基本sql语句练习
1.学生表的基本操作
老老实实把下面的打完,相信我。
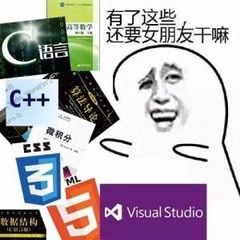
CREATE TABLE t_stu(
stu_id BIGINT PRIMARY KEY auto_increment COMMENT '学生id',
stu_name VARCHAR(50) not null DEFAULT '马云' COMMENT '学生姓名',
stu_no VARCHAR(30) UNIQUE not null COMMENT '学号',
stu_age int COMMENT '年龄',
stu_birth TIMESTAMP DEFAULT NOW() COMMENT '生日'
)ENGINE=INNODB auto_increment=1000 charset=utf8 COMMENT '学生表';
INSERT into t_stu(stu_no,stu_age) VALUES('20190101',25);
INSERT into t_stu(stu_no,stu_age,stu_birth) VALUES('20190102',25,'2000-10-10');
select * from t_stu;
INSERT into t_stu(stu_name,stu_no,stu_age)
values('x1','20190108',25),('x3','20190104',26),('x6','20190107',20);
INSERT into t_stu value(2000,'x8','20190105',25,'2000-11-11');
INSERT into t_stu(stu_no,stu_age) VALUES('20190110',30);
CREATE table stu2(
stu_no VARCHAR(30) not null,
stu_name varchar(30) not null,
PRIMARY key(stu_no)
);
INSERT into stu2 values('1','a'),('2','b');
SELECT stu_no,stu_name from stu2;
CREATE table stu3(
stu_no VARCHAR(30) not null,
stu_name varchar(30) not null,
PRIMARY key(stu_no,stu_name)
);
CREATE table course(
course_id int auto_increment PRIMARY key,
course_name VARCHAR(30) not null UNIQUE,
update_time TIMESTAMP DEFAULT NOW()
);
CREATE table stu_course(
stu_id BIGINT,
cour_id int,
PRIMARY key(stu_id,cour_id),
CONSTRAINT FOREIGN key(stu_id) REFERENCES t_stu(stu_id),
constraint foreign key(cour_id) REFERENCES course(course_id)
);
select * from t_stu;
select * from course;
INSERT into course(course_id,course_name)
values(200,'java'),(300,'html'),(400,'js')
insert INTO stu_course(stu_id,cour_id)
values(1002,200),(1002,400),(1003,300),(1003,400)
select * from stu_course
select * from t_stu,stu_course,course WHERE t_stu.stu_id = stu_course.stu_id AND
stu_course.cour_id = course.course_id
select stu_name,course_name from t_stu,stu_course,course WHERE t_stu.stu_id = stu_course.stu_id AND
stu_course.cour_id = course.course_id and t_stu.stu_name = 'x1'
select stu_id from t_stu where stu_name = 'x1'
select cour_id from stu_course where stu_id =
(select stu_id from t_stu where stu_name = 'x1')
select course_name from course where course_id in (
select cour_id from stu_course where stu_id =
(select stu_id from t_stu where stu_name = 'x1')
)
select s.*,sc.*,c.* from t_stu s
INNER JOIN stu_course sc ON s.stu_id = sc.stu_id
INNER JOIN course c on sc.cour_id = c.course_id
where s.stu_name = 'x1';
SELECT s.stu_id id, s.stu_name as name,s.stu_no as '学号' from t_stu s;
SELECT * from t_stu;
select * from stu_course;
SELECT * from t_stu s LEFT JOIN stu_course sc ON s.stu_id = sc.stu_id
SELECT * from stu_course sc LEFT JOIN t_stu s ON s.stu_id = sc.stu_id
SELECT * from t_stu s right JOIN stu_course sc ON s.stu_id = sc.stu_id
SELECT * from stu_course sc right JOIN t_stu s ON s.stu_id = sc.stu_id
select * from t_stu;
update t_stu set stu_age = 25 where stu_age >= 20 and stu_age < 26
DELETE from t_stu where stu_no = '20190110';
select * from t_stu where 1=1 ;
select count(*) nums from t_stu where 1 = 1;
select count(stu_id) nums from t_stu where 1 = 1;
select count(stu_age) nums from t_stu where 1 = 1;
select count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1;
select stu_id,count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1;
select * from t_stu;
select stu_id,count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1 GROUP BY stu_age;
select * from t_stu;
select stu_id,count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1 and stu_id > 1001 GROUP BY stu_age;
select * from t_stu;
select stu_id,count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1 GROUP BY stu_age HAVING stu_id > 1001;
select * from t_stu;
select stu_id,count(*),MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1 GROUP BY stu_age HAVING count(*) > 1;
select * from t_stu;
select stu_id,count(*) nums,MIN(stu_age),MAX(stu_age),SUM(stu_age),AVG(stu_age) from
t_stu where 1 = 1 and stu_id > 1001 GROUP BY stu_age ORDER BY nums ASC;
SELECT * from course;
select * from stu_course;
select * from t_stu;
select count(*) nums from course
select stu_id ,count(*) from stu_course GROUP BY stu_id
select stu_id ,count(*) from stu_course GROUP BY stu_id HAVING count(*) = (
select count(*) nums from course
)
select stu_name from t_stu where stu_id in (
select stu_id from stu_course GROUP BY stu_id HAVING count(*) = (
select count(*) nums from course
)
)
select s.stu_id,s.stu_name,count(*) from t_stu s
INNER JOIN stu_course sc on s.stu_id = sc.stu_id
INNER JOIN course c on sc.cour_id = c.course_id
GROUP BY s.stu_id,s.stu_name
HAVING count(*) = (select count(*) nums from course)
select *,CONCAT(stu_name,'-',stu_no) from t_stu;
update t_stu set stu_name=CONCAT(stu_name,"123") where stu_id=1001
update t_stu set stu_name=CONCAT(stu_name,stu_no) where stu_id=1001
SELECT * from t_stu;
INSERT into t_stu values(2002,'x5','20190705',30,'2019-07-05'),(2003,'x7','20190706',22,CURRENT_DATE());
INSERT into t_stu values (2004,'x9','20190707',24,CURRENT_TIMESTAMP());
select CURRENT_TIME();
SELECT stu_birth, YEAR(stu_birth),month(stu_birth),day(stu_birth) from t_stu;
select stu_birth,DATE_SUB(stu_birth,INTERVAL 30 day) from t_stu
select stu_birth,DATE_add(stu_birth,INTERVAL 5 day) from t_stu
select stu_birth,DATE_add(stu_birth,INTERVAL 5 HOUR) from t_stu
select stu_birth,CURRENT_DATE(),DATEDIFF(CURRENT_DATE(),stu_birth) from t_stu
select stu_birth,CURRENT_DATE(),YEAR(stu_birth),year(CURRENT_DATE()),(year(CURRENT_DATE())-YEAR(stu_birth)) b from t_stu
select stu_birth,EXTRACT(YEAR FROM stu_birth),EXTRACT(month FROM stu_birth),EXTRACT(day FROM stu_birth),
EXTRACT(HOUR FROM stu_birth),EXTRACT(MINUTE FROM stu_birth),EXTRACT(SECOND FROM stu_birth) from t_stu;
select 3*5 a;
select FLOOR(3.65),FLOOR(3.64),CEIL(3.65),CEIL(3.64),ROUND(3.654,2),ROUND(3.656,2)
select * from t_stu
select TRUNCATE(3.656,2) ,TRUNCATE(3.654,2)
show TABLES
select * from stu2;
select * from stu3;
delete from stu2;
TRUNCATE table stu2;
CREATE table copy_stu3 as select * from stu3;
select * from copy_stu3
CREATE table copy_stu31 as select stu_no from stu3;
select * from copy_stu31
CREATE table copy_stu32 as select * from stu3 where 1=2;
select * from copy_stu32;
select * from copy_stu31;
desc copy_stu32
select * from copy_stu3;
select * from copy_stu32;
update copy_stu3 set stu_name = null where stu_no=2
INSERT into copy_stu32(stu_no,stu_name) SELECT stu_no,IFNULL(stu_name,'马云') from copy_stu3
select * from copy_stu3 where stu_name = null;
select * from copy_stu3 where stu_name is null;
select * from t_stu;
select *,(
CASE
WHEN stu_age >=20 THEN '成年人'
WHEN stu_age >=10 and stu_age < 20 THEN '未成年'
ELSE '小孩'
END
)type from t_stu;
select * from t_stu;
select count(*) from t_stu;
select stu_id from t_stu UNION select count(*) from t_stu;
select stu_id,(select count(*) from t_stu) nums from t_stu ;
select 5*3
select * from t_stu;
select * from t_stu LIMIT 0,3;
select * from t_stu LIMIT 3,3;
select * from t_stu LIMIT 6,3;
select * from t_stu LIMIT 4,4;
select count(*) from t_stu;
select count(*),CEIL(count(*)/2) from t_stu;
select s.stu_id,s.stu_name,count(*) from t_stu s
INNER JOIN stu_course sc on s.stu_id = sc.stu_id
INNER JOIN course c on sc.cour_id = c.course_id
where s.stu_id > 1000
GROUP BY s.stu_id,s.stu_name
HAVING count(*) >=1
limit 3,3
SELECT t.stu_id,t.stu_name from
(select s.stu_id,s.stu_name from t_stu s
INNER JOIN stu_course sc on s.stu_id = sc.stu_id
INNER JOIN course c on sc.cour_id = c.course_id
where s.stu_id > 1000 limit 3,3) as t
GROUP BY t.stu_id,t.stu_name
HAVING count(*) >=1
2.创表多对多关系,sql的基本操作、分组统计、聚合
drop table student;
create table student(
stu_id int auto_increment PRIMARY key,
stu_name VARCHAR(25) COMMENT '学生姓名',
stu_no VARCHAR(25),
stu_birth TIMESTAMP
);
insert into student(stu_name,stu_no,stu_birth) values('lisi','2222',NOW());
insert into student(stu_name,stu_no,stu_birth) values('zhangsan','3333',NOW());
insert into student(stu_name,stu_no,stu_birth) values('wangwu','5555','2015-06-07');
insert into student(stu_name,stu_no,stu_birth) values('zhaoliu','6666','2015-06-07');
select * from student;
drop table if EXISTS course;
create table course(
cur_id int PRIMARY key,
cur_name VARCHAR(25),
create_time TIMESTAMP DEFAULT NOW() COMMENT '数据插入的时间'
)
insert into course(cur_id,cur_name) values (100,'java');
insert into course(cur_id,cur_name) values (200,'html');
insert into course(cur_id,cur_name) values (300,'javascript');
insert into course(cur_id,cur_name) values (400,'mysql');
insert into course(cur_id,cur_name) values (500,'oracle');
select * from course;
create table stu_course(
stu_id int,
cur_id int,
PRIMARY key(stu_id,cur_id),
CONSTRAINT FOREIGN key(stu_id) REFERENCES student(stu_id),
CONSTRAINT FOREIGN key(cur_id) REFERENCES course(cur_id)
);
INSERT into stu_course(stu_id,cur_id) values(1,100);
INSERT into stu_course(stu_id,cur_id) values(1,200);
INSERT into stu_course(stu_id,cur_id) values(2,100);
INSERT into stu_course(stu_id,cur_id) values(3,100);
INSERT into stu_course(stu_id,cur_id) values(3,200);
INSERT into stu_course(stu_id,cur_id) values(3,300);
INSERT into stu_course(stu_id,cur_id) values(3,400);
select * from stu_course;
select s.stu_id from student s where s.stu_name = 'lisi';
select sc.cur_id from stu_course sc ;
select sc.cur_id from stu_course sc where sc.stu_id = (select s.stu_id from student s where s.stu_name = 'lisi');
select c.cur_name from course c where c.cur_id in (
select sc.cur_id from stu_course sc where sc.stu_id = (select s.stu_id from student s where s.stu_name = 'lisi')
);
select s.*,sc.*,c.* from student s
INNER JOIN stu_course sc on s.stu_id = sc.stu_id
INNER join course c on sc.cur_id = c.cur_id
where s.stu_name = 'lisi'
select s.*,sc.* from student s
inner JOIN stu_course sc on s.stu_id = sc.stu_id
select s.*,sc.* from student s
left JOIN stu_course sc on s.stu_id = sc.stu_id
select c.*,sc.* from stu_course sc
right JOIN course c on c.cur_id = sc.cur_id
select * from student;
ALTER table student add COLUMN age int;
select count(*) '小于25的学生个数' from student where age <= 25;
select count(*),avg(age),max(age),min(age),sum(age) from student;
select * from student WHERE stu_id >= 1 ORDER BY age asc;
select * from student WHERE stu_id >= 1 ORDER BY age desc;
select * from student WHERE stu_id >= 1 ORDER BY age asc,stu_name desc;
SELECT count(*), age from student GROUP BY age;
SELECT count(*), age from student GROUP BY age HAVING age >18;
SELECT count(*), age from student GROUP BY age HAVING max(age) > 25;
SELECT count(*), age from student where stu_id >= 2 GROUP BY age HAVING age >=18 ;
SELECT count(*), age from student where stu_id >= 2 GROUP BY age HAVING age >=18 ORDER BY age desc;
SELECT count(*) from course;
select * from stu_course;
select stu_id,count(*) from stu_course GROUP BY stu_id;
select stu_id,count(*) from stu_course GROUP BY stu_id HAVING count(*) = (SELECT count(*) from course);
select * from student where stu_id in(
select stu_id from stu_course GROUP BY stu_id HAVING count(*) = (SELECT count(*) from course)
);
select s.*,t.* from student s INNER JOIN
( select stu_id,count(*) from stu_course GROUP BY stu_id HAVING count(*) = (SELECT count(*) from course) ) t
on s.stu_id = t.stu_id;