<template>
<div>
<el-table :data="tableData" style="width: 100%">
<template v-for="(col, index) in tableHeader">
<el-table-column
:prop="col.columnName"
:key="index"
:label="col.comments"
row-class-name="tableRow"
>
<template slot="header" slot-scope="scope">
<li
v-show="showWaring(col, scope)"
class="el-icon-warning waring"
></li>
<span>{{ col.comments }}</span>
<span v-if="col.columnName === 'VALUE_DATE'">
<el-popover width="100" trigger="click" placement="right">
<div>
<el-checkbox v-model="dateFormat" class="mr10">
日期格式化
</el-checkbox>
</div>
<div>
<el-button
class="right"
size="mini"
@click="formateDate(col, scope)"
>
确认
</el-button>
</div>
<li
slot="reference"
v-if="col.columnName === 'VALUE_DATE'"
class="el-icon-s-open point"
></li>
</el-popover>
</span>
<span v-else-if="col.columnName === 'numberOne'">
<el-popover width="100" trigger="click" placement="right">
<el-checkbox-group v-model="checkList">
<el-checkbox label="去除%"></el-checkbox>
<el-checkbox label="NA替换"></el-checkbox>
<el-checkbox label="展示负号"></el-checkbox>
</el-checkbox-group>
<div>
<el-button
class="right"
size="mini"
@click="formateNumOne(col, scope)"
>
确认
</el-button>
</div>
<li
slot="reference"
v-if="col.columnName === 'numberOne'"
class="el-icon-s-open point"
></li>
</el-popover>
</span>
<span v-else-if="col.columnName === 'numberTwo'">
<el-popover width="100" trigger="click" placement="right">
<el-checkbox-group v-model="checkListTwo">
<el-checkbox label="去除,"></el-checkbox><br />
<el-checkbox label="去除-"></el-checkbox>
</el-checkbox-group>
<div>
<el-button
class="right"
size="mini"
@click="formateNumTwo(col, scope)"
>
确认
</el-button>
</div>
<li
slot="reference"
v-if="col.columnName === 'numberTwo'"
class="el-icon-s-open point"
></li>
</el-popover>
</span>
</template>
<template slot-scope="scope">
<span
:class="
warningCell(scope.row[col.columnName], col.columnName)
? 'waringcell'
: ''
"
>
{{ scope.row[col.columnName] }}
</span>
</template>
</el-table-column>
</template>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
// 数据格式正则校验匹配
REGEX_NA: /(NA)|(N\.A)|(na)|(n\.a)/g, //na NA n.a. N.A替换
REGEX_S: /\s+/g, //替换所有空格
REGEX_FU: /(^-$)|(^-$)/g, //替换-号(这里的替换的只是 - )
REGEX_PERCENT: /%/g, //百分号
REGEX_COMMA: /(,)|(,)/g, //去掉逗号
REGEX_SPECIAL: /(?<=\(|().*(?=\)|))/g, //有(A),有则,替换为 -A
REGEX_VALUEDATE_1: /^(\d{2}|\d{4})-\d{1,2}-\d{1,2}$/g, //YYYY-MM-DD 改为 YYYYMMDD
REGEX_VALUEDATE_2: /^(\d{2}|\d{4})\/\d{1,2}\/\d{1,2}$/g, //YYYY/MM/DD 改为 YYYYMMDD
REGEX_VALUEDATE_3: /^(\d{2}|\d{4})年\d{1,2}月\d{1,2}日$/g, //YYYY年MM月DD日(以及YYYY年M月DD日,YYYY年MM月D日,YYYY年M月D日) 改为 YYYYMMDD
REGEX_VALUEDATE_4: /^(\d{2}|\d{4})-\d{1,2}$/g, //YYYY-M-D,YYYY-MM-D,YYYY-M-DD 改为 YYYYMMDD
REGEX_VALUEDATE_5: /^(\d{2}|\d{4})\/\d{1,2}$/g, //YYYY/M/D,YYYY/MM/D,YYYY/M/DD 改为 YYYYMMDD
REGEX_VALUEDATE_6: /^(\d{2}|\d{4})年\d{1,2}月$/g,
REGEX_VALUEDATE_7: /^\d{2}$/g,
REGEX_VALUEDATE_8: /^(\d{2}|\d{4})年$/g,
REGEX_VALUEDATE_EXEC: /-|\/|年|月|日/g, //包含-/年月日替换
checkList: [],
checkListTwo: [],
dateFormat: true,
tableHeader: [
{
comments: "数据日期",
columnName: "VALUE_DATE",
dataType: "VARCHAR2(100)",
},
{
comments: "数值型字段1",
columnName: "numberOne",
dataType: "NUMBER",
},
{
comments: "数值型字段2",
columnName: "numberTwo",
dataType: "NUMBER",
},
],
tableData: [
{
VALUE_DATE: "2021年09月09日",
numberOne: "na",
numberTwo: "11,11",
},
{
VALUE_DATE: "2021/09/09",
numberOne: "NA",
numberTwo: "-",
},
{
VALUE_DATE: "2021-08-08",
numberOne: "n.a",
numberTwo: "123",
},
{
VALUE_DATE: "2021年09月9日",
numberOne: "N.A",
numberTwo: "12-12",
},
{
VALUE_DATE: "2021年9月9日",
numberOne: "1 5",
numberTwo: "-",
},
{
VALUE_DATE: "20年09月09日",
numberOne: "22%",
numberTwo: "-",
},
{
VALUE_DATE: "20220909",
numberOne: "(4.2)",
numberTwo: "11,11",
},
],
};
},
mounted() {},
methods: {
showWaring(col, scope) {
//日期格式
for (let i = 0; i < this.tableData.length; i++) {
if (
this.warningCell(this.tableData[i]["VALUE_DATE"], "VALUE_DATE") &&
col.columnName === "VALUE_DATE"
) {
return true;
} else if (
this.warningCell(this.tableData[i]["numberOne"], "numberOne") &&
col.columnName === "numberOne"
) {
return true;
} else if (
this.warningCell(this.tableData[i]["numberTwo"], "numberTwo") &&
col.columnName === "numberTwo"
) {
return true;
}
}
return false;
},
warningCell(val, columnName) {
if (
columnName === "VALUE_DATE" &&
(this.REGEX_VALUEDATE_1.test(val) ||
this.REGEX_VALUEDATE_2.test(val) ||
this.REGEX_VALUEDATE_3.test(val) ||
this.REGEX_VALUEDATE_4.test(val) ||
this.REGEX_VALUEDATE_5.test(val) ||
this.REGEX_VALUEDATE_6.test(val) ||
this.REGEX_VALUEDATE_7.test(val) ||
this.REGEX_VALUEDATE_8.test(val))
) {
this.REGEX_VALUEDATE_1.lastIndex = 0;
this.REGEX_VALUEDATE_2.lastIndex = 0;
this.REGEX_VALUEDATE_3.lastIndex = 0;
this.REGEX_VALUEDATE_4.lastIndex = 0;
this.REGEX_VALUEDATE_5.lastIndex = 0;
this.REGEX_VALUEDATE_6.lastIndex = 0;
this.REGEX_VALUEDATE_7.lastIndex = 0;
this.REGEX_VALUEDATE_8.lastIndex = 0;
return true;
} else if (
columnName === "numberOne" &&
(this.REGEX_PERCENT.test(val) ||
this.REGEX_NA.test(val) ||
this.REGEX_SPECIAL.test(val))
) {
this.REGEX_PERCENT.lastIndex = 0;
this.REGEX_NA.lastIndex = 0;
this.REGEX_SPECIAL.lastIndex = 0;
return true;
} else if (
columnName === "numberTwo" &&
(this.REGEX_COMMA.test(val) || this.REGEX_FU.test(val))
) {
this.REGEX_COMMA.lastIndex = 0;
this.REGEX_FU.lastIndex = 0;
return true;
}
return false;
},
formateDate(col, scope) {
for (let i = 0; i < this.tableData.length; i++) {
if (col.columnName === "VALUE_DATE") {
let val = this.tableData[i]["VALUE_DATE"];
if (this.warningCell(val, "VALUE_DATE")) {
//删除可能存在的空字符串
let res = val.split(this.REGEX_VALUEDATE_EXEC);
res = res.filter((item) => item !== "");
if (res.length === 1) {
this.tableData[i]["VALUE_DATE"] = this.formateY(res[0]);
} else if (res.length === 2) {
this.tableData[i]["VALUE_DATE"] =
this.formateY(res[0]) + this.formatM(res[1]);
} else if (res.length) {
this.tableData[i]["VALUE_DATE"] =
this.formateY(res[0]) +
this.formatM(res[1]) +
this.formatD(res[2]);
}
}
}
}
},
/**
* 年补零
* @param {*} val
* @returns
*/
formateY(val) {
if (val && val.length === 2) {
return "20" + val;
} else {
return val;
}
},
/**
* 月补零
* @param {*} val
* @returns
*/
formatM(val) {
if (val && val.length === 1) {
return "0" + val;
} else {
return val;
}
},
/**
* 日补零
* @param {*} val
* @returns
*/
formatD(val) {
if (val && val.length === 1) {
return "0" + val;
} else {
return val;
}
},
formateNumOne(col, scoped) {
for (let i = 0; i < this.tableData.length; i++) {
if (this.checkList.includes("去除%")) {
this.tableData[i].numberOne = this.tableData[i].numberOne.replace(
this.REGEX_PERCENT,
""
);
}
if (this.checkList.includes("NA替换")) {
this.tableData[i].numberOne = this.tableData[i].numberOne.replace(
this.REGEX_NA,
""
);
}
if (this.checkList.includes("展示负号")) {
let matchRes = this.tableData[i].numberOne.match(this.REGEX_SPECIAL);
if (matchRes && matchRes.length > 0) {
if (matchRes[0].indexOf("-") > -1) {
this.tableData[i].numberOne = matchRes[0].replace("-", "");
} else {
this.tableData[i].numberOne = "-" + matchRes[0];
}
}
}
}
},
formateNumTwo(col, scoped) {
for (let i = 0; i < this.tableData.length; i++) {
if (this.checkListTwo.includes("去除,")) {
this.tableData[i].numberTwo = this.tableData[i].numberTwo.replace(
this.REGEX_COMMA,
""
);
}
if (this.checkListTwo.includes("去除-")) {
this.tableData[i].numberTwo = this.tableData[i].numberTwo.replace(
this.REGEX_FU,
""
);
}
}
},
},
};
</script>
<style lang="less">
.waring {
color: red;
}
.waringcell {
color:
font-weight: bold;
}
</style>
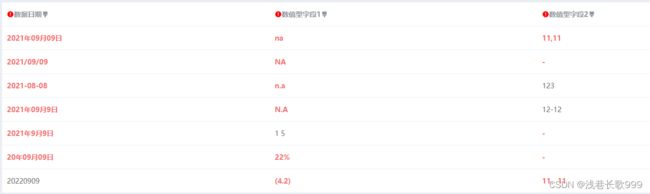
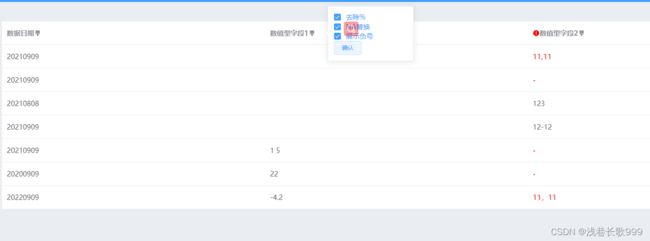