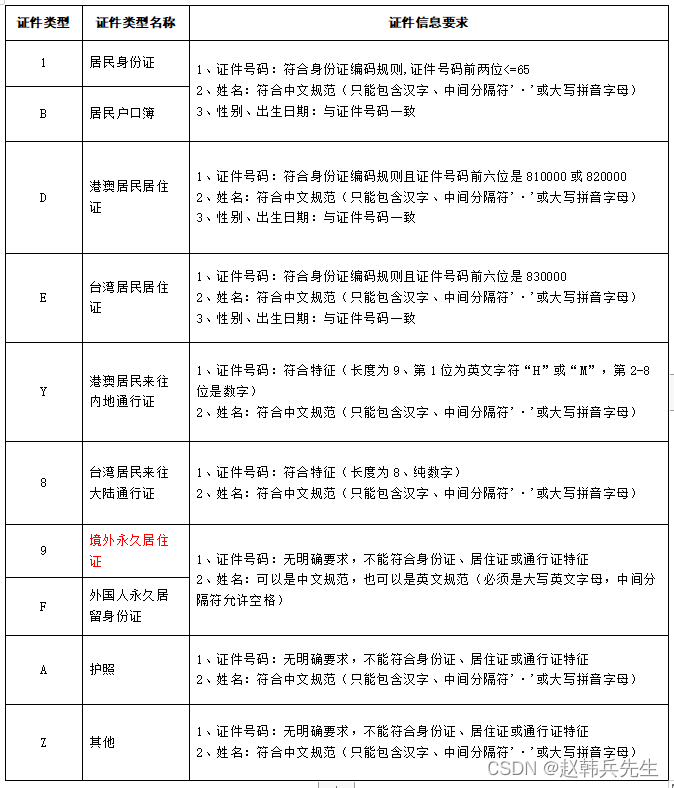
校验工具类
import com.xx.xx.dic.CertificateType;
public class CertificateValidateUtil {
public static String validate(String xm, String xb, String zjlx, String zjhm, String csrq) {
if (ValidatorUtil.isSfzh(zjhm)) {
int theFirstTwo = Integer.parseInt(zjhm.substring(0, 2));
if (theFirstTwo <= 65 && !CertificateType.JMSFZ.getCode().equals(zjlx) && !CertificateType.JMHKB.getCode().equals(zjlx)) {
return "E00_证件类型与证件号码不一致(证件号码符合[居民身份证]编码规则)";
}
if ((theFirstTwo == 81 || theFirstTwo == 82) && !CertificateType.GAJMJZZ.getCode().equals(zjlx)) {
return "E00_证件类型与证件号码不一致(证件号码符合[港澳居民居住证]编码规则)";
}
if (theFirstTwo == 83 && !CertificateType.TWJMJZZ.getCode().equals(zjlx)) {
return "E00_证件类型与证件号码不一致(证件号码符合[台湾居民居住证]编码规则)";
}
}
if (!isSfzh(zjhm)) {
if (zjhm.length() == 9) {
char theFirst = zjhm.charAt(0);
String theTwo2Eight = zjhm.substring(1, 8);
if ((theFirst == 'H' || theFirst == 'M') && ValidatorUtil.isNumber(theTwo2Eight) && !CertificateType.GAJMLWNDTXZ.getCode().equals(zjlx)) {
return "E00_证件类型与证件号码不一致(证件号码符合[港澳居民来往内地通行证]特征)";
}
}
if (zjhm.length() == 8 && ValidatorUtil.isNumber(zjhm) && !CertificateType.TWJMLWNDTXZ.getCode().equals(zjlx)) {
return "E00_证件类型与证件号码不一致(证件号码符合[台湾居民来往大陆通行证]特征)";
}
}
if (CertificateType.JMSFZ.getCode().equals(zjlx) || CertificateType.JMHKB.getCode().equals(zjlx)) {
if (!isSfzh(zjhm)) {
return "E00_证件号码不规范(不符合[居民身份证编码]规则)";
}
int theFirstTwo = Integer.valueOf(zjhm.substring(0, 2));
if (theFirstTwo > 65) {
return "E00_证件号码不规范(不符合[居民身份证编码]规则)";
}
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
String theSeven2Twelve = zjhm.substring(6, 14);
if (!theSeven2Twelve.equals(csrq)) {
return "E00_出生日期与证件号码不一致";
}
int theSeventeen = Integer.valueOf(zjhm.charAt(16));
if (!((theSeventeen % 2 == 0 && xb.equals("女")) || (theSeventeen % 2 != 0 && xb.equals("男")))) {
return "E00_性别与证件号码不一致";
}
}
if (CertificateType.GAJMJZZ.getCode().equals(zjlx)) {
if (!isSfzh(zjhm)) {
return "E00_证件号码不规范(不符合[港澳居民居住证]编码规则)";
}
String theFirstSix = zjhm.substring(0, 6);
if (!theFirstSix.equals("810000") && !theFirstSix.equals("820000")) {
return "E00_证件号码不规范(不符合[港澳居民居住证]编码规则)";
}
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
String theSeven2Twelve = zjhm.substring(6, 14);
if (!theSeven2Twelve.equals(csrq)) {
return "E00_出生日期与证件号码不一致";
}
int theSeventeen = Integer.valueOf(zjhm.charAt(16));
if (!((theSeventeen % 2 == 0 && xb.equals("女")) || (theSeventeen % 2 != 0 && xb.equals("男")))) {
return "E00_性别与证件号码不一致";
}
}
if (CertificateType.TWJMJZZ.getCode().equals(zjlx)) {
if (!isSfzh(zjhm)) {
return "E00_证件号码不规范(不符合[台湾居民居住证]编码规则)";
}
String theFirstSix = zjhm.substring(0, 6);
if (!theFirstSix.equals("830000")) {
return "E00_证件号码不规范(不符合[台湾居民居住证]编码规则)";
}
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
String theSeven2Twelve = zjhm.substring(6, 14);
if (!theSeven2Twelve.equals(csrq)) {
return "E00_出生日期与证件号码不一致";
}
int theSeventeen = Integer.valueOf(zjhm.charAt(16));
if (!((theSeventeen % 2 == 0 && xb.equals("女")) || (theSeventeen % 2 != 0 && xb.equals("男")))) {
return "E00_性别与证件号码不一致";
}
}
if (CertificateType.GAJMLWNDTXZ.getCode().equals(zjlx)) {
if (zjhm.length() != 9) {
return "E00_证件号码不规范(与[港澳居民来往内地通行证]证件特征不符)";
}
char theFirst = zjhm.charAt(0);
String theTwo2Eight = zjhm.substring(1, 8);
if ((theFirst != 'H' && theFirst != 'M') || !ValidatorUtil.isNumber(theTwo2Eight)) {
return "E00_证件号码不规范(与[港澳居民来往内地通行证]证件特征不符)";
}
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
}
if (CertificateType.TWJMLWNDTXZ.getCode().equals(zjlx)) {
if (zjhm.length() != 8 || !ValidatorUtil.isNumber(zjhm)) {
return "E00_证件号码不规范(与[台湾居民来往大陆通行证]证件特征不符)";
}
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
}
if (CertificateType.JWYJJZZ.getCode().equals(zjlx) || CertificateType.WGRYJJLSFZ.getCode().equals(zjlx)) {
if (!isGoodChineseName(xm) && !isEnglishName(xm)) {
return "E00_姓名不规范";
}
}
if (CertificateType.HZ.getCode().equals(zjlx) || CertificateType.QT.getCode().equals(zjlx)) {
if (!isGoodChineseName(xm)) {
return "E00_姓名不规范";
}
}
return "";
}
}
证件类型枚举
import org.apache.commons.lang.StringUtils;
public enum CertificateType {
JMSFZ("居民身份证", "1"), JMHKB("居民户口簿", "B"), GAJMJZZ("港澳居民居住证", "D"),
TWJMJZZ("台湾居民居住证", "E"), GAJMLWNDTXZ("港澳居民来往内地通行证", "Y"), TWJMLWNDTXZ("台湾居民来往大陆通行证", "8"),
JWYJJZZ("境外永久居住证", "9"), WGRYJJLSFZ("外国人永久居留身份证", "F"), HZ("护照", "A"), QT("其他", "Z");
private String name;
private String code;
CertificateType(String name, String code) {
this.name = name;
this.code = code;
}
public String getName() {
return name;
}
public String getCode() {
return code;
}
public static CertificateType getByName(String name) {
if (StringUtils.isBlank(name)) {
return QT;
} else {
CertificateType[] items = values();
for (CertificateType item : items) {
if (item.getName().equals(name)) {
return item;
}
}
return QT;
}
}
public static CertificateType getByCode(String code) {
if (StringUtils.isBlank(code)) {
return QT;
} else {
CertificateType[] items = values();
for (CertificateType item : items) {
if (item.getCode().equals(code)) {
return item;
}
}
return QT;
}
}
}
身份证规则校验
public static boolean isSfzh(String sfzh) {
return StringUtils.isBlank(sfzh) ? false : contains(sfzh, "(^\\d{15}$)|(^(\\d){17}([\\d|X|x|x|X])$)");
}
中文英文姓名校验
public static boolean isGoodChineseName(String xm) {
if (StringUtils.isBlank(xm)) {
return false;
}
if (xm.startsWith("·") || xm.endsWith("·")) {
return false;
}
String str = xm.replaceAll("[\u4e00-\u9fa5A-Z·]", "");
if (str.length() > 0) {
return false;
}
return true;
}
public static boolean isGoodEnglishName(String xm) {
if (StringUtils.isBlank(xm)) {
return false;
}
Pattern p = Pattern.compile("[\\s]{2,}");
Matcher m = p.matcher(xm);
if (m.find()) {
return false;
}
String str = xm.replaceAll("[A-Z,\\s]", "");
return str.length() == 0;
}