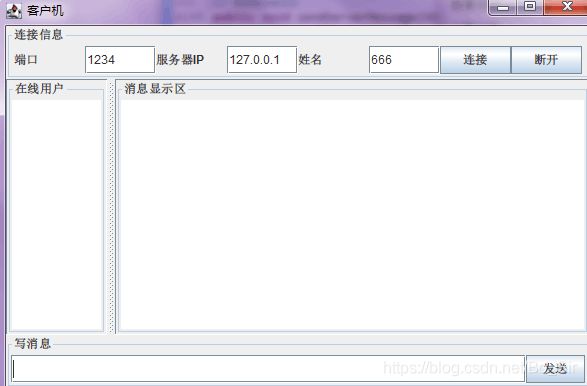
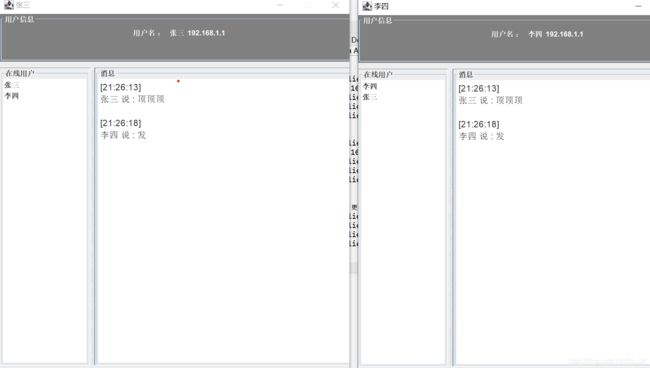
package Client;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.net.Socket;
import java.net.UnknownHostException;
import java.text.SimpleDateFormat;
import java.util.HashMap;
import java.util.Map;
import java.util.StringTokenizer;
import javax.sound.sampled.AudioFormat;
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.TargetDataLine;
import javax.swing.DefaultListModel;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.JTextPane;
import javax.swing.border.TitledBorder;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
import Pojo.UserInfo;
public class ChatClient extends JFrame{
private ChatClient chatClient = this;
private int screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;
private int screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;
private JTextPane txtShow;
private JTextPane txtShowPri;
private JTextField txtMsg;
private JLabel infoName;
private JLabel infoIp;
private JButton send;
private JButton clear;
private JButton record_start;
private JButton record_stop_send;
private JPanel nPanel;
private JPanel sPanel;
private JScrollPane rScroll;
private JScrollPane lScroll;
private JSplitPane Split;
private JComboBox<String> comboBox;
private SimpleAttributeSet attrset;
private DefaultListModel<String> listModel;
private JList<String> userList;
private Socket socket;
private static PrintWriter writer;
private static BufferedReader reader;
private MessageThread messageThread = null;
private Map<String, UserInfo> onLineUsers = new HashMap<String, UserInfo>();
private boolean isConnected = false;
private int port = 30000;
private String ip = "";
private String Userip = "";
private String name;
private String UserValue = "";
private int info_ip_ = 0;
public ChatClient(String userName,String ip) {
super(userName);
this.Userip = ip;
this.name = userName;
this.setVisible(true);
this.setResizable(false);
infoName = new JLabel(userName);
txtShow = new JTextPane();
txtShow.setEditable(false);
txtShowPri = new JTextPane();
txtShowPri.setEditable(false);
attrset = new SimpleAttributeSet();
StyleConstants.setFontSize(attrset, 15);
txtMsg = new JTextField();
send = new JButton("发送");
clear = new JButton("清屏");
record_start = new JButton("私聊");
record_stop_send = new JButton("群聊");
comboBox = new JComboBox<>();
comboBox.addItem("ALL");
listModel = new DefaultListModel<>();
userList = new JList<>(listModel);
nPanel = new JPanel();
nPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
JLabel infoNameTip = new JLabel("用户名 : ");
TitledBorder infoTitle = new TitledBorder("用户信息");
infoNameTip.setForeground(Color.WHITE);
infoName.setForeground(Color.WHITE);
infoTitle.setTitleColor(Color.WHITE);
nPanel.add(infoNameTip);
nPanel.add(infoName);
nPanel.setBorder(infoTitle);
rScroll = new JScrollPane(txtShow);
TitledBorder txtTitle = new TitledBorder("消息");
txtTitle.setTitleColor(Color.DARK_GRAY);
rScroll.setBorder(txtTitle);
lScroll = new JScrollPane(userList);
TitledBorder userTitle = new TitledBorder("在线用户");
userTitle.setTitleColor(Color.DARK_GRAY);
lScroll.setBorder(userTitle);
sPanel = new JPanel(new BorderLayout());
sPanel.setLayout(null);
txtMsg.setBounds(0,0,600,100);
txtMsg.setBackground(Color.GRAY);
send.setBounds(420, 110, 150, 35);
send.setForeground(Color.DARK_GRAY);
comboBox.setBounds(30, 110, 100, 35);
comboBox.setForeground(Color.DARK_GRAY);
clear.setBounds(160, 110, 100, 35);
clear.setForeground(Color.DARK_GRAY);
sPanel.add(txtMsg);
sPanel.add(send);
sPanel.add(comboBox);
sPanel.add(clear);
sPanel.add(record_start);
Split = new JSplitPane(JSplitPane.HORIZONTAL_SPLIT, lScroll, rScroll);
Split.setDividerLocation(150);
this.setLayout(null);
nPanel.setBounds(0,0,600,80);
nPanel.setBackground(Color.GRAY);
Split.setBounds(0, 90, 600, 500);
sPanel.setBounds(0,600,600,200);
this.add(nPanel);
this.add(Split);
this.add(sPanel);
this.setBounds(0, 0, 600, 800);
screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;
screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;
this.setLocation((screenWidth - chatClient.getWidth()) / 2, (screenHeight - chatClient.getHeight()) / 2);
this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
ConnectServer();
txtMsg.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
ComboBoxValue();
}
});
send.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
ComboBoxValue();
}
});
clear.addActionListener(new ClearListener(txtShow));
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
if (isConnected) {
try {
boolean flag = ConnectClose();
if (flag == false) {
throw new Exception("断开连接发生异常!");
} else {
JOptionPane.showMessageDialog(chatClient, "成功断开!");
txtMsg.setEnabled(false);
send.setEnabled(false);
}
} catch (Exception e4) {
JOptionPane.showMessageDialog(chatClient, "断开连接服务器异常:" + e4.getMessage(), "错误",
JOptionPane.ERROR_MESSAGE);
}
} else if (!isConnected) {
ConnectServer();
txtMsg.setEnabled(true);
send.setEnabled(true);
}
}
});
comboBox.addItemListener(new ItemListener() {
public void itemStateChanged(ItemEvent evt) {
try {
if (ItemEvent.SELECTED == evt.getStateChange()) {
String value = comboBox.getSelectedItem().toString();
System.out.println(value);
UserValue = value;
}
} catch (Exception e) {
System.out.println("GGGFFF");
}
}
});
}
public static void sendMessage(String message) {
writer.println(message);
writer.flush();
}
private void ConnectServer() {
try {
socket = new Socket(ip, port);
writer = new PrintWriter(new OutputStreamWriter(socket.getOutputStream()));
reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
infoIp = new JLabel(Userip);
infoIp.setForeground(Color.WHITE);
if (info_ip_ == 0) {
nPanel.add(infoIp);
JOptionPane.showMessageDialog(this, name + " 连接服务器成功!");
}
info_ip_++;
sendMessage(name + "@" + "IP" + "@" + Userip);
sendMessage(name + "@" + "ADD");
sendMessage(name + "@" + "USERLIST");
sendMessage(name + "@" + "Login"+"@"+ Userip);
messageThread = new MessageThread(reader);
messageThread.start();
isConnected = true;
this.setVisible(true);
} catch (Exception e) {
isConnected = false;
JOptionPane.showMessageDialog(this, "连接服务器异常:" + e.getMessage(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
@SuppressWarnings("deprecation")
public synchronized boolean ConnectClose() {
try {
sendMessage(name + "@" + "DELETE");
messageThread.stop();
if (reader != null) {
reader.close();
}
if (writer != null) {
writer.close();
}
if (socket != null) {
socket.close();
}
isConnected = false;
return true;
} catch (IOException e) {
JOptionPane.showMessageDialog(this, name + " 断开连接服务器失败!");
isConnected = true;
return false;
}
}
public void ComboBoxValue() {
sendMessage(name + "@" + "USERLIST");
String message = txtMsg.getText();
if (message == null || message.equals("")) {
JOptionPane.showMessageDialog(this, "消息不能为空!", "错误", JOptionPane.ERROR_MESSAGE);
return;
}
if (UserValue.equals("ALL")) {
sendMessage(this.getTitle() + "@" + "ALL" + "@" + message + "@" + "not");
} else {
sendMessage(this.getTitle() + "@" + comboBox.getSelectedItem().toString() + "@" + message + "@" + "not");
}
txtMsg.setText(null);
}
class MessageThread extends Thread {
private BufferedReader reader;
public MessageThread(BufferedReader reader) {
this.reader = reader;
}
public void run() {
String message = "";
while (true) {
try {
message = reader.readLine();
StringTokenizer stringTokenizer = new StringTokenizer(message, "/@");
String[] str_msg = new String[10];
int j = 0;
while (stringTokenizer.hasMoreTokens()) {
str_msg[j++] = stringTokenizer.nextToken();
}
String command = str_msg[1];
if (command.equals("SERVERClOSE")) {
Document docs = txtShow.getDocument();
try {
docs.insertString(docs.getLength(), "服务器已关闭!\\r\\n", attrset);
} catch (BadLocationException e) {
e.printStackTrace();
}
closeCon();
return;
}
else if (command.equals("Login")) {
String username = "";
String userIp = "";
username = str_msg[0];
userIp = str_msg[2];
UserInfo user = new UserInfo(username, userIp);
System.out.print("更新上线列表");
onLineUsers.put(username, user);
listModel.addElement(username);
comboBox.addItem(username);
}
else if (command.equals("Logout")) {
String username = str_msg[0];
UserInfo user = (UserInfo) onLineUsers.get(username);
onLineUsers.remove(user);
listModel.removeElement(username);
comboBox.removeItem(username);
}
else if (command.equals("USERLIST")) {
String username = null;
String userIp = null;
for (int i = 2; i < str_msg.length; i += 2) {
if (str_msg[i] == null)
break;
username = str_msg[i];
userIp = str_msg[i + 1];
UserInfo user = new UserInfo(username, userIp);
onLineUsers.put(username, user);
if (listModel.contains(username))
;
else
listModel.addElement(username);
int len = comboBox.getItemCount();
int _i = 0;
for (; _i < len; _i++) {
if (comboBox.getItemAt(_i).toString().equals(username))
break;
}
if (_i == len)
comboBox.addItem(username);
else
;
}
}
else if (command.equals("MAX")) {
closeCon();
JOptionPane.showMessageDialog(chatClient, "服务器人数已满,请稍后再试!", "提示", JOptionPane.CANCEL_OPTION);
return;
}
else if (command.equals("ALL")) {
SimpleDateFormat df = new SimpleDateFormat("HH:mm:ss");
String time = df.format(new java.util.Date());
Document docs = txtShow.getDocument();
try {
docs.insertString(docs.getLength(),
"[" + time + "]\r\n" + str_msg[0] + " 说 : " + str_msg[2] + "\r\n\n", attrset);
} catch (BadLocationException e) {
e.printStackTrace();
}
}
else if (command.equals("ONLY")) {
SimpleDateFormat df = new SimpleDateFormat("HH:mm:ss");
String time = df.format(new java.util.Date());
Document docs = txtShow.getDocument();
try {
docs.insertString(docs.getLength(), "[" + time + "]\r\n" + str_msg[0] + "\r\n\n",
attrset);
} catch (BadLocationException e) {
e.printStackTrace();
}
}
str_msg = null;
}
catch (IOException e1) {
e1.printStackTrace();
System.out.println("客户端接受 消息 线程 run() e1:" + e1.getMessage());
break;
} catch (Exception e2) {
e2.printStackTrace();
System.out.println("客户端接收 消息 线程 run() e2:" + e2.getMessage());
break;
}
}
}
public synchronized void closeCon() throws Exception {
listModel.removeAllElements();
if (reader != null) {
reader.close();
}
if (writer != null) {
writer.close();
}
if (socket != null) {
socket.close();
}
isConnected = false;
}
}
public class ClearListener implements ActionListener{
private JTextPane txtShow;
public ClearListener(JTextPane txtShow) {
this.txtShow = txtShow;
}
@Override
public void actionPerformed(ActionEvent e) {
txtShow.setText("");
}
}
}
更多信息关注微信公众号:长长的路我们慢慢走吧
回复:Java考试系统