本章为本人学习过程中的一些笔记和demo记录,理解比较片面,本人为学习Linux菜鸟,如果本文能够帮助各位理解,本人倍感荣幸,若文章有不足之处或错误欢迎指出,不喜勿碰。
进程:一个进程只能做一件事情,进程是线程的载体,在一个进程中设计多线程可以使一个进程完成多个任务,比如贪吃蛇游戏同时刷新地图和接受键盘输入的方向控制。在一个程序中多个进程也可以执行多个任务,但是在执行多个进程是CPU需要为进程开启空间,在Linux系统中执行多进程时,系统需要分配独立的空间,进程间的数据段为写时拷贝这样对CPU的消耗较大,而线程则不需要独立的空间,他相当于寄生在进程上,和进程共享数据,比较节省CPU的资源,线程需要依赖进程而存在,当一个进程意外退出结束时,该进程中相对应的所有线程将提前结束。线程是一种轻量化的多任务处理方式。
创建线程的API:int pthread_create(pthread_t *thread, const pthread_attr_t *attr,void *(*start_routine) (void *), void *arg);
第一个参数为线程号,即线程ID,第二个参数是的对应的属性,一般可为NULL,第三个参数是线程对应的线程开始的函数地址,第四个参数为给线程传入的参数。给线程传入个参数时采用结构体为线程传参。
代码1:#include
#include
int count = 0;
//int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
void *fun1()
{
while(1)
{
count++;
printf(“count = %d\n”,count);
printf(“this is thread 1\n”);
sleep(1);
}
}
void *fun2()
{
while(1)
{
count++;
printf(“count = %d\n”,count);
printf(“this is thread 2\n”);
sleep(1);
}
}
int main()
{
pthread_t t1,t2;
pthread_create(&t1,NULL,fun1,NULL);
pthread_create(&t2,NULL,fun2,NULL);
// while(1);
return ;
}
运行结果:
修改上述demo的代码,将main函数中的while(1);语句注释取消,运行结果如下图所示:
代码2:`#include
#include
void *fun1(void arg)
{
static int num;
printf(“Enter t1 thread:\n”);
printf(“count = %d\n”,++(((int *)arg)));
num = *((int *)arg);
pthread_exit((void *)&num);
}
int main()
{
pthread_t t1;
int count = 0;
int *ret = NULL;
printf(“Main thread start\n”);
pthread_create(&t1,NULL,fun1,(void *)&count);
pthread_join(t1,(void **)&ret);
printf(“ret = %d\n”,*ret);
printf(“Main thread quit\n”);
return 0;
}
在原始代码中由于未来得及进入创建的线程,进程就已经退出,故没有任何现象。当加上while(1)时,程序并未退出,此时多个线程同时竞争,决定进入线程1或者线程二,因此便出现线程一和二交替运行的现象。实际在pthread.h库中有配合线程进入和退出的库函数,分别是: int pthread_join(pthread_t thread, void **retval);和void pthread_exit(void *retval);等待线程进入函数pthread_join有两个参数第一个参数是线程号(即线程id)第二个参数是一个无类型的二级指针,用来收集线程的返回值,和pthread_exit的参数用来返回线程退出时的返回值。如上述代码2所示。运行结果如下: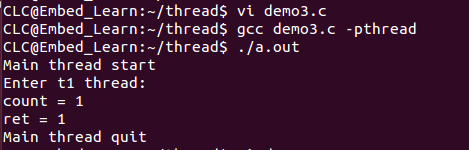 多线程返传入多个参数demo:
#include
#include
#include
struct test
{
int a;
char str[128];
};
void *fun1(void arg)
{
static int num = 10;
printf(“Enter t1 thread:\n”);
printf(“count = %d\n”,++(((int *)arg)));
pthread_exit((void *)&num);
}
void *fun2(void arg)
{
// static int num = 10;
printf(“Enter t2 thread:\n”);
// printf(“count = %d\n”,++(((int *)arg)));
struct test *t = (struct test *)arg;
printf(“a = %d\n”,t->a);
printf(“str = %s\n”,t->str);
pthread_exit(NULL);
// pthread_exit((void *)&num);
}
int main()
{
struct test t = {12,“this is thread1”};
pthread_t t1;
pthread_t t2;
int count = 0;
int ret1,ret2;
int *ret = NULL;
printf(“Main thread start\n”);
ret1 = pthread_create(&t1,NULL,fun1,(void *)&count);
ret2 = pthread_create(&t2,NULL,fun2,(void *)&t);
if(ret1 != 0)
{
printf(“create thread failure\n”);
exit(0);
}
pthread_join(t1,(void **)&ret);
pthread_join(t2,NULL);
printf(“ret = %d\n”,*ret);
printf(“Main thread quit\n”);
return 0;
}
`