输入
数字输入(为什么类型的数字就是sc.next类型)float sum=sc.nextFloat() int x=sc.nextInt();
import java.util.Scanner;
public class 相加问题 {
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
int x=sc.nextInt();
int y=sc.nextInt();
System.out.print(x+y);
}
}
单个字符输入
import java.util.Scanner;
public class 字母转化 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
char x=sc.next().charAt(0);
char y=(char)(x-('a'-'A'));
System.out.print(y);
}
}
字符串输入
import java.util.Scanner;
public class 数字翻转 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
String str=sc.next();
int len=str.length();
for (int i=len-1;i>=0;i--)
{
System.out.print(str.charAt(i));
}
//System.out.print(len);
}
}
保留几位小数
double num = 123.4567899; System.out.print(String.format("%.2f", num)); //直接在print中使用
逻辑运算符
& | !与c语言是一样的
^ 异或
如果两个表达式一个是false一个是true 那么这个表达式就是true
如果两个表达式都是false或者true,那么这个表达式就是false
短路逻辑运算符
&&
如果左边为false那么右边就不进行了
||
如果有左边为true那么右边就不进行了
一些题目
# 超级玛丽游戏
## 题目背景
本题是洛谷的试机题目,可以帮助了解洛谷的使用。
建议完成本题目后继续尝试 [P1001](/problem/P1001)、[P1008](/problem/P1008)。
另外强烈推荐[新用户必读贴](/discuss/show/241461)
## 题目描述
超级玛丽是一个非常经典的游戏。请你用字符画的形式输出超级玛丽中的一个场景。
```
********
************
####....#.
#..###.....##....
###.......###### ### ###
........... #...# #...#
##*####### #.#.# #.#.#
####*******###### #.#.# #.#.#
...#***.****.*###.... #...# #...#
....**********##..... ### ###
....**** *****....
#### ####
###### ######
##############################################################
#...#......#.##...#......#.##...#......#.##------------------#
###########################################------------------#
#..#....#....##..#....#....##..#....#....#####################
########################################## #----------#
#.....#......##.....#......##.....#......# #----------#
########################################## #----------#
#.#..#....#..##.#..#....#..##.#..#....#..# #----------#
########################################## ############
```
## 输入格式
无
## 输出格式
如描述
## 提示
**广告**
洛谷出品的算法教材,帮助您更简单的学习基础算法。[【官方网店绝赞热卖中!】>>>](https://item.taobao.com/item.htm?id=637730514783)
[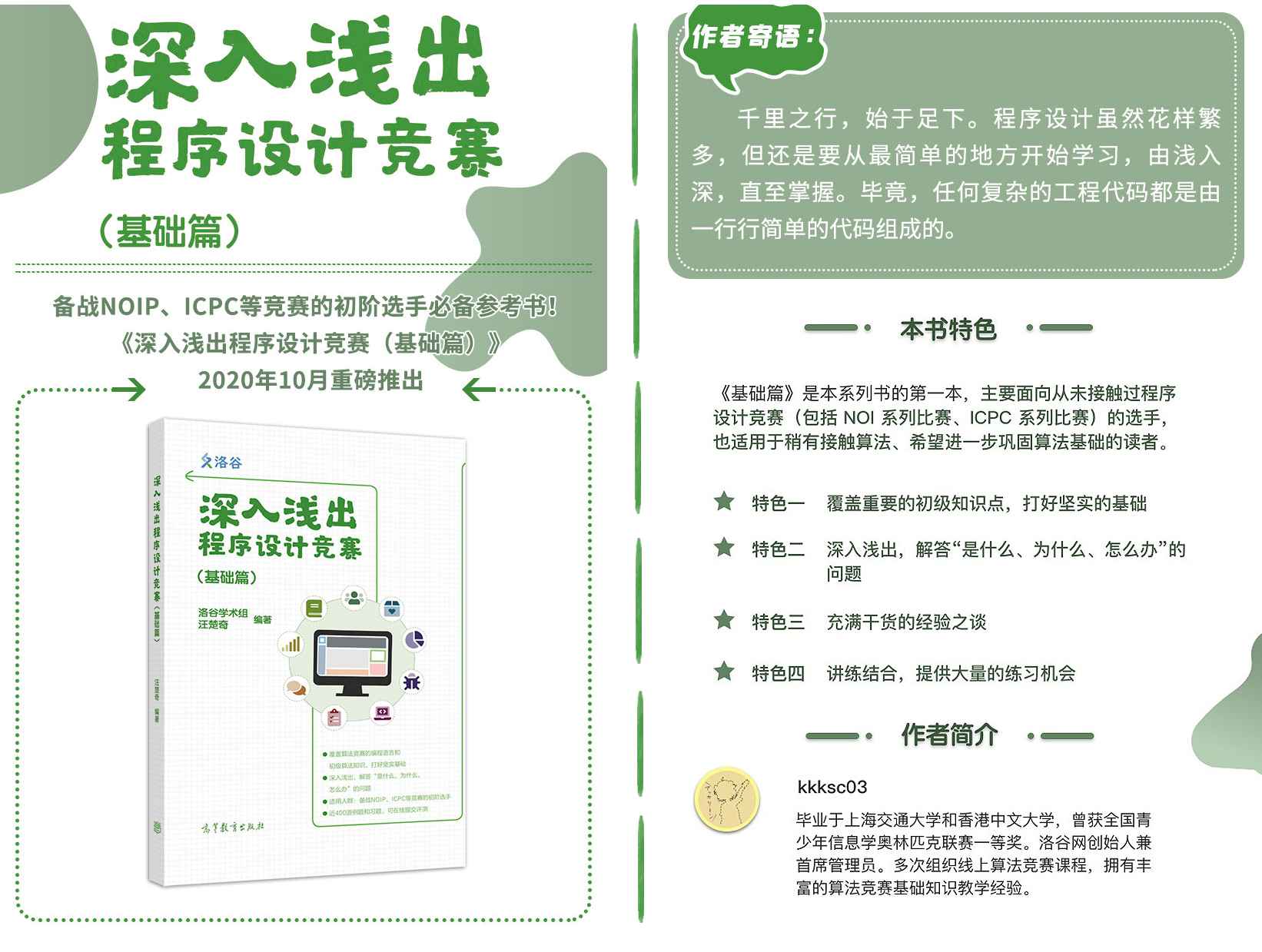](https://item.taobao.com/item.htm?id=637730514783)
题解:就是简单是输出,为了熟悉java写的
// 按两次 Shift 打开“随处搜索”对话框并输入 `show whitespaces`,
// 然后按 Enter 键。现在,您可以在代码中看到空格字符。
public class Main {
public static void main(String[] args) {
System.out.print(" ********\n" +
" ************\n" +
" ####....#.\n" +
" #..###.....##....\n" +
" ###.......###### ### ###\n" +
" ........... #...# #...#\n" +
" ##*####### #.#.# #.#.#\n" +
" ####*******###### #.#.# #.#.#\n" +
" ...#***.****.*###.... #...# #...#\n" +
" ....**********##..... ### ###\n" +
" ....**** *****....\n" +
" #### ####\n" +
" ###### ######\n" +
"##############################################################\n" +
"#...#......#.##...#......#.##...#......#.##------------------#\n" +
"###########################################------------------#\n" +
"#..#....#....##..#....#....##..#....#....#####################\n" +
"########################################## #----------#\n" +
"#.....#......##.....#......##.....#......# #----------#\n" +
"########################################## #----------#\n" +
"#.#..#....#..##.#..#....#..##.#..#....#..# #----------#\n" +
"########################################## ############");
}
}
# 字符三角形
## 题目描述
给定一个字符,用它构造一个底边长 $5$ 个字符,高 $3$ 个字符的等腰字符三角形。
## 输入格式
输入只有一行,包含一个字符。
## 输出格式
该字符构成的等腰三角形,底边长 $5$ 个字符,高 $3$ 个字符。
## 样例 #1
### 样例输入 #1
```
*
```
### 样例输出 #1
```
*
***
*****
```
## 提示
对于 $100 \%$ 的数据,输入的字符是 ASCII 中的可见字符。
import java.util.Scanner;
public class Main {
public static void main(String[] args){
Scanner sc=new Scanner(System.in);
char x=sc.next().charAt(0);
System.out.print(" "+x+" \n");
System.out.print(" "+x+x+x+" \n");
System.out.print(""+x+x+x+x+x);
}
}
# 【深基2.例6】字母转换
## 题目描述
输入一个小写字母,输出其对应的大写字母。例如输入 q[回车] 时,会输出 Q。
## 输入格式
## 输出格式
## 样例 #1
### 样例输入 #1
```
q
```
### 样例输出 #1
```
Q
```
题解:强制类型转化
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
char x=sc.next().charAt(0);
char y=(char)(x-('a'-'A'));
System.out.print(y);
}
}
# 【深基2.例7】数字反转
## 题目描述
输入一个不小于 $100$ 且小于 $1000$,同时包括小数点后一位的一个浮点数,例如 $123.4$ ,要求把这个数字翻转过来,变成 $4.321$ 并输出。
## 输入格式
一行一个浮点数
## 输出格式
一行一个浮点数
## 样例 #1
### 样例输入 #1
```
123.4
```
### 样例输出 #1
```
4.321
```
题解:字符串的输入输出
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
String str=sc.next();
int len=str.length();
for (int i=len-1;i>=0;i--)
{
System.out.print(str.charAt(i));
}
//System.out.print(len);
}
}
明天把剩下的写完
下班下班!