_x +green_bg.getWidth()),(int)y_d-greatRound.getHeight()/2);
canvas.drawBitmap(green_bg,src,dst,mPaint);
//画左侧的图标
Rect rect_h= getRectByMidLine(y_h);
canvas.drawBitmap(priceNumber,null,rect_h,mPaint);
Rect rect_d= getRectByMidLine(y_d);
canvas.drawBitmap(priceNumber,null,rect_d,mPaint);
//画左侧的数字
//上边文本坐标
float text_u_x = (3*rect_h.width()/4 - mPaint.measureText(String.valueOf(price_h)))/2;
float text_u_y = rect_h.top - mPaint.ascent() + PRICE_PADDING;
//下边文字坐标
float text_d_x = (3*rect_d.width()/4 - mPaint.measureText(String.valueOf(price_d)))/2;
float text_d_y = rect_d.top - mPaint.ascent() + PRICE_PADDING;
canvas.drawText(String.valueOf(price_h), text_u_x, text_u_y, mPaint);
canvas.drawText(String.valueOf(price_d), text_d_x, text_d_y, mPaint);
//恢复之前的保存的画布
canvas.restore();
super.onDraw(canvas);
}
private Rect getRectByMidLine(float y) {
Rect mRect =new Rect();
mRect.left=0;
mRect.right=(int)greatRoundX;
float text_h = mPaint.descent() - mPaint.ascent();
mRect.top = (int)(y-text_h/2) - PRICE_PADDING ;
mRect.bottom = (int)(y+text_h/2) + PRICE_PADDING ;
return mRect;
}
private float getBtnYLocationByPrice(int price) {
Log.i("ms","price:"+price);
float y=0;
if (price < price_0) {
price = price_0;
}
if (price>price_10000){
price=price_10000;
}
if (price>=price_0&&price=price_200&&price=price_500&&price10000
price = price_10000;
}else if(y>=half_height&&yhalf_height+danHeight&&yhalf_height+2*danHeight&&ygreatRoundX&&downXy_h-greatRound.getHeight()/2&&downY<=y_h+greatRound.getHeight()/2){
isUpTouched = true;
isDownTouched = false;
}
if (downY>y_d-greatRound.getHeight()/2&& downY<=y_d+greatRound.getHeight()/2){
isUpTouched = false;
isDownTouched = true;
}
}
break;
case MotionEvent.ACTION_MOVE:
float y2=event.getY()/scale;
if (isUpTouched){
price_h=getPriceByPosition(y2);
if (price_h<=price_d){
price_h=price_d;
}
}
if (isDownTouched){
price_d=getPriceByPosition(y2);
if (isDownTouched){
if (price_d>price_h){
price_d=price_h;
}
}
}
//开始重新绘制
invalidate();
break;
case MotionEvent.ACTION_UP:
isDownTouched=false;
isUpTouched=false;
break;
}
return true;
}
}
从上面的源代码可以看出,重写的onMeasure方法,测量子类的宽高,然后就是绘制,关键的地方我都写了注释,一看就明白!
本人做android开发多年,以后会陆续更新关于android高级UI,NDK开发,性能优化等文章,更多请关注我的微信公众号:谢谢!
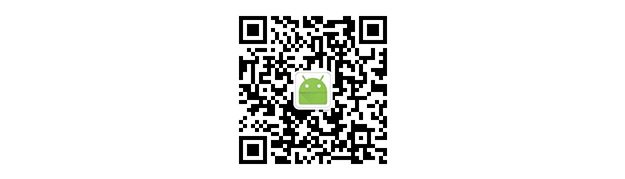
android的那点事.jpg