显示loading–mySpin
如何使用
{mySpin({ spinning: checking, tip: "校验中" })}
export function mySpin(params) {
const { spinning = false, tip = "加载中..." } = params;
if (!spinning) return "";
return (
<div
style={{
position: "absolute",
width: "100%",
height: "100%",
display: "flex",
alignItems: "center",
justifyContent: "center",
zIndex: 999,
background: "rgba(255,255,255,0.5)",
}}
>
<Spin tip={tip} spinning={spinning} />
</div>
);
}
下载文件–文件流–exportFile
使用情况是只需要传下载文件的url,入参就可以把文件下载下来,文件名是后端的
export async function exportFile(params, total = 1000) {
const { url, payload, fileName = "未命名", time = 10 } = params;
addLoading("正在下载文件,请稍后...");
request(
url,
{ ...payload },
(res) => {
const { data, headers } = res;
let blob = new Blob([res.data]);
if (headers["content-disposition"] || fileName) {
let name = headers["content-disposition"] ? headers["content-disposition"].replace(
/attachment;filename=/,
""
) : fileName;
name = decodeURIComponent(name) || fileName;
saveAs(blob, name);
} else if(size>0){
saveAs(blob, fileName);
} else {
let reader = new FileReader();
reader.readAsText(data, "utf-8");
reader.onload = function () {
let data1 = JSON.parse(reader.result);
message.warning(data1.message);
};
}
},
(err) => {
removeLoading();
message.warning(err.message || "下载失败!");
},
true
);
}
通用表格 comTable
在and表格的基础上进行扩展
import React, { useState, useEffect } from "react";
import { TableProps as RcTableProps } from "rc-table/lib/Table";
import { Button, Modal, message, Table } from "antd";
import ComPagination from "./comPaginationX";
import "../../style/comTable.scss";
import { throttling } from "../../util/x_util";
interface tableProps extends RcTableProps {
dropdownPrefixCls?: string;
dataSource?: Array<any>;
columns?: any;
pagination?:
| false
| {
pageSize?: number;
current?: number;
total?: number;
pageChange?: (pageNum: number, pageSize: number) => void;
sizeChange?: (pageNum: number, pageSize: number) => void;
};
loading?: boolean;
size?: "small" | "middle" | "large" | undefined;
bordered?: boolean;
locale?: object;
onChange?: (pagination: any, filters: any, sorter: any, extra: any) => void;
rowSelection?: any;
getPopupContainer?: any;
scroll?: object;
sortDirections?: any;
showSorterTooltip?: any;
className?: string;
id?: string;
}
declare type ComTableProps = {
tableProps: tableProps;
extraProps?: {
extraEl?: string;
tableSpacing?: number;
distinguishRow?: boolean;
pageSpacing?: number;
minHeight?: number;
};
};
export default function ComTable(props: ComTableProps) {
const [tableHeight, setTableHeight] = useState(200);
const {
tableProps,
extraProps = { distinguishRow: true, minHeight: 100, pageSpacing: 30 },
} = props;
const { pagination, dataSource, footer } = tableProps;
const [fixedAntEmpty, setfixedAntEmpty] = useState(false);
let pageSpacing: number = 30;
if (extraProps.pageSpacing) {
pageSpacing = extraProps.pageSpacing;
}
const comPaginationProps = {
...pagination,
position: "right",
id: (tableProps.id || tableProps.className || "") + "Pagination",
pageChange: (pageNum: number, pageSize: number) => {
if (tableProps && !tableProps.loading) {
if (
pagination != false &&
typeof pagination.pageChange === "function"
) {
pagination.pageChange(pageNum, pageSize);
}
}
},
showSizeChanger: true,
showQuickJumper: false,
pageSizeOptions: ["20", "50", "100"],
};
async function getScrollY() {
setTimeout(async () => {
let paginationDiv = document.querySelector(
getElementName("paginationDiv")
) as HTMLElement;
let tfooterTbody = document.querySelector(
getElementName("tfooterTbody")
) as HTMLElement;
if (tfooterTbody) {
if (!tfooterTbody.classList.toString().includes("heji"))
tfooterTbody.classList.add("heji");
}
let elFooter = document.querySelector(
getElementName("tfooter")
) as HTMLElement;
let summary = document.querySelector(
getElementName("summary")
) as HTMLElement;
let elHeader = document.querySelector(
getElementName("theader")
) as HTMLElement;
let elBody4 = document.querySelector(
getElementName("tbody")
) as HTMLElement;
if (elHeader) {
let sHeight: number;
sHeight =
window.innerHeight -
elHeader.getBoundingClientRect().top -
elHeader.getBoundingClientRect().height;
if (paginationDiv) {
sHeight = sHeight - paginationDiv.getBoundingClientRect().height;
}
if (
extraProps &&
extraProps.extraEl &&
document.querySelector(extraProps.extraEl)
)
sHeight =
sHeight -
document.querySelector(extraProps.extraEl).getBoundingClientRect()
.height;
sHeight = sHeight - pageSpacing;
if (extraProps && extraProps.tableSpacing) {
sHeight = sHeight - extraProps.tableSpacing;
}
if (elFooter) {
sHeight = sHeight - elFooter.clientHeight;
}
if (summary) {
sHeight = sHeight - summary.clientHeight;
}
const prent = elHeader.parentNode.parentNode.parentNode as HTMLElement;
const f = elHeader.clientWidth > prent.clientWidth + 2;
if (fixedAntEmpty != f) {
setfixedAntEmpty(f);
}
let min = extraProps.minHeight || 100;
if (sHeight < min) {
sHeight = min;
}
setTableHeight(sHeight);
changeClassName();
}
const fixedEmpty = document.querySelector(
".fixed-ant-empty .ant-table-placeholder .ant-empty-normal"
)! as HTMLElement;
if (fixedEmpty && elBody4) {
fixedEmpty.style.left = `${
elBody4.clientWidth / 2 - 32 + elBody4.getBoundingClientRect().left
}px`;
}
}, 100);
}
function changeClassName() {
let paginationDiv = document.querySelector(
getElementName("paginationDiv")
) as HTMLElement;
let tfooterTbody = document.querySelector(
getElementName("tfooterTbody")
) as HTMLElement;
if (tfooterTbody) {
if (!tfooterTbody.classList.toString().includes("heji"))
tfooterTbody.classList.add("heji");
}
let elFooter = document.querySelector(
getElementName("tfooter")
) as HTMLElement;
let summary = document.querySelector(
getElementName("summary")
) as HTMLElement;
let elHeader = document.querySelector(
getElementName("theader")
) as HTMLElement;
let elBody4 = document.querySelector(
getElementName("tbody")
) as HTMLElement;
const prent = elHeader.parentNode.parentNode.parentNode as HTMLElement;
const hasHorizontalScroll = elHeader.clientWidth > prent.clientWidth + 2;
const hasVerticalScroll =
(elBody4.children[0] as HTMLElement).clientHeight > elBody4.clientHeight;
let container = document.querySelector(getElementName("container"));
const hasFooter =
elFooter != undefined && elFooter != null && elFooter.clientHeight > 0;
const hasHidescrollBar = hasFooter && hasHorizontalScroll;
if (hasHidescrollBar) {
document.querySelector(getElementName("tbody")).scrollLeft = 1;
document.querySelector(getElementName("tfooterTbody")).scrollLeft = 1;
}
updateName(container, "has-horizontal-scroll", hasHorizontalScroll);
updateName(container, "has-vertical-scroll", hasVerticalScroll);
updateName(container, "has-footer", hasFooter);
updateName(elBody4, "has-hidescrollBar", hasHidescrollBar);
}
function updateName(element: Element, classNmae: string, flag: boolean) {
if (element) {
if (flag) {
if (!element.classList.toString().includes(classNmae)) {
element.classList.add(classNmae);
}
} else {
element.classList.remove(classNmae);
}
}
}
function onScrollCapture(e: any) {
let parentList = getParentTag(e.target);
let isFooter =
parentList?.filter((a: any) => a.className == "ant-table-footer").length >
0;
let tbody = document.querySelector(getElementName("tbody"));
if (isFooter) {
tbody.scrollLeft = e.target.scrollLeft;
}
}
function getParentTag(startTag: any, parentTagList = []) {
if (!(startTag instanceof HTMLElement))
return console.error("receive only HTMLElement");
if ("BODY" !== startTag.parentElement.nodeName) {
parentTagList.push(startTag.parentElement);
return getParentTag(startTag.parentElement, parentTagList);
}
else return parentTagList;
}
function getElementName(name: string) {
const defaultName = ".ant-table";
const obj = {
tbody: ".ant-table-body",
theader: "table thead.ant-table-thead",
tbodytbody: ".ant-table-body table .ant-table-tbody",
tfooterTbody: ".ant-table-footer .ant-table-body",
tfooter: ".ant-table-footer",
summary: ".ant-table-summary",
container: ".ant-table-container",
};
if (name === "paginationDiv") {
return tableProps.id
? `#${tableProps.id}Pagination.comPagination`
: tableProps.className
? `.${tableProps.className}.comPagination`
: ".comPagination";
}
return tableProps.id
? `#${tableProps.id} ${obj[name]}`
: tableProps.className
? `.${tableProps.className} ${obj[name]}`
: `${defaultName} ${obj[name]}`;
}
useEffect(() => {
getScrollY();
}, [tableProps, extraProps]);
useEffect(() => {
getScrollY();
window.addEventListener("resize", throttling(getScrollY, 1000));
return () => {
window.removeEventListener("resize", throttling(getScrollY, 1000));
};
}, []);
let className = fixedAntEmpty
? `fixed-ant-empty ${tableProps.className || ""}`
: `${tableProps.className || ""}`;
return (
<div className="comTable" onScrollCapture={onScrollCapture}>
<Table
scroll={{ y: tableHeight }}
{...tableProps}
footer={(dataSource || []).length ? footer : null}
pagination={false}
className={className}
rowClassName={(row, index) => {
if (!(extraProps && extraProps.distinguishRow)) {
return "";
}
return index % 2 === 0 ? "evenrow" : "oddrow";
}}
/>
{pagination && dataSource.length ? (
<ComPagination {...comPaginationProps} />
) : (
""
)}
</div>
);
}
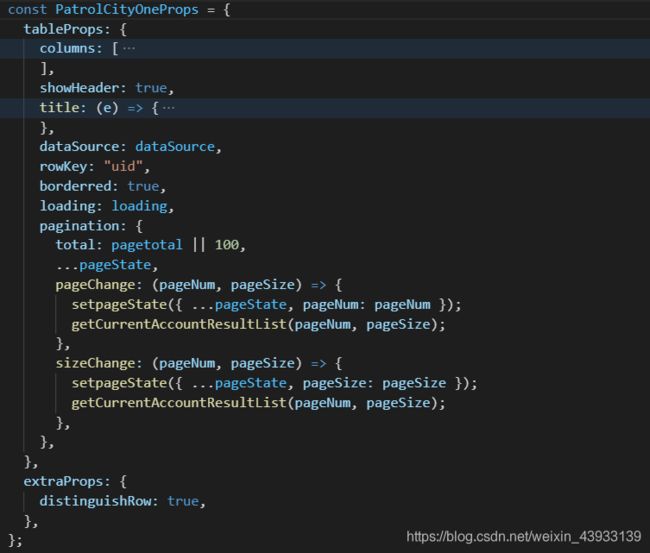