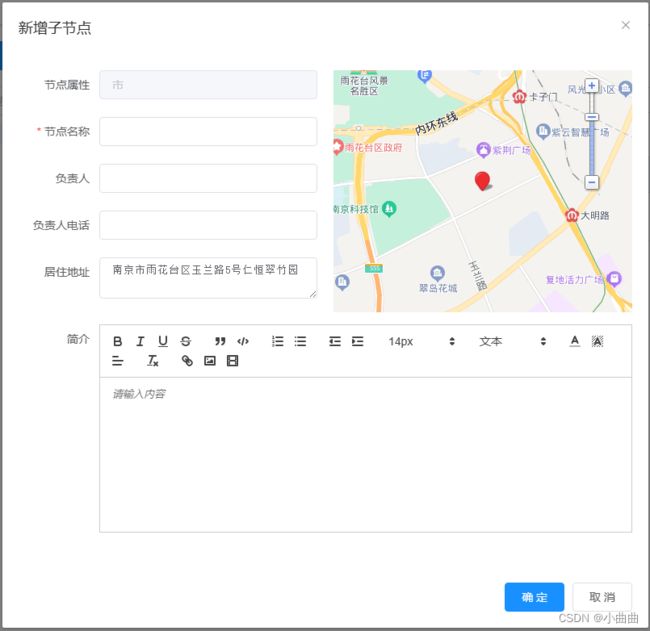
- 地图加载:需要监听弹窗组件opened中加载,否则会因为取不到地图html元素报错
- 新增进来可以默认获取浏览器当前定位经纬度,然后重新设置地图显示中心点
map.setCenter(point)
- 修改不需要获取当前定位,修改需要获取到经纬度以后再加载地图
- 地图不设置中心点会显示蓝色
- bug 两个弹窗组件都有百度地图,页面刷新后先操作一个弹窗,在操作另一个弹窗,地图会空白
- 解决方法:修改地图DOM的 id=“map-container” 为不一致
<template>
<div>
<el-dialog @opened="openModel" @close="closeModel" :title="title" :visible.sync="open" width="800px" append-to-body>
<el-form ref="form" :model="form" :rules="rules" label-width="100px">
<el-row :gutter="20">
<el-col :span="12">
<el-form-item label="节点属性">
<el-input disabled v-model="form.communityType"></el-input>
</el-form-item>
<el-form-item label="节点名称" prop="communityName">
<el-input v-model="form.communityName"></el-input>
</el-form-item>
<el-form-item label="负责人" prop="leader">
<el-input v-model="form.leader"></el-input>
</el-form-item>
<el-form-item label="负责人电话" prop="phone">
<el-input v-model="form.phone"></el-input>
</el-form-item>
<el-form-item label="居住地址" prop="communityAddress">
<el-autocomplete popper-class="autoAddressClass" :fetch-suggestions="querySearchAsync"
:trigger-on-focus="false" @select="handleSelect" type="textarea" size="small" clearable
v-model="form.communityAddress">
<template slot-scope="{ item }">
<i class="el-icon-search fl mgr10" />
<div style="overflow: hidden">
<div class="title">{{ item.title }}</div>
<span class="address ellipsis">{{ item.address }}</span>
</div>
</template></el-autocomplete>
</el-form-item>
</el-col>
<el-col :span="12">
<div id="map-container" style="width: 100%; height: 300px" />
</el-col>
</el-row>
<el-row class="mt-10">
<el-form-item label="简介" prop="synopsis">
<editor v-model="form.synopsis" :min-height="192" />
</el-form-item>
</el-row>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button type="primary" @click="submitForm">确 定</el-button>
<el-button @click="open = false">取 消</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
import {
communityDetail,
addCommunity,
editCommunity,
} from "@/api/serviceObject/service";
import loadBMap from "@/utils/loadMap";
import { checkPhoneNumber } from "@/utils/index";
function checkPhone(rule, value, callback) {
const reg = /^1[3-9](\d{9})$/;
if (value && !reg.test(value)) {
return callback(new Error("号码格式不正确"));
} else {
return callback();
}
}
export default {
data() {
return {
open: false,
title: "",
communityId: "",
modelLevel: '',
typeFlag: '',
form: {
communityLongitude: '',
communityLatitude: '',
communityType: '',
communityName: '',
leader: '',
phone: '',
communityAddress: null,
synopsis: '',
},
rules: {
communityName: [
{ required: true, message: "节点名称不能为空", trigger: "blur" },
],
phone: [
{
validator: checkPhone,
trigger: "blur",
},
],
},
};
},
methods: {
init(title, modelLevel, typeFlag, communityId) {
loadBMap("WMZPnL9pUEMeY4a3sQ3sV28jwq7dWp6L");
this.typeFlag = typeFlag
this.open = true
this.modelLevel = modelLevel
this.getType()
this.title = title
this.communityId = communityId
},
getType() {
let arr = ['省', '市', '区', '街道', '社区']
this.$set(this.form, 'communityType', arr[this.modelLevel * 1 - 1])
},
openModel() {
if (this.typeFlag == 1) {
this.initMap()
} else if (this.typeFlag == 2) {
communityDetail(this.communityId).then((res) => {
this.form.communityLongitude = res.data.communityLongitude
this.form.communityLatitude = res.data.communityLatitude
this.form.communityName = res.data.communityName
this.form.leader = res.data.leader
this.form.phone = res.data.phone
this.form.communityAddress = res.data.communityAddress
this.form.synopsis = res.data.synopsis
this.initMap()
});
}
},
closeModel() {
this.title = ""
this.modelLevel = 1
this.typeFlag = ''
this.$refs['form'].resetFields();
this.form = {
communityLongitude: '',
communityLatitude: '',
communityType: '',
communityName: '',
leader: '',
phone: '',
communityAddress: null,
synopsis: '',
}
},
submitForm() {
this.$refs["form"].validate((valid) => {
if (valid) {
if (this.typeFlag == 1) {
let query = { ...this.form, parentId: this.modelLevel == 1 ? '' : this.communityId }
addCommunity(query).then((res) => {
this.$modal.msgSuccess("增加节点成功");
this.open = false;
this.$emit('update')
});
} else {
let query = { ...this.form, communityId: this.communityId }
editCommunity(query).then((res) => {
this.$modal.msgSuccess("修改节点成功");
this.open = false;
this.$emit('update')
});
}
}
});
},
initMap() {
let that = this;
const map = new BMap.Map("map-container", { enableMapClick: false });
this.map = map
console.log(888888888, this.form.communityLongitude, this.form.communityLatitude)
let point = new BMap.Point(
this.form.communityLongitude,
this.form.communityLatitude
);
this.map.centerAndZoom(point, 15);
if (!this.form.communityLongitude) {
var geolocation = new BMapGL.Geolocation();
geolocation.getCurrentPosition(function (r) {
if (this.getStatus() == BMAP_STATUS_SUCCESS) {
var mk = new BMapGL.Marker(r.point);
map.addOverlay(mk);
map.panTo(r.point);
point = new BMap.Point(r.point.lng, r.point.lat);
map.setCenter(point);
}
else {
console.log('failed' + this.getStatus());
}
});
}
this.map.enableScrollWheelZoom(true);
this.mk = new BMap.Marker(point, { enableDragging: true });
this.map.addOverlay(this.mk);
this.mk.addEventListener("dragend", function (e) {
that.getAddrByPoint(e.point);
});
this.map.addControl(
new BMap.NavigationControl({
anchor: BMAP_ANCHOR_TOP_RIGHT,
type: BMAP_NAVIGATION_CONTROL_LARGE,
})
);
this.map.addEventListener("click", function (e) {
that.getAddrByPoint(e.point);
});
},
getAddrByPoint(point) {
let that = this;
let geco = new BMap.Geocoder();
geco.getLocation(point, function (res) {
that.mk.setPosition(point);
that.map.panTo(point);
that.form.communityLongitude = point.lng;
that.form.communityLatitude = point.lat;
if (res.surroundingPois.length != 0) {
that.form.communityAddress =
res.surroundingPois[0].address + res.surroundingPois[0].title;
} else {
that.form.communityAddress = res.address;
}
});
},
querySearchAsync(str, cb) {
let options = {
onSearchComplete: function (res) {
let s = [];
if (local.getStatus() == BMAP_STATUS_SUCCESS) {
for (let i = 0; i < res.getCurrentNumPois(); i++) {
s.push(res.getPoi(i));
}
cb(s);
} else {
cb(s);
}
},
};
let local = new BMap.LocalSearch(this.map, options);
local.search(str);
},
handleSelect(item) {
this.form.communityAddress = item.address + item.title;
this.form.communityLongitude = item.point.lng;
this.form.communityLatitude = item.point.lat;
this.map.clearOverlays();
this.mk = new BMap.Marker(item.point);
this.map.addOverlay(this.mk);
this.map.panTo(item.point);
},
},
};
</script>
<style lang="scss" scoped>
::v-deep .el-autocomplete {
width: 100%;
}
::v-deep .el-button {
cursor: default;
}
.app-container {
.autoAddressClass {
li {
i.el-icon-search {
margin-top: 11px;
}
.mgr10 {
margin-right: 10px;
}
.title {
text-overflow: ellipsis;
overflow: hidden;
}
.address {
line-height: 1;
font-size: 12px;
color: #b4b4b4;
margin-bottom: 5px;
}
}
}
}
</style>