效果
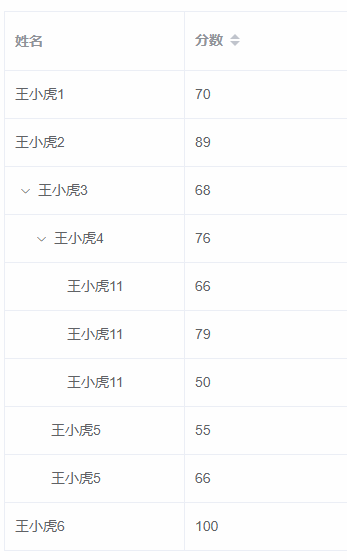
代码
// sortable必须设置为"custom",代表远程排序,不然会调用element自己的排序
export default {
name: "infinitList",
data() {
return {
tableData: [],
data: [
{
id: 1,
name: "王小虎1",
score: 70,
},
{
id: 2,
name: "王小虎2",
score: 89,
},
{
id: 3,
name: "王小虎3",
score: 68,
children: [
{
id: 31,
name: "王小虎4",
score: 76,
children: [
{
id: 34,
name: "王小虎11",
score: 66,
},
{
id: 35,
name: "王小虎11",
score: 79,
},
{
id: 36,
name: "王小虎11",
score: 50,
},
],
},
{
id: 32,
name: "王小虎5",
score: 55,
},
{
id: 33,
name: "王小虎5",
score: 66,
},
],
},
{
id: 4,
name: "王小虎6",
score: 100,
},
],
sortInfo: {
// 当前排序信息
prop: "", // 排序字段
order: "", // 排序类型
},
};
},
created() {
this.getData();
},
methods: {
/**
* 模拟接口获取列表数据
*/
getData() {
this.tableData = this.infiniteSort({
arr: this.deepClone(this.data),
...this.sortInfo,
});
},
/**
* 对象数组深拷贝
* @param {string} source 对象或数组
* @return {object} 新对象数组
*/
deepClone(source) {
const newObj = source.constructor === Array ? [] : {};
Object.keys(source).forEach((keys) => {
if (source[keys] && typeof source[keys] === "object") {
newObj[keys] = this.deepClone(source[keys]);
} else {
newObj[keys] = source[keys];
}
});
return newObj;
},
/**
* 无限级列表排序
* @param {Array} arr 列表数据
* @param {string} prop 排序字段
* @param {string} order 排序类型
* @return {Array} 排序后数组
*/
infiniteSort({ arr, prop, order }) {
if (prop && order && arr?.length > 0) {
const onSort = (arr) => {
arr.sort((a, b) => {
return order === "ascending"
? a[prop] - b[prop]
: b[prop] - a[prop];
});
};
this.recursive(arr, onSort);
}
return arr || [];
},
/**
* 递归函数
* @param {Array} arr 递归数据
* @param {Function} func 递归过程所要实现函数
*/
recursive(arr, func) {
func(arr);
for (const item of arr) {
if (
item.children &&
item.children.length > 1 &&
this.recursive(item.children, func)
) {
return true;
}
}
},
async handleSortChange({ column, prop, order }) {
this.sortInfo = { prop, order };
this.getData();
},
},
};
注意
sortable必须设置为"custom",代表远程排序,不然会调用element自己的排序