目录
-
- 1.项目目录
- 2.pom.xml
- 3.application.yml
- 4.pojo
- 5.dao
- 6service及serviceimpl
- 7.controller
- 8.邮箱测试(简单邮箱,HTML邮箱,带文件邮箱)
- 9.页面(thymeleaf静态化模板)
-
- 9.1index.xml
- 9.2update.html
- 9.3注册页面
1.项目目录
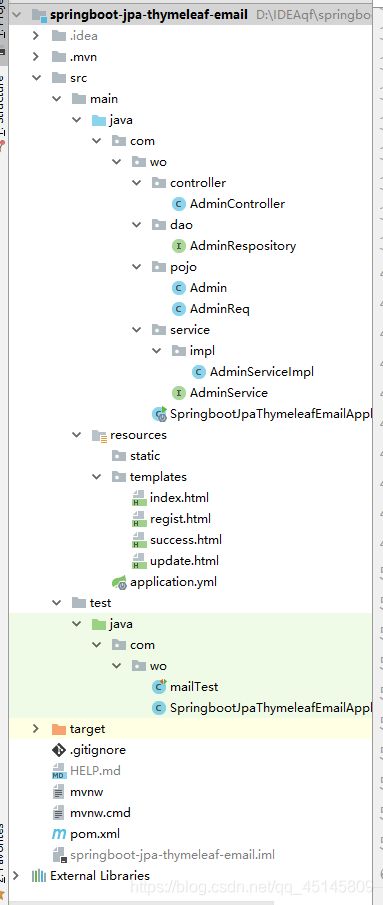
2.pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.3.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.wo</groupId>
<artifactId>springboot-jpa-thymeleaf-email</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-jpa-thymeleaf-email</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--JPA-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- thymeleaf-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- 整合邮箱发送-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3.application.yml
server:
port: 8088
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
username: root
password: 123456
url: jdbc:mysql://127.0.0.1:3306/qf?useUnicode=true&characterEncoding=utf8&useSSL=false
jpa:
database: mysql
show-sql: true
generate-ddl: true
mail:
host: smtp.qq.com
username: 自己的邮箱
password: 提供的发送密码
properties:
smtp:
auth: true
starttls:
enable: true
required: true
default-encoding: utf-8
4.pojo
@Data
@Entity
@Table(name = "admin")
public class Admin {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String name;
private String password;
private String email;
}
@Data
public class AdminReq {
private String email;
private String code;
private String name;
private String password;
}
5.dao
public interface AdminRespository extends JpaRepository<Admin,Integer>{
}
6service及serviceimpl
List<Admin> findAll();
Admin findById(int id);
void update(Admin admin);
void delete(int id);
String sendEmail(String mail, HttpServletRequest request);
String regist(AdminReq adminReq,HttpServletRequest request);
@Service
public class AdminServiceImpl implements AdminService{
@Autowired
AdminRespository adminRespository;
@Autowired
JavaMailSender javaMailSender;
@Value("${spring.mail.username}")
private String from;
@Override
public List<Admin> findAll() {
return adminRespository.findAll();
}
@Override
public Admin findById(int id) {
Optional<Admin> byId = adminRespository.findById(id);
if(byId.isPresent()){
Admin admin = byId.get();
return admin;
}
return null;
}
@Override
public void update(Admin admin) {
adminRespository.saveAndFlush(admin);
}
@Override
public void delete(int id) {
adminRespository.deleteById(id);
}
@Override
public String sendEmail(String mail, HttpServletRequest request) {
SimpleMailMessage simpleMailMessage=new SimpleMailMessage();
simpleMailMessage.setFrom(from);
simpleMailMessage.setTo(mail);
simpleMailMessage.setSubject("注册验证");
int num=(int) ((Math.random() * 9 + 1) * 100000);
String code=Integer.toString(num);
System.out.println("****************验证码是"+num);
simpleMailMessage.setText(code);
javaMailSender.send(simpleMailMessage);
HttpSession session=request.getSession();
session.setAttribute(mail,code);
return "success";
}
@Override
public String regist(AdminReq adminReq, HttpServletRequest request) {
String mail=adminReq.getEmail();
String code=adminReq.getCode();
HttpSession session=request.getSession();
String attribute = (String) session.getAttribute(mail);
if (code.equals(attribute)) {
Admin admin=new Admin();
BeanUtils.copyProperties(adminReq,admin);
adminRespository.saveAndFlush(admin);
return "success";
}
return "fail";
}
}
7.controller
@Controller
public class AdminController {
@Autowired
AdminService adminService;
@RequestMapping("/")
public ModelAndView fingAll(){
List<Admin> adminList = adminService.findAll();
ModelAndView modelAndView=new ModelAndView();
modelAndView.addObject("list",adminList);
modelAndView.setViewName("index");
return modelAndView;
}
@RequestMapping("/findById")
public ModelAndView findById(@RequestParam("id") Integer id){
Admin admin = adminService.findById(id);
ModelAndView modelAndView=new ModelAndView();
modelAndView.addObject("admin",admin);
modelAndView.setViewName("update");
return modelAndView;
}
@RequestMapping("/insertAndUpdate")
public String insertAndUpdate(Admin admin){
adminService.update(admin);
return "redirect:/";
}
@RequestMapping("/goUpdate")
public String goUpdate(Model model){
model.addAttribute("admin",new Admin());
return "update";
}
@RequestMapping("/deleteById")
public String deleteById(@RequestParam("id") int id){
adminService.delete(id);
return "redirect:/";
}
@RequestMapping("/sendMail")
@ResponseBody
public String sendMail(@RequestParam("mail") String mail, HttpServletRequest request){
return adminService.sendEmail(mail,request);
}
@RequestMapping("/go")
public String goRegist(){
return "regist";
}
@RequestMapping("/regist")
public String regist(AdminReq adminReq,HttpServletRequest request){
String regist = adminService.regist(adminReq, request);
if(regist.equals("success")){
return "success";
}
return "redirect:/regist";
}
}
8.邮箱测试(简单邮箱,HTML邮箱,带文件邮箱)
@RunWith(SpringRunner.class)
@SpringBootTest
public class mailTest {
@Autowired
JavaMailSender javaMailSender;
@Value("${spring.mail.username}")
private String from;
@Test
public void testSend(){
SimpleMailMessage simpleMailMessage=new SimpleMailMessage();
simpleMailMessage.setFrom(from);
simpleMailMessage.setTo("******@qq.com");
simpleMailMessage.setSubject("注册验证");
simpleMailMessage.setText("123456");
javaMailSender.send(simpleMailMessage);
}
@Test
public void testHtmlSend() throws MessagingException {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setFrom(from);
mimeMessageHelper.setTo("*******@qq.com");
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("这是html邮件");
mimeMessageHelper.setText(stringBuffer.toString(),true);
mimeMessageHelper.setSubject("测试");
javaMailSender.send(mimeMessage);
}
@Test
public void testFileSend() throws MessagingException {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true);
mimeMessageHelper.setFrom(from);
mimeMessageHelper.setTo("*****@qq.com");
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("这是html邮件");
mimeMessageHelper.setText(stringBuffer.toString(),true);
FileSystemResource fileSystemResource = new FileSystemResource(new File("E://1.jpg"));
mimeMessageHelper.addAttachment("lxl.jpg",fileSystemResource);
mimeMessageHelper.setSubject("测试");
javaMailSender.send(mimeMessage);
}
}
9.页面(thymeleaf静态化模板)
9.1index.xml
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>thymeleaf的首页面</h1>
<a th:href="@{/goUpdate}">增加</a>
<table border="1">
<tr>
<th>序号</th>
<th>ID</th>
<th>name</th>
<th>password</th>
<th>email</th>
<th>操作</th>
</tr>
<!--
admin,adminind:${list}
admin:循环获取到的当前对象
adminind:获取到的数组第一个
th:text 展示文本数据
th:utext 解析样式展示到html
th:each 循环list
-->
<tr th:each="admin,adminind:${list}">
<td th:text="${adminind.index+1}"></td>
<td th:text="${admin.id}"></td>
<td th:text="${admin.name}"></td>
<td th:text="${admin.password}"></td>
<td th:text="${admin.email}"></td>
<!--根据1或0显示男女
<td><span th:if="${admin.sex eq '1'}">男</span>-->
<!--<span th:if="${admin.sex eq '0'}">女</span>-->
<!--</td>-->
<td>
<a th:href="@{'findById?id='+${admin.id}}">修改|</a>
<a th:href="@{'deleteById?id='+${admin.id}}">|删除</a>
</td>
</tr>
</table>
</body>
</html>
9.2update.html
<body>
<form th:action="@{/insertAndUpdate}" th:method="post">
<input th:type="hidden" th:name="id" th:value="${admin.id}">
name: <input th:type="text" th:name="name" th:value="${admin.name}"><br/>
password: <input th:type="text" th:name="password" th:value="${admin.password}"><br/>
email: <input th:type="text" th:name="email" th:value="${admin.email}"><br/>
<input th:type="submit" th:value="提交">
</form>
</body>
9.3注册页面
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="http://cdn.bootcss.com/jquery/1.10.2/jquery.min.js"></script>
</head>
<body>
<div>
用户邮箱:<input id="email" th:name="email"><button onclick="sendMail()">发送验证码</button><br>
验证码:<input id="code" th:name="code"><br>
姓名:<input id="name" th:name="name"><br>
密码:<input id="password" th:name="password"><br>
<input onclick="regist()" th:type="button" th:value="提交">
</div>
</body>
<script>
function sendMail() {
var email=$("#email").val()
alert(email)
$.ajax({
url:'/sendMail',
data:{"mail":email},
success:function (res) {
alert(res);
}
})
}
function regist(){
var email=$("#email").val()
var code=$("#code").val()
var name=$("#name").val()
var password=$("#password").val()
$.ajax({
url:'/regist',
data:{"email":email,"code":code,"name":name,"password":password},
async:true
})
}
</script>
</html>